.c file (main program):
Keywords:MCU
Reference address:The single chip microcomputer STC5A60S2 completes audio acquisition and output
/*------------------------------------------------ ------------------*/ //General description //The microcontroller used is STC5A60S2, and the external crystal oscillator is 32.768M //Use the AD and PWM of the microcontroller as DA output //Two buttons, one for recording and one for output. The default state after power on is real-time output //External RAM uses 62256 /*------------------------------------------------ ------------------*/ #include#include #include #include //#include //Call the digital filter header file, the content is complicated, and the debugging proof effect is not obvious #define uchar unsigned char #define uint unsigned int sfr AUXR=0x8E; //Control the working speed of timer 0 and 1 sfr BUS_SPEED=0xA1; //Control external RAM speed register sfr P1M1=0x91; //Set the I/O port working status sfr P1M0=0x92; sbit P15=P1^5; //P1^5 connects to button 1 sbit P16=P1^6; //P1^6 connects to button 2 uchar keycode; //define key code variable uchar xdata C5A60S2_ADC[32768]; //Use off-chip RAM to store sample values /*------------------------------------------------ ------------------*/ void keyscan(void) //Scan keyboard subroutine { P15=1; _nop_(); _nop_(); P16=1; _nop_(); _nop_(); if(P15==0) { delay(1); if(P15==0); keycode=1; //Key 1 controls the output off and the acquisition on } if(P16==0) { delay(1); if(P16==0) //Button 2 controls the output on and the acquisition off keycode=2; } } /*------------------------------------------------ ------------------*/ void Init_T() { //BUS_SPEED=0; //Control the external RAM speed to read and write in 1T state. Note that the maximum speed of 62256 is about 14M //PT0=1; //Set priority //PT1=0; AUXR=0Xc0; //Timer 0 and 1 work in non-frequency division state, that is, the speed is 12 times the normal speed P1M1=1; // Make P1.0 work in high impedance state for AD use, P1M0=9; // PWM strong push-pull output current reaches 20mA EA=1; ET0=1; TMOD=0X11; //Timer works in mode 1 TR0=1; //Start timer 0 TL0=0xa0; //Ensure that the sampling speed is consistent with the output speed TH0=0xf0; ET1=1; TR1=1; //Start timer 0 TL1=0xa0; // Ensure that the sampling speed is consistent with the output speed. The sampling speed is 540 clocks. TH1=0xf0; } /*------------------------------------------------ ---------------- */ time0() interrupt 1 using 2 //Timer 0 interrupt is used to collect audio regularly { static uint ram_in=0; //Save into which ram unit ADC_CONTR=0xe8; // Clear the conversion completion flag and start the next conversion C5A60S2_ADC[ram_in++]=ADC_RES;; if(ram_in==32768) ram_in=0; //If RAM is full, return to the first position TL0=0xa0; // The sampling rate is ? clocks TH0=0xf0; } /*------------------------------------------------ ------------------*/ time1() interrupt 3 using 3 //Timer 0 interrupt is used to output audio at a fixed time { static uint ram_out=0; //output the ram unit PWM(C5A60S2_ADC[ram_out++]); //output audio if(ram_out==32768) ram_out=0; //If RAM is read, return to the first position TL1=0xa0; //Ensure that the sampling speed is equal to the output speed TH1=0xf0; } /*------------------------------------------------ ------------------*/ void main() { Init_ADC(); //AD initialization Init_PWM(); //PWM initialization Init_T(); //Initialize the timer while(1) { keyscan() ; if(keycode==1){ TR0=1;TR1=0;CCAPM0=0;keycode=0;} //Key 1 controls the acquisition on and the output off if(keycode==2){ TR0=0;TR1=1;CCAPM0=0x42;keycode=0;} //Key 2 controls the collection off and the output on. } } .H file 1 (AD.H): [page] #define uchar unsigned char #define uint unsigned int /*------------------------------------------------ ---*/ //Note that the AD conversion uses the internal clock /*------------------------------------------------ ---*/ //Define special function registers sfr ADC_CONTR=0xBC; //AD control register sfr ADC_RES=0xBD; //AD output high eight-bit register sfr ADC_LOW2=0xBE; //AD output last two digits register sfr PLASF=0x9D; //Control which of the P1 ports is used as the analog port sfr IPH=0XB7; //interrupt priority control bit sfr AUXR1=0XA2; //Set the storage mode of AD conversion result register //Define the control bits of the registers related to AD #define ADC_POWER 0X80 //AD power control bit #define ADC_FLAG 0X10 //AD conversion completed flag, must be cleared by software #define ADC_START 0X08 //Control AD start conversion bit #define ADC_SPEEDLL 0X00 //540 clocks #define ADC_SPEEDL 0X20 //360 clocks #define ADC_SPEEDH 0X40 //180 clocks #define ADC_SPEEDHH 0X60 //90 clocks sbit EADC=IE^5; //Define the internal AD interrupt flag of the MCU /*------------------------------------------------ --------*/ //Delay subroutine void delay(uint n) { while(n--); } /*------------------------------------------------ --------*/ //Initialize the registers related to the AD built into the microcontroller void Init_ADC() { //IPH=0X20; //AD interrupt is set to the highest priority 0x20 //IP=0X20; // Turn on the interrupt master switch and AD interrupt switch 0x20 //EA=1; //EADC=1; //Turn on the AD interrupt switch. The AD switch flag is the same as the T2 flag of 89C52 //AUXR1=0; //The storage mode of AD conversion result register is 8 high + 2 low. This is the default value and can be left unchanged. PLASF=0x01; //Set P1.0 as analog port ADC_RES=0; //Clear the AD output high eight-bit register //The default AD output storage mode is 8+2 ADC_CONTR=0xe8; //(ADC_POWER|ADC_SPEEDLL|ADC_START|0x00); //Turn on the AD power supply, sample at 90 clock speed, and select P1.0 as the input port delay(1000); //When turning on the internal AD analog power supply for the first time, a proper delay is required to allow the power supply to stabilize. } /*------------------------------------------------ --------*/ .H file 2 (PWM.H): #define uchar unsigned char #define uint unsigned int sfr CCON=0XD8; //PCA control register sfr CMOD=0XD9; //Working mode register sfr CL=0XE9; //PCA counter low 8-bit register sfr CH=0XF9; //PCA counter high 8-bit register sfr CCAPM0=0XDA; //PCA module 0 compare/capture register sfr CCAP0L=0XEA; //PCA module 0 low 8-bit capture/compare register sfr CCAP0H=0XFA; //PCA module 0 high 8-bit capture/compare register sfr PCAPWM0=0XF2; //PWM register of PCA module 0 sbit CCF0=CCON^0; //PCA module 0 interrupt flag, must be cleared by software sbit CCF1=CCON^1; //PCA module 1 interrupt flag, must be cleared by software sbit CR=CCON^6; //PCA count control bit sbit CF=CCON^7; //PCA counter overflow flag void Init_PWM() //Initialize PWM related registers { CCON=0; //PCA control register clear CL=0; //PCA counter low 8-bit register CH=0; //PCA counter high 8-bit register CMOD=0X08; //Count pulse selection: system clock (the higher the better), disable CF bit interrupt?????? CCAPM0=0X42; //8-bit PWM, no interrupt PCAPWM0=0X00; //Combined with CCAP0H, CCAP0L to form a 9-digit number CR=1; //Start PCA counting, must be cleared by software } void PWM(uchar dutyfactor) //Duty cycle adjustment subroutine { CCAP0H=CCAP0L=255-dutyfactor; //Control duty cycle } //The above program is given in the form of a header file. The format is incomplete and I have no time to organize it. If you need reference or have any questions, please leave a message.
Previous article:Simple calculator source code
Next article:Finding the way of C language in reflection
Recommended ReadingLatest update time:2024-11-15 16:53
STMicroelectronics' new MCUs advance electric vehicles and help software-defined electric vehicles
STMicroelectronics' new MCUs advance electric vehicles and help software-defined electric vehicles Stellar E-Series EV-specific microcontrollers promote centralized electrical architecture Simplify the design of high-efficiency power modules and digital power conversion systems for on-board chargers February 2
[Automotive Electronics]
ICC AVR MCU Beginner's Guide
AVR ICC uses the Quick Start Menu to explain the next page: The first program for a novice, to achieve a continuous flashing of the traffic light .
ICC AVR is a very useful AVR compiler software, the official website: www.imagecraft.com The latest version is 7.0, all the examples on this site are based on ICC AVR as t
[Microcontroller]
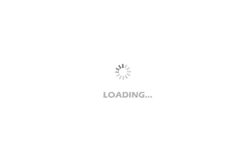
STM8 MCU PWM Application (IAR)
The multiplexed function of PD3 is TIM2_CC2, which can be used to test the PWM function. This example uses a potentiometer to adjust the PWM pulse width to adjust the brightness of LED1 connected to PD3.
#include iostm8s207sb.h
void CLK_init(void)
{
CLK_CKDIVR = 0x08; // 16M internal RC is divid
[Microcontroller]
Design of LED guardrail control system based on STC single chip microcomputer
LED guardrail tube, also known as Lide tube, is an advanced LED decorative lighting product. With red, green and blue LED as the light source, it uses microelectronics and digital technology, can perform color chasing, color transition gradient, grayscale change and seven-color change, and can produce a very rich colo
[Microcontroller]
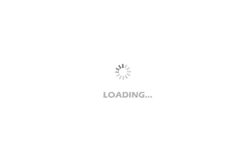
Detailed explanation of MCU source current and sink current
Friends who majored in electronics have all learned 51 single-chip microcomputers and microcomputer principles in college. The above mentioned the current sinking and current sourcing of single-chip microcomputers. Were you confused and didn’t understand at the time? At that time, I remember that when the teacher was
[Microcontroller]
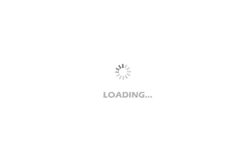
How to calculate the value assigned to the microcontroller register
In previous articles, I mentioned that microcontrollers are very simple devices in the electrical field. From the outside, they are just a chip with many pins. Inside, they are a bunch of registers. Different microcontrollers may have different shapes, pin numbers, and pin names on the outside, and different register
[Microcontroller]
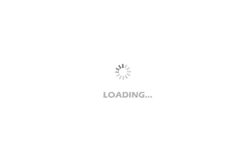
Design of infrared receiving program based on AVR microcontroller
Using the integrated infrared receiver, it can be decoded directly. Usually, the infrared receiver outputs a high level. When there is infrared data, it will have a corresponding level conversion according to the infrared data sent. You can find a remote control board at will, measure the infrared receiving waveform,
[Microcontroller]
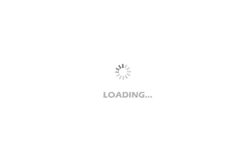
Microcontroller C language programming experience
In the process of writing this 8*8 key program, whether I was writing it myself or referring to other people's programs, I found that I didn't understand many basic knowledge points and programming specifications of C language. Some of them were due to my previous bad programming habits, and some were the manifestatio
[Microcontroller]
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
MoreDaily News
- Huawei's Strategic Department Director Gai Gang: The cumulative installed base of open source Euler operating system exceeds 10 million sets
- Download from the Internet--ARM Getting Started Notes
- Learn ARM development(22)
- Learn ARM development(21)
- Learn ARM development(20)
- Learn ARM development(19)
- Learn ARM development(14)
- Learn ARM development(15)
- Analysis of the application of several common contact parts in high-voltage connectors of new energy vehicles
- Wiring harness durability test and contact voltage drop test method
Guess you like
- FPGA Essay - On Deficiencies
- [NUCLEO-L552ZE Review] + ADC-based fluorescence value reading
- TI Live: Detailed talk on "Bidirectional CLLLC Resonant, Dual Active Bridge (DAB) Reference Design", watch and win gifts (64 in total)
- How does AD software collect company information?
- [GD32L233C-START Review] 11. GD32 ISP software does not support GD32L233 yet
- Last day! Answer questions to win prizes! Practical sharing | Design a reliable switching power supply from scratch
- How to detect whether the switching power supply transformer is good or bad?
- micropython update: 2021.4
- The battery voltage is pulled down
- Is there any abnormal sound from the common mode inductor?