The microcontroller source program is as follows:
/********51 single chip microcomputer intelligent curtain design********/
/****************JJ Electronics Direct Store****************/
/***************original design***************/
#include #define uchar unsigned char #define uint unsigned int #define CS P3_0 //ADC0804 CS port #define RD P3_1 //ADC0804 RD port #define WR P3_2 //ADC0804 WR port #define g_kz P2_5 //digit control of digital tube #define s_kz P2_4 //Nude tube ten-digit control #define b_kz P2_3 //digital tube hundreds digit control #define q_kz P2_2 //code tube thousands control #define SMG_XS P0 //Digital tube display port #define AD_data P1 //ADC0804 output port #define Up P2_1 //Upper limit switch port #define Down P2_0 //Lower limit switch port #define Key1 P3_3 //Manual/auto switch button #define Key2 P3_4 //Timing/light control function switch button #define Key3 P3_5 //Set the key #define Key4 P3_6 //Add button (manual mode: open curtains timing mode: open curtains at a fixed time) #define Key5 P3_7 //Decrease button (manual mode: close curtains timing mode: close curtains at a fixed time) #define IA P2_7 //DC motor control port #define IB P2_6 //DC motor control port int adval; //ADC output variable int j; //define loop variable ij uchar flag=0; //Display flag (0: normal display 1: upper limit illumination setting (time adjustment) 2: lower limit illumination setting (minute adjustment) bit flag_gd=0; //Light control/timing flag (0: current light intensity 1: timing time) bit ms=0; //Mode (0: manual mode 1: automatic mode) bit move=0; //Timed time flag (0: time not yet reached 1: time reached) bit OFF_ON=0; //Motor forward and reverse rotation flag, indicating whether the curtain is open or closed (0: curtain closed 1: curtain open) bit Time_OFF_ON=0; //Open or close the curtains at the scheduled time (0: close the curtains at scheduled time 1: open the curtains at scheduled time) char hour=12,min=0;sec=0; //Define the time "hour, minute, second" variables. The default value is 12.00.00 when powered on. char num=0; //time base uint H_GM=240; //Define the upper limit light sensitivity setting variable, the default value is 200 when powered on uint L_GM=100; //Define the lower limit light sensitivity setting variable, the default value is 100 when powered on uchar t=1; //digital tube dynamic scanning delay parameter uchar code table[]={0xC0,0xF9,0xA4,0xB0,0x99,0x92,0x82,0xF8,0x80,0x90}; //Digital tube display array 0 1 2 3 4 5 6 7 8 9 void delay(uint time) //delay function { uint x,y; //define temporary variables xy for(x=time;x>0;x--) for(y=110;y>0;y--); //No operation } void Time_init() //Timer initialization { EA=1; TMOD=0x11; /*Timer 0 initialization (generates PWM to control motor speed)*/ TH0=0xf8; //timing 2ms TL0=0xcc; ET0=1; //Enable T0 interrupt TR0=0; //Do not start timer 0 first /*Timer 1 initialization (generating 50ms timing)*/ TH1=0x4c; //50ms TL1=0x00; ET1=1; TR1=0; //Do not start timer 1 first } void Key_cl() { static bit keybuf1=1; //Key1 value is temporarily stored, and the key scan value is temporarily saved static bit backup1=1; //Key1 value backup, save the previous scan value static bit keybuf2=1; //Key2 value is temporarily stored, and the key scan value is temporarily saved static bit backup2=1; //Key2 value backup, save the previous scan value static bit keybuf3=1; //Key3 value is temporarily stored, and the key scan value is temporarily saved static bit backup3=1; //Key3 value backup, save the previous scan value static bit keybuf4=1; //Key4 value is temporarily stored, and the key scan value is temporarily saved static bit backup4=1; //Key4 value backup, save the previous scan value static bit keybuf5=1; //Key5 value is temporarily stored, and the key scan value is temporarily saved static bit backup5=1; //Key5 value backup, save the previous scan value keybuf1=Key1; //temporarily store the current scan value of Key1 if(keybuf1!=backup1) //The current value of Key1 is not equal to the previous value, indicating that Key1 has an action { delay(1); //delay debounce if(keybuf1==Key1) //Judge whether the scan value of Key1 has changed, i.e. the key is jittering { if(backup1==1) //Key1 is pressed and effective { move=0; //Reset the time flag to 0 (time not up) TR0=0; //turn off timer 0 ms=~ms; // Mode inversion q_kz=1;b_kz=1;s_kz=1;g_kz=1;//Turn off the digital tube display IA=0;IB=0; //curtain stop } backup1=keybuf1; //Update the current value of backup Key1 for the next comparison } } if(ms==0) //manual mode { if(Key4==0) //When the curtain opening button is pressed { delay(10); //delay debounce if(Key4==0) // Check if the key is pressed { move=0; //Reset the time flag to 0 (time not up) OFF_ON=1; //curtains open TR0=1; //Start timer 0 SMG_XS=table[1]; //The digital tube displays "1" q_kz=1;b_kz=1;s_kz=1;g_kz=0; //unit display while(Key4==0); //Wait for the key to be released TR0=0; //turn off timer 0 IA=0;IB=0; //curtain stop q_kz=1;b_kz=1;s_kz=1;g_kz=1; //Turn off the digital tube } } if(Key5==0) //When the curtain closing button is pressed { delay(10); //delay debounce if(Key5==0) // Check if the key is pressed { move=0; //Reset the time flag to 0 (time not up) OFF_ON=0; //Curtains closed TR0=1; //Start timer 0 SMG_XS=table[0]; //The digital tube displays "0" q_kz=1;b_kz=1;s_kz=1;g_kz=0; //unit display while(Key5==0); //Wait for the key to be released TR0=0; //turn off timer 0 IA=0;IB=0; //curtain stop q_kz=1;b_kz=1;s_kz=1;g_kz=1; //Turn off the digital tube } } } else //In automatic mode { keybuf2=Key2; //temporarily store the current scan value of Key2 if(keybuf2!=backup2) //The current value of Key2 is not equal to the previous value, indicating that the Key2 key has an action { delay(1); //delay debounce if(keybuf2==Key2) //Judge whether the scan value of Key2 has changed, i.e. the key is jittering { if(backup2==1) //Key2 is pressed and effective { move=0; //Reset the time flag to 0 (time not up) flag_gd=~flag_gd; //Light control/timing flag inversion (0: current light intensity 1: timing time) } backup2=keybuf2; //Update the current value of backup Key2 for the next comparison } } keybuf3=Key3; //temporarily save the current scan value of Key3 if(keybuf3!=backup3) //The current value of Key3 is not equal to the previous value, indicating that Key3 has an action { delay(1); //delay debounce if(keybuf3==Key3) //Judge whether the scan value of Key3 has changed, i.e. the key is jittering { if(backup3==1) //Key3 is pressed and effective { move=0; //Reset the time flag to 0 (time not up) TR0=0; //turn off timer 0 flag++; if(flag==3) {flag=0;} //Keep flag between 0 and 2 } backup3=keybuf3; //Update the current value of backup Key3 for the next comparison } } if(flag==1) //Allow upper limit illumination setting (time adjustment) { keybuf4=Key4; //temporarily store the current scan value of Key4 if(keybuf4!=backup4) //The current value of Key4 is not equal to the previous value, indicating that Key4 has an action { delay(1); //delay debounce if(keybuf4==Key4) //Judge whether the scan value of Key4 has changed, i.e. the key is jittering { if(backup4==1) //Key4 is pressed and effective { if(flag_gd==0) //Light intensity setting allowed {
Previous article:51 single chip microcomputer realizes three-phase six-step stepper motor control
Next article:What does a nop in a 51 MCU mean?
Recommended ReadingLatest update time:2024-11-23 11:48
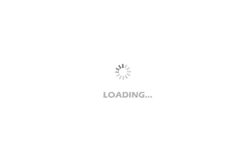
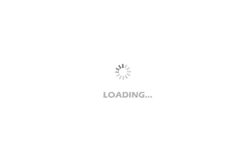
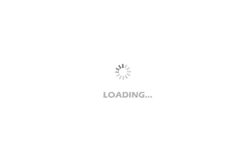
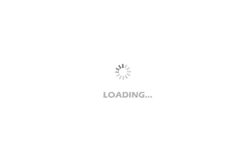
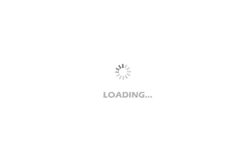
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- FAQ: Developing secure IoT edge-to-cloud applications on Linux using PKCS #11 and secure devices
- CC2640 TIRTOS adds IIC configuration
- ARM co-founder launches "SAVE ARM" campaign to intervene in NVIDIA acquisition
- How to convert PWM signal into analog signal
- Uf2 Introduction
- IPTV operation should adopt the third-party model and network transformation should be adapted to local conditions
- 【K210 Series】5. Calculation of Pi
- [AT32WB415 Review] 01 Development Board First Experience
- Wi-Fi-7?
- Analog Series: RF: In the 5G Era, RF Front-End Shines