How to write LCD driver
1. Allocate an fb_info structure using the framebuffer_alloc() function
2. Set the parameters in fb_info,
1) Fixed parameters (fix)
2) Variable parameters (var)
3) Set the operation function fbops
4) Other settings such as pseudo_palette and screen_size
5) Allocate video memory dma_alloc_writecombine(), the returned value is the virtual address
3. Register fb_info using register_framebuffer
4. Hardware related operations, LCD registers, etc.
Test Methods:
virtual machine:
1. Make menuconfig to remove the original driver
->Device Drivers
->Graphics support
2、make uImage make modules cp arch/arm/boot/uImage /work/nfs_root/mydriver/uImage_nolcd cp drivers/video/cfb*.ko /work/nfs_root/mydriver/ Development Board: 3. Start the development board using the new uImage nfs 30000000 192.168.34.251:/work/nfs_root/uImage_nolcd bootm 30000000 4. Load the driver insmod cfbfillrect.ko insmod cfbimgblt.ko insmod cfbcopyarea.ko insmod lcd.ko echo hello > /dev/tty1 //You can see hello on the LCD cat lcd.ko > /dev/fb0 //Display screen 5. Modify inittab vi /etc/inittab Add a row tty1::askfirst:-/bin/sh reboot insmod cfbfillrect.ko insmod cfbimgblt.ko insmod cfbcopyarea.ko insmod lcd.ko insmod buttons.ko //Driver in the input subsystem Function description: s3c_lcd->screen_base = dma_alloc_writecombine(NULL, s3c_lcd->fix.smem_len, &s3c_lcd->fix.smem_start, GFP_KERNEL); meaning: s3c_lcd->screen_base: The virtual starting address of the memory, which is used by the kernel to operate the allocated memory NULL: can be specified in the platform initialization, mainly used for parameters such as dma_mask, refer to framebuffer s3c_lcd->fix.smem_len: actual allocation size, pass in dma_map_size &s3c_lcd->fix.smem_start : Returns the physical address of the memory, which can be used by DMA. s3c_lcd->screen_base and s3c_lcd->fix.smem_start are one-to-one corresponding. s3c_lcd->screen_base is the virtual address, s3c_lcd->fix.smem_start is the bus address. Any operation will change the write buffer content. lcd.c #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include #include static int s3c_lcdfb_setcolreg(unsigned int regno, unsigned int red, unsigned int green, unsigned int blue, unsigned int transp, struct fb_info *info); struct lcd_regs { unsigned long lcdcon1; unsigned long lcdcon2; unsigned long lcdcon3; unsigned long lcdcon4; unsigned long lcdcon5; unsigned long lcdsaddr1; unsigned long lcdsaddr2; unsigned long lcdsaddr3; unsigned long redlut; unsigned long greenlut; unsigned long bluelut; unsigned long reserved[9]; unsigned long dithmode; unsigned long tpal; unsigned long lcdintpnd; unsigned long lcdsrcpnd; unsigned long lcdintmsk; unsigned long lpcsel; }; static struct fb_ops s3c_lcdfb_ops = { .owner = THIS_MODULE, .fb_setcolreg = s3c_lcdfb_setcolreg, .fb_fillrect = cfb_fillrect, .fb_copyarea = cfb_copyarea, .fb_imageblit = cfb_imageblit, }; static struct fb_info *s3c_lcd; static volatile unsigned long *gpbcon; static volatile unsigned long *gpbdat; static volatile unsigned long *gpccon; static volatile unsigned long *gpdcon; static volatile unsigned long *gpgcon; static volatile struct lcd_regs* lcd_regs; static u32 pseudo_palette[16]; /* from pxafb.c */ static inline unsigned int chan_to_field(unsigned int chan, struct fb_bitfield *bf) { chan &= 0xffff; chan >>= 16 - bf->length; return chan << bf->offset; } static int s3c_lcdfb_setcolreg(unsigned int regno, unsigned int red, unsigned int green, unsigned int blue, unsigned int transp, struct fb_info *info) { unsigned int val; if (regno > 16) return 1; /* Use the three primary colors red, green, and blue to construct val */ val = chan_to_field(red, &info->var.red); val |= chan_to_field(green, &info->var.green); val |= chan_to_field(blue, &info->var.blue); //((u32 *)(info->pseudo_palette))[regno] = val; pseudo_palette[kingdom] = val; return 0; } static int lcd_init(void) { /* 1. Allocate a fb_info **************************************************/ s3c_lcd = framebuffer_alloc(0, NULL); /* 2. Setup */ /* 2.1 Set fixed parameters*/ strcpy(s3c_lcd->fix.id, "mylcd"); s3c_lcd->fix.smem_len = 480*272*16/8; s3c_lcd->fix.type = FB_TYPE_PACKED_PIXELS; s3c_lcd->fix.visual = FB_VISUAL_TRUECOLOR; /* TFT */ s3c_lcd->fix.line_length = 480*2; /* 2.2 Setting variable parameters*/ s3c_lcd->var.xres = 480; s3c_lcd->var.yres = 272; s3c_lcd->var.xres_virtual = 480; s3c_lcd->var.yres_virtual = 272; s3c_lcd->var.bits_per_pixel = 16; /* RGB:565 */ s3c_lcd->var.red.offset = 11; s3c_lcd->var.red.length = 5; s3c_lcd->var.green.offset = 5; s3c_lcd->var.green.length = 6; s3c_lcd->var.blue.offset = 0; s3c_lcd->var.blue.length = 5; s3c_lcd->var.activate = FB_ACTIVATE_NOW; /* 2.3 Setting operation function*/ s3c_lcd->fbops = &s3c_lcdfb_ops; /* 2.4 Other settings*/ s3c_lcd->pseudo_palette = pseudo_palette; //s3c_lcd->screen_base = ; /* Virtual address of video memory*/ s3c_lcd->screen_size = 480*272*16/8; /* 3. Hardware related operations*/ /* 3.1 Configure GPIO for LCD */ gpbcon = ioremap(0x56000010, 8); //ioremap function: map an IO address space to the kernel's virtual address space for easy access; gpbdat = gpbcon+1; gpccon = ioremap(0x56000020, 4); gpdcon = ioremap(0x56000030, 4); gpgcon = ioremap(0x56000060, 4); *gpccon = 0xaaaaaaaa; /* GPIO pins are used for VD[7:0], LCDVF[2:0], VM, VFRAME, VLINE, VCLK, LEND */ *gpdcon = 0xaaaaaaaa; /* GPIO pins are used for VD[23:8] */ *gpbcon &= ~(3); /*GPB0 is set as output pin*/ *gpbcon |= 1; *gpbdat &= ~1; /* Output low level*/ *gpgcon |= (3<<8); /* GPG4 is used as LCD_PWREN */ /* 3.2 Set the LCD controller according to the LCD manual, such as the frequency of VCLK, etc.*/ lcd_regs = ioremap(0x4D000000, sizeof(struct lcd_regs)); /* bit[17:8]: VCLK = HCLK / [(CLKVAL+1) x 2], LCD manual P14 * 10MHz(100ns) = 100MHz / [(CLKVAL+1) x 2] * CLKVAL = 4 * bit[6:5]: 0b11, TFT LCD * bit[4:1]: 0b1100, 16 bpp for TFT * bit[0] : 0 = Disable the video output and the LCD control signal. */ lcd_regs->lcdcon1 = (4<<8) | (3<<5) | (0x0c<<1); #if 1 /* Time parameters in vertical direction * bit[31:24]: How long does it take after VBPD and VSYNC to send the first line of data? * LCD manual T0-T2-T1=4 * VBPD=3 * bit[23:14]: how many lines, 320, so LINEVAL=320-1=319 * bit[13:6] : VFPD, how long does it take to send VSYNC after sending the last line of data? * LCD manual T2-T5=322-320=2, so VFPD=2-1=1 * bit[5:0] : VSPW, pulse width of VSYNC signal, LCD manual T1=1, so VSPW=1-1=0 */ //lcd_regs->lcdcon2 = (3<<24) | (319<<14) | (1<<6) | (0<<0); lcd_regs->lcdcon2 = (1<<24) | (271<<14) | (1<<6) | 9; //The values of some bits of the same model of LED screens from the same manufacturer are the same, check the manual for details /* Horizontal time parameters * bit[25:19]: HBPD, how long does it take after VSYNC to send the first line of data * LCD manual T6-T7-T8=17 * HBPD=16 * bit[18:8]: number of columns, 240, so HOZVAL=240-1=239 * bit[7:0] : HFPD, how long does it take to send HSYNC after sending the last pixel data in the last line? * LCD manual T8-T11=251-240=11, so HFPD=11-1=10 */ //lcd_regs->lcdcon3 = (16<<19) | (239<<8) | (10<<0); lcd_regs->lcdcon3 = (1<<19) | (479<<8) | (1); /* Horizontal synchronization signal * bit[7:0] : HSPW, pulse width of HSYNC signal, LCD manual T7=5, so HSPW=5-1=4 */ //lcd_regs->lcdcon4 = 4; lcd_regs->lcdcon4 = 40; lcd_regs->lcdcon5 =1<<11|1<<9|1<<8|1<<1; #else lcd_regs->lcdcon2 = S3C2410_LCDCON2_VBPD(5) | S3C2410_LCDCON2_LINEVAL(319) | S3C2410_LCDCON2_VFPD(3) | S3C2410_LCDCON2_VSPW(1); lcd_regs->lcdcon3 = S3C2410_LCDCON3_HBPD(10) | S3C2410_LCDCON3_HOZVAL(239) | S3C2410_LCDCON3_HFPD(1); lcd_regs->lcdcon4 = S3C2410_LCDCON4_MVAL(13) | S3C2410_LCDCON4_HSPW(0); #endif /* Polarity of the signal * bit[11]: 1=565 format * bit[10]: 0 = The video data is fetched at VCLK falling edge * bit[9]: 1 = HSYNC signal is to be inverted, i.e. low level is valid * bit[8]: 1 = VSYNC signal is to be inverted, i.e. low level is valid * bit[6]: 0 = VDEN does not need to be inverted * bit[3] : 0 = PWREN output 0 * bit[1] : 0 = BSWP * bit[0] : 1 = HWSWP 2440 manual P413 */ // lcd_regs->lcdcon5 = (1<<11) | (0<<10) | (1<<9) | (1<<8) | (1<<0); /* 3.3 Allocate video memory (framebuffer) and tell the LCD controller the address*/ s3c_lcd->screen_base = dma_alloc_writecombine(NULL, s3c_lcd->fix.smem_len, &s3c_lcd->fix.smem_start, GFP_KERNEL); lcd_regs->lcdsaddr1 = (s3c_lcd->fix.smem_start >> 1) & ~(3<<30); lcd_regs->lcdsaddr2 = ((s3c_lcd->fix.smem_start + s3c_lcd->fix.smem_len) >> 1) & 0x1fffff; lcd_regs->lcdsaddr3 = 0<<11|(480*2/2); /* Length of a line (unit: 2 bytes) */ //s3c_lcd->fix.smem_start = xxx; /* Physical address of video memory*/ /* Start LCD */ lcd_regs->lcdcon1 |= (1<<0); /* Enable LCD controller */
Previous article:Touch screen driver-JZ2440
Next article:Button-LED
Recommended ReadingLatest update time:2024-11-16 11:49
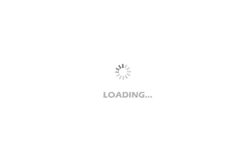
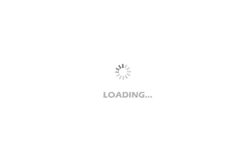
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Multimodal perception parameterized decision making for autonomous driving
-
Power Integrated Circuit Technology Theory and Design (Wen Jincai, Chen Keming, Hong Hui, Han Yan)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University ---- Learn myRIO with me
- Can 868.3MHZ, used as a remote control, go through walls?
- How are diodes classified?
- I want to discuss the direction of work
- MSP430F5438 study notes initialization XT1
- Classic MATLAB simulation model for parameter identification of three-phase asynchronous motor
- PCB popular data download collection
- Infrared thermometer calibration method
- [Environmental Expert’s Smart Watch] Part 14: APP data visualization!
- CCTV comments on Hongmeng, Huawei announces the latest progress, is Google getting anxious? - Focus, do you want to play with the Hongmeng development board?