We have talked about two commonly used serial ports, UART and SPI. This time, we will talk about another commonly used serial port: IIC (I2C) communication.
Popular Science IIC: Generally there are two signal lines, one is the bidirectional data line SDA, the other is the clock line SCL. All serial data SDA connected to the IIC bus device is connected to the bus SDA, and the clock line SCL of each device is connected to the bus SCL.
Communication process:
In master mode, the IIC interface starts data transmission and generates a clock signal. Serial data transmission always starts with a start condition and ends with a stop condition. Both the start condition and the stop condition are generated by software control in master mode.
In slave mode, the IIC interface can recognize its own address (7 or 10 bits) and the general call address. The software can control the opening or closing of the recognition of the general call address.
Data and address are transmitted in 8 bits/byte, with the high bit first. The 1 or 2 bytes following the start condition are the address (1 byte in 7-bit mode and 2 bytes in 10-bit mode). The address is only sent in master mode.
During the 9th clock after 8 clocks of a byte transmission, the receiver must send back an acknowledge bit (ACK) to the transmitter. Refer to the figure below.
For detailed protocol, please refer to: http://blog.csdn.net/subkiller/article/details/6854910
Similar to SPI, using IIC is nothing more than initialization, data sending, and data receiving, three major functions.
Initialization is divided into master and slave, but generally the microcontroller that communicates with external chips acts as the master.
void IIC_Master_Init(void)
{
CLK_PCKENR1 |= 0x01; //Enable IIC peripheral clock
PB_DDR &= 0xcf;
PB_CR1 &= 0xcf;
PB_CR2 &= 0xcf;
I2C_CR1 = 0x00; //Allow clock stretching, disable broadcast call, disable iic
I2C_FREQR = 0x01; //Input clock frequency 8MHz
I2C_OARH = 0x40; // seven-bit address mode
I2C_OARL = 0xa0; //Own address 0xa0
I2C_CCRL = 0xff; //
I2C_CCRH = 0x00; //Standard mode
I2C_TRISER = 0x02;
I2C_CR1 |= 0x01; // Enable iic peripheral
}
Here we use the IIC pins PB4 and PB5 on the STM8S105 chip. In addition, we need to enable the IIC clock.
Here we only give a simple example of sending and receiving data, because different external chips have different communication methods, but generally they are:
Read operation: Start -> Send peripheral address -> Start -> Send register address to be read -> Read one byte -> (may read another byte) -> ... -> End
Write operation: Start -> Send peripheral address -> Send register address to be written -> Write one byte ->
void IIC_Write_Byte(u8 DeviceAddress, u8 Address, u8 Data)
{
vu8 temp = 0;
while((I2C_SR3 & 0x02) != 0); //Wait for the IIC bus to be idle
IIC_Start();
while((I2C_SR1 & 0x01) == 0); //EV5, the start signal has been sent
I2C_DR = (DeviceAddress & 0xfe); // Send the physical address of the iic slave device, the lowest bit is 0, write operation
while((I2C_SR1 & 0x02) == 0); //The address has been sent
temp = I2C_SR1; //Clear ADDR flag
temp = I2C_SR3;
while((I2C_SR1 & 0x80) == 0); //Wait for the send register to be empty
I2C_DR = Address; //Send the register address to be written
while((I2C_SR1 & 0x04) == 0); //Wait for sending to complete
while((I2C_SR1 & 0x80) == 0); //Wait for the send register to be empty
I2C_DR = Data; //Send the data to be written
while((I2C_SR1 & 0x04) == 0); //Wait for sending to complete
temp = I2C_SR1; //Clear BTF flag
temp = I2C_DR;
IIC_Stop(); //Send stop signal
}
unsigned char IIC_Read_Byte(u8 DeviceAddress, u8 Address)
{
vu8 temp = 0;
short read_data = 0;
while((I2C_SR3 & 0x02) != 0); //Wait for the IIC bus to be idle
I2C_CR2 |= 0x04; //Enable ACK
IIC_Start();
while((I2C_SR1 & 0x01) == 0); //EV5, the start signal has been sent
I2C_DR = (DeviceAddress & 0xfe); // Send the physical address of the iic slave device, the lowest bit is 0, write operation
while((I2C_SR1 & 0x02) == 0); //The address has been sent
temp = I2C_SR1; //Clear ADDR flag
temp = I2C_SR3;
while((I2C_SR1 & 0x80) == 0); //Wait for the send register to be empty
I2C_DR = Address; // Send the register address to be read
while((I2C_SR1 & 0x04) == 0); //Wait for data to be sent
IIC_Start();
while((I2C_SR1 & 0x01) == 0); //EV5, the start signal has been sent
I2C_DR = (DeviceAddress | 0x01); // Send the physical address of the IIC slave device, the lowest bit is 1, read operation
while((I2C_SR1 & 0x02) == 0); //The address has been sent
temp = I2C_SR1; //Clear ADDR flag
temp = I2C_SR3;
while((I2C_SR1 & 0x40) == 0); //Wait for the receive data register to be non-empty
read_data = I2C_DR;
I2C_CR2 &= 0xfb; //When reading data, sending stop must disable ack to release the slave
temp = I2C_SR1; //Clear BTF flag
temp = I2C_DR;
IIC_Stop();
return read_data;
}
Attached is the project of stm8s in IAR environment, including the initialization code of on-chip hardware such as SPI, IIC, PWM, AWU, USART, EEPROM, etc.
http://download.csdn .NET /detail/devintt/9454188
Read operation: Start -> Send peripheral address -> Start -> Send register address to be read -> Read one byte -> (may read another byte) -> ... -> End
Previous article:stm8s development (nine) Use of EEPROM: Use EEPROM to store data!
Next article:stm8s development (VII) Use of SPI: SPI host communication!
Recommended ReadingLatest update time:2024-11-16 16:33
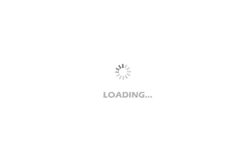
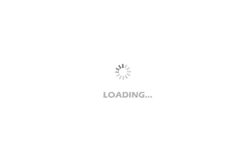
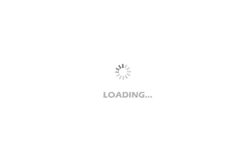
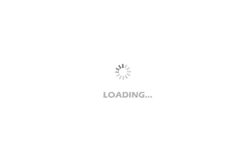
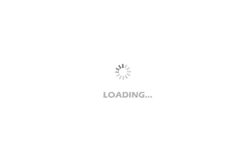
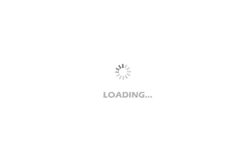
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- One watt of GaN is less than one yuan, and it is free shipping by SF Express? Lenovo has launched a price war on GaN.
- B-U585I-IOT02A uses WIFI function
- How many read and write clock cycles does SRAM have?
- Mini OLED Game Console
- How does a trace moisture meter perform for factory equipment testing?
- Does anyone have the IPM driver board?
- Oximeter Principle
- Those sneaky tech giants
- Playing with Zynq Serial 26 - Functional Simulation of PL in Vivado
- EMC testing of Bluetooth electronic products powered by lithium batteries