The four programs S3C2440A.s; 2440lib.c; main.c; timer.c need to be added to the project.
Main program:
/*****************************************************************************************************
* File name: main.c
* Author: ZXL
* Description: Use the timer to make the LED light flash once every 0.5 seconds and the buzzer beep once every 0.5 seconds
* History: 2013.5.8
*************************************************************************************************/
#include "def.h"
#include "option.h"
#include "2440addr.h"
#include "2440lib.h"
void dely(U32 tt)
{
U32 i;
for(;tt>0;tt--)
for(i=0;i<10000;i++);
}
void init()
{
U32 i;
U8 key;
U32 mpll_val=0;
i = 2 ;
switch ( i ) //Set the clock frequency of 2440
{
case 0: //200
key = 12;
mpll_val = (92<<12)|(4<<4)|(1);
break;
case 1: //300
key = 13;
mpll_val = (67<<12)|(1<<4)|(1);
break;
case 2: //400
key = 14;
mpll_val = (92<<12)|(1<<4)|(1);
break;
case 3: //440!!!
key = 14;
mpll_val = (102<<12)|(1<<4)|(1);
break;
default:
key = 14;
mpll_val = (92<<12)|(1<<4)|(1);
break;
}
//MDIV=92,PDIV=1,SDIV=1 Determined by configuring MPLLCON, MPLL, that is, FCLK=400MHZ
ChangeMPllValue((mpll_val>>12)&0xff, (mpll_val>>4)&0x3f, mpll_val&3);
ChangeClockDivider(key, 12); //FCLK:HCLK:PCLK = 1:1/4:1/8 =400M:100M:50M
Port_Init();
Uart_Init(0,115200);
Uart_Select(0);
Uart_Printf("n flashes once and beeps once every 0.5 seconds!n");
}
int main(int argc, char **argv)
{
init();
led1_port_init();
beep_init();
timer0_init();
Uart_Printf("nTimer Test beginn");
while(1);
}
Timer program:
/*****************************************************************************************************
* File name: timer.c
* Author: ZXL
* Description: Use the timer to make the LED light flash once every 0.5 seconds and the buzzer beep once every 0.5 seconds
* History: 2013.5.8
*************************************************************************************************/
#include "def.h"
#include "option.h"
#include "2440addr.h"
#include "2440lib.h"
#define LED1_ON ~(1<<5)
#define LED2_ON ~(1<<6)
#define LED3_ON ~(1<<7)
#define LED4_ON ~(1<<8)
#define LED1_OFF (1<<5)
#define LED2_OFF (1<<6)
#define LED3_OFF (1<<7)
#define LED4_OFF (1<<8)
void led1_port_init()
{
rGPBCON &= ~(0x3<<10); //Control register clear
rGPBCON |= (0x1<<10); //Control register is set to output
rGPBDAT |= (LED1_OFF)|(LED2_OFF)|(LED3_OFF)|(LED4_OFF); //All LED lights are off
}
void led1_run()
{
if(rGPBDAT &(1<<5)) rGPBDAT &=~(1<<5); //The LED light was originally on——> then it turns off
else rGPBDAT |=(1<<5); //The LED light was off ——> it turns on
}
void beep_init()
{
rGPBCON &= ~(3<<0); //Control register clear
rGPBCON |= (1<<0); //Control register is set to output
}
void beep_run()
{
if(rGPBDAT &(1<<0)) rGPBDAT &=~(1<<0); //Buzzer beeps——> then stops
else rGPBDAT |=(1<<0); //Buzzer stops——> then it will beep
}
void __irq IRQ_Timer0_Handle(void)
{
if(rINTPND==BIT_TIMER0) //If BIT_TIMER0 triggers an interrupt, the corresponding BIT_TIMER0 bit in the INTPEND register is set to 1
ClearPending(BIT_TIMER0); // Clear BIT_TIMER0 interrupt
led1_run(); //LED flashes
beep_run(); //Buzzer beeps
}
void timer0_init()
{
rTCFG0 = 49; //The first 50 division pclk/(49+1)=50M/50=1M
rTCFG1 = 0x03; //The second 16-division frequency = 62500HZ = 1M/16
rTCNTB0 = 62500/2; //TCNTB0[15:0]=count value
rTCMPB0 = 0;
rTCON |=(1<<1); //Load the count value into TCNTB0, TCMPB0
rTCON =0x09; //Start timer 0 and automatically reload
rINTMOD = 0x0; // Set to IRQ mode
ClearPending(BIT_TIMER0); // Clear BIT_TIMER0 interrupt
pISR_TIMER0 = (U32)IRQ_Timer0_Handle; //Correspond the timer execution function to the timer interrupt entry address
EnableIrq(BIT_TIMER0); //Enable BIT_TIMER0 interrupt
Uart_Printf("n interrupt type INTMSK=0x%xn",rINTMSK); //Display the internal type of interrupt that the CPU will service 1: shield 0: serviceable
}
Previous article:2440 bare metal program - serial port sending and receiving data
Next article:2440 bare metal program - key interrupt
Recommended ReadingLatest update time:2024-11-16 14:45
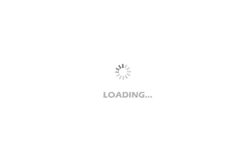
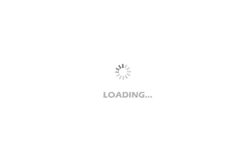
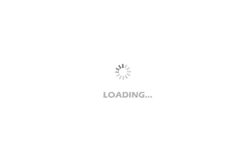
![[JZ2440 Notes] Bare metal experiment using NandFlash](https://6.eewimg.cn/news/statics/images/loading.gif)
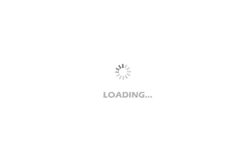
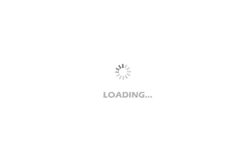
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- capacitance
- The basic principles and methods of SRIO error handling
- FPGA button problem
- How to effectively choose a lithium battery protection board
- About continue in for loop
- ARM programming mode and some conceptual understanding
- Detailed explanation and selection of pull-up resistors and pull-down resistors for microcontrollers
- Squelch Tuning Audio Switching/Mixing Circuit Diagram
- Principles of peripheral expansion of single-chip microcomputer hardware system
- How to output full load of power module