This is also a routine in the development board, with explanations of the key points. The program is as follows:
/*********************************************************
Program notes:
First, you can choose whether to turn on the internal reference voltage or use the external reference voltage
Each channel can independently select the reference voltage
If an external reference voltage is connected, be careful to turn off the internal reference voltage to prevent damage
Microcontroller
Program function: The MCU's on-chip ADC converts the voltage of the P6.0 port
The analog voltage value is displayed on the 1602 LCD.
----------------------------------------------------------
Dip switch setting: turn LCD to ON and other positions to OFF
Test instructions: Adjust the knob of potentiometer W1 and observe the changes in the numbers displayed on the LCD.
*********************************************************/
#include
#include "cry1602.h"
#include "cry1602.c"
//typedef unsigned char uchar;
//typedef unsigned int uint;
#define Num_of_Results 32
fly shuzi[] = {"0123456789."};
uchar tishi[] = {"The volt is:"};
static uint results[Num_of_Results]; //Array to save ADC conversion results
void Trans_val(uint Hex_Val);
/****************************Main function********************************/
void main(void)
{
WDTCTL = WDTPW+WDTHOLD; //Turn off the watchdog
/*The following six lines of code close all IO ports*/
P1DIR = 0XFF;P1OUT = 0XFF;
P2DIR = 0XFF;P2OUT = 0XFF;
P3DIR = 0XFF;P3OUT = 0XFF;
P4DIR = 0XFF;P4OUT = 0XFF;
P5DIR = 0XFF;P5OUT = 0XFF;
P6DIR = 0XFF;P6OUT = 0XFF;
P6DIR |= BIT2;P6OUT |= BIT2; //Disable level conversion
LcdReset(); //Reset 1602 LCD
DispNChar(2,0,12,tishi); //Display prompt information
Disp1Char(11,1,'V'); //Display voltage unit
P6SEL |= BIT0; // Enable ADC channel
ADC12CTL0 = ADC12ON+SHT0_8+MSC; // Turn on ADC and set sampling time
// The above configuration does not turn on the internal reference voltage
//ADC12MCTLx is used to select the channel and reference voltage. This register is not configured to the default value.
// The default value is that the reference voltage is selected as AVCC (3.3V), the channel is A0, so the measurement range is 0-3.3V
ADC12CTL1 = SHP+CONSEQ_2; // Use sampling timer
//When the register configuration sample hold trigger source is selected, ADC12SC is used to collect the signal generated by the sampling timing circuit.
// The conversion mode is single-channel repeated conversion. The above settings must be set when ENC=0
ADC12IE = BIT0; // Enable ADC interrupt
ADC12CTL0 |= ENC; // Enable conversion
ADC12CTL0 |= ADC12SC; // Start conversion
_EINT(); // Enable global interrupt
LPM0;
}
/*******************************************
Function name: ADC12ISR
Function: ADC interrupt service function, here we use multiple averages
Calculate the analog voltage value of P6.0 port
Parameters: None
Return value: None
********************************************/
#pragma vector=ADC_VECTOR
__interrupt void ADC12ISR (void)
{
static uint index = 0;
results[index++] = ADC12MEM0; // Store the conversion results into the array
if(index == Num_of_Results) //If the array is full
{
flying i;
unsigned long sum = 0;
index = 0; //Start saving from the beginning, the original data will be overwritten
for(i = 0; i < Num_of_Results; i++) //Calculate and
{
sum += results[i];
}
sum >>= 5; //divide by 32
Trans_val(sum);
}
}
/*******************************************
Function name: Trans_val
Function: Convert hexadecimal ADC data into three-digit decimal
Real analog voltage data, and displayed on LCD
Parameter: Hex_Val--hexadecimal data
n--The denominator of the transformation is equal to 2 to the power of n
Return value: None
********************************************/
void Trans_val(uint Hex_Val)
{
unsigned long caltmp;
uint Curr_Volt;
fly t1,i;
float ptr[4];
caltmp = Hex_Val;
caltmp = (caltmp << 5) + Hex_Val; //caltmp = Hex_Val * 33
caltmp = (caltmp << 3) + (caltmp << 1); //caltmp = caltmp * 10
Curr_Volt = caltmp >> 12; //Curr_Volt = caltmp / 2^n
// The reference voltage is 3.3V, so the calculation formula should be Hex_val*3.3/2^n
// Multiplication and division calculations can be performed by shifting to effectively improve program running efficiency
ptr[0] = Curr_Volt / 100; //Hex->Dec conversion
t1 = Curr_Volt - (ptr[0] * 100);
ptr[2] = t1 / 10;
ptr[3] = t1 - (ptr[2] * 10);
ptr[1] = 10; //The 10th bit in the shuzi table corresponds to the symbol "."
//Display the transformed result on the LCD
for(i = 0;i < 4;i++)
Disp1Char((6 + i),1,shuzi[ptr[i]]);
}
Previous article:MSP430 Study Notes 11- Eight-way ADC acquisition Nokia 5110 LCD display
Next article:MSP430 Study Notes 9-PS2 Keyboard Decoding
Recommended ReadingLatest update time:2024-11-23 04:54
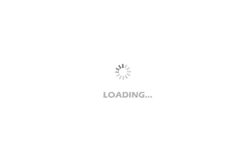
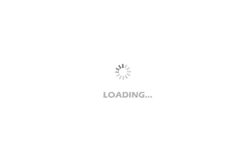
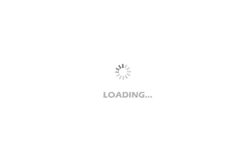
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Some misunderstandings in FPGA learning.zip
- MSP430F5438A supports communication scheme verification based on COAP protocol
- I want to design a sine wave generator.
- [RT-Thread reading notes] Part 1 Simple principles of the kernel
- ASR
- I worked hard to drive the CC2640 Hanshuo electronic tag.
- Solution to the error empty character constant
- FPGA simulates a vending machine source code
- Newbie wants to use PLC to control air conditioner
- Managing Power, Ground, and Noise in Microcontroller/Analog Applications