I. Introduction
Today I would like to recommend a small experiment with 51 microcontroller to you, using the 51 microcontroller to make a simple electronic clock. The timing module uses the DS1302 hardware module, and the display uses an LCD display. Let’s take a look at how to implement it!
2. Introduction to DS1302 module
DS1302 is a trickle charging clock chip launched by DALLAS Company. It contains a real-time clock and 31 bytes of static RAM, and communicates with the microcontroller through a simple serial interface. The real-time clock and calendar circuit provide information on seconds, minutes, hours, days, weeks, months, and years, as well as various functions such as the number of days in each month and automatic compensation for leap years. The clock operation can be indicated by AM/PM. The DS1302 and the microcontroller can simply communicate through synchronous serial. Simple three-wire SPI communication mode:
RES reset
I/O data line
SCLK serial clock
SPI is a high-speed, full-duplex, synchronous communication bus that only occupies four lines on the chip pins, saving chip pins and saving space in the PCB layout, providing convenience and correctness. Because of this simple and easy-to-use feature, more and more chips now integrate this communication protocol, such as the MSP430 microcontroller series processors.
The working principle of DS1302 clock chip is as follows:
There is a set of timers and registers inside the DS1302 chip, through which clock reading and writing operations can be realized.
DS1302 is connected to external devices through three pins, namely RST, DAT and CLK. RST pin is used for reset
DS1302, DAT pin is used for data transmission, and CLK pin is used for clock signal.
The DS1302 chip uses BCD code to represent time information, that is, a 4-digit binary number is used to represent a decimal number. For example, the BCD codes of minutes are 00H to 59H.
3. Code to drive DS1302
The following is the code for using 51 microcontroller to drive the DS1302 clock chip. The specific implementation steps are as follows:
3.1 Initialize DS1302 clock chip
voidDS1302Init(){
//Initialize DS1302 clock chip
DS1302WriteByte(0x8E,0x00);
//Turn off write protection
DS1302WriteByte(0x90,0x00);
}
3.2 Read the time of DS1302 clock chip
voidDS1302ReadTime(unsignedchar*p){
//Read the time of DS1302 clock chip
unsignedchari;
DS1302WriteByte(0xBF,0x00);
for(i=0;i< 7; i++) {
p[i] = DS1302ReadByte();
}
}
3.3 Set the time of DS1302 clock chip
voidDS1302WriteTime(unsignedchar*p){
//Set the time of DS1302 clock chip
unsignedchari;
DS1302WriteByte(0xBE,0x00);
for(i=0;i< 7; i++) {
DS1302WriteByte(p[i], 0x00);
}
}
3.4 Read the RAM of the DS1302 clock chip
//Read one byte of data from DS1302
voidDS1302ReadByte(uchar*dat){
uchari;
for(i=0;i< 8; i++) {
SCLK = 0;
_nop_();
*dat |= IO << i;
SCLK = 1;
_nop_();
}
}
4. Read the RAM of the DS1302 clock chip
The DS1302 clock chip has 31 bytes of RAM space, which can be used to store some data. In practical applications, we may need to read these stored data. Reading the RAM of the DS1302 is similar to reading the register. You also need to send the RAM read command to the DS1302 first, and then read the contents of the RAM.
Reading the RAM of the DS1302 requires the use of another pin of the DS1302 - the CE (Chip Select Enable) pin, which needs to be kept low when reading and writing the RAM of the DS1302. The process of reading RAM is as follows:
4.1 Send command to read RAM
Write the command to read RAM to DS1302: 0x61. DS1302 will automatically switch to RAM read mode and prepare to transfer the data in RAM to the microcontroller.
DS1302Write(0x61);//Send read RAM command
4.2 Read the contents of RAM
After sending the read RAM command, the data in RAM can be read. Reading RAM data requires first reading the high-level pulse on the data pin (IO pin) of DS1302, and then reading 8 bits of data. The specific reading process can be implemented using the DS1302ReadByte function, which reads one byte of data.
for(i=0;i< 31; i++) {
DS1302ReadByte(&byte); // Read one byte of data
ram[i] = byte; // store in array
}
After reading the RAM, we can store it in an array for subsequent use.
4.3 Read part of the separate code implementation (focus on logic)
#include
#include
#defineucharunsignedchar
#defineuintunsignedint
sbitSCLK=P2^0;
sbitIO=P2^1;
sbitCE=P2^2;
uchards1302_read_ram(ucharddress)
{
uchari,dat;
CE=0;
_nop_();
SCLK=0;
_nop_();
CE=1;
_nop_();
IO=0;//Write command
SCLK=0;
_nop_();
SCLK=1;
_nop_();
IO=address|0xc0;//Select address and read RAM
for(i=0;i< 8; i++) {
SCLK = 0;
_nop_();
SCLK = 1;
_nop_();
}
IO = 0; //Receive data
for (i = 0; i < 8; i++) {
dat >>=1;
if(IO)dat|=0x80;
SCLK=0;
_nop_();
SCLK=1;
_nop_();
}
CE=0;
returndat;
}
In the above code, the ds1302_read_ram function receives a parameter address, which is used to specify the RAM address to be read, and returns a byte representing the RAM data at that address.
The specific implementation process of this function is as follows:
Set CE to low level and delay for a period of time.
Set SCLK to low level and delay for a period of time.
Set CE to high level and delay for a period of time.
Set IO to low level to indicate a write command.
Set SCLK to low level and delay for a period of time.
Set SCLK to high level and delay for a period of time.
Set IO to address | 0xc0, that is, select the address and read RAM.
8 rising clock edges are performed in sequence, and the data bits are read on each rising edge.
Set CE low.
Return the read data.
It should be noted that when reading RAM data, the highest bit of the address (ie bit7) needs to be set to 1 to indicate that RAM is to be read. In addition, when reading the data bits, 8 rising clock edges are required in sequence, and each time the data is read, the data needs to be shifted to the right first, and then the new data is shifted to the left and the read data bits are up or down.
5. Overall code implementation
The following is the code implementation of a simple electronic clock using 51 microcontroller and DS1302 clock chip. The code contains basic functions such as initialization, clock reading, and RAM reading and writing of DS1302.
#include
#include"LCD1602.h"
#include"DS1302.h"
#defineucharunsignedchar
#defineuintunsignedint
sbitbeep=P3^6;//Define buzzer interface
voidmain()
{
ucharyear,month,day,hour,minute,second;//year, month, day, hour, minute, second
ucharstr_data[11],str_time[11]; //Used to store the date and time displayed on the LCD
LCD_Init();//Initialize LCD display
DS1302_Init();//Initialize DS1302 clock chip
//Initialize the time to 0:00:00 on February 21, 2023
DS1302_Write(0x8e,0);//Turn off write protection
DS1302_Write(0x80,0x23);//Year
DS1302_Write(0x82,0x02);//Month
DS1302_Write(0x84,0x21);//Date
DS1302_Write(0x86,0x00);//Clock
DS1302_Write(0x88,0x00);//minutes
DS1302_Write(0x8a,0x00); //Seconds
DS1302_Write(0x8e,0x80);//Enable write protection
while(1)
{
//Read the year, month, day, hour, minute and second in the DS1302 clock chip
year=DS1302_Read(0x80);
month=DS1302_Read(0x82);
day=DS1302_Read(0x84);
hour=DS1302_Read(0x86);
minute=DS1302_Read(0x88);
second=DS1302_Read(0x8a);
//Convert year, month, day, hour, minute and second into string
sprintf(str_data,"Data:20%02x-%02x-%02x",year,month,day);
sprintf(str_time,"Time:%02x:%02x:%02x",hour,minute,second);
//Display date and time on LCD
LCD_Write_String(0,0,str_data);
LCD_Write_String(0,1,str_time);
Delay_Ms(1000);//Delay 1 second
}
}
6. Display effect
Previous article:Information and source code instructions for making an alarm using a microcontroller
Next article:An article analyzing 51 microcontroller PWM dual servo control
Recommended ReadingLatest update time:2024-11-21 23:21
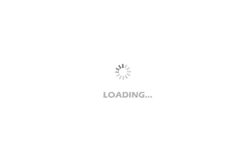
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
-
A Practical Tutorial on ASIC Design (Compiled by Yu Xiqing)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Europe's three largest chip giants re-examine their supply chains
- Breaking through the intelligent competition, Changan Automobile opens the "God's perspective"
- The world's first fully digital chassis, looking forward to the debut of the U7 PHEV and EV versions
- Design of automotive LIN communication simulator based on Renesas MCU
- When will solid-state batteries become popular?
- Adding solid-state batteries, CATL wants to continue to be the "King of Ning"
- The agency predicts that my country's public electric vehicle charging piles will reach 3.6 million this year, accounting for nearly 70% of the world
- U.S. senators urge NHTSA to issue new vehicle safety rules
- Giants step up investment, accelerating the application of solid-state batteries
- Guangzhou Auto Show: End-to-end competition accelerates, autonomous driving fully impacts luxury...
- Design and Application of GPRS and GPS in Automobile Information Service System
- Misiqi's project cooperation based on ESP32 development board IOT Bluetooth WIFI
- Is this a circuit that can prevent the MCU from hanging?
- When southern girls start soldering irons to do projects, what do science and engineering men need? - Arduino, hardware, project tutorials
- The principle and method of generating multiple PWM waveforms using one timer
- The impact of 5G on positioning technology
- Why is the output waveform of Quartus 13.0 always at a low level?
- MicoPython can now use extended SDRAM
- A comprehensive look at the entire family of MSP430 microcontrollers
- I2C Bus Specification