Optimization level under option -> c/c++ -> language/code genderation -> optimization
Optimization level description (for reference only):
The Code Optimization column is used to set the optimization level of C51. There are 9 optimization levels in total (as written in the book), and the high optimization level includes all the previous optimization levels. The following is a description of each level:
Level 0 optimization:
1. Constant folding: Whenever possible, the compiler performs calculations to convert expressions into constant numbers, including the calculation of running addresses.
2. Simple access optimization: Access optimization of internal data and bit addresses of the 8051 system.
3. Jump optimization: The compiler always postpones jumps to the final target, so the commands between jumps are deleted.
Level 1 optimization:
1. Dead code elimination: Useless code segments are eliminated.
2. Jump rejection: Based on a test backtrace, conditional jumps are carefully checked to determine whether they can be simplified or deleted.
Level 2 optimization:
1. Data coverage: Data and bit segments suitable for static coverage are identified and marked. The connection locator BL51 selects segments that can be statically covered by analyzing the global data flow.
Level 3 optimization:
1. "Peephole" optimization: Remove redundant MOV commands, including unnecessary operations of loading objects and constants from memory. In addition, if it can save storage space or program execution time, complex operations will be replaced by simple operations.
Level 4 optimization:
1. Register variables: Make automatic variables and function parameters in working registers as much as possible, and no data memory space will be reserved for these variables whenever possible.
2. Extended access optimization: Variables from IDATA, XDATA, PDATA and CODE areas are directly included in the operation, so most of the time there is no need to load them into intermediate registers.
3. Local common sub-expression elimination: If there is a repeated calculation in the expression, the result of the first calculation is saved and will be used for subsequent calculations whenever possible, so complex calculations can be eliminated from the code. 4.
CASE/SWITCH statement optimization: Optimize CASE/SWITCH statements as jump tables or jump strings.
Level 5 optimization:
1. Global common sub-expression elimination: Whenever possible, the same sub-expression inside the function is calculated only once. The intermediate result is stored in a register to replace the new calculation.
2. Simple loop optimization: loops that occupy a section of memory with constants are optimized when they are run again.
Level 6 optimization:
1. Loop loop: if the program code can be executed faster and more efficiently, the program loop will loop.
Level 7 optimization:
1. Extended entry optimization: use DPTR data pointers for register variables when appropriate, pointer and array access are optimized to reduce program code and increase execution speed.
Level 8 optimization:
1. Common tail merge: when there are multiple calls to the same function, some setup code can be reused, thereby reducing the length of the program code.
Level 9 optimization:
1. Common subroutine blocks: detect reused instruction sequences and convert them into subroutines. C51 will even rearrange the code to obtain more reused instruction sequences.
Of course, the optimization level is not the higher the better, it should be selected appropriately according to specific requirements.
In-depth analysis of Keil C51 bus peripheral operation problems
After reading an article titled "Keil C51's Continuous Reading Method for the Same Port" (original text) in the "Experience Exchange" column of the 10th issue of "Microcontrollers and Embedded System Applications" magazine in 2005, the author believes that the article did not conduct an in-depth and accurate analysis of the problem, and the two solutions mentioned in the article are not direct and simple. The author believes that this is not a problem that Keil C51 cannot handle continuous reading and writing of a port, but a problem of not being familiar enough with the use of Kei1 C51 and not being detailed enough in the design, so this article was written.
This article proposes three solutions to the problems mentioned in the original article that are different from the original ones. Each method is more direct and simpler than the method mentioned in the original article, and the design is more standardized. (No criticism intended, please forgive the original author)
1 Review and analysis of the problem
The original article mentioned: In actual work, when the same port was read repeatedly and continuously, the Keil C51 compilation did not achieve the expected results. The original author analyzed the assembly program compiled by C and found that the second read statement of the same port was not compiled. Unfortunately, the original author did not analyze the reason for the failure to compile, but hurriedly used some less standardized methods to test out two solutions.
For this problem, it is easy to find out by reading the manual of Keil C51: KeilC51 compiler has an optimization setting. Different optimization settings will produce different compilation results. Generally, the default compilation optimization setting is set to level 8 optimization, and the actual maximum can be set to level 9 optimization:
1. Dead code elimination.
2. Data overlaying.
3. Peephole optimization.
4. Register variables.
5. Common subexpression elimination.
6. Loop rotation.
7. Extended Index Access Optimizing.
8. Reuse Common Entry Code.
9. Common Block Subroutines.
The above problems are caused by Keil C51 compiler optimization. Because in the original program, the peripheral address is directly defined as follows:
unsigned char xdata MAX197 _at_ 0x8000
Use _at_ to define the variable MAX197 to the external expansion RAM address 0x8000. Therefore, Keil C51 optimization compiler naturally thinks that repeating the second read is useless, and the result of the first read is enough, so the compiler skips the second read statement. At this point, the problem is clear at a glance.
2 Solutions
It is easy to come up with a good solution from the above analysis.
2.1 The simplest and most direct solution
The program does not need to be modified at all, just set the Keil C51 compilation optimization selection to 0 (no optimization). Select Target in the project window, then open the "Options for Target" setting dialog box, select the "C51" tab, and select "0: Costant folding" in the "Code Optimiztaion" "Level". After compiling again, you will find that the compilation results are:
CLR MAXHBEN
MOV DPTR,#MAX197
MOVX A,@DPTR
MOV R7,A
MOV down8,R7
SETB MAXHBEN
MOV DPTR,#MAX197
MOVX A,@DPTR
MOV R7,A
MOV up4,R7
Both read operations are compiled.
2.2 The best method
Tell Keil C51 that this address is not a general extended RAM, but a connected device with "volatile" characteristics, and each read is meaningful. You can modify the variable definition and add the "volatile" keyword to describe its characteristics:
unsigned char volatile xdata MAX197 _at_ 0x8000;
You can also include the system header file in the program; "#include", and then modify the variable in the program and define it as a direct address:
#define MAX197 XBYTE
In this way, the Keil C51 settings can still retain advanced optimization, and in the compilation results, the same two reads will not be skipped by optimization.
Appendix: Optimization level and optimization effect level in Keil C51 Description
0 Constant merging: The compiler pre-calculates the result and replaces the expression with constants as much as possible. Including running address calculation.
Optimize simple access: The compiler optimizes access to internal data and bit addresses of the 8051 system.
Jump optimization: The compiler always expands the jump to the final target, and multi-level jump instructions are deleted.
1 Dead code removal: Unused code segments are deleted.
Reject jump: Strictly check conditional jumps to determine whether the test logic can be inverted to improve or delete.
2 Data coverage: Data and bit segments suitable for static coverage are determined and internally marked. The BL51 connector/locator can select segments that can be covered through global data flow analysis.
3 Peephole optimization: Eliminate redundant MOV instructions. This includes unnecessary loads from storage areas and constant load operations. When storage space or execution time can be saved, replace complex operations with simple operations.
4 Register variables: Automatic variables and function parameters are allocated to registers when possible. The storage area reserved for these variables is omitted.
Optimize extended access: Variables of IDATA, XDATA, PDATA and CODE are directly included in the operation. It is not necessary to use intermediate registers most of the time.
Local common subexpression elimination: If the same calculation is repeated with an expression, the result of the first calculation is saved and may be used later. Redundant calculations are eliminated.
Case/Switch optimization: Code containing SWITCH and CASE is optimized to jump tables or jump queues.
5 Global common subexpression elimination: The same subexpression within a function is calculated only once when possible. Intermediate results are saved in registers and used in a new calculation.
Simple loop optimization: Loop programs that fill storage areas with a constant are modified and optimized.
6 Loop optimization: The program optimizes loops if the resulting program code is faster and more efficient.
7 Extended index access optimization: Use DPTR for register variables when appropriate. Pointer and array access are optimized for execution speed and code size.
8 Common tail merging: When a function has multiple calls, some setup code can be reused, thus reducing program size.
9 Common block subroutines: Detect loop instruction sequences and convert them into subroutines. Cx51 even rearranges the code to get larger loop sequences.
Previous article:Keil compilation error about the use of __use_no_semihosting_swi
Next article:Keil uVision4 code editor Chinese characters garbled problem
Recommended ReadingLatest update time:2024-11-16 03:21
![[C51 self-study notes] Flashing light + running light (four methods) + 74HC573 chip + keil4 software simulation (debug)](https://6.eewimg.cn/news/statics/images/loading.gif)
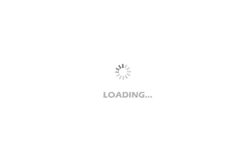
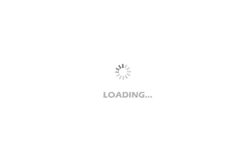
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University - Brushless Motor Tutorial
- Introduction to FPGA design process and tool software
- EEWORLD University----[High Precision Laboratory] Interface: RS-485
- 【Perf-V Review】VIVADO Flowing Light
- [The third stop of Shijian’s ADI journey] Learn about cutting-edge IoT devices and solutions, and win Kindle and other gifts
- Are there any netizens working in the field of motors?
- Evaluation report summary: Mir MYS-8MMX
- 2020 Share the beauty of Qingdao in my eyes
- Zigbee Technology Exchange
- ST's new 400W power board