There is no need to explain much about the role of network communication. The work carried out this time is to initialize the hardware parts needed in the Ethernet communication process, and also introduce the methods of sending and receiving data.
Due to its complexity, the ASF framework is used, but the implementation of the library functions used will also be introduced.
1. MAC, PHY and MII
IEEE 802.3 is a commonly used Ethernet standard, which defines the standards of the physical layer (Physical Layer, PHY) and the media access control layer (Media Access Control, MAC). In addition, in the OSI model, MAC is at the bottom of the data link layer.
In terms of hardware implementation, the GMAC peripheral used by M4 implements the MAC function in 802.3. The development board carries a PHY chip model KSZ8051MNL and an RJ45 interface to implement the physical layer functions:
The interface between MAC and PHY is the Media Independent Interface (MII). MII includes a data communication interface and a management interface (MDIO). Since the PHY interface is oriented to MAC, we need to manage PHY and exchange data through MAC.
In addition, the Ethernet II frame developed earlier is the frame format commonly used in Ethernet transmission.
2. GMAC DMA Buffer
The GMAC uses a DMA interface. Like the M4's general purpose DMAC, it can also automatically perform multiple transfers, but the method is slightly different. The GMAC's DMA uses different buffer lists for transmit and receive, and the buffer descriptor list is an array, not a linked list like the DMAC uses. The starting position of the array is stored in registers (GMAC_RBQB, GMAC_TBQB), and there is a field in the buffer descriptor (Wrap) to indicate whether it is the last descriptor in the array. For example, for the receive buffer:
During operation, DMA accesses each buffer descriptor sequentially, and when the last descriptor is accessed, it restarts the traversal.
For the receive buffer, the length of each buffer in the list is the same, which is specified by the DRBS field in the DMA configuration register (GMAC_DCFGR). When the DMA writes data to the receive buffer, it also sets the corresponding fields of the descriptor to indicate the start and end of each frame; at the same time, it also marks relevant information, such as whether it is a broadcast frame, etc.
For the send buffer, the frame length, whether to add CRC and other control information are also indicated in the descriptor. After the data is prepared, the send operation can be triggered by writing the TSTART field to the GMAC_NCR register.
3. Initialize GMAC using ASF
Since PHY is accessed through MAC, GMAC settings must be completed before setting PHY.
GMAC has about 94 registers, of which about 40 are statistical registers, about 15 registers are related to 1588 and PTP, and about 15 registers are related to special addresses and IDs. In addition, in some status registers, you need to write 1 to a specific bit to clear the status of the bit.
The ASF module used is Ethernet GMAC, and then parameters such as MAC address, IP address, subnet mask, gateway and buffer size can be set in conf_eth.h.
Then call the gmac_dev_init() function to initialize GMAC:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | pmc_enable_periph_clk(ID_GMAC); //MAC address uint8_t mac_address[] = { ETHERNET_CONF_ETHADDR0, ETHERNET_CONF_ETHADDR1, ETHERNET_CONF_ETHADDR2,ETHERNET_CONF_ETHADDR3, ETHERNET_CONF_ETHADDR4, ETHERNET_CONF_ETHADDR5 }; // GMAC options gmac_options_t gmac_option; gmac_option.uc_copy_all_frame = 0; // Do not copy all frames gmac_option.uc_no_boardcast = 0; // Do not ignore broadcast memcpy(gmac_option.uc_mac_addr, mac_address, sizeof(mac_address)); //Copy MAC address // GMAC driver settings gmac_device_t gmac_dev; gs_gmac_dev.p_hw = GMAC; //Specify the GMAC register base address // Initialize GMAC gmac_dev_init(GMAC, &gmac_dev, &gmac_option); |
The gmac_dev_init(Gmac* p_gmac, gmac_device_t* p_gmac_dev, gmac_options_t* p_opt) function completes the following tasks:
Disable transmission and reception, disable all GMAC interrupts; clear the statistics registers and the transmission and reception status registers.
Set the GMAC_NCFGR register. According to p_opt, determine whether to copy all frames and whether to ignore broadcasts. At the same time, add GMAC_NCFGR_PEN and GMAC_NCFGR_IRXFCS bits. (I think this should be a BUG. Judging from the comments, the GMAC_NCFGR_RFCS bit should be added.)
After setting up the DMA buffer, call gmac_init_mem() to initialize the buffer descriptor, etc. This function also enables sending and receiving, and also enables a series of interrupts. After the setup is complete, the DMA buffer information will be stored in p_gmac_dev.
Write the MAC address into special address register 1.
4. PHY Address
During MDIO communication, each PHY will have a 4-bit address. The KSZ8051MNL chip carried by the development board can set the lower 3 bits of the address according to the pin when powered on or reset:
In the development board, the value of PHYAD[2:0] is 001 when powered on, that is, its address is 0x1.
In particular, address 0 can be used as the broadcast address of the chip, and the development board has also been configured in this way. In addition, in ASF, the address of PHY is incorrectly defined as 0. The reason why this works correctly is only because 0 is the broadcast address, and the development board has only one PHY chip. For the sake of rigor, modify this address to the correct value:
1 2 3 4 | #ifdef BOARD_GMAC_PHY_ADDR #undef BOARD_GMAC_PHY_ADDR #endif #define BOARD_GMAC_PHY_ADDR 1 |
5. Using PHY in ASF
The module used is Ethernet Physical Transceiver. The macro needs to be declared in conf_board.h:
1 2 | /* Using ETH PHY: KSZ8051MNL */ #define CONF_BOARD_KSZ8051MNL |
initialization.
After the PHY is powered on, you need to wait for a while for it to stabilize. Then you can initialize it:
12345if (ethernet_phy_init(GMAC, BOARD_GMAC_PHY_ADDR,sysclk_get_cpu_hz())!= GMAC_OK) {puts("PHY Initialize ERROR!\r");return -1;}In the ethernet_phy_init() function, the following work is done:
Set the MDIO clock MDC.
Send a reset command to the PHY via MDIO.
Check whether the address is correct. The logic of the check is to first read the content of PHYID1 of PHY, and then determine whether the read content is correct. In the KSZ8051MNL chip, the value of this register is 0x22.
If the address is invalid, because there are only 32 valid MDIO addresses, these addresses are iterated, and then the reset command is resent using the new address checked.
If initialization is successful, GMAC_OK is returned.
Self-negotiation.
Then you need to let PHY negotiate the communication rate and duplex mode:
123456ethernet_phy_auto_negotiate(GMAC, BOARD_GMAC_PHY_ADDR);if (ethernet_phy_set_link(GMAC, BOARD_GMAC_PHY_ADDR, 0)!= GMAC_OK) {puts("Set link ERROR!\r");return -1;}The ethernet_phy_auto_negotiate() function completes the PHY negotiation and sets the GMAC rate and duplex mode based on the negotiation result. The ethernet_phy_set_link() function checks the link status and applies the PHY auto-negotiation result to the GMAC based on the parameter (the third one).
Interrupt handling.
The GMAC module of ASF needs to obtain relevant interrupts to perform related work: such as updating information related to the send buffer descriptor, or calling user-defined callback functions.
12345// Need to enable related interrupts in NVICvoid GMAC_Handler(void){gmac_handler(&gs_gmac_dev);}Data reception.
Prepare a buffer first, then call gmac_dev_read() to read the contents of the received frame.
12345//#define GMAC_FRAME_LENTGH_MAX 1536uint8_t eth_buffer[GMAC_FRAME_LENTGH_MAX];uint32_t frm_size;gmac_dev_read(&gmac_dev, (uint8_t *) eth_buffer,sizeof(eth_buffer), &frm_size);Data is sent.
1gmac_dev_write(&gmac_dev, (uint8_t *)eth_buffer, frm_size, NULL);This function can be used to send data using GMAC. The fourth parameter is the callback function after the sending is completed. The callback function is called in gmac_handler().
Previous article:SAM4E MCU Tour - 21. DMAC USART Echo
Next article:SAM4E MCU Tour - 23. Using FPU in AS6 (GCC)
Recommended ReadingLatest update time:2024-11-23 06:01
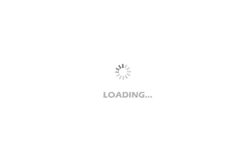
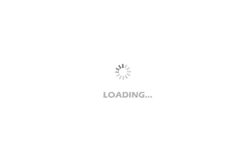
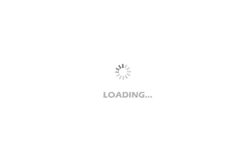
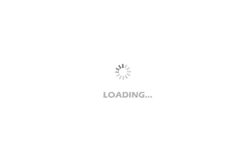
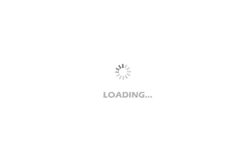
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Are 2G and 3G going to be phased out? Why is 3G being phased out faster than 2G?
- A strange problem occurred in a piece of code I wrote.
- Image Plane of PCB (I) Definition of Image Plane
- TMS320C6000 Basics
- Multimeter to measure transistor
- Lichee RV 86 PANEL Review (3)——WSL single system solution environment construction
- Some problems encountered when using RSL10 and their solutions
- Isolated power supply topology (transformer introduced Buck and Boot topology)
- Driver problem, please help!
- [RVB2601 Creative Application Development] Online Music Playback