Display custom characters
The steps are as follows:
1. Write the custom characters into CGRAM first; 2. Send the custom characters in CGRAM to DDRAM for display.
It is very simple: look
at the CGROM character code table of LCD1602, you can find that the content from 00000000B~00000111B (00H~07H) address is not defined, it is left for the user to define. The user can define the content in CGRAM of LCD1602 first, and then call the custom characters in the same way as calling CGROM characters (here is a tip, up to 8 custom characters can be written), so how to set the content in CGRAM?
First, we need to extract the "font" corresponding to the 5X8 dot matrix of the character to be written. We can extract it through related software or manually. To put it simply, the dots that are displayed in a row of the dot matrix are represented by 1, and the dots that are not displayed are represented by 0, so as to form the font data corresponding to the row.
To set the content of CGRAM, you need to set it line by line. Each row corresponds to a CGRAM. 5X8 dot matrix, 5 dots per row, a total of 8 rows, so the font data of 8 rows should be written into CGRAM. After writing, you can call it like
calling CGROM characters.
Define the content of a row in two steps:
1. Set the row address (CGRAM address):
The commands used are as follows:
RS R/W DB7 DB6 DB5 DB4 DB3 DB2 DB1 DB0
0 0 0 1 DATA
Among them: DB5, DB4, DB3 are character numbers, that is, the character addresses you will use when you want to display the character in the future.
DB2, DB1, DB0 are row numbers.
2. Set the CGRAM data (content) command code as follows:
RS R/W DB7 DB6 DB5 DB4 DB3 DB2 DB1 DB0
1 0 DATA
Among them: DB4, DB3, DB2, DB1, DB0 correspond to the font data of 5 dots per line.
DB7, DB6, DB5 can be any data, generally "000".
1 #include
2 __CONFIG(1,XT); //Crystal oscillator is external 4M
3 __CONFIG(2,WDTDIS); //watchdog off
4 __CONFIG(4,LVPDIS); //Disable low voltage programming
5
6 #define RSPIN RB5 //Data or Instrument Select
7 #define RWPIN RB4 //Write or Read
8 #define EPIN RB3 //6800 mode Enable single
9 unsigned char table[]="LCD check ok"; //The content to be displayed is put into the array table1
10
11 //This function defines eight characters to be written into eight addresses of CGRAM respectively
12 unsigned char pic[]={
13 0x03,0x07,0x0f,0x1f,0x1f,0x1f,0x1f,0x1f,
14 0x18,0x1E,0x1f,0x1f,0x1f,0x1f,0x1f,0x1f,
15 0x07,0x1f,0x1f,0x1f,0x1f,0x1f,0x1f,0x1f,
16 0x10,0x18,0x1c,0x1E,0x1E,0x1E,0x1E,0x1E,
17 0x0f,0x07,0x03,0x01,0x00,0x00,0x00,0x00,
18 0x1f,0x1f,0x1f,0x1f,0x1f,0x0f,0x07,0x01,
19 0x1f,0x1f,0x1f,0x1f,0x1f,0x1c,0x18,0x00,
20 0x1c,0x18,0x10,0x00,0x00,0x00,0x00,0x00
twenty one
twenty two };
twenty three ///---------------------------------------
24 //Name: Delay function
25 //----------------------------------------
26 void delay(unsigned int t)
27 {
28 unsigned int i,j;
29 for(i=0;i 30 { 31 for(j=0;j<10;j++); 32 } 33 } 34 35 //------------------------------------------ 36 //Name: 1602 busy detection function 37 //---------------------------------------- 38 void lcd_wait_busy(void) 39 { 40 TRISD7=1; //Prepare for reading status 41 RSPIN=0; //Select instruction register 42 RWPIN=1; //Select read 43 EPIN=1; //Enable line level change 44 while(RD7==1); //Read busy status, exit when not busy 45 EPIN=0; //Restore the enable line level 46 TRISD7=0; 47 } 48 //------------------------------------------ 49 //Name: 1602 write command function 50 //---------------------------------------- 51 void lcd_write_com(unsigned char combuf) 52 { 53 RSPIN=0; //Select instruction register 54 RWPIN=0; //Select write 55 PORTD=combuf; //Send the command word into RD 56 EPIN=1; //Enable line level change, command sent to 1602 8-bit data port 57 asm("NOP"); 58 EPIN=0; //Restore the enable line level 59 } 60 //------------------------------------------ 61 //Name: 1602 write command function (with busy detection) 62 //---------------------------------------- 63 void lcd_write_com_busy(unsigned char combuf) 64 { 65 lcd_wait_busy(); //Call busy detection function 66 lcd_write_com(combuf); //Call write command function 67 } 68 //------------------------------------------ 69 //Name: 1602 write data function (with busy detection) 70 //---------------------------------------- 71 void lcd_write_data(unsigned char databuf) 72 { 73 lcd_wait_busy(); //Call busy detection function 74 RSPIN=1; //Select data register 75 RWPIN=0; //Select write 76 PORTD = databuf; // send the data word to P2 77 EPIN = 1; // Enable line level change, command sent to 1602 8-bit data port 78 asm("NOP"); 79 EPIN=0; //Restore the enable line level 80 } 81 //------------------------------------------ 82 //Name: 1602 display address write function 83 //---------------------------------------- 84 void lcd_write_address(unsigned char x, unsigned char y) 85 { 86 x&=0x0f; //Column address is limited to 0-15 87 y&=0x01; //Row address is limited to 0-1 88 if(y==0x00) 89 lcd_write_com_busy(x|0x80); //write the column address of the first row 90 else 91 lcd_write_com_busy((x+0x40)|0x80); //Write the column address of the second row 92 } 93 //------------------------------------------ 94 //Name: 1602 initialization function 95 //---------------------------------------- 96 void lcdreset(void) 97 { 98 lcd_write_com_busy(0x38); //8-bit data, double column, 5*7 font 99 lcd_write_com_busy(0x08); //Display function off, no cursor 100 lcd_write_com_busy(0x01); // clear screen command 101 lcd_write_com_busy(0x06); //After writing new data, the cursor moves right but the display screen does not move 102 lcd_write_com_busy(0x0c); //Display function on, no cursor, 103 } 104 //------------------------------------------ 105 //Name: Specify address write function 106 //---------------------------------------- 107 void lcd_write_char(unsigned char x, unsigned char y, unsigned char buf) 108 { 109 lcd_write_address(x,y); //write address 110 lcd_write_data(buf); //Write display data 111 } 112 113 //Display string, cannot be applied accurately yet 114 void lcd1602_write_character(unsigned char add,unsigned char *p) 115 { 116 lcd_write_com_busy(add); 117 while (*p!='\0') 118 { 119 lcd_write_data(*p++); 120 } 121 } 122 123 //Write custom characters into CGRAM 124 //add is the address of CGRAM (0~7) 125 //*pic_num points to the first address of an 8-bit group 126 void lcd1602_write_pic(unsigned char add,unsigned char pic_num) 127 { 128 unsigned char i; 129 add=add<<3; 130 for(i=0;i<8;i++) 131 { 132 lcd_write_com_busy(0x40|add+i); //Set the row address, DB6=1, so the address must be added with 0X40; 133 lcd_write_data(pic_num); 134 } 135 } 136 137 //------------------------------------------ 138 //Name: Main function 139 //---------------------------------------- 140 void main(void) 141 { 142 char i,m; 143 ADCON1=0X06; //All IO are digital ports, analog input is prohibited 144 TRISB=0B11000111; //RB3-5 is set as output 145 TRISD=0B00000000; //RD is set as output 146 lcdreset(); //Reset 1602 147 lcd_write_char(6,0,0x31); //1 148 lcd_write_char(7,0,0x36); //6 149 lcd_write_char(8,0,0x30); //0 150 lcd_write_char(9,0,0x32); //2 151 lcd_write_char(10,0,0x35); //5 152 lcd_write_char(11,0,'g'); //g 153 lcd_write_char(12,0,'o'); //o 154 lcd_write_char(13,0,'o'); //o 155 lcd_write_char(14,0,'d'); //d 156 lcd_write_com_busy(0x80+0x44); //8. Reset the display address to 0xc0, which is the 0th position in the bottom row 157 for(m=0;m<12;m++) //Write the data in table1[] into 1602 for display 158 { 159 lcd_write_data(table[m]); 160 } 161 //Write eight custom characters to CGRAM 162 lcd_write_com_busy(0x40); //7. Set CGRAM address to 0100; 0x06 163 for(i=0;i<64;i++) //Write the heart-shaped code into CGRAM 164 lcd_write_data(pic[i]);//lcd1602_write_pic(i,pic[i]); 165 //Display eight custom characters 166 lcd_write_com_busy(0x80); //Set the upper display position 167 for(i=0;i<0x04;i++)//Display the upper part of the heart-shaped pattern 168 lcd_write_data(i); 169 lcd_write_com_busy(0xc0); ////Transfer the display coordinates to the corresponding positions in the lower and upper rows 170 for(i=4;i<0x08;i++) 171 lcd_write_data(i); 172 while(1) 173 { 174 175 } 176 } This is the third step to draw the custom characters using 4-wire data transmission #define lcd1602_io_com lcd1602_rs=0;
. When the refresh rate of the LCD screen is not high, we can use another 4-wire data transmission of 1602 to reduce the number of io ports used. I hope you like it. Since I didn't find any relevant information, I just posted the program here for your reference.
#include
#include
sbit lcd1602_rs=P0^7;
sbit lcd1602_rw=P0^6;
sbit lcd1602_e=P0^5;
/*#define lcd1602_io_writ lcd1602_rw=0;
#define lcd1602_io_read lcd1602_rw=1;
#define lcd1602_io_dat lcd1602_rs=1;
#define lcd1602_io_able lcd1602_e=0; #define lcd1602_io_unable lcd1602_e=1
;
*/
sbit lcd1602_dat1= P0^0;
sbit lcd1602_dat2=P0^1;
sbit lcd1602_dat3=P0^2 ;
sbit lcd1602_dat4=P0^3;
//unsigned char code table[]="ball hahhahhaha";
//unsigned char code table1[]="THE BEST!";
void delay(unsigned int z)
{
unsigned int a,b ;
for(a=z;a>0;a--)
for(b=114;b>0;b--);
}
void lcd1602_write(bit cd,unsigned char dat)//cd=0, command; c
{
int i;
unsigned char j;
lcd1602_rs=cd;
for(j=0;j<2;j++)
{
lcd1602_e=1;
for(i=0;i<200;i++)
_nop_();
lcd1602_dat4=dat&0x80;
lcd1602_dat3= (dat<<1)&0x80;
lcd1602_dat2=(dat<<2)&0x80;
lcd1602_dat1=(dat<<3)&0x80;
lcd1602_e=0;
//lcd1602_e=1;
dat<<=4;
}
}
/*unsigned char lcd1602_read_dat(unsigned char add)
{
unsigned char dat;
return dat;
}*/
void main()
{
lcd1602_rw=0;
lcd1602_e=0;
lcd1602_write( 0,0x28);
lcd1602_write(0,0x0f);
lcd1602_write(0,0x06);
lcd1602_write(0,0x01);
lcd1602_write(0,0x80);
lcd1602_write(1,'q');
while(1);
}
Previous article:PIC18FXX2 INT0 interrupt
Next article:PIC18F4521602 simple display rules
Recommended ReadingLatest update time:2024-11-15 10:31
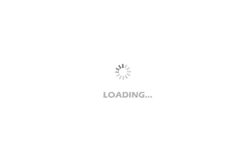
![[51 MCU] The functions of 1602 CGRAM, CGROM and DDRAM](https://6.eewimg.cn/news/statics/images/loading.gif)
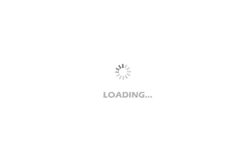
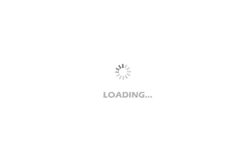
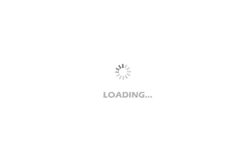
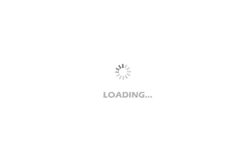
- Popular Resources
- Popular amplifiers
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- How to turn on the internal enable of Panasonic servo drive?
- What is the rigidity setting of Panasonic servo drive?
- How to change the inertia ratio of Panasonic servo drive
- What is the inertia ratio of the servo motor?
- Is it better for the motor to have a large or small moment of inertia?
- What is the difference between low inertia and high inertia of servo motors?
- 【Share】Output adjustable
- [N32L43x Review] 6. Software and Hardware I2C Driver for 0.96-inch OLED
- GD32E231 DIY Competition (2) - Unboxing and Testing
- 【Case Study】Application of Wireless Wake-up Technology in Irrigation System
- 【Share】Parallel power supply
- [MM32 eMiniBoard Review] Give feedback on this board
- IWR6843 Smart mmWave Sensor Antenna-Package Evaluation Module
- Three designs of constant current circuit
- How can an outdoor Bluetooth speaker output the same power as a 12V lead-acid battery using two 7.4V lithium batteries?
- 【GD32F307E-START】+I2C driver problem