Experiment name: DS1302 clock experiment
wiring instructions:
Experimental phenomenon: After downloading the program, the electronic clock hours, minutes and seconds are displayed on the digital tube in the format of "XX-XX-XX"
Notes:
************* *************************************************** ************************/
#include "public.h"
#include "smg.h"
#include "ds1302.h"
/****************************************************** ******************************
* Function name: main
* Function function: main function
* Input: None
* Output: None
* *************************************************** ******************************/
void main()
{
u8 time_buf[8];
ds1302_init();//Initialize DS1302
while(1)
{
ds1302_read_time();
time_buf[0]=gsmg_code[gDS1302_TIME[2]/16];
time_buf[1]=gsmg_code[gDS1302_TIME[2]&0x0f];
time_buf[2]=0x40;
time_buf[3]= time_buf [
7 ] =gsmg_code[gDS1302_TIME[0]&0x0f]; smg_display(time_buf,1); } }
#include "ds1302.h"
#include "intrins.h"
//---DS1302 address commands for writing and reading hours, minutes and seconds---//
//---seconds, minutes, hours, days, months, and anniversary lowest read and write bits;-------//
u8 gREAD_RTC_ADDR[ 7] = {0x81, 0x83, 0x85, 0x87, 0x89, 0x8b, 0x8d};
u8 gWRITE_RTC_ADDR[7] = {0x80, 0x82, 0x84, 0x86, 0x88, 0x8a, 0x8c};
//---DS1302 clock initialized Thursday, May 20, 2021 at 13:51:47. ---//
//---The storage sequence is seconds, minutes, days, months and anniversaries, and the storage format is BCD code---//
u8 gDS1302_TIME[7] = {0x47, 0x51, 0x13, 0x20, 0x04, 0x05, 0x21};
/****************************************************** ******************************
* Function name: ds1302_write_byte
* Function function: DS1302 writes single byte
* Input: addr: address /command
dat:data
* output: None
****************************************** ******************************************/
void ds1302_write_byte(u8 addr,u8 dat)
{
u8 i=0;
DS1302_RST=0;
_nop_();
DS1302_CLK=0;//CLK low level
_nop_();
DS1302_RST=1;//RST changes from low to high
_nop_();
for(i=0;i<8;i++)//Loop 8 times, write 1 bit each time, write the low bit first and then the high bit
{
DS1302_IO=addr&0x01;
addr>>=1;
DS1302_CLK=1
; _nop_();
DS1302_CLK =0;//CLK generates a rising edge from low to high, thereby writing data_nop_
();
}
for(i=0;i<8;i++)//Loop 8 times, write 1 bit each time, write first Write the low bit then the high bit
{
DS1302_IO=dat&0x01;
dat>>=1;
DS1302_CLK=1;
_nop_();
DS1302_CLK=0;
_nop_();
}
DS1302_RST=0;//RST pull low
_nop_();
}
/****************************************************** ******************************
* Function name: ds1302_read_byte
* Function function: DS1302 reads single byte
* Input: addr: address /Command
* Output: Read data
****************************************** ****************************************/
u8 ds1302_read_byte(u8 addr)
{
u8 i=0 ;
u8 temp=0;
u8 value=0;
DS1302_RST=0;
_nop_();
DS1302_CLK=0;//CLK low level
_nop_();
DS1302_RST=1;//RST changes from low to high
_nop_();
for(i=0;i<8;i++) //Loop 8 times, write 1 bit each time, write the low bit first and then the high bit
{
DS1302_IO=addr&0x01;
addr>>=1;
DS1302_CLK=1;
_nop_();
DS1302_CLK=0; //CLK generates one from low to high Rising edge, thus writing
data_nop_();
}
for(i=0;i<8;i++)//Loop 8 times, read 1 bit each time, read the low bit first and then the high bit
{
temp=DS1302_IO;
value=( temp<<7)|(value>>1);//First shift value to the right by 1 bit, then shift temp to the left by 7 bits, and finally perform the OR operation
DS1302_CLK=1;
_nop_();
DS1302_CLK=0
; _nop_();
}
DS1302_RST=0;//RST pull low_nop_
();
DS1302_CLK=1;//For the real thing, the P3.4 port does not have an external pull-up resistor, so the code needs to be added here to make the data port have a rising edge pulse.
_nop_();
DS1302_IO = 0;
_nop_();
DS1302_IO = 1;
_nop_();
return value;
}
/****************************************************** ****************************
* Function name: ds1302_init
* Function function: DS1302 initialization time
* Input: None
* Output: None
*************************************************** *****************************/
void ds1302_init(void)
{
u8 i=0;
ds1302_write_byte(0x8E,0X00);
for( i=0;i<7;i++)
{
ds1302_write_byte(gWRITE_RTC_ADDR[i],gDS1302_TIME[i]);
}
ds1302_write_byte(0x8E,0X80);
}
/****************************************************** ****************************
* Function name: ds1302_read_time
* Function function: DS1302 read time
* Input: None
* Output: none
************************************************* ******************************/
void ds1302_read_time(void)
{
u8 i=0;
for(i=0;i< 7;i++)
{
gDS1302_TIME[i]=ds1302_read_byte(gREAD_RTC_ADDR[i]);
}
}
#include "smg.h"
//The common cathode digital tube displays the segment code data of 0~F
u8 gsmg_code[17]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,
0x7f,0x6f,0x77,0x7c,0x39,0x5e,0x79 ,0x71};
/****************************************************** ****************************
* Function name: smg_display
* Function function: Dynamic digital tube display
* Input: dat: to be displayed Data
pos: starting from the left position, range 1-8
* Output: None
****************************** *************************************************** */
void smg_display(u8 dat[],u8 pos)
{
u8 i=0;
u8 pos_temp=pos-1;
for(i=pos_temp;i<8;i++)
{
switch(i)//bit selection
{
case 0: LSC=1;LSB=1;LSA=1;break;
case 1: LSC=1;LSB=1; LSA=0;break;
case 2: LSC=1;LSB=0;LSA=1;break;
case 3: LSC=1;LSB=0;LSA=0;break;
case 4: LSC=0;LSB=1 ;LSA=1;break;
case 5: LSC=0;LSB=1;LSA=0;break;
case 6: LSC=0;LSB=0;LSA=1;break;
case 7: LSC=0;LSB= 0;LSA=0;break;
}
SMG_A_DP_PORT=dat[i-pos_temp];//Transmit segment selection data
delay_10us(100);//Delay for a period of time, waiting for the display to stabilize
SMG_A_DP_PORT=0x00;//Mute the sound
}
}
#include "public.h"
/****************************************************** ****************************
* Function name: delay_10us
* Function function: Delay function, when ten_us=1, the delay is approximately 10us
* Input: ten_us
* Output: None
**************************************** ***************************************/
void delay_10us(u16 ten_us)
{
while(ten_us --);
}
/****************************************************** ****************************
* Function name: delay_ms
* Function function: ms delay function, when ms=1, approximately Delay 1ms
* Input: ms: ms delay time
* Output: None
********************************** *********************************************/
void delay_ms(u16 ms)
{
u16 i,j;
for(i=ms;i>0;i--)
for(j=110;j>0;j--);
}
Previous article:51 microcontroller learning: infrared remote control experiment
Next article:51 microcontroller learning: DS18B20 temperature sensor experiment
Recommended ReadingLatest update time:2024-11-16 19:54
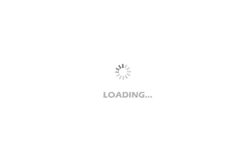
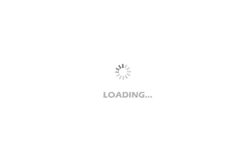
- Popular Resources
- Popular amplifiers
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
Teach you to learn 51 single chip microcomputer-C language version (Second Edition) (Song Xuefeng)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- If the clamp meter (multimeter) does not measure but there are still numbers, is it a malfunction?
- RK3399 open source motherboard hardware and software information released - free download
- EEWORLD University Hall - Digital Oscilloscope Operation Digital Oscilloscope Operation_National Taiwan Normal University_Teacher Zhang Guowei
- [ESP32-Audio-Kit Audio Development Board Review] Part 1: Building esp-idf and esp-adf development environments based on vs code
- Live broadcast has ended | Microchip's latest SAM and PIC32 microcontroller software development platform - MPLAB Harmony V3
- [GD32E503 Review]——07.LCD Part 2: Drawing Custom Patterns
- Xiaozhi Science Popularization丨Why do instruments generally have set values and readback values?
- SensorTile.box emulates STEVAL-MKI109V3
- TI.com live broadcast: Interpretation of popular smart electronic locks, visual doorbells, smart sensors and network camera solutions
- 【TI Recommended Course】#What can universal fast charging bring?#