Why use a watchdog
The matter is very simple. A data collection product I made before was abnormal for some reason, fell into an infinite loop and then "frozen". After analyzing it many times, I couldn't find the reason, but every time I restarted it, I could collect data normally. Later I found a solution: watchdog! The purpose is to automatically reset when the program enters an infinite loop or the hardware is abnormal, so that you can get the same effect as after restarting the power.
The platform I use: stm32f103 series microcontroller
The burning and debugging mode used is Jlink SWD mode.
Use the STM32 official template library.
There are two types of ST series microcontroller watchdogs:
1. Independent watchdog, 2. Window watchdog.
Independent watchdog:
See Independent watchdog (IWDG) in the RM (reference Manual)
Of course, just take a brief look at the introduction in RM (as for the operation of registers, we can skip it because we use library development, but the basic process must be understood!).
Here we have to grasp a few key points:
a. The stm32f10x series has two watchdogs. The watchdog is mainly used to detect problems caused by software errors and trigger the system to automatically reset or trigger an interrupt (only for window watchdogs).
b. The clock source of the independent watchdog is LSI, and it can remain activated even if the main clock fails. The clock source of the window watchdog is the APB1 clock, and the frequency division value can be modified.
c. Independent watchdog: A system fault detector with an independent clock (internal low-speed clock LSI), so it is not affected by the system hardware. It is mainly used to monitor hardware errors. The accuracy requirement is relatively low.
d. Window watchdog: The clock is the same as the system. If the system clock stops, the watchdog will lose its function. It is mainly used to monitor software errors. Higher accuracy is required.
Brief introduction to the watchdog principle: There is a register that is constantly decreasing according to the clock source (there is a dog that consumes energy continuously). When the register is 0, it will trigger a system reset (the dog will bark). In order to prevent the register from being 0, we must reset the register value on time (feed the dog). In this way, when the software works normally (feed the dog normally, the dog will not bark), constantly resetting the register will not cause a reset. If the software falls into an infinite loop and no longer resets the register (the dog will bark if it is not fed), a reset will occur.
Therefore, if we have a software that sometimes has a memory error or falls into an infinite loop, we can use an independent watchdog to reset the device.
Without further ado:
Code example: Come on, help us!
//---------------------
void IWDG_Init()
{
//Enable write access to IWDG_PR and IWDG_RLR registers
IWDG_WriteAccessCmd(IWDG_WriteAccess_Enable);
//Configure the IWDG prescaler
IWDG_SetPrescaler(IWDG_Prescaler_16); //10k
//Configure the IWDG counter value
IWDG_SetReload(2500); // Bits11:0 RL[11:0]: Watchdog counter reload value ~ Only 12bit ~max value = 4096
IWDG_ReloadCounter();
IWDG_Enable();
}
//---------------------
What? How was this code written? Don't worry, let me tell you!
Since we are using the official ST library, there are a lot of documentation! Just look at the comments! As follows:
First, open any template of the official library: Use keil MDK to open the following directory
stsw-stm32062.zip\STM32F2xx_StdPeriph_Lib_V1.1.0\Project\STM32F2xx_StdPeriph_Template\MDK-ARM
Then you will see a file like the one below on the left. After a brief inspection, we will find that the function we want to use is IWDG. Therefore, it must be the file stm32f2xx_iwdg.c! (Only the library of f2xx series has comments, but not 10x. But it is almost the same, maybe there are more tutorials for 10x.
After opening it, there will be detailed introduction!
* ================================================= ==================
* How to use this driver
* ================================================= ==================
* 1. Enable write access to IWDG_PR and IWDG_RLR registers using
* IWDG_WriteAccessCmd(IWDG_WriteAccess_Enable) function
*
* 2. Configure the IWDG prescaler using IWDG_SetPrescaler() function
*
* 3. Configure the IWDG counter value using IWDG_SetReload() function.
* This value will be loaded in the IWDG counter each time the counter
* is reloaded, then the IWDG will start counting down from this value.
*
* 4. Start the IWDG using IWDG_Enable() function, when the IWDG is used
* in software mode (no need to enable the LSI, it will be enabled
* by hardware)
*
* 5. Then the application program must reload the IWDG counter at regular
* intervals during normal operation to prevent an MCU reset, using
* IWDG_ReloadCounter() function.
Don't say you don't understand!
Verify the entire watchdog process as follows:
IWDG_Init();
IWDG_ReloadCounter();
printf("SysInit\r\n");
while(1)
{
Delay_us(1000);
IWDG_ReloadCounter();
printf("1000 \r\n");
Delay_us(10000);
IWDG_ReloadCounter();
printf("10000 \r\n");
Delay_us(100000);
IWDG_ReloadCounter();
printf("100000 \r\n");
Delay_us(200000);
IWDG_ReloadCounter();
printf("200000 \r\n");
Delay_us(300000);
IWDG_ReloadCounter();
printf("200000 \r\n");
Delay_us(400000);
IWDG_ReloadCounter();
printf("400000 \r\n");
Delay_us(500000);
IWDG_ReloadCounter();
printf("500000 \r\n");
Delay_us(600000);
IWDG_ReloadCounter();
printf("600000 \r\n");
Delay_us(700000);
IWDG_ReloadCounter();
printf("700000 \r\n");
Delay_us(800000);
IWDG_ReloadCounter();
printf("800000 \r\n");
Delay_us(900000);
IWDG_ReloadCounter();
printf("900000 \r\n");
Delay_us(1000000);
IWDG_ReloadCounter();
printf("1000000 \r\n");
IWDG_ReloadCounter();
Delay_us(2000000);
printf("2000000\r\n");
}
In this way, the watchdog must be fed once every 1s. Therefore, the print function with a delay of 2s will not be printed out and will be reset again.
In addition, it is important to note that:
The ReloadCounter register of the independent watchdog has only 12 bits~! This means that the maximum value is 2 to the 12th power = 4096, which must not be exceeded!
The clock of the independent watchdog is 40khz as shown above.
Previous article:Do you know these 8 GPIO working modes in STM32?
Next article:STM32 development of STM32 hardware IIC operation
Recommended ReadingLatest update time:2024-11-23 11:56
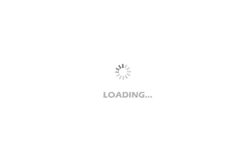
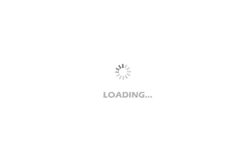
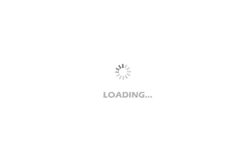
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Rechargeable battery knowledge
- Power Management and Applications
- I bought 8 apples last week and ate one every day, starting with the small ones. The last one had a rotten hole in it, so...
- node-red simulates LED flashing light control system program
- Are Decoupling Capacitors Really What You Need?
- 【i.MX6ULL】Driver Development 3——GPIO Register Configuration Principle
- 【Renovation of old things】 Finished product of graffiti desk lamp
- Prototyped a PCB expansion board
- [Practical sharing] Sharing of analog circuit diagrams commonly used by electronic engineers
- MSP432 MCU realizes speech recognition technology