Q: What is the SysTick timer?
SysTick is a 24-bit countdown timer. When it counts to 0, it will automatically reload the initial value of the timer from the RELOAD register. As long as its enable bit in the SysTick control and status register is not cleared, it will never stop.
Q: Why set the SysTick timer?
(1) Generate the clock beat of the operating system.
The SysTick timer is tied to the NVIC and is used to generate the SYSTICK exception (exception number: 15). In the past, most operating systems required a hardware timer to generate the tick interrupt required by the operating system as the time base of the entire system. Therefore, a timer is needed to generate periodic interrupts, and it is best not to allow user programs to access its registers at will to maintain the rhythm of the operating system's "heartbeat".
(2) Facilitate program porting between different processors.
The Cortex-M3 processor contains a simple timer. Because all CM3 chips have this timer, the porting of software between different CM3 devices is simplified. The clock source of this timer can be the internal clock (FCLK, the free-running clock on the CM3) or the external clock (STCLK signal on the CM3 processor).
However, the specific source of STCLK is determined by the chip designer, so the clock frequency between different products may be very different. You need to check the device manual of the chip to decide what to choose as the clock source. The SysTick timer can generate an interrupt. CM3 has a special exception type for it and it has a place in the vector table. It makes it much easier to port the operating system and other system software between CM3 devices because it is handled the same in all CM3 products.
(3) As an alarm to measure time.
In addition to serving the operating system, the SysTick timer can also be used for other purposes: such as as an alarm, for measuring time, etc. It should be noted that when the processor is halted during debugging, the SysTick timer will also stop operating.
Q: How does Systick work?
First set the counter clock source, CTRL->CLKSOURCE (control register). Set the reload value (RELOAD register) and clear the count register VAL (CURRENT in the figure below). Set CTRL->ENABLE to start timing.
If it is an interrupt, allow Systick interrupts and handle them in the interrupt routine. If the query mode is used, read the COUNTFLAG flag of the control register continuously to determine whether the timing is zero. Or take one of the following methods.
When the SysTick timer counts from 1 to 0, it will set the COUNTFLAG bit; and the following method can clear it:
1. Read the SysTick Control and Status Register (STCSR)
2. Write any data to the SysTick Current Value Register (STCVR).
Only when the VAL value is 0, the counter automatically reloads RELOAD.
Q: How to use SysTicks as a system clock?
The biggest mission of SysTick is to generate exception requests regularly as the system's time base. The OS needs this "tick" to promote task and time management. To enable the SysTick exception, set STCSR.TICKINT. In addition, if the vector table is relocated to SRAM, it is also necessary to create a vector for the SysTick exception and provide the entry address of its service routine.
Q: How to use SysTick to complete a delay?
Query method reference: http://blog.ednchina.com/atom6037/188271/message.aspx
Interrupt method reference:
Put the initialization function SysTick_Configuration(void) outside the while() loop and execute it once:
view plaincopy to clipboardprint?
void SysTick_Configuration(void)
{
/* Select AHB clock(HCLK) as SysTick clock source Set AHB clock to SysTick clock*/
SysTick_CLKSourceConfig(SysTick_CLKSource_HCLK);
/* Set SysTick Priority to 3 Set SysTicks interrupt preemption priority 3, from priority 0*/
NVIC_SystemHandlerPriorityConfig(SystemHandler_SysTick, 3, 0);
/* SysTick interrupt each 1ms with HCLK equal to 72MHz SysTick interrupt occurs every 1ms*/
SysTick_SetReload(72000);
/* Enable the SysTick Interrupt */
SysTick_ITConfig(ENABLE);
}
void SysTick_Configuration(void)
{
/* Select AHB clock(HCLK) as SysTick clock source Set AHB clock as SysTick clock */
SysTick_CLKSourceConfig(SysTick_CLKSource_HCLK);
/* Set SysTick Priority to 3 Set SysTicks interrupt preemption priority 3, from priority 0 */
NVIC_SystemHandlerPriorityConfig(SystemHandler_SysTick, 3, 0);
/* SysTick interrupt each 1ms with HCLK equal to 72MHz
SysTick_SetReload(72000);
/* Enable the SysTick Interrupt */
SysTick_ITConfig(ENABLE);
}
Delay function, call when delay is needed:
view plaincopy to clipboardprint?
void Delay(u32 nTime)
{
/* Enable the SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Enable);
TimingDelay = nTime;
while(TimingDelay != 0)
; //Wait for the count to reach 0
/* Disable the SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Disable);
/* Clear the SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Clear);
}
void Delay(u32 nTime)
{
/* Enable the SysTick Counter */
SysTick_CounterCmd(SysTick_Counter_Enable);
TimingDelay = nTime;
while(TimingDelay != 0)
; //Wait for the count to reach 0
/* Disable the SysTick Counter Disable SysTick counter */
SysTick_CounterCmd(SysTick_Counter_Disable);
/* Clear the SysTick Counter Clear SysTick counter */
SysTick_CounterCmd(SysTick_Counter_Clear);
}
Interrupt function, called when the timer is reduced to zero, placed in the stm32f10x_it.c file
Previous article:STM32 learning assert_failed
Next article:STM32-External Interrupt Experiment
Recommended ReadingLatest update time:2024-11-15 13:52
![[STM32 motor vector control] Record 13——EXTI external interrupt](https://6.eewimg.cn/news/statics/images/loading.gif)
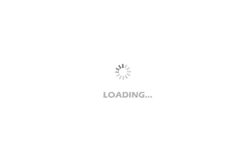
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Brief Analysis of Automotive Ethernet Test Content and Test Methods
- How haptic technology can enhance driving safety
- Let’s talk about the “Three Musketeers” of radar in autonomous driving
- Why software-defined vehicles transform cars from tools into living spaces
- How Lucid is overtaking Tesla with smaller motors
- Wi-Fi 8 specification is on the way: 2.4/5/6GHz triple-band operation
- Wi-Fi 8 specification is on the way: 2.4/5/6GHz triple-band operation
- Vietnam's chip packaging and testing business is growing, and supply-side fragmentation is splitting the market
- Vietnam's chip packaging and testing business is growing, and supply-side fragmentation is splitting the market
- Three steps to govern hybrid multicloud environments
- Four-axis special PID parameter tuning method and principle
- [Hua Diao Experience] 10-line empty board hardware control pinpong library series test (Part 3)
- Can this schematic control the LDO enable and disable through transistors?
- Huada HC32F4A0 replaces STM32F405/407/427 series chips--simple replacement comparison
- What is the principle of this temperature measuring water cup? Doesn't it need to replace the battery?
- Why can byte addresses overlap with bit addresses?
- Creative Cube
- 10 ways to dissipate heat from PCB!!!
- LOTO Lesson 3: Frequency Response Curve Plotting --- 3 groups of RC low-pass filter tests with different parameters
- Three major programming languages for the development of the Internet of Things