Switching the clock source mainly involves the following registers: main clock switching register CLK_SWR and switching control register CLK_SWCR.
The reset value of the master clock switch register is 0xe1, which means switching to the internal high-speed RC oscillator. When 0xb4 is written to the register, it means switching to the external high-speed crystal oscillator.
In the actual switching process, the SWEN (bit 1) in the switching control register should be set to 1 first, then the value of CLK_SWCR should be set, and finally, it should be determined whether the SWIF flag in the switching control register is switched successfully.
The following experimental program first switches the main clock source to an external crystal oscillator with an oscillation frequency of 8MHZ, and then quickly flashes the LED indicator. Next, the main clock source is switched to the internal oscillator with an oscillation frequency of 2MHZ, and then the LED indicator flashes slowly. By observing the flashing frequency of the LED indicator, it can be seen that the same loop code changes the flashing frequency and duration due to the change of the main clock source.
Still using ST's development tools, generate a C language program framework, and then modify main.c. The modified code is as follows.
// Program description: Change the CPU running speed by switching the CPU's main clock source
#include "STM8S207C_S.h"
// Function: Delay function
// Input parameter: ms -- the number of milliseconds to delay, assuming the CPU frequency is 2MHZ
// Output parameters: None
// Return value: None
// Note: None
void DelayMS(unsigned int ms)
{
unsigned char i;
while(ms != 0)
{
for(i=0;i<250;i++)
{
}
for(i=0;i<75;i++)
{
}
ms--;
}
}
main()
{
int i;
// Set PD3 to push-pull output to drive the LED
PD_DDR = 0x08;
PD_CR1 = 0x08;
PD_CR2 = 0x00;
// Start the external high speed crystal oscillator
CLK_ECKR = 0x01; // Allow external high speed oscillator to work
while((CLK_ECKR & 0x02) == 0x00); // Wait for the external high-speed oscillator to be ready
// Note that after reset, the CPU clock source comes from the internal RC oscillator
for(;;) // Enter an infinite loop
{
// Next, switch the CPU clock source to an external high-speed crystal oscillator. The frequency on the development board is 8MHZ.
// Through the LED, we can see that the speed of program running has indeed been significantly improved
CLK_SWCR = CLK_SWCR | 0x02; // SWEN <- 1
CLK_SWR = 0xB4; // Select the high-speed oscillator outside the chip as the main clock
while((CLK_SWCR & 0x08) == 0); // Wait for the switch to succeed
CLK_SWCR = CLK_SWCR & 0xFD; // Clear the switch flag
for(i=0;i<10;i++) //LED flashes 10 times at high speed
{
PD_ODR = 0x08;
DelayMS(100);
PD_ODR = 0x00;
DelayMS(100);
}
// Next, switch the CPU clock source to the internal RC oscillator. Since the reset value of CLK_CKDIVR is 0x18
// Therefore, the 16MHZ RC oscillator must be divided by 8 before it can be used as the main clock, so the frequency is 2MHZ
// Through the LED, it can be seen that the speed of program running has indeed dropped significantly
CLK_SWCR = CLK_SWCR | 0x02; // SWEN <- 1
CLK_SWR = 0xE1; // Select HSI as the main clock source
while((CLK_SWCR & 0x08) == 0); // Wait for the switch to succeed
CLK_SWCR = CLK_SWCR & 0xFD; // Clear the switch flag
for(i=0;i<10;i++) //LED flashes 10 times at low speed
{
PD_ODR = 0x08;
DelayMS(100);
PD_ODR = 0x00;
DelayMS(100);
}
}
}
Previous article:STM8 UART
Next article:Use of STC12C5A16S2 dual serial ports
Recommended ReadingLatest update time:2024-11-16 13:41
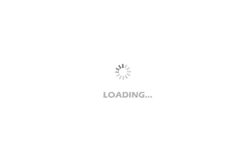
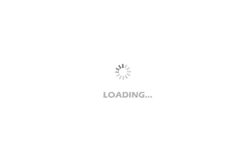
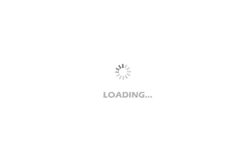
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- STM32F7 CubeMX automatically generates ADC self-calibration program
- The applications of TI audio chips used in smart speakers are revealed.
- ART-Pi evaluation application RL-TCPnet realizes Ethernet transceiver function and DTU
- Is it just simple impedance control? Answer with real examples!
- Common integrator applications: signal processing, sensor conditioning, signal generation, filtering
- [NXP Rapid IoT Review] Hello Sensor
- Find TI, NXP, ALTERA, XILINX, ST, Microchip factory stock chips
- Record the use of TM1668 driver
- Newbie help, ESP8266 program problem
- GaN Applications in RF and Electronics