For the relevant special registers, please refer to the relevant data
program code as follows:
Timer
1 #include
2 // Make the LED light up for 1000ms, dark for 300ms, light up for 300ms, dark for 1000ms, light up for 1000ms
. Repeat this cycle
.
3 /*
4 Test the 4 working modes of the timer
5 The microcontroller clock is 11.0592MHz, and 1 machine cycle is 1.085us.
6 Calculate the initial value: Take timer 0 as an example, the calculated value is Nus
7 Mode 0: TH0 = (2^13 - (N/1.085)) / 2^5 = (8192 - N/1.085) / 32, TL0 = (8192 - N/1.085) % 32;
8 Mode 1: TH0 = (2^16 - (N/1.085)) / 2^8 = (65536 - N/1.085) / 256, TL0 = (65536 - N/1.085) % 256;
9 Mode 2: TH0 = TL0 = 2^8 - N/1.085 = 256 - N/1.085;
10 Mode 3: For timer 0, divide into 2 8-bit counters; For timer 1, stop counting
11 */
12
13 unsigned char t0Count, t1Count; //Store the number of timer interrupts
14 unsigned char t0H, t0L, t1H, t1L; //Store the initial value
15
16 //T0 mode 0, timing 5ms
17 void time0() interrupt 1
18 {
19 t0Count++;
20 }
21
22 //T1 mode 1, timing 50ms
23 void time1() interrupt 3
24 {
25 t1Count++;
26 }
27
28 void main()
29 {
30 t0Count = 0;
31 t1Count = 0;
32 t0H = (8192 - 5000 / 1.085) / 32;
33 t0L = (8192 - 5000 / 1.085);
34 t0L %= 32;
35 t1H = (65536 - 50000 / 1.085) / 256;
36 t1L = (65536 - 50000 / 1.085);
37 t1L %= 256;
38
39 TH0 = t0H; TL0 = t0L; // Initial value, timing 5ms
40 TH1 = t1H; TL1 = t1L; // Timing 50ms
41
42 EA = 1; //Enable interrupts
43 ET0 = 1; //Enable timer 0, timer 1 interrupts
44 ET1 = 1;
45 TMOD = 0x10; //T1 mode 1, T0 mode 0: TMOD = 0001 0000B
46 P1 = 0;
47 TR1 = 1; //Start timing T1, stop T0
48 TR0 = 0;
49 while(1)
50 {
51 if(t1Count==20) //Enough for 1000ms
52 {
53 if(!P1) //The diode is on, then change the frequency
54 {
55 TR1 = 0; //Start timing T0, stop T1
56 TR0 = 1;
57 }
58 TH1 = t1H; TL1 = t1L; //Time 50ms
59 t1Count = 0;
60 P1 = ~P1;
61 }
62 else if(t0Count==60) //Enough for 300ms
63 {
64 if(!P1) //The diode is on, then change the frequency
65 {
66 TR1 = 1; //Let T1 start timing, T0 stop
67 TR0 = 0;
68 }
69 TH0 = t0H; TL0 = t0L; //Timing 5ms
70 t0Count = 0;
71 P1 = ~P1;
72 }
73 }
74 }
Previous article:MCU Exercise - DS18B20 Temperature Conversion and Display
Next article:Microcontroller Exercise - Timer
Recommended ReadingLatest update time:2024-11-16 14:42
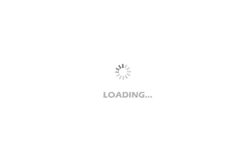
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Is there any MEMS or sensor expert?
- MSP430 Registers
- GPIO Features of TMS320C6748
- Simplifying isolated CAN, power interfaces for HEV 48-V systems
- Active and passive filters
- Can I use LPS22HB without connecting SD0/SA0 to anything?
- App Client
- Limitations of Flash_API for C2000 Series (28335) DSP
- EEWORLD University - Power stage protection, current sensing, efficiency analysis and related TI designs
- Amplifier