Design requirements:#
Using DC motor as fan
The keyboard can adjust the fan speed
Design Overview:
According to the design requirements, the fan needs to be turned on and off with an independent keyboard, and the speed control needs to use PWM technology. The required single-chip microcomputer chip is STC89C52, and the hardware tool used is the smart car based on STC89C52 developed by Huaqing Yuanjian. The car is equipped with the required independent key module and DC motor module. The independent key module is controlled by the P3 port, and the DC motor module is controlled by the P1 port. STC89C52 is a low-power, high-performance 8-bit microcontroller, which is an enhanced version of the 80C51 single-chip microcomputer, but like the 80C51 single-chip microcomputer, it does not have a PWM hardware module, so we need to write a program to simulate the PWM square wave by software.
PWM is a square wave that can realize digital signal control of analog circuits. It has two important parameters: period or frequency and duty cycle. Duty cycle = high level time/period, with a minimum of 0% and a maximum of 100%. By adjusting the duty cycle, the proportion of high level and low level can be controlled, thereby controlling the speed of the DC motor.
DC motor drive: The motor enable terminal P1.4 is valid at high level. The motor enable terminal must be set to 1 before using the DC motor; the forward and reverse rotation of the motor are determined by the level status of P1.2 and P1.3. When the motor rotates forward, P1.2 is set to 1 and P1.3 is set to 0. When the motor rotates reversely, P1.2 is set to 0 and P1.3 is set to 1.
Independent keyboard driver: The independent keyboard has four keys, s2, s3, s4, and s5, which are controlled by P3.0, P3.1, P3.2, and P3.3 respectively. When the key is pressed, P3 is turned on to a low level. When P3.0 is set to 0, it means the key is pressed, and when it is set to 1, it means the key is released. The same is true for the other three keys.
Fan speed: There are two settings for the fan speed.
source code:#
#include
sbit key_s2 = P3^0; //Fan first speed button
sbit key_s3 = P3^1; //Fan second speed button
sbit key_s4 = P3^2; // Turn off the fan button
sbit EN1 = P1^4; //1, motor enable
sbit IN1 = P1^2; //1 means the motor rotates forward
sbit IN2 = P1^3; //1 means the motor will reverse
/*Fan speed 1*/
void fan_motor1()
{
//Define a variable pwm, and adjust the duty cycle of PWM by accumulating pwm
unsigned int pwm;
while(1)
{
for(pwm = 0;pwm <= 1000;pwm++)
{
if(pwm == 700)
{
EN1 = 1;
IN1 = 1;
IN2 = 0;
}
else if(pwm == 1000)
{
EN1 = 0;
}
}
// Press one of the keys to exit the loop and execute the corresponding code section
if(key_s3 == 0 || key_s4 == 0)
break;
}
}
/*Fan second gear speed function*/
void fan_motor2()
{
EN1 = 1;
IN1 = 1;
IN2 = 0;
}
/*Fan shutdown function*/
void fan_motor_stop()
{
EN1 = 0;
}
/*Delay function*/
void delays(unsigned int ms)
{
//If volatile is not added, the compiler will automatically ignore the for loop without loop body
volatile unsigned int i,j;
for(i=ms;i>0;i--)
{
for(j=110;j>0;j--)
;
}
}
/*Timer interrupt service function*/
void timer0 () interrupt 1
{
if(key_s2 == 0) // Check if the key is pressed
{
delays(10); //delay debounce
if(key_s2 == 0) //Confirm that the key has been pressed
{
fan_motor1(); //Open the first gear
}
}
else if(key_s3 == 0)
{
delays(10);
if(key_s3 == 0)
{
fan_motor2(); //Open second gear
}
while(!key_s3);//Wait for the key to be released
}
else if(key_s4 == 0)
{
delays(10);
if(key_s4 == 0)
{
fan_motor_stop(); //Turn off the fan
}
while(!key_s4);
}
}
void main()
{
TMOD |= 1<<1; //Change the state of the bit through the shift operator "<<"
TMOD &= ~(1<<0); //Set the timer/counter to work in mode 2
TMOD &= ~(1<<2); //Select the timing working mode
TMOD &= ~(1<<3); //Gate bit: start the timer by the run control bit TR
TL0 = 156;
TH0 = 156; //100us enters an interrupt, 0.1 milliseconds
ET0 = 1; //Timer 0 interrupt
EA = 1; //CPU interrupt
TR0 = 1; //Start
while(1) //Prevent the program from running away
;
}
Schematic diagram of some modules of the car: #
Previous article:Counter based on STC51 microcontroller
Next article:C51_MCU Development_Use of XBYTE
Recommended ReadingLatest update time:2024-11-16 09:29
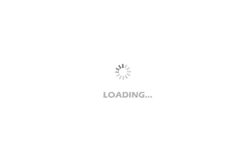
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Can a 100A current flow through a PCB? Tips for setting up high current paths
- hcnr201 isolation circuit problem
- recruitment
- Boot hardware settings
- 2.5V to 6V Input 1.5MHz 2A DC Step-Down Circuit
- 【CODING TALK】How would you implement a queue?
- RVB2601 Development Board Quick Start Guide
- How to input xy coordinates for hole location in pads packaging
- Strange behavior of Firefox browser
- Detailed explanation of ADC function in C8051F020 microcontroller