The clock source of the STM8 microcontroller can be selected as internal or external, and can be easily switched during system operation.
The following experimental program first switches the main clock source to an external crystal oscillator with an oscillation frequency of 8MHZ, and then quickly flashes the LED indicator. Next, the main clock source is switched to the internal oscillator with an oscillation frequency of 2MHZ, and then the LED indicator flashes slowly. By observing the flashing frequency of the LED indicator, it can be seen that the same loop code changes the flashing frequency and duration due to the change of the main clock source.
Still using ST's development tools, generate an assembler framework, and then modify main.asm. The modified code is as follows.
stm8/
#include "mapping.inc"
#include "STM8S207C_S.INC"
; Define the starting and ending positions of the stack space
stack_start.w EQU $stack_segment_start
stack_end.w EQU $stack_segment_end
segment 'rom'; Below is the definition of a segment, which is located in ROM
main.l ; Define the label of the first instruction after reset (i.e. entry address)
;
; First, initialize the stack pointer
LDW X,#stack_end
LDW SP,X
LD A,#08
LD PD_DDR,A ; Set PD3 to output
LD A,#08
LD PD_CR1,A ; Set PD3 to push-pull output
LD A,#00
LD PD_CR2,A ;
LD A,#$01
LD CLK_ECKR,A ; Allow external high speed oscillator to work
WAIT_HSE_READY.L
LD A,CLK_ECKR
AND A,#$02
JREQ WAIT_HSE_READY ; Wait for the external high speed oscillator to be ready
; Note: After the above switch, the main clock switches from HSI/8 (2MHZ) to HSE (8MHZ)
;
MAIN_LOOP.L
; Set the CPU clock divider below so that the CPU clock = main clock
; Through the LED, it can be seen that the speed of program running has indeed been significantly improved
LD A,#$02
LD CLK_SWCR,A ; SWEN <- 1
LD A,#$B4
LD CLK_SWR,A ; Select the high-speed oscillator outside the chip as the main clock
WAIT_CLK_SWITCH_1.L
LD A,CLK_SWCR
AND A,#$08
JREQ WAIT_CLK_SWITCH_1 ; Wait for the switch to succeed
LD A,#$00 ; Clear the switch flag
LD CLK_SWCR,A
LD A,#10; LED flashes 10 times at high speed
HIGH_SPEED.L
PUSH A ; save register
LD A,#08
LD PD_ODR,A ; Set the output of PD3 to 1
LD A,#100
CALL DELAY_MS ; Delay 100MS
LD A,#00
LD PD_ODR,A ; Set the output of PD3 to 1
LD A,#100
CALL DELAY_MS ; Delay 100MS
POP A ; restore register
DEC A
JRNE HIGH_SPEED
; Set the CPU clock divider below so that CPU clock = main clock / 4
; Through the LED, it can be seen that the speed of program running has indeed dropped significantly
LD A,#$02
LD CLK_SWCR,A ; SWEN <- 1
LD A,#$E1 ; Select HSI as the main clock source
LD CLK_SWR,A
WAIT_CLK_SWITCH_2.L
LD A,CLK_SWCR
AND A,#$08
JREQ WAIT_CLK_SWITCH_2 ; Wait for the switch to succeed
LD A,#$00 ; Clear the switch flag
LD CLK_SWCR,A
; Note: After the above switch, the main clock switches from HSE (8MHZ) to HSI/8 (2MHZ)
LD A,#10; LED flashes 10 times at low speed
LOW_SPEED.L
PUSH A ; save register
LD A,#08
LD PD_ODR,A ; Set the output of PD3 to 1
LD A,#100
CALL DELAY_MS ; Delay 100MS
LD A,#00
LD PD_ODR,A ; Set the output of PD3 to 1
LD A,#100
CALL DELAY_MS ; Delay 100MS
POP A ; restore register
DEC A
JRNE LOW_SPEED
JRA MAIN_LOOP
; Function: Delay
; Input parameter: Register A - the number of milliseconds to delay, assuming the CPU frequency is 2MHZ
; Output parameters: None
; Return value: None
; Note: None
DELAY_MS.L
PUSH A ; save the entry parameters to the stack
LD A,#250; Register A<-250, as the following loop number
DELAY_MS_1.L
NOP; Use no operation instruction to delay 4T
NOP
NOP
NOP
NOP
DEC A; Register A<-A-1, the execution time of this instruction is 1T
JRNE DELAY_MS_1; If not equal to 0, then loop,
; The execution time of this instruction is 2T (jump time) or 1T (no jump time)
POP A ; restore entry parameters from the stack
DEC A ; The number of MSs to be delayed - 1
JRNE DELAY_MS; If not equal to 0, then loop
RET ; function returns
interrupt NonHandledInterrupt
NonHandledInterrupt.l
iret
; The interrupt vector table is defined below
segment 'vectit'
dc.l {$82000000+main} ; reset
dc.l {$82000000+NonHandledInterrupt}; trap
dc.l {$82000000+NonHandledInterrupt}; irq0
dc.l {$82000000+NonHandledInterrupt}; irq1
dc.l {$82000000+NonHandledInterrupt}; irq2
dc.l {$82000000+NonHandledInterrupt}; irq3
dc.l {$82000000+NonHandledInterrupt}; irq4
dc.l {$82000000+NonHandledInterrupt}; irq5
dc.l {$82000000+NonHandledInterrupt}; irq6
dc.l {$82000000+NonHandledInterrupt}; irq7
dc.l {$82000000+NonHandledInterrupt}; irq8
dc.l {$82000000+NonHandledInterrupt}; irq9
dc.l {$82000000+NonHandledInterrupt} ; irq10
dc.l {$82000000+NonHandledInterrupt} ; irq11
dc.l {$82000000+NonHandledInterrupt} ; irq12
dc.l {$82000000+NonHandledInterrupt} ; irq13
dc.l {$82000000+NonHandledInterrupt} ; irq14
dc.l {$82000000+NonHandledInterrupt} ; irq15
dc.l {$82000000+NonHandledInterrupt} ; irq16
dc.l {$82000000+NonHandledInterrupt} ; irq17
dc.l {$82000000+NonHandledInterrupt} ; irq18
dc.l {$82000000+NonHandledInterrupt} ; irq19
dc.l {$82000000+NonHandledInterrupt}; irq20
dc.l {$82000000+NonHandledInterrupt} ; irq21
dc.l {$82000000+NonHandledInterrupt}; irq22
dc.l {$82000000+NonHandledInterrupt}; irq23
dc.l {$82000000+NonHandledInterrupt} ; irq24
dc.l {$82000000+NonHandledInterrupt}; irq25
dc.l {$82000000+NonHandledInterrupt} ; irq26
dc.l {$82000000+NonHandledInterrupt}; irq27
dc.l {$82000000+NonHandledInterrupt}; irq28
dc.l {$82000000+NonHandledInterrupt} ; irq29
end
Previous article:STM8与汇编语言(13)--修改CPU的时钟
Next article:STM8 and Assembly Language (15) - AD Conversion
Recommended ReadingLatest update time:2024-11-15 18:45
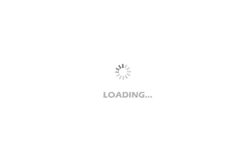
![stm8 IAR compile error atal Error[Pe035]: #error directive](https://6.eewimg.cn/news/statics/images/loading.gif)
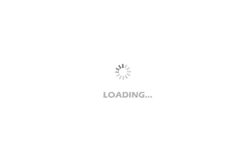
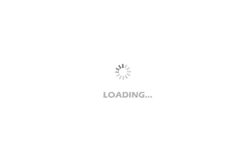
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
Signal Integrity and Power Integrity Analysis (Eric Bogatin)
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Sandia Labs develops battery failure early warning technology to detect battery failures faster
- Ranking of installed capacity of smart driving suppliers from January to September 2024: Rise of independent manufacturers and strong growth of LiDAR market
- Industry first! Xiaopeng announces P7 car chip crowdfunding is completed: upgraded to Snapdragon 8295, fluency doubled
- P22-009_Butterfly E3106 Cord Board Solution
- Keysight Technologies Helps Samsung Electronics Successfully Validate FiRa® 2.0 Safe Distance Measurement Test Case
- Innovation is not limited to Meizhi, Welling will appear at the 2024 China Home Appliance Technology Conference
- ADI & Shijian New Infrastructure Series, Episode 1 - Answer questions about Industrial Ethernet and win prizes!
- Is the 6-pin 2.54mm double-row pin 3 in a row or 6 in a row?
- 【Silicon Labs Development Kit Review 08】_stateflow implements Uart scheduling
- Activated carbon to remove formaldehyde
- nRF51822 Open
- FC Insulation Connection Technology
- Development of STM32 online burning program
- National VI OBD emission terminal H6S remote online monitoring production test tool
- Design and Implementation of VGA Image Controller Based on CPLDFPGA
- [Live broadcast has ended] TI's new generation of high-performance, low-power Zigbee solutions