Setting Pin Function
Set systick to 1s interrupt
Using systick interruption, you can get 1s time
/// ...
The systick of stm32 is set by a few programs. After using the systick_config() function, its loaded value is your parameter, and the interrupt is automatically turned on, and the interrupt is set to the lowest priority, and its clock is set to HCLK, that is, the system clock 72mhz, and the count register is reset to start counting. The clock can also be set to eight times the frequency of HCKL by using SysTick_CLKSourceConfig(SysTick_CLKSource_HCLK_Div8) immediately following systick_config(), and changing the priority uses the NVIC_SetPriority(SysTick_IRQn,...) function, and the time base unit is set using the following formula, Reload Value = SysTick Counter Clock (Hz) x Desired Time base (s) Reload Value is the parameter passed in. But the parameter cannot exceed 0xFFFFFF.
When using systick delay, you can also read and write its registers directly without enabling interrupts.
SysTick_Config(uint32_t
(SysTick_CTRL_CLKSOURCE_Msk), so the timing time = ticks
////////////////////////////////////////////////////// ////////////////////////////////////////////////////// ////////////////////////////////////////////////////// /////////////////////////////
The 3.4 library is used here, note that it is SystemCoreClock, the 3.0 versions use the word SystemFrequency, the methods of other 2.x versions are different.
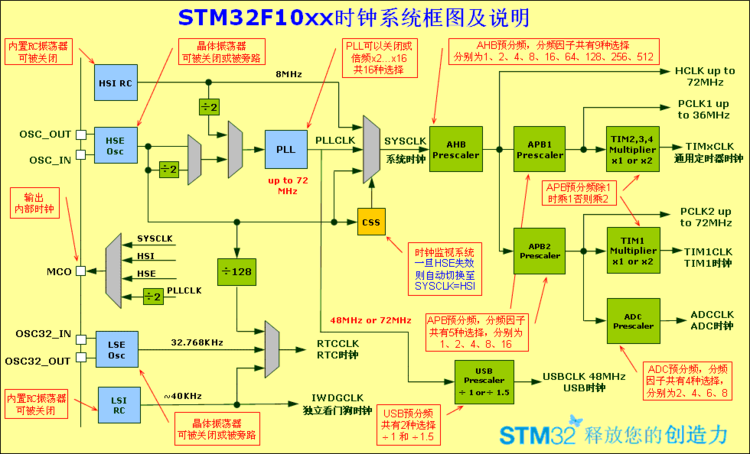
Note: The global variable TimingDelay must be defined as volatile type, and the delay time will not change with the system clock frequency.
![[Reprint] STM32 Quick Learning 6——SysTick Timing 1s Control LED - ╄→Wind, Blowing Away - ╄→Wind, Blowing Away](http://img6.ph.126.net/3rDV5VrojL3BalTF5xdlww==/6597656905865474656.jpg)
Main file
#include "stm32f10x.h"
void RCC_Configuration(void);
void GPIO_Configuration(void);
void SysTick_Configuration(void);
void Delay(volatile uint32_t nTime);
static volatile uint32_t TimingDelay;
int main(void)
{
RCC_Configuration();
GPIO_Configuration();
SysTick_Configuration();
while(1)
{
GPIO_SetBits(GPIOA, GPIO_Pin_0);
Delay(1000);
GPIO_SetBits(GPIOA, GPIO_Pin_1);
Delay(1000);
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
Delay(1000);
GPIO_ResetBits(GPIOA, GPIO_Pin_1);
Delay(1000);
}
}
void RCC_Configuration(void) /*Use external 8M*/
{
ErrorStatus HSEStartUpStatus;
/* RCC system reset(for debug purpose) */
RCC_DeInit();
/* Enable HSE */
RCC_HSEConfig(RCC_HSE_ON);
/* Wait till HSE is ready */
HSEStartUpStatus = RCC_WaitForHSEStartUp();
if(HSESTartUpStatus == SUCCESS)
{
/* Enable Prefetch Buffer Prefetch buffer enable */
FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable);
/* Flash 2 wait state, FLASH memory delay clock cycles*/
FLASH_SetLatency(FLASH_Latency_2);
/* HCLK = SYSCLK */
RCC_HCLKConfig(RCC_SYSCLK_Div1);
/* PCLK2 = HCLK */
RCC_PCLK2Config(RCC_HCLK_Div1);
/* PCLK1 = HCLK/2 */
RCC_PCLK1Config(RCC_HCLK_Div2);
/* PLLCLK = 8MHz * 9 = 72 MHz */
RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9);
/* Enable PLL */
RCC_PLLCmd(ENABLE);
/* Wait till PLL is ready */
while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET)
{
}
/* Select PLL as system clock source */
RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK);
/* Wait till PLL is used as system clock source */
while(RCC_GetSYSCLKSource() != 0x08)
{
}
}
/* Enable GPIOC clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void SysTick_Configuration()
{
if (SysTick_Config(SystemCoreClock / 1000))
{
/* Capture error */
while (1);
}
}
void Delay(volatile uint32_t nTime)
{
TimingDelay = nTime;
while(TimingDelay != 0);
}
void TimingDelay_Decrement(void)
{
if (TimingDelay != 0x00)
{
TimingDelay--;
}
}
Stm32f10x_it.c added
void TimingDelay_Decrement(void);
void SysTick_Handler(void)
{
TimingDelay_Decrement();
}
Previous article:STM32 power-off detection + Flash access
Next article:stm32 quick learning 2-light up the LED
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Flash (STM32) "Big Explanation"
- 【Live FAQ】Develop AI intelligent robots based on TI's newly released Robotics SDK
- ISA bus interface.pdf
- Please explain the assembly program of digital voltmeter
- DSP structural characteristics and computing performance
- Touch screen interface definition
- Compile QtE5.7 source code on itop4412 development board
- Overview of 5G System Standard Development
- 2. [Learning LPC1768 library functions] Keystroke experiment
- How to change UDP point-to-point communication to UDP broadcast communication