Recently I have been learning and writing programs for single-chip microcomputers. Today I had some free time and wrote a program for communicating with DS18B20 based on a single bus by imitating the DS18B20 temperature measurement and display experiment.
DS18B20 digital temperature sensor (reference: Principle and application of intelligent temperature sensor DS18B20) is a 1-Wire, or single bus device, produced by DALLAS. It has the characteristics of simple circuit and small size. Therefore, it can be used to form a temperature measurement system with simple circuit. Many such digital thermometers can be connected on one communication line. Features of DS18B20 products:
(1) Only one I/O port is required to achieve communication.
(2) Each device in DS18B20 has a unique serial number.
(3) In actual application, no external components are required to achieve temperature measurement. (4
) The measurement temperature range is between -55 and +125℃; the error is ±5℃ in the range of -10 ~ +85℃;
(5) The resolution of the digital thermometer can be selected from 9 bits to 12 bits. The time required to convert a 12-bit temperature value into a digital value is no more than 750ms;
(6) There are internal temperature upper and lower limit alarm settings.
DS18B20 pinout diagram
DS18B20 detailed pin function description:
1. GND ground signal;
2. DQ data input and output pin. Open drain single bus interface pin. When used under parasitic power supply, this pin can provide power to the device; drain open, often too high level. Usually requires an external pull-up resistor of about 5kΩ.
3. VDD optional VDD pin. Voltage range: 3~5.5V; When working under parasitic power supply, this pin must be grounded.
DS18B20 memory structure diagram
The first two bytes of the temporary memory are the low and high bytes of the measured temperature information;
the 3rd and 4th bytes are volatile copies of TH and TL, which will be refreshed at each power reset;
the 5th byte is a volatile copy of the configuration register, which is also refreshed at power reset;
the 9th byte is the CRC check value of the previous 8 bytes.
The command content of the configuration register is as follows:
0 | R1 | R0 | 1 | 1 | 1 | 1 | 1 |
MSB LSB
R0 and R1 are the temperature value resolution bits, which are configured according to the following table. The default factory setting is R1R0 = 11, i.e. 12 bits.
Temperature value resolution configuration table
R1 | R0 | Resolution | Maximum conversion time (ms) |
0 | 0 | 9bit | 93.75(tconv/8) |
0 | 1 | 10bit | 183.50(tconv/4) |
1 | 0 | 11bit | 375(tconv/2) |
1 | 1 | 12bit | 750 (tconv) |
The temperature resolutions corresponding to the four resolutions are 0.5℃, 0.25℃, 0.125℃, 0.0625℃ (i.e. the temperature value represented by the lowest bit).
The specific format of the two temperature bytes at 12-bit resolution is as follows:
Low byte:
2^3 | 2^2 | 2^1 | 2^0 | 2^-1 | 2^-2 | 2^-3 | 2^-4 |
High Byte:
S | S | S | S | S | 2^6 | 2^5 | 2^4 |
The first 5 bits of the high byte are the sign bit S. If the resolution is lower than 12 bits, the lowest bit is set to 0. For example, when the resolution is 10 bits, the low byte is:
2^3 | 2^2 | 2^1 | 2^0 | 2^-1 | 2^-2 | 0 | 0 |
, the high byte remains unchanged....
Some temperatures and converted output numbers are as follows:
temperature | Digital Output | Convert to hexadecimal |
+125℃ | 00000111 11010000 | 07D0H |
+85℃ | 00000101 01010000 | 0550H |
+25.0625℃ | 00000001 10010001 | 0191H |
+10.125℃ | 00000000 10100010 | 00A2H |
+0.5℃ | 00000000 00001000 | 0008H |
0℃ | 00000000 00000000 | 0000H |
-0.5℃ | 11111111 11111000 | FFF8H |
-10.125℃ | 11111111 01011110 | FFE5H |
-25.0625℃ | 11111110 01101111 | FF6FH |
-55℃ | 11111100 10010000 | FC90H |
As can be seen from the table above, when the output is negative temperature, the complement code is used to facilitate computer calculations (if using C language, the result can be directly assigned to an int variable).
How to use DS18B20:
Since DS18B20 uses the 1-Wire bus protocol, that is, bidirectional data transmission is achieved on a data line, for the microcontroller, we must use software methods to simulate the protocol timing of the single bus to complete the access to the DS18B20 chip.
Since DS18B20 reads and writes data on an I/O line, there are strict timing requirements for the data bits read and written.
DS18B20 has a strict communication protocol to ensure the correctness and integrity of each bit of data transmission.
The protocol defines the timing of several signals: initialization timing (implemented by dsInit()), read timing (readByte()), and write timing (writeByte()).
All timings use the host as the master device and the single bus device as the slave device. Each command and data transmission starts with the host actively starting the write sequence. If the single bus device is required to send back data, the host needs to start the read sequence to complete data reception after issuing the write command. The transmission of data and commands is low-order first.
DS18B20 and MCU connection circuit diagram:
Use software to simulate the single-line protocol and commands of DS18B20: The host must follow the following sequence to operate DS18B20
1. Initialization
All operations on the single-line bus start with initialization. The process is as follows:
1) Request: The host generates a reset pulse by pulling down the single line for more than 480us, and then releases the line to enter the Rx receiving mode. When the host releases the bus, a rising edge pulse is generated.
DQ: 1 -> 0(480us+) -> 1
2) Response: After DS18B20 detects the rising edge, it delays 15~60us and generates a response pulse by pulling down the bus for 60~240us.
DQ: 1(15~60us) -> 0(60~240us)
3) Receive response: After the host receives the response pulse from the slave, it means that there is a single-line device online. At this point, initialization is completed.
DQ: 0
2. ROM operation command
When the host detects the response pulse, it can initiate a ROM operation command. There are 5 types of ROM operation commands, as shown in the following table
Command Type | Command Byte | Function |
Read Rom | 33H | Reading 64 bits in the laser ROM can only be used for a single DS18B20 device on the bus. Data conflicts will occur when multiple devices are connected |
Match Rom | 55H | This command is followed by a 64-bit ROM serial number, addressing the corresponding DS18B20 on the multi-hang bus. Only the DS18B20 with a completely matching serial number can respond to the following memory operation command, and the others that do not match will wait for the reset pulse. It can be used for single hang or multiple hang situations. |
Skip Rom | CCH | It can run memory operation commands without providing the 64-bit ROM serial number. It can only be used for single hanging. |
Search Rom | F0H | Through a process of elimination, identify the ROM serial numbers of all devices on the bus |
Alarm Search | ECH | The command flow is the same as Search Rom, but DS18B20 will respond to this command only if the most recent temperature measurement meets the alarm trigger condition. |
3. Memory operation commands
can only be used after the ROM operation command is successfully executed. There are 6 memory operation commands:
Command Type | Command Byte | Function |
| 4EH | Write the 3 bytes at address 2 to address 4 in the scratchpad (TH, TL and configuration register) before initiating the reset pulse. All 3 bytes must be written. |
| BEH | Read the contents of the register, from byte 0 to byte 8, a total of 9 bytes. The host can initiate a reset pulse at any time to stop this operation. Usually we only need to read the first 5 bytes. |
Copy | 48H | Copies the contents of the scratchpad into EERAM so that the temperature alarm trigger byte is stored in non-volatile memory. If the host generates a read time slot after this command, the device will output 0 as long as the copy is in progress and 1 after the copy is completed. |
| 44H | Start temperature conversion operation. If the host generates a time slot after this command, the device will output 0 as long as it is still converting temperature, and 1 after the conversion is completed. |
Recall E2 | B8H | The temperature alarm trigger value and configuration register value stored in EERAM are copied back to the temporary register. This operation is automatically generated when the DS18B20 is powered on. |
Read Power | B4H | After the host initiates this command, in each reading time slot, DS18B20 will send a signal to notify its power supply mode: 0 parasitic power supply, 1 external power supply. |
4. Data processing
DS18B20 requires strict timing to ensure data integrity. On the single-line DQ, there are six signal types: reset pulse, response pulse, write 0, write 1, read 0, and read 1. Except for the response pulse, the others are generated by the host. The reading and writing of data bits are realized through read and write time slots.
1) Write time slot: When the host pulls the data line from high level to low level, a write time slot is generated. All write time slots must be more than 60us, and a recovery time of 1us must be guaranteed between each write time slot.
Write "1": The host pulls the data line DQ low first, then releases it for 15us, and then pulls the data line DQ high;
write "0": The host pulls DQ low and keeps it for at least 60us.
2) Read time slot: When the host pulls the data line DQ from high level to low level, a read time slot is generated. All read time slots must last for at least 60us, A 1us recovery time must be guaranteed between each read time slot.
Read: The host pulls DQ down for at least 1us. At this time, the host immediately pulls DQ high, and then you can delay 15us and read DQ.
Source code: (measurement range: 0 ~ 99 degrees)
DS18B20
1 #include
2 //Test the current ambient temperature through DS18B20, and display the current temperature through the digital tube
3 sbit wela = P2^7; //Digital tube bit selection
4 sbit dula = P2^6; //Digital tube segment selection
5 sbit ds = P2^2;
6 //0-F digital tube coding (common cathode)
7 unsigned char code table[]={0x3f,0x06,0x5b,0x4f,0x66,
8 0x6d,0x7d,0x07,0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71};
9 //0-9 digital tube coding (common cathode), with decimal point
10 unsigned char code tableWidthDot[]={0xbf, 0x86, 0xdb, 0xcf, 0xe6, 0xed, 0xfd,
11 0x87, 0xff, 0xef};
12
13 //Delay function, for example, i=10, the delay is about 10ms.
14 void delay(unsigned char i)
15 {
16 unsigned char j, k;
17 for(j = i; j > 0; j--)
18 {
19 for(k = 125; k > 0; k--);
20 }
21 }
22
23 //Initialize DS18B20
24 //Let DS18B20 be low level for a relatively long time, and then high level for a relatively short time, then it can be started
25 void dsInit()
26 {
27 //Be sure to use unsigned int type, the time of an i++ instruction, As a small time interval for communicating with DS18B2028
// The following are all using unsigned int type29
unsigned int i;
30 ds = 0;
31 i = 103;
32 while(i>0) i--;
33 ds = 1;
34 i = 4;
35 while(i>0) i--;
36 }
37
38 //Read one bit of data from DS18B2039 //Read one bit, let DS18B20 be low level for a small cycle, then high level for two small cycles, 40 //Then DS18B20 will output one bit of data for a period of time41 bit readBit() 42 { 43 unsigned int i; 44 bit b; 45 ds = 0; 46 i++; 47 ds = 1; 48 i++; i++; 49 b = ds; 50 i = 8; 51 while(i>0) i--; 52 return b; 53 } 54 55 //Read one byte of data, implemented by calling readBit() 56 unsigned char readByte() 57 { 58 unsigned int i; 59 unsigned char j, dat; 60 dat = 0; 61 for(i=0; i<8; i++) 62 { 63 j = readBit(); 64 //The lowest bit is read first
65 dat = (j << 7) | (dat >> 1);
66 }
67 return dat;
68 }
69
70 //Write one byte of data to DS18B20
71 void writeByte(unsigned char dat)
72 {
73 unsigned int i;
74 unsigned char j;
75 bit b;
76 for(j = 0; j < 8; j++)
77 {
78 b = dat & 0x01;
79 dat >>= 1;
80 //Write "1", let the low level last for 2 small delays, and the high level last for 8 small delays
81 if(b)
82 {
83 ds = 0;
84 i++; i++;
85 ds = 1;
86 i = 8; while(i>0) i--;
87 }
88 else //Write "0", let the low level last for 8 small delays,
and the high level last for 2 small delays89 {
90 ds = 0;
91 i = 8; while(i>0) i--;
92 ds = 1;
93 i++; i++;
94 }
95 }
96 }
97
98 //Send temperature conversion command to DS18B2099
void sendChangeCmd()
100 {
101 dsInit(); //Initialize DS18B20
102 delay(1); //Delay 1ms
103 writeByte(0xcc); //Write skip serial number command word
104 writeByte(0x44); //Write temperature conversion command word
105 }
106
107 //Send read data command to DS18B20108
void sendReadCmd()
109 {
110 dsInit();
111 delay(1);
112 writeByte(0xcc); //Write the skip sequence number command
word113 writeByte(0xbe); //Write the read data command
word114 }
115
116 //Get the current temperature value117
unsigned int getTmpValue()
118 {
119 unsigned int value; //Store the temperature value120
float t;
121 unsigned char low, high;
122 sendReadCmd();
123 //Continuously read two bytes of data124
low = readByte();
125 high = readByte();
126 //Combining the high and low bytes into an integer variable127
value = high;
128 value <<= 8;
129 value |= low;
130 //The accuracy of DS18B20 is 0.0625 degrees, that is, the lowest bit of the read data represents 0.0625 degrees
131 t = value * 0.0625;
132 //Magnify it 10 times so that it can display one decimal place, and round the second decimal place
133 //For example, if t=11.0625, after counting, we get value = 111, that is, 11.1 degrees
134 value = t * 10 + 0.5;
135 return value;
136 }
137
138 //Display the current temperature value, accurate to one decimal place
139 void display(unsigned int v)
140 {
141 unsigned char count;
142 unsigned char datas[] = {0, 0, 0};
143 datas[0] = v / 100;
144 datas[1] = v % 100 / 10;
145 datas[2] = v % 10;
146 for(count = 0; count < 3; count++)
147 {
148 //Chip select
149 wela = 0;
150 P0 = ((0xfe << count) | (0xfe >> (8 - count))); //Select the (count + 1)th digital tube
151 wela = 1; //Open the latch and give it a falling edge
152 wela = 0;
153 //Segment select
154 dula = 0;
155 if(count != 1)
156 {
157 P0 = table[datas[count]]; //Display numbers
158 }
159 else
160 {
161 P0 = tableWidthDot[datas[count]]; //Display numbers with decimal point
162 }
163 dula = 1; //Open the latch and give it a falling edge
164 dula = 0;
165 delay(5); //Delay 5ms, that is, light up for 5ms
166
167 //Clear the segment first, turn off the digital tube, remove the impact on the next bit, and remove the high bit on the low bit ghost
168 //If you want to know the effect, you can remove this code by yourself
169 //Because the digital tube is common cathode, all the codes for turning off are: 00H
170 dula = 0;
171 P0 = 0x00; //Display numbers
172 dula = 1; //Open the latch and give it a falling edge
173 dula = 0;
174 }
175 }
176
177 void main()
178 {
179 unsigned char i;
180 unsigned int value;
181 while(1)
182 {
183 //Start temperature conversion
184 sendChangeCmd();
185 value = getTmpValue();
186 //Display 3 times
187 for(i = 0; i < 3; i++)
188 {
189 display(value);
190 }
191 }
192 }
Display effect:
Flowchart:
Improve code: Expand the measurement range to -55 degrees to +125 degrees, and strictly follow the above process to design the software
3.15 1:34 Correct the influence of the next digit display on the previous digit in the display() function
Improved code
1 #include
2 #include
3 #include
4 //Test the current ambient temperature through DS18B20, and display the current temperature value through the digital tube. The current display range is: -55~ +125 degrees
5 sbit wela = P2^7; //Digital tube bit selection
6 sbit dula = P2^6; //Digital tube segment selection
7 sbit ds = P2^2;
8 int tempValue;
9
10 //0-F digital tube encoding (common cathode)
11 unsigned char code table[]={0x3f,0x06,0x5b,0x4f,0x66,
12 0x6d,0x7d,0x07,0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71};
13 //0-9 digital tube coding (common cathode), with decimal point
14 unsigned char code tableWidthDot[]={0xbf, 0x86, 0xdb, 0xcf, 0xe6, 0xed, 0xfd,
15 0x87, 0xff, 0xef};
16
17 //Delay function, for 11.0592MHz clock, for example i=10, the delay is about 10ms.
18 void delay(unsigned int i)
19 {
20 unsigned int j;
21 while(i--)
22 {
23 for(j = 0; j < 125; j++);
24 }
25 }
26
27 //Initialize DS18B20
28 //Let DS18B20 be low level for a relatively long time, and then high level for a relatively short time, to start
29 void dsInit()
30 {
31 //For 11.0592MHz clock, unsigned int type i, the time for an i++ operation is greater than 8us
32 unsigned int i;
33 ds = 0;
34 i = 100; //Pull down for about 800us, which meets the protocol requirement of more than 480us
35 while(i>0) i--;
36 ds = 1; //Generate a rising edge and enter the waiting response state
37 i = 4;
38 while(i>0) i--;
39 }
40
41 void dsWait()
42 {
43 unsigned int i;
44 while(ds);
45 while(~ds); //Detect response pulse
46 i = 4;
47 while(i > 0) i--;
48 }
49
50 //Read one bit of data from DS18B20
51 //Read one bit, let DS18B20 be low level for a small period, then high level for two small periods,
52 //Then DS18B20 will output one bit of data for a period of time
53 bit readBit()
54 {
55 unsigned int i;
56 bit b;
57 ds = 0;
58 i++; //Delay about 8us, at least 1us in accordance with the protocol requirement
59 ds = 1;
60 i++; i++; //Delay about 16us, at least 15us in accordance with the protocol requirement
61 b = ds;
62 i = 8;
73 for(i=0; i<8; i++) 74 { 75 j = readBit () ; 76 //The first data to be read is the lowest bit
77 dat = (j << 7 ) | (dat >> 1 ); 78 } 79 return dat ; 80 } 81 82 // Write a byte of data to DS18B20 83 void writeByte( unsigned char dat) 84 { 85 unsigned int i; 86 unsigned char j; 87 bit b; 88 for(j = 0; j < 8; j++) 89 { 90 b = dat & 0x01; 91 dat >>= 1; 92 //Write "1", pull DQ low for 15us, then pull DQ high within 15us~60us to complete writing 1 93 if(b) 94 { 95 ds = 0; 96 i++; i++; //Pull down for about 16us, the sign requires 15~60us 97 ds = 1; 98 i = 8; while(i>0) i--; //Delay about 64us, in line with the requirement that the write time slot is not less than 60us 99 } 100 else //Write "0", pull DQ low for 60us~120us 101 { 102 ds = 0; 103 i = 8; while(i>0) 117 writeByte ( 0x44 ) ; // Write temperature conversion command word Convert T 118 } 119 120 //Send a read data command to DS18B20 121 void sendReadCmd() 122 { 123 dsInit(); 124 dsWait(); 125 delay(1);
126 writeByte(0xcc); //Write Skip Rom command word
127 writeByte(0xbe); //Write Read Scratchpad command word
128 }
129
130 //Get current temperature value
131 int getTmpValue()
132 {
133 unsigned int tmpvalue;
134 int value; //Store temperature value
135 float t;
136 unsigned char low, high;
137 sendReadCmd();
138 //Read two bytes of data continuously
139 low = readByte();
140 high = readByte();
141 //Combining the high and low bytes into an integer variable
142 //Negative numbers are represented by two's complement in the computer
143 //If it is a negative value, the value read out is represented by two's complement and can be directly assigned to int value
144 tmpvalue = high;
145 tmpvalue <<= 8;
146 tmpvalue |= low;
147 value = tmpvalue;
148
149 //Use the default resolution of DS18B20, 12 bits, with an accuracy of 0.0625 degrees, i.e. the lowest bit of the read-back data represents 0.0625 degrees
150 t = value * 0.0625;
151 //Enlarge it 100 times so that it can display two decimal places, and round the third decimal place
152 //For example, if t=11.0625, after counting, we get value = 1106, i.e. 11.06 degrees
153 //For example, if t=-11.0625, after counting, we get value = -1106, i.e. -11.06 degrees
154 value = t * 100 + (value > 0 ? 0.5 : -0.5); //If it is greater than 0, add 0.5; if it is less than 0, subtract 0.5
155 return value;
156 }
157
158 unsigned char const timeCount = 3; //Time interval of dynamic scanning
159 //Display the current temperature value, accurate to one decimal place
160 //If you select the bit first and then the segment, since the IO port outputs a high level by default, the digital tube will display garbled characters when the bit is selected first
161 void display(int v)
162 {
163 unsigned char count;
164 unsigned char datas[] = {0, 0, 0, 0, 0};
165 unsigned int tmp = abs(v);
166 datas[0] = tmp / 10000;
167 datas[1] = tmp % 10000 / 1000;
168 datas[2] = tmp % 1000 / 100;
169 datas[3] = tmp % 100 / 10;
170 datas[4] = tmp % 10;
171 if(v < 0)
172 {
173 //Turn off the bit selection to remove the influence on the previous bit
174 P0 = 0xff;
175 wela = 1; //Turn on the latch and give it a falling edge
176 wela = 0;
177 //Segment selection
178 P0 = 0x40; //Display "-"
179 dula = 1; //Turn on the latch and give it a falling edge
180 dula = 0;
181
182 //Bit selection
183 P0 = 0xfe;
184 wela = 1; //Open the latch and give it a falling edge
185 wela = 0;
186 delay(timeCount);
187 }
188 for(count = 0; count != 5; count++)
189 {
190 //Turn off the bit selection to remove the influence on the previous bit
191 P0 = 0xff;
192 wela = 1; //Open the latch and give it a falling edge
193 wela = 0;
194 //Segment selection
195 if(count != 2)
196 {
197 /* if((count == 0 && datas[count] == 0)
198 || ((count == 1 && datas[count] == 0) && (count == 0 && datas[count - 1] == 0)))
199 {
200 P0 = 0x00; //When the highest bit is 0, no display
201 }
202 else*/
203 P0 = table[datas[count]]; //Display numbers
204 }
205 else
206 {
207 P0 = tableWidthDot[datas[count]]; //Display numbers with decimal points
208 }
209 dula = 1; //Open the latch and give it a falling edge
210 dula = 0;
211
212 //Bit selection
213 P0 = _crol_(0xfd, count); //Select the (count + 1)th digital tube
214 wela = 1; //Open the latch and give it a falling edge
215 wela = 0;
216 delay(timeCount);
217 }
218 }
219
220 void main()
221 {
222 unsigned char i;
223
224 while(1)
225 {
226 //Start temperature conversion
227 sendChangeCmd();
228 //Display 5 times
229 for(i = 0; i < 40; i++)
230 {
231 display(tempValue);
232 }
233 tempValue = getTmpValue();
234 }
235 }
The improved effect diagram:
there is only one decimal place
and two decimal places, and the influence of the next place on the previous place is eliminated
(PS: During the writing of this article, the late winter of 2007 passed, and the temperature rose by 5℃...warm^_^)
Other references:
1. "51 Single-Chip Microcomputer C Language Application Design Example Detailed Lecture", edited by Dai Jia and Dai Weiheng, published by Electronics Industry Press.
Previous article:Microcontroller Exercise - DA Conversion
Next article:Microcontroller Exercise - Timer
Recommended ReadingLatest update time:2024-11-16 16:23
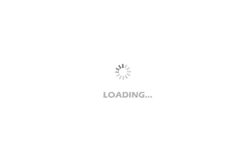
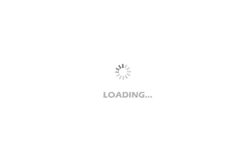
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Working Principle and Topology of Class D Amplifier
- [Me and Yatli] + From nothing to something, from something to big, I hope to develop better!
- EEWORLD University Hall----mmWave sensors improve artificial intelligence algorithms to use elevators more efficiently
- Nuvoton Solution Sharing: IoT Development Based on NUC472 Development Board and Connected to Gizwits
- [Project Source Code] Altera SOPC FrameBuffer System Design Tutorial
- What is FRAM?
- Can 1N4007 be used to isolate 220V mains?
- W5500 TCP Client Network Debug Assistant cannot connect
- Analog circuits are much more difficult to learn than digital circuits. China’s real weak spot is actually analog chips.
- DIY a server for 5 dollars?