LCD Controller
programming:
1. Turn on the backlight
2. Timing settings
3. Write data in FrameBuffer (different pixels have different formats)
/*
* FILE: lcddrv.c
* Provides low-level functions for operating LCD controllers, palettes, etc.
*/
#include #include "s3c24xx.h" #include "lcddrv.h" #define GPB0_tout0 (2<<(0*2)) #define GPB0_out (1<<(0*2)) #define GPB1_out (1<<(1*2)) #define GPB0_MSK (3<<(0*2)) #define GPB1_MSK (3<<(1*2)) unsigned int fb_base_addr; unsigned int bpp; unsigned int xsize; unsigned int ysize; static const unsigned short DEMO256pal[]={ 0x0b5e,0xce9a,0xffd9,0x9d99,0xb63a,0xae7c,0xdd71,0x6c57,0xfd4d,0x00ae,0x9c4d,0xb5f8,0xad96,0x0131,0x0176,0xefff,0xcedd,0x9556,0xe4bf,0x00b6,0x22b7,0x002b,0x89de,0x002c,0x57df,0xab5f,0x3031,0x14bf,0x797e,0x5391,0x93ab,0x7239,0x7453,0xafdf,0x71b9,0x8c92,0x014d,0x302e,0x5175,0x0029,0x0969,0x004e,0x2a6d,0x0021,0x3155,0x4b6e,0xd677,0xf6b6,0x9b5f,0x4bb5,0xffd5,0x0027,0xdfdf,0x74d8,0x1256,0x6bcd,0x9b08,0x2ab2,0xbd72,0x84b5,0xfe52,0xd4ad,0x00ad,0xfffc,0x422b,0x73b0,0x0024,0x5246,0x8e5e,0x28b3,0x0050,0x3b52,0x2a4a,0x3a74,0x8559,0x3356,0x1251,0x9abf,0x4034,0x40b1, 0x8cb9,0x00b3,0x5c55,0xdf3d,0x61b7,0x1f5f,0x00d9,0x4c59,0x0926,0xac3f,0x925f,0x85bc,0x29d2,0xc73f,0xef5c,0xcb9f,0x827b,0x5279,0x4af5,0x01b9,0x4290,0xf718,0x126d,0x21a6,0x515e,0xefbd,0xd75e,0x42ab,0x00aa,0x10b3,0x7349,0x63b5,0x61a3,0xaadf,0xcb27,0x87df,0x6359,0xc7df,0x4876,0xb5bc,0x4114,0xfe2e,0xef5e,0x65be,0x43b9,0xe5df,0x21c9,0x7d16,0x6abb,0x5c11,0x49f7,0xbc0b,0x9e1a,0x3b0f,0x202b,0xff12,0x821b,0x842f,0xbccf,0xdefb,0x8a3e,0x68fa,0xa4f1,0x38ae,0x28b7,0x21ad,0x31d7,0x0073,0x182b,0x1831,0x3415,0xbdf6,0x2dbf,0x0a5d,0xc73d,0x182c,0x293e,0x7b3d,0x643d,0x3cbd, 0x92dd,0x09d4,0x1029,0x7cdd,0x6239,0x182e,0x5aea,0x11eb,0x8abc,0x7bfa,0x00a7,0x2153,0x1853,0x1318,0x0109,0x54fa,0x72a7,0x89e3,0x01cf,0x3a07,0x7b17,0x1a14,0x2150,0x23dc,0x4142,0x1b33,0x00a4,0xf6df,0x08fc,0x18ae,0x3a7e,0x18d1,0xa51c,0xff5a,0x1a0f,0x28fa,0xdfbe,0x82de,0x60d7,0x1027,0x48fa,0x5150,0x6213,0x89d6,0x110d,0x9bbb,0xbedd,0x28e1,0x1925,0xf449,0xaa79,0xd5f4,0x693c,0x110a,0x2889,0x08a2,0x923d,0x10a6,0xd9bc,0x5b2e,0x32ec,0xcf7f,0x1025,0x2148,0x74b4,0x6d59,0x9d14,0x0132,0x00f0,0x56bf,0x00f1,0xffff,0x0173,0x0133,0x00b0,0x00b1,0xf7ff,0x08b1,0xfffe,0x08b0, 0x0171,0xf7bf,0x10f3,0xf7fe,0x08ef,0x1192,0xefbe,0x1131,0x2177,0xff9f,0x1116,0xffbc,0x5914,0x22ef,0xb285,0xa6df, }; /* * Initialize the pins for the LCD */ void Lcd_Port_Init(void) { GPCUP = 0xffffffff; // Disable internal pull-up GPCCON = 0xaaaaaaaa; // GPIO pins for VD[7:0], LCDVF[2:0], VM, VFRAME, VLINE, VCLK, LEND GPDUP = 0xffffffff; // Disable internal pull-up GPDCON = 0xaaaaaaaa; // GPIO pins for VD[23:8] GPBCON &= ~(GPB0_MSK); // Power enable pin GPBCON |= GPB0_out; GPBDAT &= ~(1<<0); // Power off printf("Initializing GPIO ports..........n"); } /* * Initialize LCD controller * Input parameters: * type: display mode * MODE_TFT_8BIT_240320 : 240*320 8bpp TFT LCD * MODE_TFT_16BIT_240320 : 240*320 16bpp TFT LCD * MODE_TFT_8BIT_640480 : 640*480 8bpp TFT LCD * MODE_TFT_16BIT_640480 : 640*480 16bpp TFT LCD */ void Tft_Lcd_Init(int type) { switch(type) { case MODE_TFT_8BIT_480272: /* * Set the LCD controller's control registers LCDCON1~5 * 1. LCDCON1: * Set the frequency of VCLK: VCLK(Hz) = HCLK/[(CLKVAL+1)x2] * Select LCD type: TFT LCD * Set display mode: 8BPP * Disable LCD signal output first * 2. LCDCON2/3/4: * Set the time parameters of the control signal * Set the resolution, i.e. the number of rows and columns * Now, the display frequency can be calculated according to the formula: * When HCLK=100MHz, * Frame Rate = 1/[{(VSPW+1)+(VBPD+1)+(LIINEVAL+1)+(VFPD+1)}x * {(HSPW+1)+(HBPD+1)+(HFPD+1)+(HOZVAL+1)}x * {2x(CLKVAL+1)/(HCLK)}] * * 3. LCDCON5: * When the display mode is set to 8BPP, the data format in the palette is: 5:6:5 * Set the polarity of HSYNC and VSYNC pulses (this needs to refer to the specific LCD interface signal): Invert * Byte swap enable */ LCDCON1 = (CLKVAL_TFT_480272<<8) | (LCDTYPE_TFT<<5) | (BPPMODE_8BPP<<1) | (ENVID_DISABLE<<0); LCDCON2 = (VBPD_480272<<24) | (LINEVAL_TFT_480272<<14) | (VFPD_480272<<6) | (VSPW_480272); LCDCON3 = (HBPD_480272<<19) | (HOZVAL_TFT_480272<<8) | (HFPD_480272); LCDCON4 = HSPW_480272; LCDCON5 = (FORMAT8BPP_565<<11) | (HSYNC_INV<<9) | (VSYNC_INV<<8) | (BSWP<<1); /* * Set the LCD controller address register LCDSADDR1~3 * The frame memory is completely consistent with the viewpoint, * The image data format is as follows (at 8BPP, the data in the frame buffer is the index value in the palette): * |----PAGEWIDTH----| * y/x 0 1 2 479 * 0 idx idx idx ... idx * 1 idx idx idx ... idx * 1. LCDSADDR1: * Set LCDBANK, LCDBASEU * 2. LCDSADDR2: * Set LCDBASEL: frame buffer end address A[21:1] * 3. LCDSADDR3: * OFFSIZE is equal to 0, PAGEWIDTH is equal to (480/2) */ LCDSADDR1 = ((LCDFRAMEBUFFER>>22)<<21) | LOWER21BITS(LCDFRAMEBUFFER>>1); LCDSADDR2 = LOWER21BITS((LCDFRAMEBUFFER+ (LINEVAL_TFT_480272+1)*(HOZVAL_TFT_480272+1)*1)>>1); LCDSADDR3 = (0<<11) | (LCD_XSIZE_TFT_480272/2); /* Disable temporary palette register */ TPAL = 0; fb_base_addr = LCDFRAMEBUFFER; bpp = 8; xsize = 480; ysize = 272; break; case MODE_TFT_16BIT_480272: /* * Set the LCD controller's control registers LCDCON1~5 * 1. LCDCON1: * Set the frequency of VCLK: VCLK(Hz) = HCLK/[(CLKVAL+1)x2] * Select LCD type: TFT LCD * Set display mode: 16BPP * Disable LCD signal output first * 2. LCDCON2/3/4: * Set the time parameters of the control signal * Set the resolution, i.e. the number of rows and columns * Now, the display frequency can be calculated according to the formula: * When HCLK=100MHz, * Frame Rate = 1/[{(VSPW+1)+(VBPD+1)+(LIINEVAL+1)+(VFPD+1)}x * {(HSPW+1)+(HBPD+1)+(HFPD+1)+(HOZVAL+1)}x * {2x(CLKVAL+1)/(HCLK)}] * = 60Hz * 3. LCDCON5: * Data format when setting display mode to 16BPP: 5:6:5 * Set the polarity of HSYNC and VSYNC pulses (this needs to refer to the specific LCD interface signal): Invert * Halfword (2-byte) swap enable */ LCDCON1 = (CLKVAL_TFT_480272<<8) | (LCDTYPE_TFT<<5) | (BPPMODE_16BPP<<1) | (ENVID_DISABLE<<0); LCDCON2 = (VBPD_480272<<24) | (LINEVAL_TFT_480272<<14) | (VFPD_480272<<6) | (VSPW_480272); LCDCON3 = (HBPD_480272<<19) | (HOZVAL_TFT_480272<<8) | (HFPD_480272); LCDCON4 = HSPW_480272; LCDCON5 = (FORMAT8BPP_565<<11) | (HSYNC_INV<<9) | (VSYNC_INV<<8) | (HWSWP<<1); /* * Set the LCD controller address register LCDSADDR1~3 * The frame memory is completely consistent with the viewpoint, * The image data format is as follows: * |----PAGEWIDTH----| * y/x 0 1 2 479 * 0 rgb rgb rgb ... rgb * 1 rgb rgb rgb ... rgb * 1. LCDSADDR1: * Set LCDBANK, LCDBASEU * 2. LCDSADDR2: * Set LCDBASEL: frame buffer end address A[21:1] * 3. LCDSADDR3: * OFFSIZE is equal to 0, PAGEWIDTH is equal to (480*2/2) */ LCDSADDR1 = ((LCDFRAMEBUFFER>>22)<<21) | LOWER21BITS(LCDFRAMEBUFFER>>1); LCDSADDR2 = LOWER21BITS((LCDFRAMEBUFFER+ (LINEVAL_TFT_480272+1)*(HOZVAL_TFT_480272+1)*2)>>1); LCDSADDR3 = (0<<11) | (LCD_XSIZE_TFT_480272*2/2); /* Disable temporary palette register */ TPAL = 0; fb_base_addr = LCDFRAMEBUFFER; bpp = 16; xsize = 480; ysize = 272; break; case MODE_TFT_8BIT_640480: /* * Set the LCD controller's control registers LCDCON1~5 * 1. LCDCON1: * Set the frequency of VCLK: VCLK(Hz) = HCLK/[(CLKVAL+1)x2] * Select LCD type: TFT LCD * Set display mode: 8BPP * Disable LCD signal output first * 2. LCDCON2/3/4: * Set the time parameters of the control signal * Set the resolution, i.e. the number of rows and columns * Now, the display frequency can be calculated according to the formula: * When HCLK=100MHz, * Frame Rate = 1/[{(VSPW+1)+(VBPD+1)+(LIINEVAL+1)+(VFPD+1)}x * {(HSPW+1)+(HBPD+1)+(HFPD+1)+(HOZVAL+1)}x * {2x(CLKVAL+1)/(HCLK)}] * = 60Hz * 3. LCDCON5: * When the display mode is set to 8BPP, the data format in the palette is: 5:6:5 * Set the polarity of HSYNC and VSYNC pulses (this needs to refer to the specific LCD interface signal): Invert * Byte swap enable */ LCDCON1 = (CLKVAL_TFT_640480<<8) | (LCDTYPE_TFT<<5) | (BPPMODE_8BPP<<1) | (ENVID_DISABLE<<0); LCDCON2 = (VBPD_640480<<24) | (LINEVAL_TFT_640480<<14) | (VFPD_640480<<6) | (VSPW_640480); LCDCON3 = (HBPD_640480<<19) | (HOZVAL_TFT_640480<<8) | (HFPD_640480); LCDCON4 = HSPW_640480; LCDCON5 = (FORMAT8BPP_565<<11) | (HSYNC_INV<<9) | (VSYNC_INV<<8) | (BSWP<<1); /* * Set the LCD controller address register LCDSADDR1~3
Previous article:TQ2440 Study Notes —— 21. Interrupt Architecture
Next article:TQ2440 Study Notes—— 31. Transplanting U-Boot [Source code analysis of the second stage of U-Boot's startup process]
Recommended ReadingLatest update time:2024-11-16 19:58
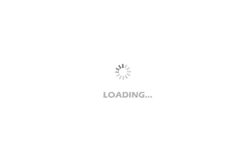
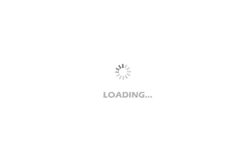
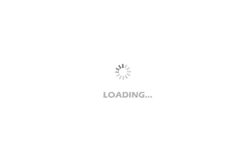
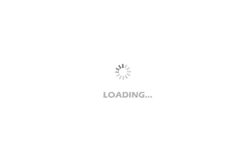
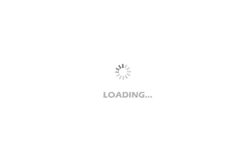
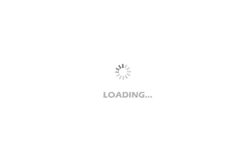
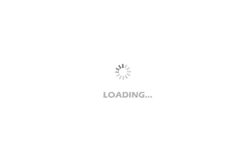
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Reshaping the engineer experience: Tektronix invites you to attend the global launch of the new 2-series MSO at 2 pm on June 8 (today)!
- How to draw multiple boards with the same functional schematics on one PCB
- Quickly build the Hongmeng development environment for BearPi HM Nano
- Variable memory depth and segmented memory of LOTO oscilloscope
- [SAMR21 new gameplay] 21. Touchio
- STM32 learning the ninth post can not turn off the PWM pin
- I have a SOT23-6 package with a silk screen model number.
- 【CH579M-R1】+PWM breathing light and serial communication experiment
- The problem of calibrating speed
- Measuring the flow control valve signal of the auto repair actuator with an automotive oscilloscope