/******************************************************************
**
** File : SPI.c | Master Send Receive Interrupt |
** Version : 1.0
** Description: SPI interface TLC549
** Author : LightWu
** Date : 2013-4-16
**
*******************************************************************/
#include "MSP430x24x.h"
#define uint unsigned int
#define uchar unsigned char
/***设置数码管显示****/
#define L1_OFF P4OUT|=BIT0 //关L1
#define L1_NO P4OUT&=~BIT0 //点亮L1
#define L2_OFF P4OUT|=BIT1 //关L2
#define L2_NO P4OUT&=~BIT1 //点亮L2
#define L3_OFF P4OUT|=BIT2 //关L3
#define L3_NO P4OUT&=~BIT2 //点亮L3
uchar const Segment1[]={0x3f, 0x06, 0x5b, 0x4f, 0x66, 0x6d, 0x7d, 0x07, 0x7f, 0x6f}; //不带小数点编码
uchar const Segment2[]={0x40, 0x79, 0x24, 0x30, 0x19, 0x12, 0x02, 0x78, 0x00, 0x10}; //带小数点编码
uchar AdcFlag = 0;
uchar TempNum1;
uchar TempNum2;
uchar TempNum3;
unsigned char Data1;
void Display( uchar num1, uchar num2, uchar num3 );
void Delay(void)
{
uint m;
for(m=1000;m>0;m--);
}
void SpiInit(void)
{
P3SEL |= 0x0E; // P3.3,2,1 USCI_B0 option select,注意管脚配置
P3DIR |= 0x01; // P3.0 output direction
UCB0CTL0 |= UCCKPH + UCMSB + UCMST + UCSYNC; // 3-pin, 8-bit SPI mstr, MSB 1st
UCB0CTL1 |= UCSSEL_2; // SMCLK
UCB0BR0 = 0x02;
UCB0BR1 = 0;
UCB0CTL1 &= ~UCSWRST; // **Initialize USCI state machine**
IE2 |= UCB0RXIE; // 打开接收中断
}
unsigned char TLC549Read(void)
{
unsigned char Data;
P3OUT &= ~0x01; // Enable TLC549, /CS reset
UCB0TXBUF = 0x55; // Dummy write to start SPI
while (!(IFG2 & UCB0TXIFG)); // 发送完成
while (!(IFG2 & UCB0RXIFG)); // USCI_B0 RX buffer ready?
Data = UCB0RXBUF; // data = 00|DATA
P3OUT |= 0x01; // Disable TLC549, /CS set
return(Data);
}
void main(void)
{
// Stop watchdog timer to prevent time out reset
WDTCTL = WDTPW + WDTHOLD; //Turn off the watchdog
P4DIR = 0XFF; //P4 is set as output, bit code control
P4SEL = 0;
P5DIR = 0XFF; //P5 is set as output, break code control
P5SEL = 0;
P4OUT = 0XFF; //Turn off the digital tube, common anode digital tube
SpiInit();
while(1)
{
//Data1 = TLC549Read();
P3OUT &= ~0x01; // Enable TLC549, /CS reset
UCB0TXBUF = 0x55; // Transmit first character
_BIS_SR(LPM0_bits + GIE); // CPU off, enable interrupts
TempNum1 = Data1/100; //Hundreds digit
TempNum2 = Data1/10%10; //Tens digit
TempNum3 = Data1%10; //Unit
Display(TempNum1,TempNum2,TempNum3); //Display conversion value
}
}
#pragma vector=USCIAB0RX_VECTOR
__interrupt void USCIA0RX_ISR (void)
{
Data1 = UCB0RXBUF; // data = 00|DATA
P3OUT |= 0x01; // Disable TLC549, /CS set
__bic_SR_register_on_exit(LP M0_bits); // Exit LPM0
}
void Display( uchar num1, uchar num2, uchar num3 )
{
P5OUT = Segment1[ num1 ]; //
L1_NO;
Delay();
L1_OFF;
P5OUT = Segment1[ num2 ]; //
L2_NO;
Delay();
L2_OFF;
P5OUT = Segment1[ num3 ]; //
L3_NO;
Delay ();
L3_OFF;
}
Previous article:MSP430F249—SPI Master-Slave Communication
Next article:MSP430F249SPI+TLC549
Recommended ReadingLatest update time:2024-11-17 00:20
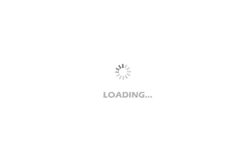
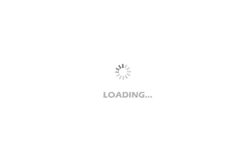
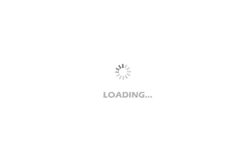
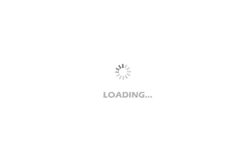
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Multi-port and shared memory architecture for high-performance ADAS SoCs
-
Digilent Vivado library
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【LoRa】LoRa development common problems 2
- Interpretation of the power amplifier circuit principle! Hand-drawn circuit diagram
- EEWORLD University Hall----Teacher Tang talks about operational amplifiers
- Tiny Raspberry Pi Pico Keyboard
- Help: How to obtain information on various packaging parameters?
- Simon Monk's new book: Programming the Pico
- Afternoon broadcast: TI's new generation low-power Bluetooth controller CC2640R2L development and application case analysis
- I used STM32MP1 to build an epidemic monitoring platform 4—Function improvement and interface redesign
- How to draw the simulation circuit diagram of a key sequencer with memory function
- EEWORLD University Hall----TI's new generation of RGB LED drivers "light up" human-computer interaction