Abstract: USB2.0 interface technology provides a flexible and efficient bidirectional data channel between peripherals and hosts. It can be widely used in data acquisition, industrial control and consumer digital. This paper briefly introduces the structure of the interface chip CY7C68013 that supports the USB2.0 protocol. The structure of the firmware program framework of the CY7C68013 chip is analyzed. The method of how to use C language to develop firmware programs under this firmware program framework is given.
Keywords
: USB2.0, interface, firmware program
Introduction:
Modern industrial production and scientific research have increasing requirements for data acquisition. In some high-speed and high-precision measurements such as transient signal measurement and image processing, high-speed data acquisition is required. The USB2.0 interface has gradually replaced the traditional ISA and PCI data buses with its advantages such as high speed and flexibility. At the same time, as a flexible and low-cost interface technology, the USB interface has become the preferred interface for various PC peripherals with its hot-swappable characteristics. Therefore, the development of USB2.0 has become a hot topic at present.
The following will take the Cypress CY7C68013 chip as an example to discuss the development of firmware programs that support the USB2.0 protocol.
1. Introduction to the interface chip CY7C68013:
The CY7C68013 chip is a powerful interface chip in the EZ-USB FX2 series of Cypress that meets both the USB2.0 protocol and is compatible with the USB1.1 protocol. Its structure is shown in the figure below [2]:
Figure 1 CY7C68013 interface chip structure
The chip has the following features [2]:
1) Integrated with an enhanced 8051 core
Compared with the ordinary 8051 microprocessor, this enhanced processor has the following improvements:
l Only 4 clock cycles are needed to complete each instruction cycle;
l The clock frequency can be soft-configured to 12/24/48MHz;
2) Integrated with a serial interface engine (SIE) and a USB2.0 transceiver
Since the USB2.0 transceiver and the serial interface engine complete the USB protocol's packetization and unpacking functions, the electrical characteristics of the underlying signal are shielded.
3) Supports soft configuration:
Using the re-enumeration technology, the firmware program can be saved on the host, and the firmware is downloaded to the chip RAM through the USB interface after each power-on. It has great flexibility.
4) General Programmable Interface (GPIF):
GPIF provides programmable interface timing, so that it can connect to peripheral chips such as DSP, ASIC, etc. without additional logic (glue logic), and also supports bus standards such as ATAPI and EPP.
5) Four programmable ports (Endpoint):
CY7C68013 has a total of 7 input and output ports: EP0, EP1OUT, EP1IN, EP2, EP4, EP6, EP8. EP2, EP4, EP6, EP8 can be configured as batch/interrupt/synchronous transmission mode, and the transmission direction can be configured as out/in.
6) Programmable buffer (Buffer) depth:
The buffer size of ports EP2 and EP6 can be programmed to 512 or 1024 bytes, and the depth can be programmed to 2/3/4 times the size; the buffer size of ports EP4 and EP8 is fixed to 512 bytes, and the depth is 2 times. Use different configuration methods to achieve data transmission with specific bandwidth and rate requirements.
2. Development Tools:
The Cypress website (http://www.cypress.com) provides a download of the CY7C68013 chip development kit. The development kit provides some resources required for developing firmware programs: Keil uVision2 integrated development environment (limited version); Cypress C51 firmware framework program and some example programs.
Keil uVision2 is a powerful integrated development environment that integrates a series of tools such as the C51 compiler, A51 assembler, and BL link locator, as well as simulation and debuggers. Therefore, it can support projects that mix C programs and assembly programs, bringing great convenience to software development [5].
Keil C51 is a high-efficiency C language compiler designed specifically for 8051 microcontrollers. It complies with ANSI standards. The generated program code runs at an extremely high speed and requires very little storage space, which is completely comparable to assembly language. At the same time, C51 has a rich library of functions, with more than 100 functional functions. Therefore, using C as the development language and Keil uVision2 as the engineering development platform to complete source code writing, simulation, and debugging will significantly reduce the difficulty of firmware development and improve development efficiency [4].
3. Firmware structure and composition:
Although the functions of the firmware program are complex and require a large number of functions when writing, its basic structure is relatively simple and includes the following parts:
3.1 Device Descriptor Table:
Before each USB device establishes data communication with the host, it must first inform the host of its specific configuration, including the manufacturer of the device, product identification number (VID, PID), power supply mode of the device, energy consumption and other important information. And this information about the device is notified to the host through the device descriptor table, so that the host can establish a connection with the device in an appropriate manner. Its structure is as follows:
DeviceDscr:
db 18;; The length of the descriptor (in bytes)
db DSCR_DEVICE;; Descriptor type
...
db 1;; There are several configurations (1 type)
3.2 Firmware program framework source code:
This file provides a firmware program framework structure, which is suitable for general data transmission control. This structure provides a program interface open to developers, and developers implement the required functions by adding appropriate code to these interface functions.
Its workflow is shown in Figure 2.
Figure 2 Firmware program framework workflow
3.3 Interface functions:
The firmware framework provides a function interface. By adding self-developed code to these interface functions, specific functions can be implemented and the difficulty of firmware development can be greatly reduced, thus accelerating the USB system development process. These interface functions are divided into three categories: task allocation, standard device request execution, and USB bus interrupt processing [3]. The following will introduce these interface functions and their uses in turn.
3.3.1 Task allocation
TD_Init()
Description: This function is mainly used to complete the initialization of FX2. It is called before FX2 enumerates again and starts task allocation. Its purpose is to initialize each port and the first-in first-out buffer of each port.
TD_Poll()
Description: This function is called repeatedly when the device is running. It should include code to complete a special task. Before this function returns, the high-priority task may have been completed. However, if it returns a false value, FX2 will not affect the device request and USB bus device suspend event. If a large amount of processing time is required, FX2 will divide the time by calling the TD_Poll() function multiple times.
TD_Suspend()
Description: This function is called before the device enters the suspended state. Developers add appropriate code to configure the working state of the device, which can put the device in a low-power state and return a true value. However, developers can modify the program code of TD_Suspend() to return false, so that FX2 does not enter the suspended state.
TD_Resume()
Description: When an external request to restart is made (for example, a Wakeup interrupt is generated by the outside world or there is a transmission activity on the USB bus), the device will restart the processor by calling this function, which is the reverse operation of the TD_Suspend() function. At this time, the device restarts under normal power supply.
3.3.2 Device Request
The device request function has the following form:
BOOL DR_xxx(void)
{
……
file://device request processing code
return(TURE);
}
It mainly completes the processing of commands and requests from the host, such as configuring ports, etc.
3.3.3 USB Interrupts
The interrupts of FX2 devices include the same interrupts as standard 8051 interrupts, and also include some FX2-specific interrupts. Since the interrupt service routine interface is provided in the firmware program, developers only need to add their own interrupt service code to the interrupt service routine interface to complete the service of the corresponding interrupt request, thus avoiding in-depth research on the logical structure of FX2's interrupt autovectors [2]. The form of these interrupt service routine interface functions is:
void ISR_xxx(void) interrupt 0
{
……
file://Developer interrupt service code
EZUSB_IRQ_CLEAR();
USBIRQ = bmXXX; // Clear the interrupt request
}
4. Summary:
After understanding the Cypress firmware program framework structure, using the Keil uVision2 development environment to develop under the Cypress firmware framework can greatly reduce the difficulty of firmware program development and shorten the development cycle to achieve higher efficiency.
Previous article:The principle of USB dual-machine communication
Next article:Interface circuit diagram of X9628 and PIC16C72
Recommended ReadingLatest update time:2024-11-16 18:08
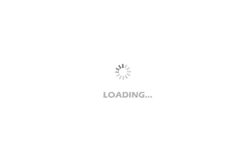
- High signal-to-noise ratio MEMS microphone drives artificial intelligence interaction
- Advantages of using a differential-to-single-ended RF amplifier in a transmit signal chain design
- ON Semiconductor CEO Appears at Munich Electronica Show and Launches Treo Platform
- ON Semiconductor Launches Industry-Leading Analog and Mixed-Signal Platform
- Analog Devices ADAQ7767-1 μModule DAQ Solution for Rapid Development of Precision Data Acquisition Systems Now Available at Mouser
- Domestic high-precision, high-speed ADC chips are on the rise
- Microcontrollers that combine Hi-Fi, intelligence and USB multi-channel features – ushering in a new era of digital audio
- Using capacitive PGA, Naxin Micro launches high-precision multi-channel 24/16-bit Δ-Σ ADC
- Fully Differential Amplifier Provides High Voltage, Low Noise Signals for Precision Data Acquisition Signal Chain
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Four aspects to understand the internal loss of switching power supply
- Some time functions in C language (time/sleep/clock)
- ESP32 MicroPython Web Server – Web page displaying sensor data
- [Good memory is not as good as bad writing] 1. F1 MDK transplantation FreeRTOS practice record 2-CUBE generation project
- National Undergraduate Electronic Design Competition Paper Album
- How to choose the size of the capacitor and resistor when connecting the signal line (clock) in series?
- DSP digital anti-noise module for airborne communication equipment
- Resolving Wi-Fi and Bluetooth issues caused by wireless interference
- A First Look at ESP32--1
- How to quickly switch between Nuvoton M0/M23/M4 development platforms - Nuvoton Tools