(II) ADC cyclically collects six voltages, using DMA.
This experiment was really depressing. My lack of understanding of DMA made me fall into misunderstandings. After understanding it, I admired the power of DMA even more.
The misunderstanding is: from the goal of the experiment, we know that this time we use DMA to transfer the data converted by ADC to an array in the memory for storage. Because 6 channels are collected, the scanning mode of ADC is enabled here. Once the ADC is started, the channels selected in SQRX will be converted in sequence. The problem is that I thought that ADC and DMA would not work in coordination at first, that is, ADC converts its own data and DMA transfers its own data. In this case, the array in the memory is not what I want. Later, I really studied it for a long time. With the reminder of a brother in the group, I realized that I might have thought too complicated. Maybe I could just convert it once in ADC and then transfer the data once in DMA. Ok, after the experiment, I learned that this idea is correct.
Okay, after saying so much nonsense, let's get to the point.
The six regular channels of ADC1 used here are: CH0, CH1, CH2, CH3, CH14, CH15,
and the corresponding pins are PA0, PA1, PA2, PA3, PC4, PC5.
About the configuration of ADC:
the scanning mode of ADC1 is started, as well as the continuous conversion mode and the independent working mode (only one ADC is used). Because DMA is used, the DMA bit must be enabled, and the external trigger (SWSTART) is used. The data is right-aligned. There are also SQRX and so on. I won’t talk about them. ADC interrupt is not needed here. The interrupt is in DMA.
About the configuration of DMA:
because the ADC request is specified in the first channel of DMA1, DMA_CH1 is used here, the peripheral address is the only data register of ADC (u32) & ADC1->DR, and the memory address is (u32) SendBuff array, which can store 6 elements. Here, the transmission completion interrupt (TCIF) is enabled, and the selection is to read from the peripheral, the circular mode, the peripheral address non-incremental mode, the memory address incremental mode, the peripheral data width is 16 bits, the memory address is 16 bits, and the non-memory to memory mode.
About DMA interrupt function:
When DMA transfers data 6 times, TCIF bit is automatically set, and the program enters the interrupt service function. First, turn off the continuous conversion of ADC. We put the array processing here, and send it to the serial port after processing. Through the computer's hyperterminal, you can see the data of the 6 pin voltages that keep changing. Don't forget to clear the interrupt flag and set the continuous conversion of ADC, and then start the conversion again.
In the main program, just initialize the system function and the serial port, then configure DMA, start the regular conversion channel, and start DMA, and then wait in an infinite loop.
Attached below is some code
-----------------------------------------------------------------------------------------------------------
void Adc_Init(void)
{
//Initialize IO port first
RCC->APB2ENR|=1<<2; //Enable PORTA port clock
RCC->APB2ENR|=1<<4; //Enable PORTC port clock
GPIOA->CRL&=0XFFFF0000; //PA0 1 2 3 anolog input
GPIOC->CRL&=0XFF00FFFF; //PC4,5 anolog input
//Channel 10/11 settings
RCC->APB2ENR|=1<<9; //ADC1 clock enable
RCC->APB2RSTR|=1<<9; //ADC1 reset
RCC->APB2RSTR&=~(1<<9); //Reset end
RCC->CFGR&=~(3<<14); //Division factor cleared
//SYSCLK/DIV2=12M ADC clock is set to 12M, ADC maximum clock cannot exceed 14M!
//Otherwise, the ADC accuracy will decrease!
RCC->CFGR|=2<<14;
ADC1->CR1&=0XF0FFFF; //Working mode cleared
ADC1->CR1|=0<<16; //Independent working mode
ADC1->CR1|=1<<8; //Scanning mode
ADC1->CR2|=1<<1; //Enable continuous conversion
ADC1->CR2|=1<<8; //Enable DMA
// ADC1->CR2&=~(1<<1); //Single conversion mode
ADC1->CR2&=~(7<<17);
ADC1->CR2|=7<<17; //Software control conversion
ADC1->CR2|=1<<20; //Use external trigger (SWSTART)!!! An event must be used to trigger
ADC1->CR2&=~(1<<11); //Right alignment
ADC1->SQR1&=~(0XF<<20);
ADC1->SQR1|=5<<20; //6 conversions in regular sequence
ADC1->SQR3 = 0X00000000;
ADC1->SQR3|= 0X1EE18820;
//Set sampling time of channels 0~3,14,15
ADC1->SMPR1&=0XFFFC0FFF; //Clear sampling time of channels 14,15
ADC1->SMPR2&=0XFFFFF000; //Clear sampling time of channels 0,1,2,3
ADC1->SMPR1|=7<<15; //Channel 15 239.5 cycles, increasing the sampling time can improve the accuracyADC1-
>SMPR1|=7<<12; //Channel 14 239.5 cycles, increasing the sampling time can improve the accuracyADC1-
>SMPR2|=7<<9; //Channel 3 239.5 cycles, increasing the sampling time can improve the accuracyADC1-
>SMPR2|=7<<6; //Channel 2 239.5 cycles, increasing the sampling time can improve the accuracyADC1-
>SMPR2|=7<<3; //Channel 1 239.5 cycles, increasing the sampling time can improve the accuracyADC1-
>SMPR2|=7<<0; //Channel 0 239.5 cycles, increasing the sampling time can improve the accuracy
ADC1->CR2|=1<<0; //Turn on AD converter
ADC1->CR2|=1<<3; //Enable reset calibration
while(ADC1->CR2&1<<3); //Wait for calibration to end
//This bit is set by software and cleared by hardware. This bit will be cleared after the calibration register is initialized.
ADC1->CR2|=1<<2; //Turn on AD calibration
while(ADC1->CR2&1<<2); //Wait for calibration to end
//This bit is set by software to start calibration and cleared by hardware when calibration ends
}
void MYDMA_Config(DMA_Channel_TypeDef*DMA_CHx,u32 cpar,u32 cmar,u16 cndtr)
{
u32 DR_Base; //Used as a buffer, I don't know why. It is necessary
RCC->AHBENR|=1<<0; //Turn on DMA1 clock
DR_Base=cpar;
DMA_CHx->CPAR=DR_Base; //DMA1 peripheral address
DMA_CHx->CMAR=(u32)cmar; //DMA1, memory address
DMA1_MEM_LEN=cndtr; //Save DMA transfer data amount
DMA_CHx->CNDTR=cndtr; //DMA1, transfer data amount
DMA_CHx->CCR=0X00000000; //Reset
DMA_CHx->CCR|=1<<1; //Allow interrupt after transfer
DMA_CHx->CCR|=0<<4; //Read from peripheral
DMA_CHx->CCR|=1<<5; //Cyclic mode
DMA_CHx->CCR|=0<<6; //Peripheral address non-incremental mode
DMA_CHx->CCR|=1<<7; //Memory incremental mode
DMA_CHx->CCR|=1<<8; //Peripheral data width is 16 bits
DMA_CHx->CCR|=1<<10; //Memory data width is 16 bits
DMA_CHx->CCR|=1<<12; //Medium priority
DMA_CHx->CCR|=0<<14; //Non-memory to memory mode
MY_NVIC_Init(1,3,DMA1_Channel1_IRQChannel ,2);
}
//Enable a DMA transfer
void MYDMA_Enable(DMA_Channel_TypeDef*DMA_CHx)
{
DMA_CHx->CCR&=~(1<<0); //Disable DMA transfer
DMA_CHx->CNDTR=DMA1_MEM_LEN; //DMA1, amount of data transferred
DMA_CHx->CCR|=1<<0; //Start DMA transfer
}
u16 ADC1_DR, adcx;
void DMAChannel1_IRQHandler(void)
{
u16 i;
u32 sum[6]={0},val=0;
LED0 =!LED0;
ADC1->CR2&=~(1<<1); //Turn off continuous conversion
for( i = 0; i<768 ;i+= 6)
{
sum[0] += SendBuff[i];
sum[1] += SendBuff[i+1];
sum[2] += SendBuff[i+2];
sum[ 3] += SendBuff[i+3];
sum[4] += SendBuff[i+4];
sum[5] += SendBuff[i+5];
}
for(i = 0;i <6;i++)
{
val = sum[i]/DMA_COUNT;
ADC1_DR = sum[i]/DMA_COUNT;
TEMP=(float)ADC1_DR*(3.3/4096);
adcx=TEMP;
LCD_ShowNum(149,70+i*20,adcx,1,16);//display voltage value
TEMP-=adcx;
TEMP*=1000;
LCD_ShowNum(165,70+i*20,TEMP,3, 16);
}
delay_ms(200);
DMA1->IFCR |= 1<<1; //Clear channel completion interrupt flag
ADC1->CR2|=1<<1; //Enable continuous conversion
ADC1->CR2| =1<<22; //Start rule conversion channel
}
--------------------------------------------------------------------------------------
Previous article:ARM processor startup process——S3C2440, S3C6410, S5PV210
Next article:STM32 uses SWV to output debug information under KEIL
Recommended ReadingLatest update time:2024-11-16 13:33
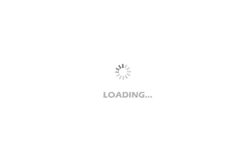
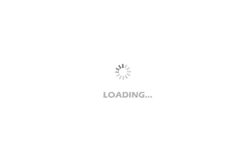
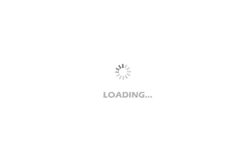
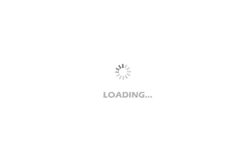
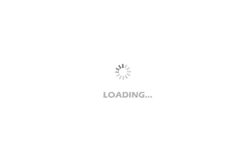
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Five Experiences in RF Circuit Design
- What is a combined hydraulic cylinder?
- [MYX-1028X] (V) Serial communication Demo
- Overall design of wireless sensor network nodes
- EEWORLD University Hall ---- Fundamentals of Circuit Theory by Zhao Jinquan, Xi'an Jiaotong University
- Anxinke NB-IoT module review - some speculation about the failure of CH340
- A low-cost differential audio signal transmission solution for automotive applications
- Understand the structure and working principle of thyristor in a few steps
- Introducing a newly invented bandwidth amplifier circuit: "Angel"
- Embedded Qt-FFmpeg design an RTSP player