STM8S103K3: External clock + internal clock switching.
First, take a look at the clock structure diagram of STM8S103K3, which can help you understand it well.
There are several clocks here, which are the clocks marked in the figure, and you need to clarify them:
fHSE: External high-speed crystal oscillator clock, which is generated by an external crystal oscillator and its size is determined by the size of the external crystal oscillator. The external crystal oscillator range of STM8S is: 1-24M, see "HSE OSC 1-24M" in the figure.
fHSI: internal RC high-speed clock, which is generated by the internal RC oscillator circuit, and its value is 16M. However, it can be divided by the frequency divider behind it, and four frequency division coefficients are available (1, 2, 4, 8). Note: The accuracy is slightly worse than that of the external crystal oscillator.
fMASTER: Master clock, which is provided by HSE or HSI. Its main function is to provide clock for peripheral devices (such as I2C, SPI, ADC, etc.) and provide clock source for CPU.
fCPU: CPU clock, which is obtained by dividing fMASTER. Its function is to provide clock to the CPU. One mechanical cycle is one fCPU clock cycle.
The following is the source code of the clock for your reference.
There are four programs written here, namely:
Using high-speed internal clock (register version)
Using high-speed internal clock (library function version)
Using an external clock (register version)
Using an external clock (library version)
The source code is as follows, you should be able to read the comments.
/*******************************************************************************
* Function Name : InitCpuClock.
* Description : Initial CPU clock, .
* Input : None.
* Output : None.
* Return : None.
*******************************************************************************/
static void InitCpuClock(void)
{
#if 1
// Register version - uses high speed internal clock
//Use HSI @8MHZ, div = 2; 8=16/2
CLK->ECKR &= ~CLK_ECKR_HSEEN; // Disable external clock
CLK->CKDIVR &= (uint8_t)(~CLK_CKDIVR_HSIDIV); // Clear internal clock prescaler
CLK->CKDIVR |= CLK_PRESCALER_HSIDIV2; // Set the internal clock prescaler to 2; For details, please refer to the datasheet (reference manual)
CLK->ICKR |= CLK_ICKR_HSIEN; // Enable internal high-speed clock
while(!(CLK->ICKR&CLK_ICKR_HSIRDY)); // Wait for the internal high-speed clock to stabilize. After stabilization, the internal clock has started running. Clock = 16/2 M
#endif
#if 0
// Library version - use internal high speed clock
//Use HSI @8MHZ, div = 2; 8=16/2
CLK_HSECmd(DISABLE); // Disable external clock
CLK_HSIPrescalerConfig(CLK_PRESCALER_HSIDIV2); // Set the internal clock prescaler to 2; For details, please refer to the data manual (reference manual)
CLK_HSICmd(ENABLE); // Enable internal high-speed clock
while(!(CLK->ICKR&CLK_ICKR_HSIRDY)); // Wait for the internal high-speed clock to stabilize. After stabilization, the internal clock has started running. Clock = 16/2 M
#endif
#if 0
// Register version - using external clock
CLK->CKDIVR |= CLK_PRESCALER_CPUDIV1; // CPU clock divided by 1, CPU clock = external clock (that is, external crystal frequency)
CLK->ECKR |= CLK_ECKR_HSEEN; // Allow external high speed oscillator to work
while(!(CLK->ECKR & CLK_ECKR_HSERDY)); // Wait for the external high-speed oscillator to be ready
CLK->SWCR |= CLK_SWCR_SWEN; // Enable switching
CLK->SWR = CLK_SOURCE_HSE; // Select the high-speed oscillator outside the chip as the main clock
while(!(CLK->SWCR&CLK_SWCR_SWIF)); // Wait for the switch to succeed
CLK->SWCR &= ~(CLK_SWCR_SWEN|CLK_SWCR_SWIF); // Clear the switch flag
#endif
#if 0
// Library version - using external clock
CLK->CKDIVR |= CLK_PRESCALER_CPUDIV1; // CPU clock divided by 1, CPU clock = external clock (that is, external crystal frequency)
CLK_ClockSwitchConfig(CLK_SWITCHMODE_AUTO, // Clock automatic switching mode, note: the parameter is automatic switching, otherwise only using this function will not switch successfully
CLK_SOURCE_HSE, // Clock to switch (here is the external clock)
DISABLE, // Whether to enable the switching completion interrupt (disabled here)
CLK_CURRENTCLOCKSTATE_ENABLE); // Whether to enable the current clock (here choose to turn off the current clock HSI)
CLK->SWCR &= ~(CLK_SWCR_SWEN|CLK_SWCR_SWIF); // Clear the switch flag
#endif
}
Previous article:stm8 clock source selection configuration
Next article:STM8 clock source switching
Recommended ReadingLatest update time:2024-11-15 17:27
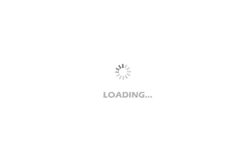
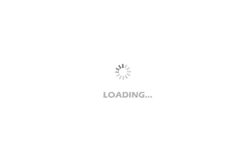
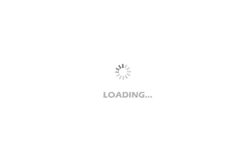
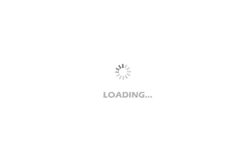
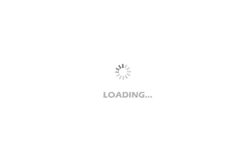
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Practice together in 2021 + review the gains and losses of 2020 work
- What to do if SYSTEM is not found in the AD status bar?
- Single analog signal input channel detects multiple signal major problems
- FPGA Design Tips
- Easy to understand and not esoteric: "Power Supply Design" - Fundamentals of Electronic Design
- EEWORLD University Hall ---- Electromagnetic Field and Electromagnetic Wave
- About the development of ST's 8032 core microcontroller uPSD3234A-40u
- I burned a stmf103 minimum board today
- Hall Effect Current Sensors in Telecom Rectifiers and Server Power Supplies
- MSP430 has 5 low power modes