In order to design a traffic light with a left turn signal at an intersection, one must first thoroughly understand the control logic of the circular traffic light.
In the design process of this example, I designed the signal control logic of this intersection: north-south straight-through release -> east-west straight-through release -> north-south left turn release -> east-west left turn release. If the display problem of the digital tube is not considered, it is only necessary to slightly modify the control program of the circular signal light. However, in actual applications, most intersections are equipped with digital display digital tubes to display the countdown seconds of the red, yellow and green lights in the straight direction to improve traffic efficiency - so it is very necessary to add the countdown display function to the design.
I drew the complete signal control logic as the following diagram.
In actual programming, since I did not fully understand the control logic at the beginning, the control program I wrote was completed by patching up the circular signal control system, which was somewhat accidental. When analyzing this example, I suggest that you first thoroughly understand the control logic, and then ignore the countdown display. After confirming that the design is correct, add the countdown display function. In addition, I have sent all the files of this example design to my resource area, and you are welcome to download and use it.
#include #include void delay()//delay 0.5s { short i, j; for(i=0;i<124;i++) for(j=0;j<500;j++) ; } void delay_night()//delay 1s { short i, j; for(i=0;i<248;i++) for(j=0;j<500;j++) ; } void main() { short int t=0, t1=0, t2=0; //t1 is responsible for timing the straight signal, t2 is responsible for timing the left turn signal, and t is responsible for the display of the digital tube P0=0; P1=0; P2=0; P3=0; while(1) { //Straight module, in this module, the left turn signals in the north-south and east-west directions are all red P0=0x12; //Green light cycle in north-south direction P2=0x14; //Green light on in north-south direction + red light on in east-west direction t1=35; while(t1>=0) { P1=t1/2/10*pow(2,4)+t1/2%10; //North-South direction digital tube setting P3=(t1/2+3)/10*pow(2,4)+(t1/2+3)%10; //Set the digital tube in the east-west direction if(t1<=5) //The green light in the north-south direction lasts for 3 seconds { if(t1%2!=0) { P2=0x10; //Green light in north-south direction is off + red light in east-west direction is on } else { P2=0x14; //Green light on in north-south direction + red light on in east-west direction } } delay(); t1--; } // Yellow light cycle in north-south direction P2=0x12; // Yellow light on in north-south direction + red light on in east-west direction t1=5; while(t1>=0) { P1=t1/2/10*pow(2,4)+t1/2%10; //North-South direction digital tube setting P3=t1/2/10*pow(2,4)+t1/2%10; //Set the digital tube in the east-west direction delay(); t1--; } //Red light cycle in north-south direction (need to add the time for turning left in both directions) P2=0x41; //Red light on in the north-south direction + green light on in the east-west direction t1=77; t=t1+88; //The north-south digital tube displays 88=41+5+39+3 while(t1>=0) { if(t1/2>2) { P1=t/2/10*pow(2,4)+t/2%10; //North-South direction digital tube setting P3=(t1/2-3)/10*pow(2,4)+(t1/2-3)%10; //Set the digital tube in the east-west direction if(t1/2<=5) //The green light in the east-west direction lasts for 3 seconds { if(t1%2!=0) { P2=0x01; //Red light on in the north-south direction + green light off in the east-west direction } else { P2=0x41; //Red light on in the north-south direction + green light on in the east-west direction } } } else //Red light on in north-south direction + yellow light on in east-west direction { P2=0x21; //Red light on in the north-south direction + yellow light on in the east-west direction P1=t/2/10*pow(2,4)+t/2%10; //North-South direction digital tube setting P3=t1/2/10*pow(2,4)+t1/2%10; //Set the digital tube in the east-west direction } delay(); t1--; t--; } //Left turn module, during which the lights for north-south and east-west directions are all red P2=0x11; //Change the light for driving in the east-west direction to red P24=1, and change the light for driving in the north-south direction to red P20=1 //Green light cycle in north-south direction P0=0x18; //Green light on in north-south direction + red light on in east-west direction P03=1, P04=1 t2=41; while(t2>=0) { if(t2<=5) //The green light in the north-south direction lasts for 3 seconds { if(t2%2!=0) { P0=0x10; //Green light in north-south direction is off + red light in east-west direction is on } else { P0=0x18; //Green light on in north-south direction + red light on in east-west direction } } P1=t/2/10*pow(2,4)+t/2%10; //North-South direction digital tube setting P3=(t+46)/2/10*pow(2,4)+(t+46)/2%10; //Set the digital tube in the east-west direction delay(); t2--; t--; } // Yellow light cycle in north-south direction P0=0x14; // Yellow light on in north-south direction + red light on in east-west direction P02=1, P04=1 t2=5; while(t2>=0) { P1=t/2/10*pow(2,4)+t/2%10; //North-South direction digital tube setting P3=(t+46)/2/10*pow(2,4)+(t+46)/2%10;;//Set the digital tube in the east-west direction 46=41+5 delay(); t2--; t--; } //Red light cycle in north-south direction P0=0x42; //Red light on in north-south direction + green light on in east-west direction P01=1, P06=1 t2=39; while(t2>=0) { if(t2/2>2) { if(t2/2<=5) //The green light in the east-west direction lasts for 3 seconds { if(t2%2!=0) { P0=0x02; //Red light on in the north-south direction + green light off in the east-west direction } else { P0=0x42; //Red light on in the north-south direction + green light on in the east-west direction } } } else //Red light in north-south direction is on + yellow light in east-west direction is on P01=1, P05=1 { P0=0x22; //Red light on in north-south direction + yellow light on in east-west direction } P1=t/2/10*pow(2,4)+t/2%10; //North-South direction digital tube setting P3=(t+46)/2/10*pow(2,4)+(t+46)/2%10; //Set the digital tube in the east-west direction delay(); t2--; t--; } } }
Previous article:8051 single chip microcomputer (STC89C52) eight-segment digital tube displays 0~7 in turn
Next article:proteus7.7+Keil2 simulation 80C51 control intersection signal light (no green light countdown + green light countdown
Recommended ReadingLatest update time:2024-11-16 20:52
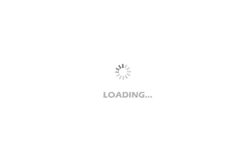
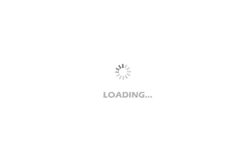
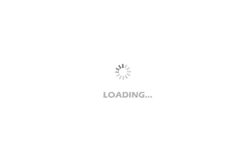
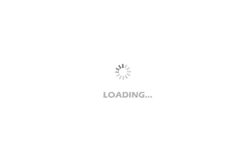
- Popular Resources
- Popular amplifiers
-
Network Operating System (Edited by Li Zhixi)
-
Microgrid Stability Analysis and Control Microgrid Modeling Stability Analysis and Control to Improve Power Distribution and Power Flow Control (
-
MATLAB and FPGA implementation of wireless communication
-
Introduction to Internet of Things Engineering 2nd Edition (Gongyi Wu)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 3.EV_HC32F460_UART debugging
- The basic physics of ultrasonic sensors and the time difference between received signals
- 【Goodbye 2021, Hello 2022】The Walking Chinese Chip
- Last day: Sign up for the ST MEMS Sensor Creative Design Competition and win big prizes with DIY
- Getting Started with Smart Home Audio Design
- Radio Frequency Integrated Circuits, how to learn this field well?
- [Project source code] Altera FPGA enables the on-chip pull-up resistor function of the pin
- Recommended Espressif ESP32+WT32-SC01
- RISC-V MCU Development (10): File Version Management
- Development of an electric bicycle charging system based on SPCE061A and CPLD