17. Why can't several output frequencies be changed when the microcontroller interrupt changes the frequency? What is the procedure? 21. When is the AD interrupt of the PIC microcontroller turned on? How should we understand the AD interrupt?
#include
#define uchar unsigned char
#define uint unsigned int
uchar T,t1;
unsigned char data table[5] = {486,236,151,111,86} ;
sbit CLK=P2^3 ;
sbit EN=P2^0 ;
void init();
void main()
{
init();
}
void init()
{
EN=1;
T=0;
TMOD=0x01;
EA=1;
TR0=1;
ET0=1;
t1=table[T];
TH0=(65536-t1)/256;
TL0=(65536-t1)%256;
}
void timer0() interrupt 1
{
TMOD=0x01;
EA=1;
TR0=1;
ET0=1;
t1=table[T];
TH0=(65536-t1)/256;
TL0=(65536-t1)%256;
CLK=~CLK;
}
Answer:
You only assign 0 value to T, how can the frequency change? You just need to add a T assignment statement in the main function, for example: while(T){T--;delay1s();}
18. How to write a microcontroller interrupt program?
Answer:
Standard form:
void function name (void) interrupt n using m
{function body statement}
n ---- interrupt number
m----- working register group number to be used
19. I want to know how the microcontroller buzzer music program interrupt responds? How to get to the interrupt program from the main program? What are the specific steps? Thank you!
#include
sbit speaker = P1^5;
unsigned char timer0h, timer0l, time;
//--------------------------------------
//The single-chip crystal oscillator adopts 11.0592MHz
// Frequency-half-cycle data table high eight bits This software saves a total of 28 frequency data of four octaves
code unsigned char FREQH[] = {
0xF2, 0xF3, 0xF5, 0xF6, 0xF7, 0xF8, //Bass 1234567
0xF9, 0xF9, 0xFA, 0xFA, 0xFB, 0xFB, 0xFC, 0xFC,//1,2,3,4,5,6,7,i
0xFC, 0xFD, 0xFD, 0xFD, 0xFD, 0xFE, //Treble 234567
0xFE, 0xFE, 0xFE, 0xFE, 0xFE, 0xFE, 0xFF}; //Super high pitch 1234567
// Frequency-half-cycle data table lower eight bits
code unsigned char FREQL[] = {
0x42, 0xC1, 0x17, 0xB6, 0xD0, 0xD1, 0xB6, //Bass 1234567
0x21, 0xE1, 0x8C, 0xD8, 0x68, 0xE9, 0x5B, 0x8F, //1,2,3,4,5,6,7,i
0xEE, 0x44, 0x6B, 0xB4, 0xF4, 0x2D, //High pitch 234567
0x47, 0x77, 0xA2, 0xB6, 0xDA, 0xFA, 0x16}; //Super high pitch 1234567
//--------------------------------------
//There is only one good mother in the world. To play different music, just modify this data table
code unsigned char sszymmh[] = {5,1,1,5,1,1,6,1,2,5,1,2,1,2,2,7,1,4,5,1,1,5,1,1,6,1,2,5,1,2,2,2,1,2,4,
5,1,1,5,1,1,5,2,2,3,2,2,1,2,2,7,1,2
};
//--------------------------------------
void t0int() interrupt 1 //T0 interrupt program, control the tone of pronunciation
{
TR0 = 0; //Close T0 first
speaker = !speaker; //Output square wave, pronunciation
TH0 = timer0h; //Next interrupt time, this time, Control the pitch
TL0 = timer0l;
TR0 = 1; //Start T0
}
//--------------------------------------
void delay(unsigned char t) //Delay program, control the length of pronunciation
{
unsigned char t1;
unsigned long t2;
for(t1 = 0; t1 < t; t1++) //Double loop, delay t half beats in total
for(t2 = 0; t2 < 8000; t2++); //During the delay period, you can enter T0 interrupt to pronounce
TR0 = 0; //Turn off T0 and stop pronouncing
}
//--------------------------------------
void song() //Play a note
{
TH0 = timer0h; //Control the tone
TL0 = timer0l;
TR0 = 1; //Start T0, and T0 outputs a square wave to pronounce
delay(time); //Control the time length
}
//--------------------------------------
void main(void)
{
unsigned char k, i;
TMOD = 1; //Set T0 timing working mode 1
ET0 = 1; //Open T0 interrupt IE=0x82;
EA = 1; //Open CPU interrupt
while(1) {
i = 0;
time = 1;
while(time) {
k = sszymmh[i] + 7 * sszymmh[i + 1] - 1;
//The i-th note is a note, The i+1th is which octave
timer0h = FREQH[k]; //Read the frequency value from the data table
timer0l = FREQL[k]; //Actually, it is the time length of the timing
time = ssz
ymmh[i + 2]; //Read the time length value
i += 3;
song(); //Send a note
}
}
}
Answer:
Just look at the main() function. First, define k and i, and then define the type of interrupt (the program uses the timer interrupt). This timer is a bit special. Its function is to define the frequency. The smaller the frequency interval, the higher the pitch, and vice versa. This frequency is the reciprocal of time, so the larger the TH value, the higher the pitch; the smaller the TL value, the lower the pitch. Then go down, while(1) means waiting for the interrupt. The timer interrupt in this program does not set the initial value, so the interrupt is almost not waiting and is triggered all the time (if there is a waiting time, the music will not be connected). In summary: this timer interrupt completes two tasks: 1. Make the MCU trigger all the time (waiting time is almost 0); 2. Control the playing frequency of the notes.
The while(time) loop is the operation of assigning values to the played notes.
20. When should the MCU interrupt enter?
Answer:
It depends on whether the interrupt is an external interrupt, a timer or a serial port interrupt.
If it is an external interrupt, it is when p3.2 and p3.3 detect the level change of these two ports (assuming that these two ports are connected to a key, then when the key is pressed, it indicates that an interrupt is generated), and then jump to the interrupt program execution.
If it is a timer interrupt, there is an interrupt flag TFx (x represents 0 or 1). For example, if you set a 1S timer program, you use 50ms as the base, generate 1s time 20 times, and then when 50ms is over, the flag bit changes, and the timer interrupt program is executed! The
serial port interrupt also has a flag bit. After receiving or sending data, the flag bit changes, and then the interrupt is executed!
Answer:
AD analog-to-digital conversion is to sample the analog voltage value and then convert it into a digital signal. This sampling and conversion takes time. It is not possible to read the digital signal data as soon as
AD is turned on. Generally speaking, the time is only a few microseconds to a few hundred microseconds (depending on the settings). If the microcontroller has no other work, you can use a loop waiting method to wait for the AD conversion to end (the DONE bit will be set after the conversion). But if your microcontroller has other work, there is no need to spend time waiting for it. You can turn on the AD operation and continue to execute other programs. After the conversion is completed, the AD interrupt can temporarily disconnect the existing hype and read the AD data in. This is the role of the AD interrupt.
When (under what circumstances) do the five interrupts of the 2251 microcontroller execute the program inside!
Answer:
External interrupt 0: P3.2 port has a low level (IT0=0)/falling edge (IT0=1).
External interrupt 1: P3.3 port has a low level (IT1=0)/falling edge (IT1=1).
Timer 0 interrupt: When timer 0 counts to FFFF and overflows
Timer 1 interrupt: When timer 1 counts to FFFF and overflows
Serial port interrupt: When the serial port receives a frame or sends a frame of data, an interrupt will be generated.
You can look up TCON and SCON on the Internet. What conditions change the value of the interrupt flag? Then it will enter the interrupt service program.
23. In the 51 single-chip microcomputer, if the interrupt function is relatively long and the interrupt is triggered halfway through execution, will the program stop and execute from the beginning, or respond after the execution is completed?
Answer:
In the 51 single-chip microcomputer, interrupts are divided into high and low priorities. High-priority interrupts can interrupt low-priority interrupts.
But interrupts of the same level cannot interrupt interrupts of the same level! No matter how long the interrupt function is, if the interrupt occurs again halfway through execution, it will still have to wait until the interrupt function is executed and another main program instruction is executed before entering the interrupt again.
However, if the host happens to modify the interrupt to a high priority in this low-priority interrupt service program, then if the interrupt function is relatively long and the interrupt is triggered halfway through execution, the interrupt function will be executed again from the beginning (interrupt nesting). This is because, except for the serial port interrupt, the interrupt flag of other interrupts has been cleared before the CPU responds to the interrupt and the program enters the interrupt function.
In addition, the serial port interrupt of 51 is special because the software needs to clear the serial port interrupt flag. Therefore, as long as the serial port interrupt flag is not cleared, the above interrupt nesting will not occur.
24. Find the 51 microcontroller program, two counters, mainly the function name and initialization settings of the interrupt function.
Answer:
void into_into() interrupt 1 Timer 0 interrupt entry function
{
. . . . Interrupt service program . . . .
TH0=0; //
TL0=0; // Reassign T0 value
}
void into_into() interrupt 3 Timer 1 interrupt entry function
{
. . . . Interrupt service program . . . .
TH1=0;//
TL1=0;// Re-assign value to T1
}
void to_to()
{
TMOD=0x11; //Timer T0 and T1 work mode 1
TH0=0;//
TL0=0;// Initialize T0
TH1=0;//
TL1=0// Initialize T1
TR0=1;// Start counting
ET0=1;// Enable T0 interrupt
TR1=1;// Start counting
ET1=1;// Enable T1 interrupt
EA=1; // Enable general interrupt
}
void main()
{
INIT_T0(); //Timer interrupt initialization
while(1)
{
...........
}
25. I would like to ask a question about microcontroller interrupts: For example, a pulse comes and the interrupt starts, but when the program in the interrupt is halfway executed, another pulse comes. At this time, does the program in the interrupt start from the beginning or continue? What
I mean is that the program is just an interrupt A, a pulse comes, A executes, and when A is halfway executed, another pulse comes, notifying A to execute. Should A be executed from the beginning or ignored?
Answer:
It depends on the specific situation, because different microcontrollers have subtle differences in interrupt mechanisms, and you need to check their information.
Generally speaking, when an interrupt source requests an interrupt, it is a one-time "interrupt registration" for the CPU. If the interrupt is not responded to at that time because the conditions are not met (for example, the CPU is "disabling interrupts", that is, "interrupt enable" is not turned on), the registration information is still there. In this way, once the interrupt enable is turned on in the future, it will still respond, just a little later.
After the interrupt is responded, there must be a way to eliminate this "interrupt registration". The function of some CPUs is: as long as the interrupt is responded, the registration is automatically eliminated. Some CPUs cannot automatically clear the registration, and the operation of "clearing interrupt registration" must be programmed in the interrupt service program, otherwise, once the interrupt enable is turned on, it will interrupt again.
In most current microcontrollers, the interrupt controller and the CPU are in the same chip, which can automatically eliminate the interrupt registration. In the past, many types of CPUs were equipped with an interrupt controller on another chip, so they naturally could not be automatically eliminated.
Some CPUs also have another kind of "unregistered" interrupt request. It must be kept continuously applied by the outside world (the device that issued the interrupt request), and when the interrupt is responded to, it will try to notify the device to cancel the application (for example, send an output signal in the interrupt service program).
In most CPUs, once the service program is entered after responding to the interrupt, the "interrupt enable" is turned off. Therefore, if the next interrupt request comes at this time, it cannot be responded immediately, and can only be registered, and can only be responded to when the interrupt is "opened" later. If the programmer wants to be able to "nest interrupts" (that is, enter another interrupt service program in the middle of the execution of an interrupt service program), it is necessary to program the "open interrupt" operation in the service program.
In principle, "nested interrupts" allow "nesting themselves", that is, the execution of an interrupt service program is interrupted in the middle and enters the same interrupt service program as itself, and executes it from beginning to end. After the end, it returns to the point where it was previously interrupted and continues to execute the second half of the service program. What effect this situation will have is something that programmers need to consider.
Some CPUs also have a "priority" mechanism, which can prohibit other interrupts with a lower priority than itself from interrupting itself in a certain level of interrupt service program. At the same time, it also provides programmers with the flexibility of "giving up priority" and "modifying priority".
The interrupt requests with lower priority that are temporarily "shielded" by the priority mechanism are still registered, and can be responded to after the high-priority interrupt ends.
However, it should be noted that in most CPUs, only one "interrupt registration" can be registered. In other words, before the number registered by the previous interrupt application is cleared, the next interrupt application comes, then the second registration cannot be registered.
However, in some processors, interrupt registration may be divided into several levels: one level inside the CPU, and another level of "preparatory registration" for each specific device in the periphery, which is more complicated. In addition,
a few more words: As mentioned above, programmers can decide whether your interrupt service program allows or does not allow "nesting".
If not allowed, you can use the method of turning off interrupts, or use the priority mechanism to shield the second interrupt request from the same interrupt source.
In this way, the second interrupt will not be responded. But it can still register a number (as long as it occurs after the previous interrupt registration number has been cleared). Then, when the interrupt service program ends, it will generally open the interrupt and release the priority mask. Then, the second interrupt request will be responded to, and the interrupt service program will be executed again.
If "nesting" is allowed, it will be as I said above:
the execution of an interrupt service program is interrupted in the middle and enters the same interrupt service program as itself, and executes it from beginning to end. After the end, it returns to the point where it was previously interrupted and continues to execute the second half of the service program.
Previous article:Introduction to 51 MCU - Use of interrupts
Next article:20 Questions and Solutions on MCU Delay Problems
Recommended ReadingLatest update time:2024-11-15 11:00
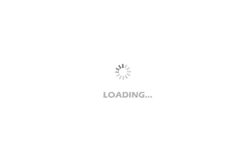
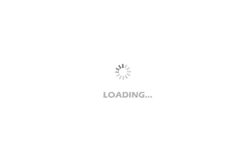
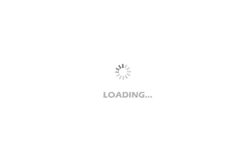
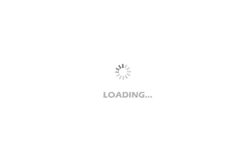
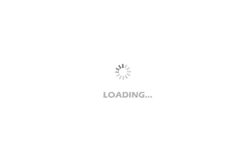
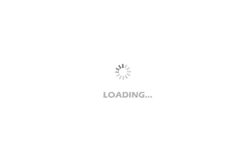
- Popular Resources
- Popular amplifiers
-
Automatic identification of garbage trucks
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- ASML predicts that its revenue in 2030 will exceed 457 billion yuan! Gross profit margin 56-60%
- Detailed explanation of intelligent car body perception system
- How to solve the problem that the servo drive is not enabled
- Why does the servo drive not power on?
- What point should I connect to when the servo is turned on?
- How to turn on the internal enable of Panasonic servo drive?
- What is the rigidity setting of Panasonic servo drive?
- How to change the inertia ratio of Panasonic servo drive
- What is the inertia ratio of the servo motor?
- Is it better for the motor to have a large or small moment of inertia?
- EEWORLD University Hall----Live Replay: Rochester Rochester Electronics tells you in detail-Challenges and solutions after semiconductor production suspension
- The fifth pitfall of domestic FPGA chips: "No devices found" reappears
- [Xianji HPM6750 Review] + The previous screen adapter board has been soldered for testing
- What should I do if I can't find an LCD screen of the right size?
- Allwinner heterogeneous multi-core AI intelligent vision V853 development board evaluation + openwrt firmware packaging pack report mbr error solution
- TI TMS320F28335 program SVPWM source program
- 【Smart Kitchen】Environmental Sensor PCB Design
- 5. Printf implementation and DMA implementation of USART0
- When debugging a program with JT-LINK simulation, a message appears saying that CORTEX-M cannot be found after just a few minutes of debugging. What is the reason?
- Unboxing - ESP32-S2 and ESP32-S3 Development Boards + Modules