/*This temperature sensor
is written by myself, the timing has been corrected, and the self-test delay time
uses the Tianxiang temperature conversion module algorithm
*/
#include __CONFIG(0x3B31); #define uint unsigned int #define uchar unsigned char #define DQ RC1 #define DQ_DIR TRISC1 #define DQ_HIGH() TRISC1=1 #define DQ_LOW() DQ=0;TRISC1=0 unsigned char shi; // tens digit unsigned char ge; // integer digit unsigned char shifen; //tenths place unsigned char baifen; //percentile unsigned char qianfen; //thousandths unsigned char wanfen; //ten thousandth place const uchar table[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f}; //Display array 0 void delayus(char x,char y) //us level delay provided by Huijing { char z; //define Z do { //Execute do once first z=y; //Give the value of Y to Z do{;}while(--z); //do empty statement, wait for --z, until z=0 ends, do--while statement, delay occurs here } while (--x); //Similarly, x is in--, and a delay is generated again } void delay(uint x) { uint a,b; for(a=x;a>0;a--) for(b=110;b>0;b--); } void display(char shi,char ge,char shifen,char baifen,char qianfen) { PORTD=table[shi]; PORTA=0x02; delay(2); PORTD=table[ge]|0x80; //or 0x80 to display the decimal point PORTA=0x04; delay(2); PORTD=table[shifen]; PORTA=0x06; delay(2); PORTD=table[baifen]; PORTA=0x08; delay(2); PORTD=table[qianfen]; PORTA=0x0a; delay(2); } void init(void) { TRISA=0;PORTA=0x00; TRISC=0xf0;PORTC=1; TRISD=0; } void reset(void) { char pe=1; while(pe) { DQ_LOW(); delayus(2,81); //delay 502us DQ_HIGH(); delayus(4,4); //delay 71us if(DQ==1)pe=1; //Judge whether to respond (pull to low level when responding), set to 1 and resend in a loop if no response else pe=0; //Otherwise it is a response, set to 0 to exit the loop delayus(2,81); //delay 502us } } void write_byte(char val) { uchar i,temp; for(i=8;i>0;i--) //Loop 8 times to form a byte { temp=val&0x01; //Take out the lowest bit, and take out 1 DQ_LOW(); delayus(1,1); //delay 15us if(temp==1)DQ_HIGH(); //If the value taken out is 1, pull it to high level and send it out delayus(3,3); //Delay 45us, if the taken out is 0, also send it DQ_HIGH(); //Pull up to high level NOP();NOP(); //Delay 2us val=val>>1; //Move right once for next extraction } } uchar read_byte(void) { uchar i,val=0; static bit j; //static bit variable, a status bit, cannot be a byte for(i=8;i>0;i--) { val=val>>1; //Move one position first DQ_LOW(); //Pull to low level NOP();NOP();NOP();NOP();NOP();NOP(); //Delay 6us DQ_HIGH(); NOP();NOP();NOP();NOP(); //Delay 4us j=DQ; //Read the state of the data line to get a state bit for data processing //So we need to define static bit j; if(j==1)val=val|0x80; //Data processing: If the value read is 1, put it in the highest bit first, and then shift it back one by one to form a byte delayus(1,6); //Delay 30us to repeat the above steps } return(val); //Construct 1 byte and return } void get_temp(void) //01:40:26 //Get temperature, device matching (multiple temperature sensors) { uchar TLV,THV,num; //tem1/tem2; there are also 2 bytes of temperature instructions float aaa; uint temper; reset(); //Reset write_byte(0xCC); //skip ROM write_byte(0x44); //Temperature conversion, delay required for(num=100;num>0;num--) //Originally, delay(1000) was used to delay the time for 1 second. But the effect disappears after a short shake. display(shi,ge,shifen,baifen,qianfen); //So use the display to replace the delay, display 100 times is about 750ms or more reset(); //Before each RAM operation, reset 18B20 and match write_byte(0xCC); //skip ROM write_byte(0xBE); //Tell it that I will read your temperature next, read the register TLV=read_byte(); //RAM has 9 bytes (we only need 2 bytes, LSB and MSB), it reads from the lowest bit THV=read_byte(); //01:45:10 + Ruizhi at 58:52 DQ_HIGH(); //Release the bus aaa=(THV*256+TLV)*0.0625*1000; //(16-bit temperature data)*0.0625 is the actual temperature (decimal number) temper=(int)aaa; //Because of the compile-time warning; there is a decimal point, it is a floating point to integer conversion; we use forced conversion to integer to get the value of the decimal point (the decimal point is not easy to get, use multiplication by 100 to get it) shi=temper/10000; //Distribute the tens to five digital tubes. It feels weird to display four digits, so use five digital tubes; at 1:51:00 ge=temper%10000/1000; //I want to use five digital tubes to display, so it is 10000 five-digit number shifen=temper%1000/100; // baifen=temper%100/10; // qianfen=temper%10; // } void main() { init(); while(1) { get_temp(); display(shi,ge,shifen,baifen,qianfen); //The compiler will not pass without formal parameters, and it will not pass with class parameters either } }
Previous article:PIC microcontroller development board independent keyboard scanning C language code
Next article:PIC microcontroller development board independent keyboard scanning + buzzer sound + digital tube C program code
Recommended ReadingLatest update time:2024-11-16 12:03
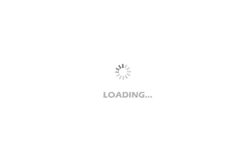
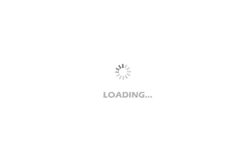
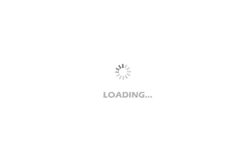
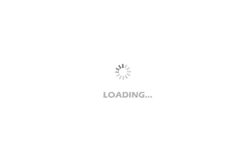
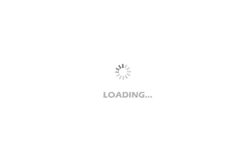
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Single-chip microcomputer technology and application - electronic circuit design, simulation and production (edited by Zhou Runjing)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Temperature coefficient of aluminum electrolysis
- TIOBE Index September 2022
- Android & BlueNRG2
- Chip selection for making LED clock products
- [Anxinke ESP32 Voice Development Board Special Topic ①] ESP32-A1S Audio Development Board Offline Voice Recognition Control LED Light
- Problems with two types of channel MOS tube circuits
- The resistor behind the rectifier bridge of this power supply acts as
- How to weld this Type-C socket to meet the requirements
- 【RVB2601 Creative Application Development】Environmental Monitoring Terminal 08-Work Submission
- Rethinking 5G