LCD display principle:
To make LCD display on JZ2440, several parts are needed: 1. LCD hardware 2. LCD controller on the development board 3. SDRAM memory to store data FramBuffer 4. A palette may also be needed (actually a piece of memory, in which data can form various colors)
Display principle: Operate the LCD controller on the development board so that the development board removes data from the SDRAM memory and sends it to the LCD screen through the LCD data pin.
Specific hardware operation steps:
1. Connection of LCD pins, configure the pins according to the LCD schematic diagram
2. Set up the LCD controller according to the LCD controller manual
3. Allocate video memory and tell the LCD controller the address
Situations where a palette is needed: LCD data lines are few, for example, 16-bit data lines cannot display all color components and can only be used as an index to point to the actual address color space on the palette
LCD display principle:
You can imagine that there is an electron gun behind the LCD. The function of the electron gun is to print the colors obtained from the FramBuffer memory to the LCD screen one by one. How to print? The order is:
Start from the upper left corner of the LCD screen, and start from left to right, one color dot at a time. When there is no more space, start to wrap and start from left to right again. It is like you open a notepad and press a character or symbol that you don't understand.
It will continuously output letters on the notepad, and when it reaches the boundary, it will start to wrap the output until it is finished. Of course, the space on the LCD screen is limited, and the speed of printing is very fast, so you feel that the image appears suddenly in front of you.
The same as before.
------------------------------------------------------------------------------------------------------------------------------------
LCD driver framework
A framework that meets the needs of character device drivers
1. The application enters the kernel through open, read, write and other functions and then calls dri_open, dri_read, dir_write and other functions to find the registration ID of the LCD driver through the device.
Enter the File_operation structure function of the driver function and operate the hardware. The main framework is shown in the figure.
LCD Driver
Assume
app: open("/dev/fb0", ...) Major device number: 29, Minor device number: 0
--------------------------------------------------------------
kernel:
fb_open
int fbidx = iminor(inode);
struct fb_info *info == registered_fb[0];
app: read()
---------------------------------------------------------------
kernel:
fb_read
int fbidx = iminor(inode);
struct fb_info *info = registered_fb[fbidx];
if (info->fbops->fb_read)
return info->fbops->fb_read(info, buf, count, ppos);
src = (u32 __iomem *) (info->screen_base + p);
dst = buffer;
*dst++ = fb_readl(src++);
copy_to_user(buf, buffer, c)
Q1. Where is registered_fb set?
A1. register_framebuffer
How to write an LCD driver?
1. Allocate an fb_info structure: framebuffer_alloc
2. Set
3. Register: register_framebuffer
4. Hardware-related operations
----------------------------------------------------------------------------------------------------------------
Initialize LCD: What to do in the main entry function
1. Allocate an fb_info structure: framebuffer_alloc
struct fb_info {
........
struct fb_var_screeninfo var; /* Current var variable parameter*/
struct fb_fix_screeninfo fix; /* Current fix fixed parameter*/
........
}
/* 1. Allocate a fb_info */
s3c_lcd = framebuffer_alloc(0, NULL);
2. Setup
/* 2. Setting*/
/* 2.1 Setting fixed parameters Current fix*/
struct fb_fix_screeninfo {
char id[16]; /* identification string eg "TT Builtin" */
unsigned long smem_start; /* Start of frame buffer mem */
/* (physical address) */
__u32 smem_len; /* Length of frame buffer mem */
__u32 type; /* see FB_TYPE_* */
__u32 type_aux; /* Interleave for interleaved Planes */
__u32 visual; /* see FB_VISUAL_* */
__u16 xpanstep; /* zero if no hardware panning */
__u16 ypanstep; /* zero if no hardware panning */
__u16 ywrapstep; /* zero if no hardware ywrap */
__u32 line_length; /* length of a line in bytes */
unsigned long mmio_start; /* Start of Memory Mapped I/O */
/* (physical address) */
__u32 mmio_len; /* Length of Memory Mapped I/O */
__u32 accel; /* Indicate to driver which */
/* specific chip/card we have */
__u16 reserved[3]; /* Reserved for future compatibility */
};
strcpy(s3c_lcd->fix.id, "mylcd");
s3c_lcd->fix.smem_len = 240*320*16/8;
s3c_lcd->fix.type = FB_TYPE_PACKED_PIXELS;
s3c_lcd->fix.visual = FB_VISUAL_TRUECOLOR; /* TFT */
s3c_lcd->fix.line_length = 240*2;
/* 2.2 Set variable parameter Current var */
struct fb_var_screeninfo {
__u32 xres; /* visible resolution */
__u32 yres;
__u32 xres_virtual; /* virtual resolution */
__u32 yres_virtual;
__u32 xoffset; /* offset from virtual to visible */
__u32 yoffset; /* resolution */
__u32 bits_per_pixel; /* guess what */
__u32 grayscale; /* != 0 Graylevels instead of colors */
struct fb_bitfield red; /* bitfield in fb mem if true color, */
struct fb_bitfield green; /* else only length is significant */
struct fb_bitfield blue;
struct fb_bitfield transp; /* transparency */
__u32 nonstd; /* != 0 Non standard pixel format */
__u32 activate; /* see FB_ACTIVATE_* */
__u32 height; /* height of picture in mm */
__u32 width; /* width of picture in mm */
__u32 accel_flags; /* (OBSOLETE) see fb_info.flags */
/* Timing: All values in pixclocks, except pixclock (of course) */
__u32 pixclock; /* pixel clock in ps (pico seconds) */
__u32 left_margin; /* time from sync to picture */
__u32 right_margin; /* time from picture to sync */
__u32 upper_margin; /* time from sync to picture */
__u32 lower_margin;
__u32 hsync_len; /* length of horizontal sync */
__u32 vsync_len; /* length of vertical sync */
__u32 sync; /* see FB_SYNC_* */
__u32 vmode; /* see FB_VMODE_* */
__u32 rotate; /* angle we rotate counter clockwise */
__u32 reserved[5]; /* Reserved for future compatibility */
};
s3c_lcd->var.xres = 240;
s3c_lcd->var.yres = 320;
s3c_lcd->var.xres_virtual = 240;
s3c_lcd->var.yres_virtual = 320;
s3c_lcd->var.bits_per_pixel = 16;
/* RGB:565 */
s3c_lcd->var.red.offset = 11;
s3c_lcd->var.red.length = 5;
s3c_lcd->var.green.offset = 5;
s3c_lcd->var.green.length = 6;
s3c_lcd->var.blue.offset = 0;
s3c_lcd->var.blue.length = 5;
s3c_lcd->var.activate = FB_ACTIVATE_NOW;
/* 2.3 Setting operation function*/
s3c_lcd->fbops = &s3c_lcdfb_ops;
/* 2.4 Other settings*/
s3c_lcd->screen_size = 240*324*16/8;
3. Hardware-related operations
/* 3.1 Configure GPIO for LCD */
/* 3.2 Set up LCD controller according to LCD manual, such as VCLK frequency, etc. */
/* 3.3 Allocate video memory (framebuffer), and tell the address to LCD controller */
4. Registration: register_framebuffer
/* 4. Register */
register_framebuffer(s3c_lcd);
Previous article:I2C Driver Detailed Explanation
Next article:LCD Driver(II)
Recommended ReadingLatest update time:2024-11-16 09:34
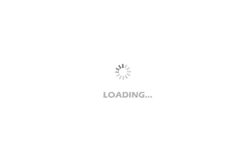
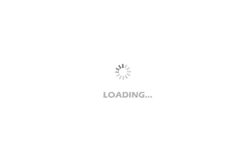
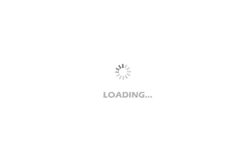
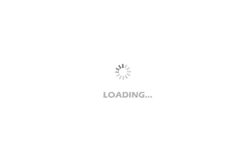
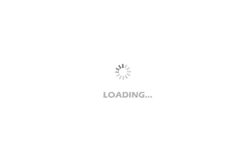
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Last day! Free review of Beetle ESP32-C3, hurry up and get it
- SVPWM principle, implementation, simulation analysis
- Why is the waveform of LM324 distorted?
- Answer the questions to win prizes | TDK special reports are waiting for you
- Today, People's Daily pushes information from the Ministry of Industry and Information Technology
- RK3399 development board Android image burning Windows system image burning
- How to set the original part not to appear in the BOM
- TouchGFX Design Whack-a-Mole
- [Fudan Micro FM33LC046N Review] + Learning UART0 DMA Transmission and Reception
- What are the abbreviations of h and f in the small signal current amplification factor hfe?