Ⅰ. Write in front
I believe that those who have read the previous article "A Deeper Understanding of I2C Bus, Protocol and Application" have a certain understanding of I2C. That article is about I2C, using microcontroller IO to simulate I2C to achieve read and write operations.
This article will describe the hardware I2C read and write operations, that is, the clock and data transmission process implemented by the processor's own hardware I2C.
Ⅱ. STM8 Hardware I2C Knowledge
The I2C module of STM8S can not only receive and send data, but also convert data from serial to parallel data when receiving and from parallel to serial data when sending. Interrupts can be enabled or disabled. The interface is connected to the I2C bus through the data pin (SDA) and the clock pin (SCL). It allows connection to a standard (up to 100kHz) or fast (up to 400kHz) I2C bus.
1. Four modes of I2C
● Slave device sending mode
● Slave receiving mode
● Master device sending mode
● Master receiving mode
2. Main features of I2C
● Parallel bus/I2C bus protocol converter
● Multi-host function: This module can be used as both a master device and a slave device
● I2C master function
─ Generate start and stop signals
● I2C slave device function
─ Programmable I2C address detection
─ Stop position detection
● Generate and detect 7-bit/10-bit address and general call
● Support different communication speeds
─ Standard speed (up to 100 kHz)
─ Fast (up to 400 kHz)
● Status flag:
─ Transmitter/receiver mode flag
─ I2C bus busy flag
─ Arbitration failure in master mode
─ Error in ACK after address/data transmission
─ False start or stop condition detected
─ Data overload or underload when clock stretching function is disabled
● 3 types of interrupts
─ 1 communication interruption
─ 1 error interrupt
─ 1 wake-up interrupt
● Wake-up function
─ In slave mode, if an address match is detected, the MCU can be woken up from low power mode
● Optional clock stretching function
3. Operation sequence required by main mode
● Set the module’s input clock in the I2C_FREQR register to generate the correct timing
● Configure the clock control register
● Configure the rise time register
● Program the I2C_CR1 register to start the peripheral
● Set the START bit in the I2C_CR1 register to 1 to generate a start condition
● The input clock frequency of the I2C module must be at least:
● Standard mode: 1MHz
● Fast mode: 4MHz
III. Software Engineering Source Code
1. About the project
The engineering code provided in this article is based on the previous software project "STM8S-A04_UART basic data transmission and reception" and adds an I2C interface. The way to read and write EEPROM is different from the previous "simulated I2C reading and writing" method.
2. Hardware I2C initialization
void I2C_Initializes(void)
{
CLK_PeripheralClockConfig(CLK_PERIPHERAL_I2C, ENABLE);
I2C_Cmd(ENABLE);
I2C_Init(I2C_SPEED, I2C_SLAVE_ADDRESS7, I2C_DUTYCYCLE_2, I2C_ACK_CURR,
I2C_ADDMODE_7BIT, 16);
}
I2C_SPEED: I2C speed, usually 100K - 400K
I2C_SLAVE_ADDRESS7: slave device address. This address has no effect when used as a master device.
I2C_DUTYCYCLE_2: Fast mode
I2C_ACK_CURR: ACK
I2C_ADDMODE_7BIT: Device address bit number
16: Input clock (unit: M)
3.EEPROM_WriteByte writes one byte
Writing a byte is divided into 5 steps:
void EEPROM_WriteByte(uint16_t Addr, uint8_t Data)
{
while(I2C_GetFlagStatus(I2C_FLAG_BUSBUSY));
/* 1. Start */
I2C_GenerateSTART(ENABLE);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_MODE_SELECT));
/* 2. Device address/write*/
I2C_Send7bitAddress(EEPROM_DEV_ADDR, I2C_DIRECTION_TX);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED));
/* 3. Data address*/
#if (8 == EEPROM_WORD_ADDR_SIZE)
I2C_SendData((Addr&0x00FF));
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
#else
I2C_SendData((uint8_t)(Addr>>8));
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
I2C_SendData((uint8_t)(Addr&0x00FF));
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
#endif
/* 4. Write one byte of data*/
I2C_SendData(Data);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
/* 5. Stop */
I2C_GenerateSTOP(ENABLE);
}
4.EEPROM_ReadByte reads one byte
Reading a byte requires two more steps than writing a byte because when reading, there is an extra switching process from writing address to reading data.
void EEPROM_ReadByte(uint16_t Addr, uint8_t *Data)
{
while(I2C_GetFlagStatus(I2C_FLAG_BUSBUSY));
/* 1. Start */
I2C_GenerateSTART(ENABLE);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_MODE_SELECT));
/* 2. Device address/write*/
I2C_Send7bitAddress(EEPROM_DEV_ADDR, I2C_DIRECTION_TX);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_TRANSMITTER_MODE_SELECTED));
/* 3. Data address*/
#if (8 == EEPROM_WORD_ADDR_SIZE)
I2C_SendData((Addr&0x00FF));
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
#else
I2C_SendData((uint8_t)(Addr>>8));
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
I2C_SendData((uint8_t)(Addr&0x00FF));
while(!I2C_CheckEvent(I2C_EVENT_MASTER_BYTE_TRANSMITTED));
#endif
/* 4. Restart */
I2C_GenerateSTART(ENABLE);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_MODE_SELECT));
/* 5. Device address/read*/
I2C_Send7bitAddress(EEPROM_DEV_ADDR, I2C_DIRECTION_RX);
while(!I2C_CheckEvent(I2C_EVENT_MASTER_RECEIVER_MODE_SELECTED));
/* 6. Read one byte of data*/
I2C_AcknowledgeConfig(I2C_ACK_NONE);
while(I2C_GetFlagStatus(I2C_FLAG_RXNOTEMPTY) == RESET);
*Data = I2C_ReceiveData();
/* 7. Stop */
I2C_GenerateSTOP(ENABLE);
}
IV. Download
STM8S information:
http://pan.baidu.com/s/1o7Tb9Yq
Two versions of software source code project (STM8S-A10_I2C read and write EEPROM (hardware)):
http://pan.baidu.com/s/1c2EcRo0
Tip: If the network disk link is invalid, you can check the update link in the "bottom menu" of the WeChat public account.
Previous article:A brief discussion of I2C bus (IV) -- Example of IIC driver file for STM8
Next article:STM8 I/O port simulates I2C
Recommended ReadingLatest update time:2024-11-16 11:46
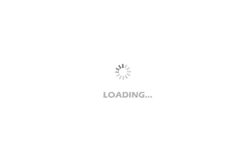
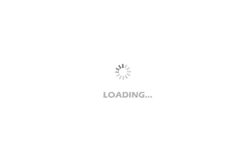
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Semantic Segmentation for Autonomous Driving: Model Evaluation, Dataset Generation, Viewpoint Comparison, and Real-time Performance
-
Arduino Development Guide: STM32
-
Arduino Advanced Development Definitive Guide (Original Book 2nd Edition)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Is it a tax on IQ?
- CCS compilation error: unresolved symbols remain solution
- Fiber Optic Project Overview
- Bluetooth module problem
- Switching Power Supply Interest Group 13th Task
- Is the ST website down? Or is it crashing?
- How to Design a Low-Cost Bluetooth Music Playback System
- I would like to ask which other manufacturers have single-pole TMR switches, except MDT (only a model, but out of stock)?
- PIC16F684 IO port output problem
- The difference between building a system and building a module