Overview:
When using the STM8L101F3 microcontroller and SPI communication to read the data of the three-axis sensor ADXL362, a series of problems occurred. I will make a note here and hope it can give you a small reference.
Question one:
During the SPI initialization process, the three pins MISO, MOSI, and SCLK were not initialized, which caused problems in writing and reading data during the communication process. So here is the SPI initialization code that can run normally after testing. (STM8L101F3, using the official standard library)
#include "stm8l10x.h"
#include "stm8l10x_spi.h"
#include "stm8l10x_gpio.h"
/***************************SPI related definitions**************************/
#define SPI_GPIO_PORT GPIOB
#define SPI_CS_PIN GPIO_Pin_4
#define SPI_SCLK_PIN GPIO_Pin_5
#define SPI_MOSI_PIN GPIO_Pin_6
#define SPI_MISO_PIN GPIO_Pin_7
/*The following settings are in accordance with the corresponding configuration of my three-axis sensor*/
#define SPI_FIRSTBIT_TYPE SPI_FirstBit_MSB
#define SPI_SPEED_PRESC SPI_BaudRatePrescaler_4
#define SPI_MODE SPI_Mode_Master
#define SPI_CPOL SPI_CPOL_Low
#define SPI_CPHA SPI_CPHA_1Edge
#define SPI_DATA_MODE SPI_Direction_2Lines_FullDuplex
#define SPI_CS_CTRL SPI_NSS_Soft
/*SPI initialization*/
void spi_init(void)
{
//Start the SPI peripheral clock
CLK_PeripheralClockConfig(CLK_Peripheral_SPI, ENABLE);
//SPI reset
SPI_DeInit();
//SPI related GPIO initialization
GPIO_Init(SPI_GPIO_PORT, SPI_CS_PIN, GPIO_Mode_Out_PP_High_Fast);
GPIO_Init(SPI_GPIO_PORT, SPI_SCLK_PIN, GPIO_Mode_Out_PP_High_Fast);
GPIO_Init(SPI_GPIO_PORT, SPI_MOSI_PIN, GPIO_Mode_Out_PP_High_Fast);
//This setting is critical. As the master device, it must be set as input
GPIO_Init(SPI_GPIO_PORT, SPI_MISO_PIN, GPIO_Mode_In_PU_No_IT);
//SPI initialization
SPI_Init(SPI_FIRSTBIT_TYPE, SPI_SPEED_PRESC, SPI_MODE, SPI_CPOL, SPI_CPHA,
SPI_DATA_MODE, SPI_CS_CTRL);
//SPI start
SPI_Cmd(ENABLE);
}
Question 2:
Regarding the data writing and data reading issues, during my use, the official library provides two functions, one for writing data and the other for receiving data. Because in my application, I need to write two bytes of command and address data first, and then read the subsequent data. Therefore, in use, I first use the SPI_SendData() function to write data without reading the corresponding received data. (In fact, to read data through SPI communication, you must write it first before you can read it, and only when you write to the slave device will there be a clock.) Then I started to read the data, and found that the data I read was always wrong. Later, through instrument analysis, I found that the data I needed was in the response data, but the first data I read was actually the data of the first byte response when I wrote it. That is to say, when writing data, if we do not read the corresponding data, and then read the data after writing the data, it is actually the data returned when the first byte is written, and the subsequent data will not be overwritten and updated. Here is the modified code.
/*This part is the code for writing and reading data. Remember to turn on and off the chip select signal before and after reading and writing data*/
void spi_write(uint8_t data)
{
while(SPI_GetFlagStatus(SPI_FLAG_TXE) == RESET);
SPI_SendData(data);
//It must not be omitted. If the corresponding data is not received, reading the data immediately will cause incorrect data to be read.
while (SPI_GetFlagStatus(SPI_FLAG_RXNE) == RESET);
u8 dd = SPI_ReceiveData();
}
uint8_t spi_read()
{
//Select an invalid data to send (customized, just to provide a clock to the slave device), and then read the corresponding data
uint8_t data = 0xff;
while (SPI_GetFlagStatus(SPI_FLAG_TXE) == RESET);
SPI_SendData(data);
while (SPI_GetFlagStatus(SPI_FLAG_RXNE) == RESET);
uint8_t rxdata = SPI_ReceiveData();
return rxdata;
}
//Write data and read required data
void spi_write2read(uint8_t *wdata, uint8_t wlen, uint8_t *rdata, uint8_t rlen)
{
uint8_t i;
if (wdata == NULL || rdata == NULL) {
return;
}
//Write data
for (i = 0; i < wlen; i++) {
spi_write(wdata[i]);
}
//Read data
for (i = 0; i < rlen; i++) {
rdata[i] = spi_read();
}
}
Summarize:
I have a better understanding of using SPI on microcontrollers, haha.
Previous article:ADC sampling frequency calculation and clock frequency selection
Next article:The difference between FLASH and EEPROM
Recommended ReadingLatest update time:2024-11-25 04:13
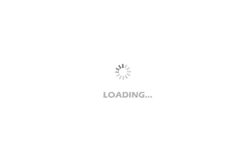
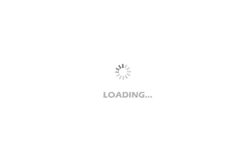
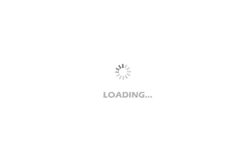
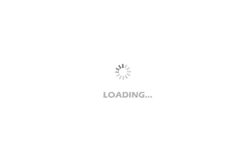
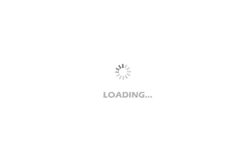
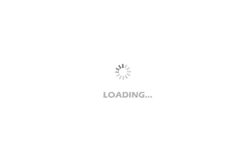
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- CATL releases October battle report
- Battery industry in October 2024: growth momentum remains unabated!
- Mercedes-Benz will launch the eCitaro equipped with NMC4 batteries to provide high energy density and long life
- Many companies have announced progress on solid-state batteries. When will solid-state batteries go into mass production?
- Xsens Sirius Series Inertial Sensors Enable 3D Inertial Navigation in Harsh Environments
- Infineon's Automotive Landscape: From Hardware to Systems
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- PWM output of MSP430 library timer TA
-
[NXP Rapid IoT Review] +
NXP Rapid IoT, a simplest cloud server prototype - Welcome to chat with me, guys~
- EEWORLD University ---- Computer Control Technology
- You are invited to watch Tektronix Live Lecture Series - Power Supply!
- What is the reason for the microcontroller compilation error?
- How to be a Star Engineer
- Please advise, why do you need to remove the motherboard before burning the flash?
- Can water start a car?
- You are invited to attend the 2018 TI Guangzhou Industrial Applications Seminar!