Host environment: Windows 7 SP1
Development environment: MDK5.14
Target board: STM32F103C8T6
Development library: STM32F1Cube library and STM32_USB_Device_Library
The STM32Cube library provides some USB-related routines. In the Applications directory under its project directory, open the STM3210E_EVAL directory and you can see the following routines:
Here we select a simple example CDC_Standalone, which is a USB communication routine. The specific implementation is a USB to serial port function, which is equivalent to a USB serial port line. Copy the files in the inc and src directories in the example to the new project. Here, the files related to USB communication are placed in the vcp directory. The file directory structure is as follows:
The BSP directory is very simple. Since the purchased board only uses USB, UART modules and an LED light, the schematic diagram is as follows:
The PA15 pin is connected to an LED light as follows:
Therefore, only the operation of LED is added in the stm32f103_demo file. The source file is as follows:
/********************************************************************************
* @file stm32f103_demo.c
* @author MCD Application Team
* @version V6.0.0
* @date 13-October-2015
* @brief This file provides a set of firmware functions to manage Leds,
* for STM32F103_DEMO
******************************************************************************
* @attention
*
*
© COPYRIGHT(c) 2014 STMicroelectronics *
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of STMicroelectronics nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "STM32f103_demo.h"
/** @addtogroup BSP
* @{
*/
/** @defgroup STM32F103_DEMO STM32F103-DEMO
* @{
*/
/** @defgroup STM32F103_DEMO_Common STM32F103-DEMO Common
* @{
*/
/** @defgroup STM32F103_DEMO_Private_TypesDefinitions Private Types Definitions
* @{
*/
/**
* @}
*/
/** @defgroup STM32F103_DEMO_Private_Defines Private Defines
* @{
*/
/**
* @brief STM32103 EVAL BSP Driver version number V6.0.0
*/
#define __STM32F103_DEMO_BSP_VERSION_MAIN (0x06) /*!< [31:24] main version */
#define __STM32F103_DEMO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */
#define __STM32F103_DEMO_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */
#define __STM32F103_DEMO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */
#define __STM32F103_DEMO_BSP_VERSION ((__STM32F103_DEMO_BSP_VERSION_MAIN << 24)\
|(__STM32F103_DEMO_BSP_VERSION_SUB1 << 16)\
|(__STM32F103_DEMO_BSP_VERSION_SUB2 << 8 )\
|(__STM32F103_DEMO_BSP_VERSION_RC))
/**
* @}
*/
/** @defgroup STM32F103_DEMO_Private_Variables Private Variables
* @{
*/
/**
* @brief LED variables
*/
GPIO_TypeDef* LED_PORT[LEDn] = {LED_GPIO_PORT};
const uint16_t LED_PINS[LEDn] = {LED_PIN};
/**
* @brief This method returns the STM32103 EVAL BSP Driver revision
* @retval version : 0xXYZR (8bits for each decimal, R for RC)
*/
uint32_t BSP_GetVersion(void)
{
return __STM32F103_DEMO_BSP_VERSION;
}
/**
* @brief Configures LED GPIO.
* @param Led: Specifies the Led to be configured.
* This parameter can be one of following parameters:
* @arg LED
* @retval None
*/
void BSP_LED_Init(Led_TypeDef Led)
{
GPIO_InitTypeDef gpioinitstruct = {0};
/* Enable the GPIO_LED clock */
LED_GPIO_CLK_ENABLE();
__HAL_RCC_AFIO_CLK_ENABLE();
__HAL_AFIO_REMAP_SWJ_DISABLE();
/* Configure the GPIO_LED pin */
gpioinitstruct.Pin = LED_PINS[Led];
gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP;
gpioinitstruct.Pull = GPIO_NOPULL;
gpioinitstruct.Speed = GPIO_SPEED_HIGH;
HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct);
HAL_GPIO_WritePin(LED_PORT[Led], LED_PINS[Led], GPIO_PIN_RESET);
}
/**
* @brief Turns selected LED On.
* @param Led: Specifies the Led to be set on.
* This parameter can be one of following parameters:
* @arg LED
* @retval None
*/
void BSP_LED_On(Led_TypeDef Led)
{
HAL_GPIO_WritePin(LED_PORT[Led], LED_PINS[Led], GPIO_PIN_RESET);
}
/**
* @brief Turns selected LED Off.
* @param Led: Specifies the Led to be set off.
* This parameter can be one of following parameters:
* @arg LED
* @retval None
*/
void BSP_LED_Off(Led_TypeDef Led)
{
HAL_GPIO_WritePin(LED_PORT[Led], LED_PINS[Led], GPIO_PIN_SET);
}
/**
* @brief Toggles the selected LED.
* @param Led: Specifies the Led to be toggled.
* This parameter can be one of following parameters:
* @arg LED
* @retval None
*/
void BSP_LED_Toggle(Led_TypeDef Led)
{
HAL_GPIO_TogglePin(LED_PORT[Led], LED_PINS[Led]);
}
/**
* @}
*/
/**
* @}
*/
/************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
The header file is as follows:
/**
******************************************************************************
* @file stm32f103_demo.h
* @author MCD Application Team
* @version V6.0.0
* @date 13-October-2015
* @brief This file contains definitions for STM32F103_DEMO's LEDs,
* hardware resources.
******************************************************************************
* @attention
*
*
© COPYRIGHT(c) 2014 STMicroelectronics *
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of STMicroelectronics nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
******************************************************************************
*/
/* Define to prevent recursive inclusion -------------------------------------*/
#ifndef __STM32F103_DEMO_H
#define __STM32F103_DEMO_H
#ifdef __cplusplus
extern "C" {
#endif
/** @addtogroup BSP
* @{
*/
/** @addtogroup STM32F103_DEMO
* @{
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f1xx_hal.h"
/** @addtogroup STM32F103_DEMO_Common STM3210E-EVAL Common
* @{
*/
/** @defgroup STM32F103_DEMO_Exported_Types Exported Types
* @{
*/
/**
* @brief LED Types Definition
*/
typedef enum
{
LED = 0,
LED_GREEN = LED,
} Led_TypeDef;
/**
* @}
*/
/** @defgroup STM32F103_DEMO_Exported_Constants Exported Constants
* @{
*/
/**
* @brief Define for STM32F103_DEMO board
*/
#if !defined (USE_STM32F103_DEMO)
#define USE_STM32F103_DEMO
#endif
/** @addtogroup STM32F103_DEMO_LED
* @{
*/
#define LEDn 1
#define LED_PIN GPIO_PIN_15 /* PA.15*/
#define LED_GPIO_PORT GPIOA
#define LED_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE()
#define LED_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE()
/** @addtogroup STM32F103_DEMO_Exported_Functions
* @{
*/
uint32_t BSP_GetVersion(void);
void BSP_LED_Init(Led_TypeDef Led);
void BSP_LED_On(Led_TypeDef Led);
void BSP_LED_Off(Led_TypeDef Led);
void BSP_LED_Toggle(Led_TypeDef Led);
/**
* @}
*/
/**
* @}
*/
#ifdef __cplusplus
}
#endif
#endif /* __STM32F103_DEMO_H */
/************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
There is almost no change in other files, just change the content of the referenced stm32f10e_eval.h header file to stm32f13_demo.h. It should be noted that the PA15 port is used as a debug interface when the STM32 is running normally, so the debug function needs to be disabled, which is reflected in the BSP_LED_Init() function. Compile the code and download it to the target board.
Before running the code, we need to install a USB serial port driver: VCP_1.40_Setup, which can be found on the ST official website. With it, our USB can be identified as a serial port on the host and can be operated using the serial port. After the hardware is connected, we can see that there are two serial ports through the device manager, as follows:
COM5 is the actual serial port of the board, and COM6 is a virtual serial port created by USB. By opening two serial terminals, serial communication can be achieved as follows:
The information sent from COM5 can be received in COM6, and the information sent from COM6 can be received in COM5. At the same time, the DEMO supports the modification of the serial port baud rate. We change the baud rate of COM6 and COM5 to 57600 and continue to communicate. It is still possible, as follows:
Of course, except for the baud rate, the data bit, stop bit, and check bit can all be modified normally. With these intuitive functional descriptions, it will be easier for us to understand the use of the USB CDC class. Next, let's analyze the code implementation of VCP step by step.
First, let's analyze the processing of physical hardware, that is, the stm32f1xx_hal_msp,c file. This file is very simple and just initializes the IO resources of UART.
/**
******************************************************************************
* @file USB_Device/CDC_Standalone/Src/stm32f1xx_hal_msp.c
* @author MCD Application Team
* @version V1.2.0
* @date 31-July-2015
* @brief HAL MSP module.
******************************************************************************
* @attention
*
*
© COPYRIGHT(c) 2015 STMicroelectronics *
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of STMicroelectronics nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "main.h"
/** @addtogroup USBD_USER
* @{
*/
/** @defgroup USBD_USR_MAIN
* @brief This file is the CDC application main file
* @{
*/
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
/* Private functions ---------------------------------------------------------*/
/**
* @brief UART MSP Initialization
* This function configures the hardware resources used in this example:
* - Peripheral's clock enable
* - Peripheral's GPIO Configuration
* - DMA configuration for transmission request by peripheral
* - NVIC configuration for DMA interrupt request enable
* @param huart: UART handle pointer
* @retval None
*/
void HAL_UART_MspInit(UART_HandleTypeDef *huart)
{
static DMA_HandleTypeDef hdma_tx;
GPIO_InitTypeDef GPIO_InitStruct;
/*##-1- Enable peripherals and GPIO Clocks #################################*/
/* Enable GPIO clock */
USARTx_TX_GPIO_CLK_ENABLE();
USARTx_RX_GPIO_CLK_ENABLE();
/* Enable USARTx clock */
USARTx_CLK_ENABLE();
/* Enable DMA clock */
DMAx_CLK_ENABLE();
/*##-2- Configure peripheral GPIO ##########################################*/
/* UART TX GPIO pin configuration */
GPIO_InitStruct.Pin = USARTx_TX_PIN;
GPIO_InitStruct.Mode = GPIO_MODE_AF_PP;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_HIGH;
HAL_GPIO_Init(USARTx_TX_GPIO_PORT, &GPIO_InitStruct);
/* UART RX GPIO pin configuration */
GPIO_InitStruct.Pin = USARTx_RX_PIN;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
HAL_GPIO_Init(USARTx_RX_GPIO_PORT, &GPIO_InitStruct);
/*##-3- Configure the NVIC for UART ########################################*/
HAL_NVIC_SetPriority(USARTx_IRQn, 4, 0);
HAL_NVIC_EnableIRQ(USARTx_IRQn);
/*##-4- Configure the DMA channels ##########################################*/
/* Configure the DMA handler for Transmission process */
hdma_tx.Instance = USARTx_TX_DMA_STREAM;
hdma_tx.Init.Direction = DMA_MEMORY_TO_PERIPH;
hdma_tx.Init.PeriphInc = DMA_PINC_DISABLE;
hdma_tx.Init.MemInc = DMA_MINC_ENABLE;
hdma_tx.Init.PeriphDataAlignment = DMA_PDATAALIGN_BYTE;
hdma_tx.Init.MemDataAlignment = DMA_MDATAALIGN_BYTE;
hdma_tx.Init.Mode = DMA_NORMAL;
hdma_tx.Init.Priority = DMA_PRIORITY_LOW;
HAL_DMA_Init(&hdma_tx);
/* Associate the initialized DMA handle to the UART handle */
__HAL_LINKDMA(huart, hdmatx, hdma_tx);
/*##-5- Configure the NVIC for DMA #########################################*/
/* NVIC configuration for DMA transfer complete interrupt (USARTx_TX) */
HAL_NVIC_SetPriority(USARTx_DMA_TX_IRQn, 5, 0);
HAL_NVIC_EnableIRQ(USARTx_DMA_TX_IRQn);
/*##-6- Enable TIM peripherals Clock #######################################*/
TIMx_CLK_ENABLE();
/*##-7- Configure the NVIC for TIMx ########################################*/
/* Set Interrupt Group Priority */
HAL_NVIC_SetPriority(TIMx_IRQn, 5, 0);
/* Enable the TIMx global Interrupt */
HAL_NVIC_EnableIRQ(TIMx_IRQn);
}
/**
* @brief UART MSP De-Initialization
* This function frees the hardware resources used in this example:
* - Disable the Peripheral's clock
* - Revert GPIO, DMA and NVIC configuration to their default state
* @param huart: UART handle pointer
* @retval None
*/
void HAL_UART_MspDeInit(UART_HandleTypeDef *huart)
{
/*##-1- Reset peripherals ##################################################*/
USARTx_FORCE_RESET();
USARTx_RELEASE_RESET();
/*##-2- Disable peripherals and GPIO Clocks #################################*/
/* Configure UART Tx as alternate function */
HAL_GPIO_DeInit(USARTx_TX_GPIO_PORT, USARTx_TX_PIN);
/* Configure UART Rx as alternate function */
HAL_GPIO_DeInit(USARTx_RX_GPIO_PORT, USARTx_RX_PIN);
/*##-3- Disable the NVIC for UART ##########################################*/
HAL_NVIC_DisableIRQ(USARTx_IRQn);
/*##-4- Disable the NVIC for DMA ###########################################*/
HAL_NVIC_DisableIRQ(USARTx_DMA_TX_IRQn);
/*##-5- Reset TIM peripheral ###############################################*/
TIMx_FORCE_RESET();
TIMx_RELEASE_RESET();
}
/**
* @}
*/
/**
* @}
*/
/**
* @}
*/
/************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Three interrupts are enabled here: UART1 interrupt, DMA1 channel 4 (UART_TX) interrupt, TIM3 timer interrupt. The initialization of these IO resources is relatively simple, clear and easy to understand. The reason why UARTIO resources are initialized here is because this DEMO implements the function of USB to serial port, that is, the data received from the UART port is sent out from the USB port, and the data received from the USB port is sent out from the UART port. The initialization of the IO resources of the USB port is in another file. The role of the timer is reflected in another file, although it is not clear why the timer is enabled here instead of being put together with its initialization. . .
Normally, USB devices add pull-up resistors to D+ and D- to detect device connection and disconnection events. In the example, an IO is used to dynamically pull up and down. The board I bought here does not have an IO port connected and directly uses a 1.5K pull-up resistor, so there is no need for a separate IO port control, as shown in the figure:
Just remove the pull-up resistor control code in the usbd_conf.c file, which involves the HAL_PCD_MspInit() function and the HAL_PCDEx_SetConnectionState() function. Modify them as follows:
/**
* @brief Initializes the PCD MSP.
* @param hpcd: PCD handle
* @retval None
*/
void HAL_PCD_MspInit(PCD_HandleTypeDef *hpcd)
{
GPIO_InitTypeDef GPIO_InitStruct;
/* Enable the GPIOA clock */
__HAL_RCC_GPIOA_CLK_ENABLE();
/* Configure USB DM/DP pins */
GPIO_InitStruct.Pin = (GPIO_PIN_11 | GPIO_PIN_12);
GPIO_InitStruct.Mode = GPIO_MODE_AF_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_HIGH;
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
/* Enable USB Clock */
__HAL_RCC_USB_CLK_ENABLE();
/* Set USB Interrupt priority */
HAL_NVIC_SetPriority(USB_LP_CAN1_RX0_IRQn, 7, 0);
/* Enable USB Interrupt */
HAL_NVIC_EnableIRQ(USB_LP_CAN1_RX0_IRQn);
}
/**
* @brief Software Device Connection
* @param hpcd: PCD handle
* @param state: connection state (0 : disconnected / 1: connected)
* @retval None
*/
void HAL_PCDEx_SetConnectionState(PCD_HandleTypeDef *hpcd, uint8_t state)
{
}
Finally, slightly modify the Toggle_Leds() function in the main function to change the cumulative counter to be cleared after increasing to 1000, that is, flipping every 1S, as follows:
/**
* @brief Toggle LEDs to shows user input state.
* @param None
* @retval None
*/
void Toggle_Leds(void)
{
static uint32_t ticks;
if (ticks++ == 1000)
{
BSP_LED_Toggle(LED);
ticks = 0;
}
}
At this point, the basic code has been modified, and all that remains is to analyze the code.
Previous article:STM32 USB learning notes 8
Next article:STM32 USB Learning Notes 6
Recommended ReadingLatest update time:2024-11-16 15:59
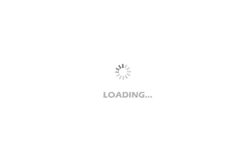
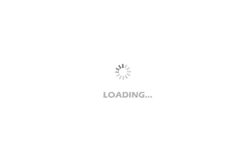
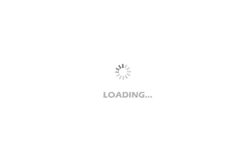
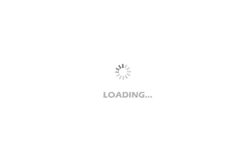
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Live Review: TI Ultrasonic Gas Flow Measurement Innovation Solution + CC13X2/CC26X2
- What a mess it is to have a 0.1uf capacitor with a problem {Complain about this here}
- PCB impedance control experience sharing
- Domestic sewage treatment online monitoring system
- Be careful when shopping on June 18: China Consumers Association warns consumers that so-called "quantum products" are "pseudo-technology"
- Getting Started with the ST SensorTile.box Sensor Kit (3) Expert Mode Experience
- Problem 2 of 7S3P battery pack
- Netizens are porting mpy to Nucleo-32
- LOTO arbitrary waveform generator SIG82 simulates the output of relay energization and disconnection signal waveforms for algorithm debugging
- For sale: 4412 development board