* File name: main.c
* Program author: kidcao1987
* Program version: V1.0
* Function description: Install LCD1602 and display the current temperature on it.
* Compiler: WinAVR-20090313
* Chip: ATmega16, external 11.0592MHZ crystal oscillator
* Technical support: http://bbs.cepark.com
**********************************************************************/
#include
#include
#define uchar unsigned char
#define uint unsigned int
#define DQ PORTC&=~(1<
#define RS PA4
#define RW PA5
#define EN PA6
unsigned char const LedData[]=
{0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xF8,
0x80,0x90,0x88,0x83,0xc6,0xa1,0x86,0x8e};
unsigned char const LedPos[]=
{0x01,0x02,0x04,0x08,0x10,0x20,0x40,0x80};
uchar DisplayNum[]={"0123456789.-C"};
uchar const CharacterChar[]={"Now the Tempera-ture is: "};
uchar Num[4];
uchar TemperatureL,TemperatureH,m;
uint Temperature;
void LCD1602_Initial(void);
void LCD1602_CommandWrite(uchar com);
void LCD1602_ByteWrite(uchar date);
void LCD1602_Display(uchar adr,uchar date);
void LCD1602_BusyCheck(void);
void DS18B20_Reset(void);
void DS18B20_PORTx_Initial(void);
void DS18B20_High_BitWrite(void);
void DS18B20_Low_BitWrite(void);
void DS18B20_ByteWrite(uchar command);
uchar DS18B20_ByteRead(void);
int main(void)
{
DS18B20_PORTx_Initial();
LCD1602_Initial();
while(1)
{
DS18B20_Reset();//复位
DS18B20_ByteWrite(0xcc);//跳过ROM匹配
DS18B20_ByteWrite(0x44);//温度转换
_delay_ms(600);//延时等待温度转换结束,这个很重要!!
DS18B20_Reset();//复位
DS18B20_ByteWrite(0xcc);//跳过ROM匹配
DS18B20_ByteWrite(0xbe);//读取温度
TemperatureL=DS18B20_ByteRead();
TemperatureH=DS18B20_ByteRead();//先低字节后高字节
DS18B20_Reset();//复位打算DS18B20的输出
/*以下是温度数据处理以及显示部分*/
Temperature=TemperatureL*6.25+TemperatureH*1600;
Num[0]=Temperature/1000;
Num[1]=(Temperature%1000/100);
Num[2]=Temperature%100/10;
Num[3]=Temperature%10;
for(m=0;m<16;m++)
{
LCD1602_Display(0x80+m,CharacterChar[m]);
}
for(m=0;m<9;m++)
{
LCD1602_Display(0x80+0x40+m,CharacterChar[16+m]);
}
LCD1602_ByteWrite(DisplayNum[Num[0]]);
LCD1602_ByteWrite(DisplayNum[Num[1]]);
LCD1602_ByteWrite(DisplayNum[10]);
LCD1602_ByteWrite(DisplayNum[Num[2]]);
LCD1602_ByteWrite(DisplayNum[Num[3]]);;
LCD1602_ByteWrite(0xdf);
LCD1602_ByteWrite(DisplayNum[12]);
}
}[page]
void DS18B20_PORTx_Initial(void)
{
DDRB=0xff;//B口全部输出
PORTB=0xff;//B口全部低电平
DQ;
DQ_ActiveHigh;
}
void DS18B20_Reset(void)
{
DQ_ActiveLow;
_delay_us(600);//延时至少480us
DQ_ActiveHigh;//释放数据线
_delay_us(70);//延时大于60us
while(DQ_Readback);//Detect the presence of signal_delay_us
(500);//Delay to ensure timing integrity
}
void DS18B20_High_BitWrite(void)
{
DQ_ActiveLow;//Pull to low
level_delay_us(2);//Maintain at least 1us
DQ_ActiveHigh;//Release the data line_delay_us
(65);//Wait for enough time for DS18B20 to sample the data line
}
void DS18B20_Low_BitWrite(void)
{
DQ_ActiveLow;//Pull to low
level_delay_us(65);//Wait for enough time for DS18B20 to sample the data line
DQ_ActiveHigh;//Release the data line
}
uchar DS18B20_BitRead(void)
{
DQ_ActiveLow;//Pull to low
level_delay_us(2);//Maintain at least 1us
DQ_ActiveHigh;//Release data line
_delay_us(4);//Delay to allow the host to read the data line in the later stage of 15us
if(DQ_Readback)
return 1;
else
return 0;
}
/*DS18B20 byte write function*/
void DS18B20_ByteWrite(uchar command)
{
uchar n=0;
for(n=0;n<8;n++)
{
if(command&0x01)
DS18B20_High_BitWrite();
else
DS18B20_Low_BitWrite();
command>>=1;
_delay_us(5);
}
}
/*DS18B20 byte read function*/
uchar DS18B20_ByteRead(void)
{
uchar n=0,Readback=0;
for(n=0;n<8;n++)
{
Readback>>=1;
if(DS18B20_BitRead())
Readback|=0x80;
_delay_us(65);
}
return Readback;
}
void LCD1602_Initial(void)
{
PORTA&=~((1<
PORTB=0x00;
DDRB=0xff;
LCD1602_CommandWrite(0x38);
_delay_ms(15);
LCD1602_CommandWrite(0x38);
_delay_ms(5);
LCD1602_CommandWrite(0x38);
_delay_ms(5);
LCD1602_CommandWrite(0x01);
_delay_ms(1);
LCD1602_CommandWrite(0x38);
_delay_ms(1);
LCD1602_CommandWrite(0x0c);
_delay_ms(1);
LCD1602_CommandWrite(0x06);
}
/*1602写命令字子函数*/
void LCD1602_CommandWrite(uchar com)
{
LCD1602_BusyCheck();
PORTA&=~(1<
PORTA|=(1<
PORTA&=~(1<
/*1602 write data sub-function*/
void LCD1602_ByteWrite(uchar date)
{
LCD1602_BusyCheck();
PORTA|=(1<
PORTA|=(1<
PORTA&=~(1<
/*Display a character at a certain position, parameter adr is the address to be written, date is the byte to be written*/
void LCD1602_Display(uchar adr,uchar date)
{
LCD1602_BusyCheck();
LCD1602_CommandWrite(adr) ; LCD1602_BusyCheck
();
LCD1602_ByteWrite(date);
}
void LCD1602_BusyCheck(void)
{
PORTB=0xff;
DDRB=0x00;
PORTA|=(1<
while(PINB&0x80);
PORTA&=~(1<
DDRB=0xff;
}
Video address: http://v.youku.com/v_show/id_XMTYxNDkyOTgw.html
Previous article:AVR M16 Experiment 7 DS1302 Test
Next article:AVR M16 Experiment 5 Matrix Keyboard
Recommended ReadingLatest update time:2024-11-16 16:02
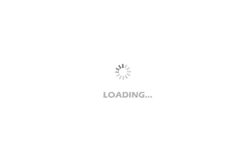
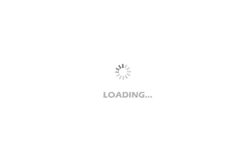
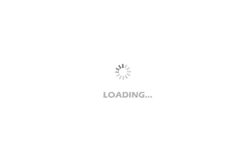
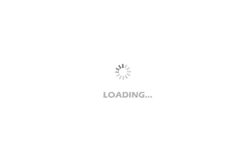
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Metronom Real-Time Operating System RTOS for AVR microcontrollers
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The world's first single-chip microcomputer was produced by TI
- EEWORLD University ---- Digital Integrated Circuit Analysis and Design
- The contradiction between insulation withstand voltage and ESD
- Buck Circuit
- High pass filter gain problem
- Using FPGA to realize accurate time keeping when GPS is out of step
- BlueNRG-1/2 Flash operations require mutual exclusion with BLE events
- 1S2192 Parameters
- Does anyone have the BAP protocol in Volkswagen's CAN protocol?
- The Definitive Guide to Automotive Ethernet