The kernel I use is linux2.6.38. At the beginning, the development board can be used as a USB flash drive, but when using the HID function, the following problems occur:
g_hid gadget: hid_setup crtl_request : bRequestType:0x21 bRequest:0xa Value:0x0
g_hid gadget: Unknown request 0xa
s3c-hsotg s3c-hsotg: writen DxEPCTL=0x04228000 to 00000900 (DxEPCTL=0x00208000)
s3c-hsotg s3c-hsotg: s3c_hsotg_rx_data: FIFO 8 bytes on ep0 but no req (DxEPCTl=0x00028000)
s3c-hsotg s3c-hsotg: s3c_hsotg_rx_data: FIFO 8 bytes on ep0 but no req (DxEPCTl=0x00028000)
s3c-hsotg s3c-hsotg: s3c_hsotg_rx_data: FIFO 8 bytes on ep0 but no req (DxEPCTl=0x00028000)
s3c-hsotg s3c-hsotg: S3C_GINTSTS_USBSusp
This is the information printed out after I turned on the debug information. I thought the device clock was not set correctly. The device clock should be set to 48M, but it turned out that this was not the problem. No matter how I changed the clock, the effect was the same. So last week, I changed the registers one by one, and found that the problem was still the same. So I started to study this: Unknown request 0xa
The general process of hid is s3c_hsotg.c->composite.c->f_hid.c. There are many specific processes on the Internet, so I will not explain them here.
material:
http://blog.csdn.net/wuyuwei45/article/details/8930830
http://blog.csdn.net/fanqipin/article/details/8450694
After struggling for a week, I spent several hours to adjust the HID of 2416. In the end, I had no choice but to do it step by step.
When the host sends a request of 0xa for 6410, the above error will appear directly. However, when the host sends 0xa for 2416, a -95 error will also appear. However, the host will continue to send 0x06 and 0x09 requests. Please check the USB protocol. I will not go into details here.
The operations of 6410 and 2416 when receiving 0xa request are basically the same. The difference is that after receiving 0xa, 2416 will ignore this request and send an empty packet to the host, so that the host will continue to send other requests, while 6410 ignores it after receiving 0xa, but does not send any data to the host, so the subsequent operations cannot be performed.
So the current job is to send an empty packet to the host when the device receives 0xa, so that the enumeration can continue.
The main modification is in the s3c_hsotg_process_control function in s3c_hsotg.c. I won’t analyze the whole process here, I don’t really understand it, haha.
/* as a fallback, try delivering it to the driver to deal with */
if (ret == 0 && hsotg->driver) {
ret = hsotg->driver->setup(&hsotg->gadget, ctrl);
if (ret < 0)
dev_dbg(hsotg->dev, "driver->setup() ret %dn", ret);
}
Here, composite_setup in composite.c processes the request after receiving it. When 0xa is received, an error of -95 is returned. Let's continue reading:
/* the request is either unhandlable, or is not formatted correctly
* so respond with a STALL for the status stage to indicate failure.
*/
if (ret < 0) {
u32 reg;
u32 ctrl;
dev_dbg(hsotg->dev, "ep0 stall (dir=%d)n", ep0->dir_in);
reg = (ep0->dir_in) ? S3C_DIEPCTL0 : S3C_DOEPCTL0;
/* S3C_DxEPCTL_Stall will be cleared by EP once it has
* taken effect, so no need to clear later. */
ctrl = readl(hsotg->regs + reg);
ctrl |= S3C_DxEPCTL_Stall;
ctrl |= S3C_DxEPCTL_CNAK;
writel(ctrl, hsotg->regs + reg);
dev_dbg(hsotg->dev,
"writen DxEPCTL=0x%08x to %08x (DxEPCTL=0x%08x)n",
ctrl, reg, readl(hsotg->regs + reg));
/* don't belive we need to anything more to get the EP
* to reply with a STALL packet */
}
When -95 is returned, only DIEPCTL0 is operated. For specific settings, please refer to the 6410 datasheet.
So we need to add the process of sending an empty packet here. If you look at s3c_hsotg.c, you will find that there is such a function: s3c_hsotg_send_zlp
The function of this function is to send an empty packet to the host. Of course, I cannot copy all of it. Copying all of it will cause problems, so my modifications are as follows:
/* the request is either unhandlable, or is not formatted correctly
* so respond with a STALL for the status stage to indicate failure.
*/
if (ret < 0) {
u32 reg;
u32 ctrl;
dev_dbg(hsotg->dev, "ep0 stall (dir=%d)n", ep0->dir_in);
reg = (ep0->dir_in) ? S3C_DIEPCTL0 : S3C_DOEPCTL0;
/* S3C_DxEPCTL_Stall will be cleared by EP once it has
* taken effect, so no need to clear later. */
if(ret != -95) {
ctrl = readl(hsotg->regs + reg);
ctrl |= S3C_DxEPCTL_Stall;
ctrl |= S3C_DxEPCTL_CNAK;
writel(ctrl, hsotg->regs + reg);
}
else {
/* issue a zero-sized packet to terminate this */
writel(S3C_DxEPTSIZ_MC(1) | S3C_DxEPTSIZ_PktCnt(1) |
S3C_DxEPTSIZ_XferSize(0), hsotg->regs + S3C_DIEPTSIZ(0));
ctrl = readl(hsotg->regs + reg);
ctrl |= S3C_DxEPCTL_CNAK; /* clear NAK set by core */
ctrl |= S3C_DxEPCTL_EPEna; /* ensure ep enabled */
ctrl |= S3C_DxEPCTL_USBActEp;
writel(ctrl, hsotg->regs + reg);
}
dev_dbg(hsotg->dev,
"writen DxEPCTL=0x%08x to %08x (DxEPCTL=0x%08x)n",
ctrl, reg, readl(hsotg->regs + reg));
/* don't belive we need to anything more to get the EP
* to reply with a STALL packet */
}
When if (ret < 0) is changed to the following, and the original settings are retained to avoid problems later, after this modification, when the host sends 0xa to the device, the device will return an empty packet to the host so that the host will continue with the subsequent operations. In this way, you can see your HID device in your computer hardware management.
My level is limited, please point out if there are any errors.
Previous article:Develop ARM programs using only serial ports and network bare metal (OK6410 development board)
Next article:Analysis on the implementation of opengl on s3c6410
Recommended ReadingLatest update time:2024-11-16 08:52
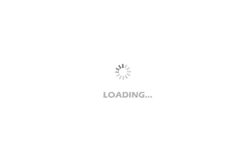
![[linux kernel] Kernel graphical cropping configuration](https://6.eewimg.cn/news/statics/images/loading.gif)
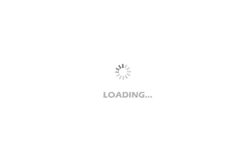
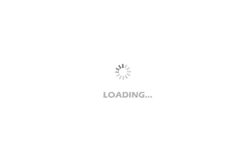
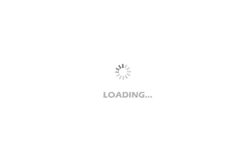
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [RVB2601 Creative Application Development] Support Scheme Language Interpreter
- CATL's sodium-ion battery will be officially mass-produced next year: 80% charge in 15 minutes
- Overview of the process of drawing PCB with KiCad
- PADS9.5 Gerber screen printing changes to copper wire
- ADF4351 chip
- TMS320C6678 Development Routine User Manual Study Part 3
- QuartusII for Altera FPGA development example 1
- Basic knowledge of smart home technology
- A DIY DC to DC negative pressure module
- What is the purpose of the PG pin?