* The frame memory is completely consistent with the viewpoint,
* The image data format is as follows (at 8BPP, the data in the frame buffer is the index value in the palette):
* |----PAGEWIDTH----|
* y/x 0 1 2 639
* 0 idx idx idx ... idx
* 1 idx idx idx ... idx
* 1. LCDSADDR1:
* Set LCDBANK, LCDBASEU
* 2. LCDSADDR2:
* Set LCDBASEL: frame buffer end address A[21:1]
* 3. LCDSADDR3:
* OFFSIZE is equal to 0, PAGEWIDTH is equal to (640/2)
*/
LCDSADDR1 = ((LCDFRAMEBUFFER>>22)<<21) | LOWER21BITS(LCDFRAMEBUFFER>>1);
LCDSADDR2 = LOWER21BITS((LCDFRAMEBUFFER+
(LINEVAL_TFT_640480+1)*(HOZVAL_TFT_640480+1)*1)>>1);
LCDSADDR3 = (0<<11) | (LCD_XSIZE_TFT_640480/2);
/* Disable temporary palette register */
TPAL = 0;
fb_base_addr = LCDFRAMEBUFFER;
bpp = 8;
xsize = 640;
ysize = 480;
break;
case MODE_TFT_16BIT_640480:
/*
* Set the LCD controller's control registers LCDCON1~5
* 1. LCDCON1:
* Set the frequency of VCLK: VCLK(Hz) = HCLK/[(CLKVAL+1)x2]
* Select LCD type: TFT LCD
* Set display mode: 16BPP
* Disable LCD signal output first
* 2. LCDCON2/3/4:
* Set the time parameters of the control signal
* Set the resolution, i.e. the number of rows and columns
* Now, the display frequency can be calculated according to the formula:
* When HCLK=100MHz,
* Frame Rate = 1/[{(VSPW+1)+(VBPD+1)+(LIINEVAL+1)+(VFPD+1)}x
* {(HSPW+1)+(HBPD+1)+(HFPD+1)+(HOZVAL+1)}x
* {2x(CLKVAL+1)/(HCLK)}]
* = 60Hz
* 3. LCDCON5:
* Data format when setting display mode to 16BPP: 5:6:5
* Set the polarity of HSYNC and VSYNC pulses (this needs to refer to the specific LCD interface signal): Invert
* Halfword (2-byte) swap enable
*/
LCDCON1 = (CLKVAL_TFT_640480<<8) | (LCDTYPE_TFT<<5) |
(BPPMODE_16BPP<<1) | (ENVID_DISABLE<<0);
LCDCON2 = (VBPD_640480<<24) | (LINEVAL_TFT_640480<<14) |
(VFPD_640480<<6) | (VSPW_640480);
LCDCON3 = (HBPD_640480<<19) | (HOZVAL_TFT_640480<<8) | (HFPD_640480);
LCDCON4 = HSPW_640480;
LCDCON5 = (FORMAT8BPP_565<<11) | (HSYNC_INV<<9) | (VSYNC_INV<<8) |
(HWSWP<<1);
/*
* Set the LCD controller address register LCDSADDR1~3
* The frame memory is completely consistent with the viewpoint,
* The image data format is as follows:
* |----PAGEWIDTH----|
* y/x 0 1 2 639
* 0 rgb rgb rgb ... rgb
* 1 rgb rgb rgb ... rgb
* 1. LCDSADDR1:
* Set LCDBANK, LCDBASEU
* 2. LCDSADDR2:
* Set LCDBASEL: frame buffer end address A[21:1]
* 3. LCDSADDR3:
* OFFSIZE is equal to 0, PAGEWIDTH is equal to (640*2/2)
*/
LCDSADDR1 = ((LCDFRAMEBUFFER>>22)<<21) | LOWER21BITS(LCDFRAMEBUFFER>>1);
LCDSADDR2 = LOWER21BITS((LCDFRAMEBUFFER+
(LINEVAL_TFT_640480+1)*(HOZVAL_TFT_640480+1)*2)>>1);
LCDSADDR3 = (0<<11) | (LCD_XSIZE_TFT_640480*2/2);
/* Disable temporary palette register */
TPAL = 0;
fb_base_addr = LCDFRAMEBUFFER;
bpp = 16;
xsize = 640;
ysize = 480;
break;
default:
break;
}
}
/*
* Set the color palette
*/
void Lcd_Palette8Bit_Init(void)
{
int i;
volatile unsigned int *palette;
LCDCON1 &= ~0x01; // stop lcd controller
LCDCON5 |= (FORMAT8BPP_565<<11); // Set the data format in the palette to 5:6:5
palette = (volatile unsigned int *)PALETTE;
for (i = 0; i < 256; i++)
*palette++ = DEMO256pal[i];
LCDCON1 |= 0x01; // re-enable lcd controller
}
/*
* Change the palette to a single color
* Input parameters:
* color: color value, format is 0xRRGGBB
*/
void ChangePalette(UINT32 color)
{
int i;
unsigned char red, green, blue;
UINT32 *palette;
red = (color >> 19) & 0x1f;
green = (color >> 10) & 0x3f;
blue = (color >> 3) & 0x1f;
color = (red << 11) | (green << 5) | blue; // 格式5:6:5
palette=(UINT32 *)PALETTE;
LCDCON1 &= ~0x01; // stop lcd controller
for (i = 0; i < 256; i++)
{
// while (((LCDCON5>>15) & 0x3) == 2); // Wait until VSTATUS is not "valid"
*palette++ = color;
}
LCDCON1 |= 0x01; // re-enable lcd controller
}
/*
* Set whether to output LCD power switch signal LCD_PWREN
* Input parameters:
* invpwren: 0 - Normal polarity when LCD_PWREN is valid
* 1 - Invert polarity when LCD_PWREN is active
* pwren: 0 - LCD_PWREN output is valid
* 1 - LCD_PWREN output is disabled
*/
void Lcd_PowerEnable(int invpwren, int pwren)
{
GPGCON = (GPGCON & (~(3<<8))) | (3<<8); // GPG4用作LCD_PWREN
GPGUP = (GPGUP & (~(1<<4))) | (1<<4); // Disable internal pull-up
LCDCON5 = (LCDCON5 & (~(1<<5))) | (invpwren<<5); // Set LCD_PWREN polarity: normal/inverted
LCDCON5 = (LCDCON5 & (~(1<<3))) | (pwren<<3); // Set whether to output LCD_PWREN
}
/*
* Set whether the LCD controller outputs a signal
* Input parameters:
* onoff:
* 0 : Disable
* 1 : Open
*/
void Lcd_EnvidOnOff(int onoff)
{
if (onoff == 1)
{
LCDCON1 |= 1; // ENVID ON
}
else
{
LCDCON1 &= 0x3fffe; // ENVID Off
}
}
/*
* Output monochrome images using temporary palette registers
* Input parameters:
* color: color value, format is 0xRRGGBB
*/
void ClearScrWithTmpPlt(UINT32 color)
{
TPAL = (1<<24)|((color & 0xffffff)<<0);
}
/*
* Stop using temporary palette registers
*/
void DisableTmpPlt(void)
{
TPAL = 0;
}
————————————————
Copyright Statement: This article is an original article by CSDN blogger "Cawen_Cao" and follows the CC 4.0 BY-SA copyright agreement. Please attach the original source link and this statement when reprinting.
Original link: https://blog.csdn.net/Cowena/article/details/48207173
Previous article:TQ2440 Study Notes —— 21. Interrupt Architecture
Next article:TQ2440 Study Notes—— 31. Transplanting U-Boot [Source code analysis of the second stage of U-Boot's startup process]
Recommended ReadingLatest update time:2024-11-16 22:34
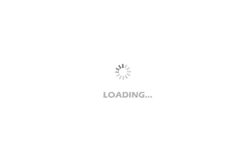
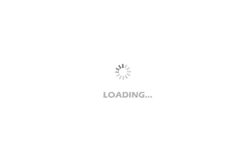
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- ABB six-axis robot and Siemens 1500PLC user manual
- [Ateli Development Board AT32F421 Review] 7. Kuga registers jointly light up OLED
- Design and FPGA implementation of digital on-screen display control core.pdf
- EL817C Optocoupler Transmission Ratio Problem
- Keysight Technologies N9020A 3.6G spectrum analyzer special sale: 8500/unit
- STM32+photosensitive sensor+serial port receiving light intensity source program is successfully produced
- How does the uart.write function output three bytes of 0XFF 0XFF 0XFF (HEX data, not string data)?
- Electric vehicles use silicon carbide power devices to successfully move out of the laboratory
- Chinese programmers VS American programmers, so vivid...
- Please tell me the setting function of deep sleep