1. STM8S external interrupt wake-up
First understand the interrupt resources of STM8S
Let's look at the interrupt management of STM8S. STM8S uses software priority and hardware priority to control the response of an interrupt. The software priority is compared first, and the hardware priority is compared only when the software priority is consistent. Since the hardware priority is unique, it ensures that only one interrupt will be processed at a certain time.
To use external interrupts, simply configure the EXTI_CR1 register and set the software priority of the main program to 0. By default, the software priority of the main program is set to 3 since reset, which is the highest software priority. Only TRAP, TLI, and RESET interrupts can interrupt, and the rest of the interrupts will not be responded to.
In order to prevent the interruption process from being interrupted by other high-priority interrupts, the current priority can be set to the highest level 3.
code show as below:
main.c code
//EXTI_CR1|=EXTI_CR1_PBIS_R; //PB5 TRINT high level trigger
EXTI_CR1|=EXTI_CR1_PCIS_R; //PC3 rising edge trigger
//#define EXTI_CR1_PCIS_R (1<<4)
RIM; //Open global interrupt, this sentence is required, otherwise it will only respond to non-maskable interrupts
//#define HALT _asm("halt")
//#define RIM _asm("rim")
//#define SIM _asm("sim")
GPIO_Init(GPIOC,TRINT,GPIO_MODE_IN_PU_IT); //Enable the corresponding IO port interrupt
stm8s_it.c code
//Transmit and receive interrupt (PC3) BJ8F101
@far @interrupt void EXTI_PORTC_IRQHandler(void)
{
//Used as a receive interrupt, please note that PSB_D, TRRDY_U will generate an interrupt, TRINT will be pulled high
if(cur_mode==RX_MODE)
{
//To exclude the first time, you can check whether PSB is high, high means it is Active Mode
if(PB_ODR&PSB)
{
ss=1;
}
}else
{
}
return;
}
In fact, the rim command only reduces the software priority of the main program to 0 so that it can be interrupted. Naturally, the sim command is suitable for raising the software priority to level 3.
Also, please note that if there are several different interrupts on a port (interrupts occur on PC3, PC4, and PC5), you can only determine which IO port is interrupted based on some other flags. In fact, this chip does not have an interrupt flag.
Another problem is that the system cannot jump out after entering the interrupt. It is likely that the instruction execution order is incorrect. For example, the rim instruction is executed first, and then the GPIO port interrupt is enabled. The corresponding IO port is set to the rising edge trigger. It is found that the system cannot jump out after entering the interrupt. The reason is that the IO port may be in an uncertain state after reset, and it will be responded immediately after executing rim. By default, both the rising and falling edges of the IO port will trigger the interrupt.
External interrupts can wake up the system, such as:
That is to say, after the halt instruction is executed in the main function, the MCU enters the halt mode (without enabling AWU), and the external interrupt can wake up the MCU from the halt mode. You can use the emulator to set breakpoints for verification, or you can use the LED light.
2. AWU automatic wakeup
In addition to the wait mode and stop mode, STM8S also provides an active stop mode. To use the active stop mode, you only need to enable AWU.
#ifdef ENABLE_AWU
void Init_AWU(void)
{
CLK_PCKENR2=CLK_PCKENR2_AWU; //Enable AWU clock
//#define AWU_AWUTB_1S 0x0C /*500ms ~ 1s*/
//#define AWU_AWUTB_2S 0x0D /*1s ~ 2s*/
AWU_TBR=AWU_AWUTB_1S; //AWU_AWUTB_2S; //1~2s
AWU_APR=0x3E; //Frequency division
AWU_CSR|=0x10; //AWU enable
#ifdef POWER_LEVEL_1 //Power consumption 1, most power-saving
CLK_ICKR|=CLK_ICKR_REGAH; //In active shutdown mode (AWU enabled), turn off the voltage regulator to save power
FLASH_CR1|=FLASH_CR1_AHALT; // Flash is powered off in active halt mode. By default, it is powered off only in halt mode, but the wake-up time is increased to microseconds.
#endif /*ENABLE POWER_LEVEL_1*/
}
#endif /*END ENABLE_AWU*/
Then after the halt instruction is executed in the main function, the MCU will continue to run until the AWU wakes up. In addition, the AWU timed wake-up of STM8S provides a maximum delay of about 30 seconds.
3. Window Watchdog
STM8S provides two types of watchdogs. I personally feel that the window watchdog can solve the contradiction between the stop mode and the use of the watchdog, so I am only interested in the window watchdog.
code show as below:
#ifdef ENABLE_WWDG
void Init_WWDG(void) //Initialize window watchdog
{
//The window watchdog resets when the count value drops to 0x3F, and the watchdog cannot be fed when it is greater than the window value, otherwise it will reset
WWDG_WR = 0x60; //Watchdog window value, the window value must be above 0x3F, but must be less than the count value, otherwise the watchdog cannot be fed
WWDG_CR = 0x7F; //Watchdog count value
WWDG_CR |= 0x80; //Enable window watchdog
//4Mhz main frequency, count value 0x7F, maximum extension time is (64 * (12288 / 4000000)) = 196ms
}
void Free_WWDG(void)
{
if ((WWDG_CR & 0x7F) < WWDG_WR) //The dog can be fed only if it is less than the window value
WWDG_CR |= 0x7F; //Re-feed the dog
}
#endif /*END ENABLE_WWDG*/
The timer cannot be used to feed the watchdog regularly. After the MCU hangs up, the timer circuit may still be working, so the watchdog loses its meaning.
The independent watchdog is not affected by the MCU stop mode or other modes. Its clock is independent, so entering the stop mode will cause a system reset.
Summarize:
1. When using interrupts, you need to pay attention to the priority setting and the corresponding IO port enable trigger conditions.
2. The use of AWU is relatively simple, you just need to make sure the clock is turned on.
3. Pay attention to feeding the window watchdog and setting the delay. The specific delay time can be calculated using step = 12288 / fclk_wwdg_ck.
Previous article:STM8S103 independent button detection
Next article:STM8S operates internal EEPROM
Recommended ReadingLatest update time:2024-11-16 04:23
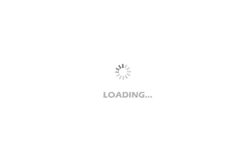
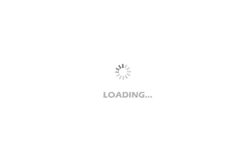
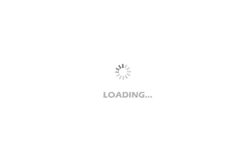
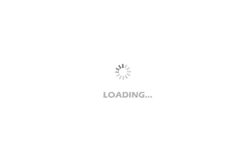
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Choosing the Correct Thermistor for Your Temperature Sensor
- EEprom write speed is slow
- CircuitPython 7.0.0 released
- They are all six non-gates. This power supply can be used even if it is connected reversely, but it cannot be replaced.
- PCB layout
- About the test of power supply Wenbo
- [N32L43x Review] 3. Using serial port receive interrupt and idle interrupt to realize variable length data reception
- EEWORLD University Hall----Live Replay: Melexis explains the implementation and technical support of semiconductor R&D functional safety
- MSP430F149 serial RS485 interface
- Optocoupler replacement