Use the IO port query method to realize the key control of the LED's light on and off
#include "s3c24xx.h"
#define LED_ON 0
#define LED_OFF 1
#define KEY1_POS (0) //The position of key 1 on the IO port. Here it is GPF0 port, so the value is 0
#define KEY2_POS (2) //The position of key 2 in the IO port, here is GPF2 port, the value is
#define KEY3_POS (3)//GPG3 EINT11
#define LED1_POS (1<<4) //The position of LED1 in the IO port, here is GPF4 port, so the value is 4
#define LED2_POS (1<<5) //LED2 is located at the IO port, here is GPF5 port, the value is
#define LED3_POS (1<<6) //GPF6
//Software related, not related to hardware buffe
#define OFS_KEY1 (0) //The state of key 1 is saved in the variable, here it is saved in the 0th position of the variable
#define OFS_KEY2 (1)//
#define OFS_KEY3 (2)
#define GPF4_out (1<<(4*2)) //Set the 8th and 9th bits of GPFCON, that is, set the GPF4 bit output
#define GPF5_out (1<<(5*2)) //Set the 10th and 11th bits of GPFCON, that is, set the GPF5 bit output
#define GPF6_out (1<<(6*2)) //Set the 12th and 13th bits of GPFCON, that is, set the GPF6 bit output
#define GPF7_out (1<<(7*2))
#define hrdReadKey1 ((GPFDAT>>KEY1_POS)&0X01) //Read the status of key 1
#define hrdReadKey2 ((GPFDAT>>KEY2_POS)&0X01) //Read the status of key 2
#define hrdReadKey3 ((GPGDAT>>KEY3_POS)&0X01) //Read the status of key 2
#define hrdLed1On (GPFDAT &= (~LED1_POS)) // Make GPF4 output 0
#define hrdLed1Off (GPFDAT |= (LED1_POS)) // Make GPF4 output 1
#define hrdLed2On (GPFDAT &= (~LED2_POS)) // Make GPF5 output 0
#define hrdLed2Off (GPFDAT |= (LED2_POS)) // Make GPF5 output 1
#define hrdLed3On (GPGDAT &= (~LED3_POS)) // Make GPG6 output 0
#define hrdLed3Off (GPGDAT |= (LED3_POS)) // Make GPG6 output 1
typedef union
{
unsigned long byte;
struct
{
unsigned long key1 :1;
unsigned long key2 :1;
unsigned long key3 :1;
unsigned long notused :5;
}bits;
}mmiFlag_t;
mmiFlag_t mmiFlag;
#define mmiFlag_Key1 mmiFlag.bits.key1 //Judge whether it is the first time to process after the key is pressed
#define mmiFlag_Key2 mmiFlag.bits.key2 //Judge whether it is the first time to process after the key is pressed
#define mmiFlag_Key3 mmiFlag.bits.key3 //Judge whether it is the first time to process after the key is pressed
typedef union
{
unsigned long byte;
struct
{
unsigned long led1 :1;
unsigned long led2 :1;
unsigned long led3 :1;
unsigned long notused :5;
}bits;
}ledStatus_t;
ledStatus_t ledStatus;
#define led1Status ledStatus.bits.led1
#define led2Status ledStatus.bits.led2
#define led3Status ledStatus.bits.led3
volatile unsigned long dgtInBuf=1; //Save the switch input status
volatile unsigned long dgtInPre; //Pre-store switch input status
volatile unsigned long dgtCount; //delay count of switch input
// Initialize IO port
void ioInitialize_IO(void)
{
GPFCON |= GPF4_out; // Set GPF4, GPF5, GPF6 as output ports, bit [9:8] = 0b01
GPFCON &=(~GPF5_out);
GPFCON |= GPF5_out;
GPFCON |= GPF6_out;
GPFDAT = 0x00000000; //GPF4 outputs 0, LED1 lights up
}
//Refresh the IO port, read or write the IO port operation here
void ioRefresh_IO(void)
{
unsigned long buf=0;
if(hrdReadKey1==0) //Read the input status of key 1
buf |= (1< buf |= (1< buf |= (1< } /*Delay*/ void wait(unsigned long dly) { for(;dly>0;dly--); } /* *******************************Key processing function********************************************* This is achieved by querying the IO port, not interrupting it. */ void mmiProcess_Key1(void) { //mmiFlag_Key1 determines whether the key is pressed for the first time, and does not determine whether it is pressed next time until it is released if((dgtInBuf&(1< mmiFlag_Key1 = 1; //The flag is set to 1 to prevent the key from being released and entering this judgment body again if(led1Status==0) //LED light status flip { led1Status = 1; hrdLed1Off; } else { led1Status = 0; hrdLed1On; } } else if((dgtInBuf&(1< mmiFlag_Key1 = 0; //Set the flag to 0 and wait for the next key press } } void mmiProcess_Key2(void) { //mmiFlag_Key2 determines whether the key is pressed for the first time, and does not determine whether it is pressed next time until it is released if((dgtInBuf&(1< mmiFlag_Key2 = 1; //The flag is set to 1 to prevent the key from being released and entering this judgment body again if(led2Status==0) //LED light status flip { led2Status = 1; hrdLed2Off; } else { led2Status = 0; hrdLed2On; } } else if((dgtInBuf&(1< mmiFlag_Key2 = 0; //Set the flag to 0 and wait for the next key press } } void mmiProcess_Key3(void) { //mmiFlag_Key3 determines whether the key is pressed for the first time, and does not determine whether it is pressed next time until it is released if((dgtInBuf&(1< mmiFlag_Key3 = 1; //The flag is set to 1 to prevent the key from being released and entering this judgment body again if(led3Status==0) //LED light status flip { led3Status = 1; hrdLed3Off; } else { led3Status = 0; hrdLed3On; } } else if((dgtInBuf&(1< mmiFlag_Key3 = 0; //Set the flag to 0 and wait for the next key press } } void mmiHandle_MMI(void) { if(dgtInBuf!=dgtInPre)//Judge whether the key input status is consistent with the last time { dgtInPre = dgtInBuf; //Assign this state to the Pre variable dgtCount = 0; //Count cleared to 0 } else //If the key input status is the same as last time { dgtCount++; // count plus 1 if(dgtCount>=5) //A delay of 5*1ms=5ms is performed. If the status is consistent for 5 times in a row, the input is considered valid. { dgtCount = 0; mmiProcess_Key1(); mmiProcess_Key2(); mmiProcess_Key3(); } } } int main() { ioInitialize_IO(); for(;;) { wait(1000); // 1ms delay ioRefresh_IO(); mmiHandle_MMI(); } return 0; }
Previous article:LCD Driver-JZ2440
Next article:Driver-button-interrupt mode
Recommended ReadingLatest update time:2024-11-16 13:56
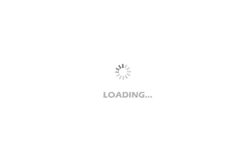
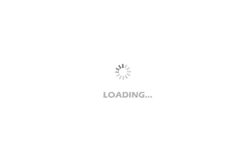
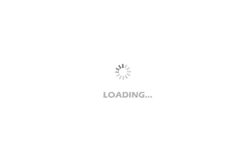
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The microcontroller drives the P-MOS to conduct abnormally through the NPN transistor?
- How to support domestic suppliers who use tricks?
- Thank you for being there + seeking change
- RVB2601 First Experience
- If the Lingte LT3789 is to output 12V10A, can it be directly connected in parallel with MOS?
- MSP430 and ATK-NEO-6M GPS module
- Saw a picture on the official account
- How much do you know about 5G antennas?
- Please help me with this circuit
- Understanding the energy storage function of decoupling capacitors