When used, the PA2 port needs to be set to push-pull output to control an external power switch. The port initialization procedure is as follows:
GPIO_DeInit(GPIOA);
GPIO_Init(GPIOA,GPIO_PIN_2,GPIO_MODE_OUT_PP_HIGH_SLOW);
After the setting is completed, the port will immediately output a high level, so another sentence is added:
GPIO_WriteLow(GPIOA,GPIO_PIN_2);
After completion, it was found that the powered device would be triggered when it was powered on for the first time, and the program did not output a high level on PA2. This powered device is equipped with a power supply battery and will start if a high level is detected on the port.
Using an oscilloscope to observe the PA2 port, I found that there was a short pulse at the moment of power-on, which seemed to be the problem. I checked the source code of the library function:
void GPIO_Init(GPIO_TypeDef* GPIOx, GPIO_Pin_TypeDef GPIO_Pin, GPIO_Mode_TypeDef GPIO_Mode)
{
assert_param(IS_GPIO_MODE_OK(GPIO_Mode));
assert_param(IS_GPIO_PIN_OK(GPIO_Pin));
/* Reset corresponding bit to GPIO_Pin in CR2 register */
GPIOx->CR2 &= (uint8_t)(~(GPIO_Pin));
/*-----------------------------*/
/* Input/Output mode selection */
*-----------------------------*/
if ((((uint8_t)(GPIO_Mode)) & (uint8_t)0x80) != (uint8_t)0x00) /* Output mode */
{
if ((((uint8_t)(GPIO_Mode)) & (uint8_t)0x10) != (uint8_t)0x00) /* High level */
{
GPIOx->ODR |= (uint8_t)GPIO_Pin;
}
else /* Low level */
{
GPIOx->ODR &= (uint8_t)(~(GPIO_Pin));
}
/* Set Output mode */
GPIOx->DDR |= (uint8_t)GPIO_Pin;
}
else /* Input mode */
{
/* Set Input mode */
GPIOx->DDR &= (uint8_t)(~(GPIO_Pin));
}
/*------------------------------------------------------------------------*/
/* Pull-Up/Float (Input) or Push-Pull/Open-Drain (Output) modes selection */
/*------------------------------------------------------------------------*/
if ((((uint8_t)(GPIO_Mode)) & (uint8_t)0x40) != (uint8_t)0x00) /* Pull-Up or Push-Pull */
{
GPIOx->CR1 |= (uint8_t)GPIO_Pin;
}
else /* Float or Open-Drain */
{
GPIOx->CR1 &= (uint8_t)(~(GPIO_Pin));
}
/*-----------------------------------------------------*/
/* Interrupt (Input) or Slope (Output) modes selection */
/*-----------------------------------------------------*/
if ((((uint8_t)(GPIO_Mode)) & (uint8_t)0x20) != (uint8_t)0x00) /* Interrupt or Slow slope */
{
GPIOx->CR2 |= (uint8_t)GPIO_Pin;
}
else /* No external interrupt or No slope control */
{
GPIOx->CR2 &= (uint8_t)(~(GPIO_Pin));
}
}
From the source code, CR2 is cleared first. Then write the DDR direction register 1 for output, 0 for input, and then CR1. This can be used with DDR to determine 4 modes: when DDR is 0, CR1 is 0 for floating input, when CR1 is 1 for pull-up input, when DDR is 1, CR1 is 0 for open-drain output, and when CR1 is 1 for push-pull output. When DDR is 0, CR1 is the switch for external interrupts, 1 is on, 0 is off, and when DDR is 1, it controls the slew rate. If a steeper edge is required, it can be set to 1.
I don't know why this happens. Hardware simulation found that CR1 will be pulled high after it is set. Later, I used the register method to set it normally. The code is as follows:
GPIOA->CR2 |= 0x04;
GPIOA->DDR |= 0x04;
GPIOA->CR1 |= 0x04;
Previous article:STM8s window watchdog
Next article:STM8S103F3--EEPROM
Recommended ReadingLatest update time:2024-11-16 12:26
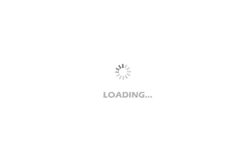
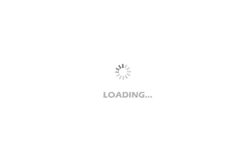
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- I have some hesitations about job hopping, I hope you can give me some advice
- Tips for you: How to design an excellent 5G small base station
- Essential knowledge about gas detectors
- How to Optimize DSP Power Budget
- Sketching the halogen lamp driver power supply schematic diagram solution
- Evaluation Weekly Report 20220926: Python Programming Learning Main Control Board, Hangkong Board, Infineon PAS CO2 Sensor Waiting for You to Test~
- [Environmental Expert's Smart Watch] Part 5: Data Communication Design between Devices
- Pocket Experiment Platform for MSP430G2553
- Urgent, help, please help me analyze the circuit, I am confused
- The national competition topic has been released. Have you chosen your topic yet?