/********************************************************************************
DS18B20 temperature measurement program
Hardware: AT89S52
(1) Single-wire ds18b20 connected to P2.2
(2) Seven-segment digital tube connected to P0 port
(3) Use external power supply to power ds18b20, no parasitic power supply
Software: Kei uVision 3
**********************************************************************************/
#include "reg52.h"
#include "intrins.h"
#define uchar unsigned char
#define uint unsigned int
sbit ds=P2^2;
sbit dula=P2^6;
sbit wela=P2^7;
uchar flag ;
uint temp; //Parameter temp must be declared as int type
uchar code table[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,
0x07,0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79,0x71}; //Digital encoding without decimal point
uchar code table1[]={0xbf,0x86,0xdb,0xcf,0xe6,0xed,0xfd,
0x87,0xff,0xef}; //Digital encoding with decimal point
/*Delay function*/
void TempDelay (uchar us)
{
while(us--);
}
void delay(uint count) //delay sub-function
{
uint i;
while(count)
{
i=200;
while(i>0)
i--;
count--;
}
}
/*Serial port initialization, baud rate 9600, mode 1 */
void init_com()
{
TMOD=0x20; //Set timer 1 to mode 2
TH1=0xfd; //Install initial value and set baud rate
TL1=0xfd;
TR1=1; //Start timer
SM0=0; //Serial port communication mode setting
SM1=1;
// REN=1; //Serial port allows receiving data
PCON=0; //Baud rate is not multiplied
// SMOD=0; //Baud rate is not multiplied
// EA=1; //Open general interrupt
//ES=1; //Open serial interrupt
}
/*Display of digital tube*/
void display(uint temp)
{
uchar bai,shi,ge;
bai=temp/100;
shi=temp%100/10;
ge=temp%100%10;
dula=0;
P0=table[bai]; //Display hundreds digit
dula=1; //From 0 to 1, there is a rising edge, release the latch and display the corresponding segment
dula=0; //From 1 to 0, latch again
wela=0;
P0=0xfe;
wela=1;
wela=0;
delay(1); //Delay about 2ms
P0=table1[shi]; //display tens digit
dula=1;
dula=0;
P0=0xfd;
wela=1;
wela=0;
delay(1);
P0=table[ge]; //Display the unit digit
dula=1;
dula=0;
P0=0xfb;
wela=1;
wela=0;
delay(1);
}
/*********************************************
Timing: initialization timing, read timing, write timing.
All timings use the host (MCU) as the master device and the single
bus device as the slave device. Each command and data transmission
starts with the host actively starting the write timing. If the single
bus device is required to send back data, after the write command, the host needs to start
the read timing to complete the data reception. The transmission of data and commands is low
-order first.
Initialization timing: reset pulse existence pulse
read; 1 or 0 timing
write; 1 or 0 timing
Only the existence pulse signal is sent from 18b20 (slave), and other
signals are sent by the host.
Existence pulse: let the host (bus) know that the slave (18b20) is
ready.
******************************************/
/*------------------------------------------------------------------------------------------------------------------------------------
Initialization: Detect the reset pulse sent by the bus controller
Any communication with ds18b20 must start with initialization
The initialization sequence consists of a reset pulse from the bus master
followed by a presence pulse from the slave.
Initialization: reset pulse + presence pulse
Specific operation:
The bus controller sends (TX) a reset pulse (a low-level signal that lasts at least 480μs), then releases the bus and
enters the receiving state (RX). The single-wire bus is pulled to a high level by a 5K pull-up resistor. After detecting the rising edge on the I/O pin,
the DS1820 waits for 15~60μs and then sends a presence pulse (a low-level signal of 60~240μs).
Specifically see the timing diagram of 18b20 single-line reset pulse timing and 1-wire presence detect "
--------------------------------------------------------------------------------------------------------------------------------*/
void ds_reset(void)
{
ds=1;
_nop_(); //1us
ds=0;
TempDelay(80); //When the bus stays at a low level for more than 480us, all devices on the bus will be reset . Here //the delay is about 530us. The bus stays at a low level for more than 480μs, and all devices on the bus
will //be reset.
_nop_();
ds=1; //After generating the reset pulse, the microprocessor releases the bus and puts the bus in an idle state. The reason is checked in //18b20 Chinese documentation
TempDelay(5); //After releasing the bus, the slave 18b20 can indicate whether it is online by pulling the bus low.
//Presence detection high level time: 15~60us, so delay 44us, perform 1-wire presence //detect (single-wire presence detection)
_nop_();
_nop_();
_nop_();
if(ds==0)
flag=1; //detect 18b20 success
else
flag=0; //detect 18b20 fail
TempDelay(20); //Presence detection low level time: 60~240us, so delay about 140us
_nop_();
_nop_();
ds=1; //Pull the bus high again to make the bus idle
/**/
}
/*----------------------------------------
Read/write time gap:
DS1820 data reading and writing is processed through time gaps
bits and command words to confirm information exchange.
------------------------------------------*/
bit ds_read_bit(void) //read one bit
{
bit dat;
ds=0; //MCU (microprocessor) pulls the bus low
_nop_(); //read time slot starts when microprocessor pulls the bus low for at least 1us
ds=1; //pull the bus low and then release the bus, so that slave 18b20 can take over the bus and output valid data
_nop_();
_nop_(); //delay a little and read the data on 18b20, because the data output from ds18b20
is valid within 15us of the falling edge of the read "time slot"
dat=ds; //host reads the data output from slave 18b20, and these data appear within 15us of the falling edge of the read time slot
TempDelay(10); //all read "time slots" must be 60~120us, here 77us
return(dat); //return valid data
}
uchar ds_read_byte(void ) //read one byte
{
uchar value,i,j;
value=0; //Don't forget to give the initial value
for(i=0;i<8;i++)
{
j=ds_read_bit();
value=(j<<7)|(value>>1); //The instructions for this step are in a word document
}
return(value); //Return a byte of data
}
void ds_write_byte(uchar dat) //Write a byte
{
uchar i;
bit onebit; //Don't forget, onebit is one bit
for(i=1;i<=8;i++)
{
onebit=dat&0x01;
dat=dat>>1;
if(onebit) //Write 1
{
ds=0;
_nop_();
_nop_(); //Look at the timing diagram, at least 1us is delayed to generate a write "time gap"
ds=1; //The data line is allowed to be pulled high within 15μs after the start of the write time gap
TempDelay(5); //All write time gaps must last at least 60us
}
else //Write 0
{
ds=0;
TempDelay(8); //The host needs to generate a write-0 time gap. It must pull the data line to a low level and maintain it for at least 60μs, 64us here.
ds=1;
_nop_();
_nop_();
}
}
}
/********************************************
The host (single-chip microcomputer) controls 18B20 to complete temperature conversion through three steps:
18B20 must be reset before each read and write, and after the reset is successful,
a ROM instruction is sent, and finally a RAM instruction is sent, so that the DS18b20 can perform
the predetermined operation.
Reset requires the main CPU to pull down the data line for 500us and then release it. When ds18B20
receives the signal, it waits for 16~60us, and then sends a 60~240us low pulse.
The main CPU receives this signal to indicate that the reset is successful
**********************************************/
/*----------------------------------------
Perform temperature conversion:
Initialize first
Then skip ROM: Skip 64-bit ROM address and send temperature conversion command directly to ds18B20, suitable for single chip operation
Send temperature conversion command
------------------------------------------*/
void tem_change()
{
ds_reset();
delay(1); //约2ms
ds_write_byte(0xcc);
ds_write_byte(0x44);
}
/*----------------------------------------
Get temperature:
------------------------------------------*/
uint get_temperature()
{
float wendu;
uchar a,b;
ds_reset();
delay(1); //about 2ms
ds_write_byte(0xcc);
ds_write_byte(0xbe);
a=ds_read_byte();
b=ds_read_byte();
temp=b;
temp<<=8;
temp=temp|a;
wendu=temp*0.0625; //Get the true decimal temperature value, because DS18B20
//can be accurate to 0.0625 degrees, so the lowest bit of the read back data represents //0.0625 degrees
temp=wendu*10+0.5; //Magnify ten times. The purpose of doing this is to
convert the first decimal point into a displayable number and perform a rounding operation.
return temp;
}
/*----------------------------------------
Read ROM
------------------------------------------*/
/*
void ds_read_rom() //Not used here
{
uchar a,b;
ds_reset();
delay(30);
ds_write_byte(0x33);
a=ds_read_byte();
b=ds_read_byte();
}
*/
void main()
{
uint a;
init_com();
while(1)
{
tem_change(); //12-bit conversion time is up to 750ms
for(a=10;a>0;a--)
{
display( get_temperature());
}
}
}
Previous article:C language program using DS18B20 temperature sensor in single chip microcomputer (reference 2)
Next article:Interrupt Priority of MCS-51 Series Microcontrollers
Recommended ReadingLatest update time:2024-11-15 17:57
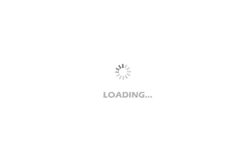
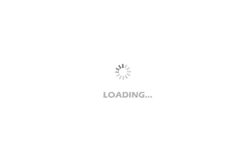
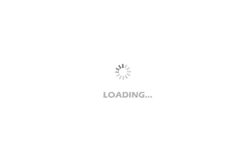
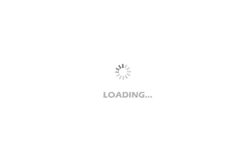
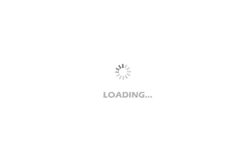
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- [Bluesun AB32VG1 RISC-V evaluation board] IDE installation and use
- It's New Year's Eve soon. I wish you all a happy New Year.
- Using Ginkgo USB-ADC and heart rate sensor to implement a heart rate tester with Android APP source code
- 【DIY Creative LED】Add touch button and install it on LED lamp
- [RISC-V MCU CH32V103 Review] - 2: Making an LED flash is not easy
- SensorTile.box uses air pressure sensors to analyze environmental changes
- When using an oscilloscope probe to measure the AC waveform between the two ends of a component, how should the probe ground wire be connected?
- 【ST NUCLEO-H743ZI Review】(2)Ethernet Test
- TI.com Online Purchasing Special (Smart Building) has a limited time offer, with discounts as low as 30%!
- [Teardown] Apple Watch Series 6, with a bigger battery, thinner ceramic and sapphire casings, stronger and more wear-resistant!