There are 8 timers in STM32F103ZET6, including 6 advanced timers including TIM1-TIM5 and TIM8.
The timer cascade function needs to be used here. Pages P388 and P399 of ST's RM0008 REV12 explain how to select the cascade function for a specific timer. See Table 86.
The timer that outputs PWM here is TIM2, and the idle timer is TIM3. TIM2 is the master timer, and TIM3 is the slave timer to count the output pulses of TIM2.
From the table, we can see that TIM3 selects TIM2 as the trigger source for the slave timer, and needs to configure TS=001, that is, select ITR1.
To achieve the function of controlling the number of PWM outputs through the timer, there can be one of the following configuration methods:
void TIM2_Master__TIM3_Slave_Configuration(u32 PulseFrequency)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
u16 nPDTemp ;
/* -----------------------------------------------------------------------
TIMx Configuration: generate 4 PWM signals with 4 different duty cycles:
TIMxCLK = 72 MHz, Prescaler = 0x0, TIMx counter clock = 72 MHz
TIMx ARR Register = 0 => TIMx Frequency = TIMx counter clock/(ARR + 1)
TIMx Frequency = 72MHz.
----------------------------------------------------------------------- */
TIM_Cmd(TIM2, DISABLE);
nPDTemp = 72000000UL/PulseFrequency;
// Time base configuration: Configure PWM output timer - TIM2
/* Time base configuration */
TIM_TimeBaseStructure.TIM_Period = nPDTemp-1;
TIM_TimeBaseStructure.TIM_Prescaler = 0;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
// Output configuration: Configure PWM output timer - TIM2
/* PWM1 Mode configuration: Channel1 */
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = nPDTemp>>1;//50%
TIM_OC1Init(TIM2, &TIM_OCInitStructure);
TIM_OC1PreloadConfig(TIM2, TIM_OCPreload_Enable);
// Time base configuration: Configure pulse count register - TIM3
TIM_TimeBaseStructure.TIM_Period = 0xFFFF;
TIM_TimeBaseStructure.TIM_Prescaler = 1;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure);
/* Output Compare Active Mode configuration: Channel1 */
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_Inactive;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = 0xFFFF; // The configuration value here is not very meaningful
TIM_OC1Init(TIM3, &TIM_OCInitStructure);
//Configure TIM2 as the master timer
/* Select the Master Slave Mode */
TIM_SelectMasterSlaveMode(TIM2, TIM_MasterSlaveMode_Enable);
/* Master Mode selection */
TIM_SelectOutputTrigger(TIM2, TIM_TRGOSource_Update);
//Configure TIM3 as a slave timer
/* Slave Mode selection: TIM3 */
TIM_SelectSlaveMode(TIM3, TIM_SlaveMode_Gated);
TIM_SelectInputTrigger(TIM3, TIM_TS_ITR1);
TIM_ITConfig(TIM3, TIM_IT_CC1, ENABLE);
TIM_Cmd(TIM2, DISABLE);
TIM_Cmd(TIM3, DISABLE);
}
The interrupt service routine is as follows:
u8 TIM2_Pulse_TIM3_Counter_OK = 0;
void TIM3_IRQHandler(void)
{
if (TIM_GetITStatus(TIM3, TIM_IT_CC1) != RESET)
{
TIM_ClearITPendingBit(TIM3, TIM_IT_CC1); // Clear interrupt flag
TIM_Cmd(TIM2, DISABLE); //Disable the timer
TIM_Cmd(TIM3, DISABLE); //Disable the timer
TIM2_Pulse_TIM3_Counter_OK = 1;
}
}
The application is:
u16 pulsecnt = 10000;
void main(void)
{
SystemSetup(); // Initialize the kernel and peripherals
TIM2_Master__TIM3_Slave_Configuration(10000); //Configure TIM2 pulse output to 10k
while(1)
{
TIM_ITConfig(TIM3, TIM_IT_CC1, DISABLE); /* TIM enable counter */
TIM_Cmd(TIM3, ENABLE);
TIM3->CCR1 = pulsecnt;
TIM3->CNT = 0;
TIM_ITConfig(TIM3, TIM_IT_CC1, ENABLE);
TIM_Cmd(TIM2, ENABLE); /* TIM enable counter */
while(TIM2_Pulse_TIM3_Counter_OK == 0);
}
}
In this configuration, the TIM3 comparison interrupt is used. I haven't tried other methods yet, but I think they should work, such as using the timer update interrupt...
Previous article:STM32 FSMC configuration SRAM
Next article:STM32|4-20mA output circuit
Recommended ReadingLatest update time:2024-11-23 10:58
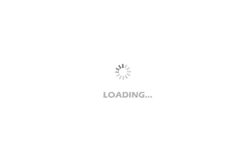
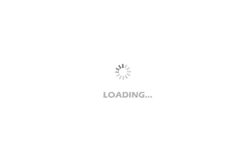
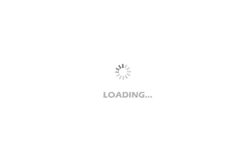
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
Intelligent Control Technology of Permanent Magnet Synchronous Motor (Written by Wang Jun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- FPGA Advanced Timing Synthesis Tutorial.pdf
- Receiving sensitivity index analysis
- MOSFET selection for USB PD+TYPE-C fast charging power supply
- The positive and negative ends of the op amp are not equal
- About CRC check
- Application of RF technology in wireless communication
- The dog days of summer have arrived
- Application of Single Chip Microcomputer Frequency Measurement in Power System Automatic Devices
- AX5201 is used as an LED driver circuit with 4.5V input and 12V output, and it is likely to be damaged.
- How to deal with impedance continuity of PCB traces?