Preface
I recently got a STM8L051/101F3 development board and planned to play around with it. After I found that the IO and timer were all OK, I started to configure the serial UART and found that the receive interrupt could not be entered, but the send interrupt was OK. Then I started a two-day troubleshooting, and checked almost all possible problem points.
STM8L051/101F3 Development Board
Code
The MCU here is STM8L051F3, and the STM8 library is used for development. The code is as follows for reference only:
main.c
#include "stm8l15x.h"
void Clock_Config(void)
{
CLK_SYSCLKDivConfig(CLK_SYSCLKDiv_1); //System clock divider
CLK_SYSCLKSourceSwitchCmd(ENABLE); //Turn on the system clock switch
CLK_SYSCLKSourceConfig(CLK_SYSCLKSource_HSI); //Set the system clock source to the internal high-speed clock 16MHz
while(CLK_GetSYSCLKSource() != CLK_SYSCLKSource_HSI); //Wait for clock switching
}
void GPIO_Config(void)
{
//USART GPIO
GPIO_Init(GPIOC, GPIO_Pin_6, GPIO_Mode_In_PU_No_IT);
GPIO_Init(GPIOC, GPIO_Pin_5, GPIO_Mode_Out_PP_High_Fast);
SYSCFG_REMAPPinConfig(REMAP_Pin_USART1TxRxPortC, ENABLE);
}
void Usart_Config(void)
{
CLK_PeripheralClockConfig(CLK_Peripheral_USART1, ENABLE);
USART_Init(USART1, (uint32)9600, USART_WordLength_8b, USART_StopBits_1, USART_Parity_No, (USART_Mode_TypeDef)(USART_Mode_Tx | USART_Mode_Rx));
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_ITConfig(USART1, USART_IT_TC, ENABLE);
USART_Cmd(USART1, ENABLE);
}
void main( void )
{
Clock_Config();
GPIO_Config();
Usart_Config();
enableInterrupts();
USART_SendData8(USART1, 0x55);
while(1)
{
}
}
void Usart1_RX_Callback(uint8_t data)
{
USART_SendData8(USART1, data);
}
stm8l15x_it.c
extern void Usart1_RX_Callback(uint8_t data);
/**
* @brief USART1 RX / Timer5 Capture/Compare Interrupt routine.
* @param None
* @retval None
*/
INTERRUPT_HANDLER(USART1_RX_TIM5_CC_IRQHandler,28)
{
/* In order to detect unexpected events during development,
it is recommended to set a breakpoint on the following instruction.
*/
if(USART_GetITStatus(USART1, USART_IT_RXNE))
{
// USART_ClearITPendingBit(USART1, USART_IT_RXNE);
Usart1_RX_Callback(USART_ReceiveData8(USART1));
}
}
Troubleshooting
First of all, I can be sure that there is no problem with the code. I have checked the registers during simulation with the specification sheet, and all the configurations are correct.
Then I accidentally discovered that the development board can burn programs via the USB serial port, and the burning port used is exactly the serial port IO I use. Then I wondered if there was a conflict between the onboard USB to serial port chip and the external USB to serial port?
Finally I unplugged the onboard RXD jumper, and the serial port receive interrupt came into play...
Remove the RXD jumper
Summarize
If the development board has a USB to serial port, do not insert a USB to serial port module (this is nonsense), otherwise it may affect the serial port transmission and reception, resulting in an inability to receive serial port data.
Previous article:STM8 comes with BootLoader serial port burning program
Next article:STM8L USART+DMA configuration, using DMA to complete serial port transmission and reception
Recommended ReadingLatest update time:2024-11-22 22:14
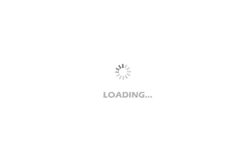
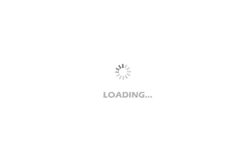
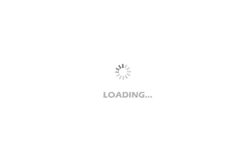
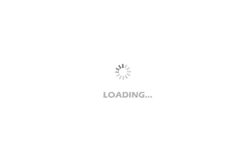
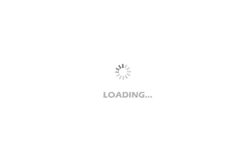
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
-
uCOS-III Kernel Implementation and Application Development Practical Guide - Based on STM32 (Wildfire)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Will directly connecting the solder joint to the copper pad cause the heat to transfer too quickly and make soldering difficult?
- Several questions about MmwaveStudio
- Circuits and electronics problem solving guide.
- [RVB2601 Creative Application Development] Establish a development environment and run a flashing DEMO
- I would like to ask, in the following picture, what is the best communication method between ZigBee?
- How to Improve Electronic Product Heat Dissipation through Good PCB Design
- The output waveform of the Wien oscillator circuit is abnormal
- Resigned
- Designing an efficient real-time audio system using VisualAudio
- Burning port, are these two resistors necessary? Data pull-up, clock pull-down