Using a single-chip microcomputer (or single-board computer) to play music is probably one of the issues that radio enthusiasts are interested in. This article starts with the basic experiment of single-chip microcomputers, discusses the design principles of music programs, and gives specific examples for reference.
Basic pronunciation experiment of single chip microcomputer
We know that the frequency spectrum of sound ranges from about tens to thousands of hertz. If we can use a program to control the "high" or low level of a certain line of a single machine, a rectangular wave of a certain frequency can be generated on the line, and a speaker can emit a sound of a certain frequency. If we use a delay program to control the duration of the "high" and "low" levels, we can change the output frequency and thus change the tone.
For example, to generate a 200Hz audio signal, connect the speaker as shown in Figure 1 (if it is a temporary experiment, the speaker can also be directly connected to the P1 port line). The experimental procedure is:
Among them, subroutine DEL is a delay subroutine. When R3 is 1, the delay time is about 20us. R3 stores the delay constant. For 200HZ audio, its period is 1/200 second, that is, 5ms. In this way, when the duration of the high or low level of P1.4 is 2.5ms, that is, when the time constant of R3 is 2500/20=125 (7DH), a 200HZ tone can be emitted. Type the above program into the learning machine, and continuously modify the constant of R3 to feel the change of tone. In the music, each note corresponds to a certain frequency. Table 1 gives the frequency of each note in C key and its corresponding time constant. Readers can send its hexadecimal code into R3 according to the constants provided in Table 1, and practice repeatedly to experience it. According to Table 1, notes can be played. This is not enough. To play a song accurately, the rhythm of the music must be accurately controlled, that is, the duration of a note.
We can use timer T0 to control the beat of the note. By inputting different initial values, we can generate different timing times. For example, the rhythm of a song is 94 beats per minute, that is, one beat is 0.64 seconds. The corresponding relationship between other beats and time is shown in Table 2.
However, since the maximum timing time of T0 can only be 131 milliseconds, it is impossible to directly change the initial value of T0 to achieve different beats. We can use T0 to generate a 10 millisecond time base, and then set an interrupt counter to control the length of the beat time by judging the value of the interrupt counter. Table 2 also gives the time constants corresponding to various beats. For example, for a 1/4 beat note, the timing time is 0.16 seconds, and the corresponding time constant is 16 (ie 10H); for a 3-beat note, the timing time is 1.92 seconds, and the corresponding time constant is 192 (ie C0H).
We take the time constant of each note and its corresponding beat constant as a group, arrange all the constants in the music into a table in order, and then use the table lookup program to take them out in turn, generate notes and control the rhythm, so that the performance effect can be achieved. In addition, the end note and the body stop note can be represented by the codes 00H and FFH respectively. If the table lookup result is 00H, it means the end of the song; if the table lookup result is FFH, the corresponding pause effect is generated. In order to produce the rhythm of hand playing, some notes (such as two identical notes) are inserted with a slightly different frequency of one time unit.
The following is a program list that can be played directly on the TD-III learning machine. For other different models of learning machines, just change the address accordingly. This program plays the folk song "Osmanthus flowers bloom everywhere in August", in the key of C, with a rhythm of 94 beats per minute. Readers can also find a song by themselves, translate the music into a code table according to the constants given in Tables 1 and 2, and input it into the machine without changing the program. This experimental method is simple. Even for people who don't understand music, it is easy to translate an unfamiliar song into code. Learning to sing a song along with the performance of the machine is endless fun.
Program listing (omitted, please refer to the source program description).
The program flowchart is shown in Figure 2.
This course is provided by the MCU Tutorial Network. Please point out any problems.
Hardware connection instructions:
Just find a simulator or a microcontroller experiment board, as long as it works, input the program, run it, then find a speaker (there should be a pair next to your computer), unplug it, connect the front end of the plug to P1.0, and find a wire to connect the back part to the ground of the microcontroller, and there should be sound. Then it is up to you to improve the hardware connection...
Music program assembly code Code 1 -------------VoICe.asm--------------------------
ORG 0000H
LJMP START
ORG 000BH
INC 20H ;Interrupt service, interrupt counter plus 1
MOV TH0,#0D8H
MOV TL0,#0EFH ;12M crystal, forming a 10 millisecond interrupt
RETI
START:
MOV SP,#50H
MOV TH0,#0D8H
MOV TL0,#0EFH
MOV TMOD,#01H
MOV IE,#82H
MUSIC0:
NOP
MOV DPTR,#DAT ;Table header address sent to DPTR
MOV 20H,#00H ;Interrupt counter cleared to 0
MOV B,#00H ;Table number cleared to 0
MUSIC1:
NOP
CLR A
MOVC A,@A+DPTR ;Look up the table to get the code
JZ END0 ;If it is 00H, then end
CJNE A,#0FFH,MUSIC5
LJMP MUSIC3
MUSIC5:
NOP
MOV R6,A
INC DPTR
MOV A,B
MOVC A,@A+DPTR ;Get the beat code and send it to R7
MOV R7,A
SETB TR0 ;Start counting
MUSIC2:
NOP
CPL P1.0
MOV A,R6
MOV R3,A
LCALL DEL
MOV A,R7
CJNE A,20H,MUSIC2 ;Is the interrupt counter (20H) = R7?
;If not equal, continue the loop
MOV 20H,#00H ;If equal, get the next code
INC DPTR
; INC B
LJMP MUSIC1
MUSIC3:
NOP
CLR TR0 ;Pause for 100 milliseconds
MOV R2,#0DH
MUSIC4:
NOP
MOV R3,#0FFH
LCALL DEL
DJNZ R2,MUSIC4
INC DPTR
LJMP MUSIC1
END0:
NOP
MOV R2,#64H ;Song ends, delay 1 second and continue
MUSIC6:
MOV R3,#00H
LCALL DEL
DJNZ R2,MUSIC6
LJMP MUSIC0
DEL:
NOP
DEL3:
MOV R4,#02H
DEL4:
NOP
DJNZ R4,DEL4
NOP
DJNZ R3,DEL3
RET
NOP
DAT:
db 26h,20h,20h,20h,20h,20h,26h,10h,20h,10h,20h,80h,26h,20h,30h,20h
db 30h,20h,39h,10h,30h,10h,30h,80h ,26h,20h,20h,20h,20h,20h,1ch,20h
db 20h,80h,2bh,20h,26h,20h,20h,20h,2bh,10h,26h,10h,2bh,80h,26h,20h
db 30h,20h,30h,20h,39h,10h,26h,10h,26h,60h,40h,10h,39h,10h,26h,20h
db 30h,20h,30h,20h,39h,10h,26h,10h,26h, 80h,26h,20h,2bh,10h,2bh,10h
db 2bh,20h,30h,10h,39h,10h,26h,10h,2bh,10h,2bh,20h,2bh,40h,40h,20h
db 20h,10h,20h,10h,2bh,10h,26h,30h,30h,80h,18h,20h,18h,20h,26h,20h
db 20h,20h,20h,40h,26h,20h,2bh,20h,30h, 20h,30h,20h,1ch,20h,20h,20h
db 20h,80h,1ch,20h,1ch,20h,1ch,20h,30h,20h,30h,60h,39h,10h,30h,10h
db 20h,20h,2bh,10h,26h,10h,2bh,10h,26h,10h,26h,10h,2bh,10h,2bh,80h
db 18h,20h,18h,20h,26h,20h,20h,20h,20h, 60h,26h,10h,2bh,20h,30h,20h
db 30h,20h,1ch,20h,20h,20h,20h,80h,26h,20h,30h,10h,30h,10h,30h,20h
db 39h,20h,26h,10h,2bh,10h,2bh,20h,2bh,40h,40h,10h,40h,10h,20h,10h
db 20h,10h,2bh,10h,26h,30h,30h,80h,00H
END
Music program assembly code Code 2 -------------Vo IC e1.asm--------------------------
;Title 'August Osmanthus Fragrance' Voice Program
;Abstract For details, see 'Radio' Issue 3, 1992
;Author Zhou ZhenanORG
0000H
LJMP START
ORG 000BH
INC 20H ;Interrupt service, interrupt counter plus 1
MOV TH0,#0D8H
MOV TL0,#0EFH ;12M crystal oscillator , forming a 10 millisecond interrupt
RETI
START:
MOV SP,#50H
MOV TH0 ,#0D8H MOV
TL0,#0EFH
MOV TMOD,#01H
MOV IE,#82H
MUSIC0:
NOP
MOV DPTR,#DAT ;Table header address sent to DPTR
MOV 20H,#00H ;Interrupt counter cleared to 0
MOV B,#00H ;Table number cleared to 0
MUSIC1:
NOP
CLR A
MOVC A,@A+DPTR ;Look up the table to get the code
JZ END0 ;If it is 00H, then end
CJNE A,#0FFH,MUSIC5
LJMP MUSIC3
MUSIC5:
NOP
MOV R6,A
INC DPTR
MOV A,B
MOVC A,@A+DPTR ;Get the beat code and send it to R7
MOV R7,A
SETB TR0 ;Start counting
MUSIC2:
NOP
CPL P1.0
MOV A,R6
MOV R3,A
LCALL DEL
MOV A,R7
CJNE A,20H,MUSIC2 ;Is the interrupt counter (20H) = R7?
;If not equal, continue the loop
MOV 20H,#00H ;If equal, then get the next code
INC DPTR
; INC B
LJMP MUSIC1
MUSIC3:
NOP
CLR TR0 ;Pause for 100 milliseconds
MOV R2,#0DH
MUSIC4:
NOP
MOV R3,#0FFH
LCALL DEL
DJNZ R2,MUSIC4
INC DPTR
LJMP MUSIC1
END0:
NOP
MOV R2,#64H ;Song ends, delay 1 second and continue
MUSIC6:
MOV R3,#00H
LCALL DEL
DJNZ R2,MUSIC6
LJMP MUSIC0
DEL:
NOP
DEL3:
MOV R4,#02H
DEL4:
NOP
DJNZ R4,DEL4
NOP
DJNZ R3,DEL3
RET
NOP
DAT:
DB 18H, 30H, 1CH, 10H
DB 20H, 40H, 1CH, 10H
DB 18H, 10H, 20H, 10H
DB 1CH, 10H, 18H, 40H
DB 1CH, 20H, 20H, 20H
DB 1CH, 20H, 18H, 20 H
DB 20H, 80H, 0FFH, 20H
DB 30H, 1CH, 10H, 18H
DB 20H, 15H, 20H, 1CH
DB 20H, 20H, 20H, 26H
DB 40H, 20H, 20H, 2BH
DB 20H, 26H, 20 H , 20H
DB 20H, 30H, 80H, 0FFH
DB 20H, 20H, 1CH, 10H
DB 18H, 10H, 20H, 20H
DB 26H, 20H, 2BH, 20H
DB 30H, 20H, 2BH, 40H
DB 20H, 20H, 1CH, 10H
DB 18H, 10H, 20H, 20H
DB 26H, 20H, 2BH, 20H
DB 30H, 20H, 2BH, 40H
DB 20H, 30H, 1CH, 10H
DB 18H, 20H, 15H, 20H
DB 1CH, 20H, 20H , 20H
DB 26H, 40H, 20H , 20H
DB 2BH, 20H, 26H , 20H
DB 20H, 20H, 30H , 80H
DB 20H, 30H, 1CH , 10H
DB 20H, 10H, 1CH , 10H
DB 20H, 2 0H, 26H, 20H
DB 2BH, 20H, 30H, 20H
DB 2BH, 40H, 20H, 15H
DB 1FH, 05H, 20H, 10H
DB 1CH, 10H, 20H, 20H
DB 26H, 20H, 2BH, 20H
DB 30H, 20H, 2BH, 40H
DB 20H, 30H, 1CH, 10H
DB 18H, 20H, 15H, 20H
DB 1CH, 20H, 20H, 20H
DB 26H, 40H, 20H, 20H
DB 2BH, 20H, 26H, 20H
DB 20H, 20H, 30H, 30H
DB 20H, 30H, 1CH, 10H
DB 18H, 40H, 1CH, 20H
DB 20H, 20H, 26H, 40H
DB 13H, 60H, 18H, 20H
DB 15H, 40H, 13H, 40H
DB 18H, 80H, 00H
end
Previous article:UART register and UART5 initialization procedure
Next article:Working Principle and Software Design of External Expansion Memory Circuit
Recommended ReadingLatest update time:2024-11-16 13:59
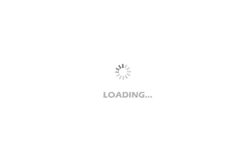
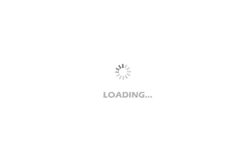
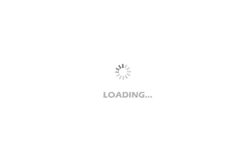
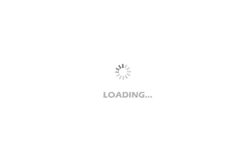
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Principle of pyroelectric infrared sensor
- Importing an old MPLAB IDE V8.9 project into MPLAB X IDE shows 'other' has different root
- [Smartwatch for environmental experts] Part 8: Code migration for personnel going down the mine
- Post count 1234, mark it here
- Open Source ESP32 Color Screen WIFI/BLE Smart Multimeter Production Process (1. Shell and Screen Selection)
- TI Automotive IC Products and Application Solutions (Free Viewing)
- B station hands-on expert shares: How to "trick" USB charger
- Differentiated innovative product-LED transparent screen
- TI's affordable Li-ion battery pack reference design for electric motorcycle batteries
- EEWORLD University Hall----Live Replay: Manufacturing Logistics Challenges of Microchip Key Security Configuration- Advantages of Discrete Security Elements