Because I just joined the company and don't know much about serial port debugging, I will sort out the serial port program in the book. Portability is relatively strong
Instructions: There are two ways to query the serial port of the MC9S12 series
1》Use interrupt mode to query
2》Use polling method to query in the main function
Both methods are reflected in the following functions, and you need to extract them separately when using them.
Mainly initialize the register configuration of SCI1CR2 and call the interrupt. Note that the interrupt number of serial port 1 is VectorNumber_Vsci1 21
Note that the interrupt number of serial port 0 is VectorNumber_Vsci0 20
The following is the function called when polling is used in the main function
/*****************************Serial port polling detection************************************************************************************/
#if 0
if(SCI1SR1_RDRF)
{
JieSHOU_Ddate[i]=SCI1DRL;
i++;
if(i==12)
{
i=0;
JieSHOU_flag=1; //Receive 12 array data
}
}
//MFD_Printf("JieSHOU_Ddate %d!!rn",JieSHOU_Ddate[i]);
#endif
*************************************************************************************************************************************************
The following is the original function, the specific usage can be modified according to your needs!
********
/*---- include ---------------------------------------*/
#include Printf.h"
#include #include /*---- config-----------------------------------------*/ #if MFD_DBGUG const char Hex[] = "0123456789ABCDEF"; unsigned int JieSHOU_Ddate[12]; char ASCALL_TO16[4]; int i=0; char JieSHOU_flag; unsigned int JieSHOU(); /*----------------------------------------------------*/ /*********************************************************** * Function name: void USART_Init(void) * Created by: ZXL * Function: Serial port initialization * Version: 1.0.0 * Date: January 17, 2019 ***********************************************************/ void USART_Init(void) { /************Only send configuration****************************/ #if 0 SCI1BD = 14; //Baud rate 115200 SCI1CR1 = 0x00; //Mode configuration data bit: 8 check bit: none stop bit: 1 SCI1CR2 = 0x08; //Send and receive configuration #endif #if 1 /************Receive interrupt mode********************/ SCI1BD = 14; //Baud rate 115200 SCI1CR1 = 0x00; //Mode configuration data bit: 8 check bit: none stop bit: 1 SCI1CR2 = 0x2c; //Send and receive configuration, receive enable, receiver full interrupt enable #endif // SCI1CR2_ILIE=1; Line idle enable /************Receive query method********************/ #if 0 SCI1BD = 14; //Baud rate 115200 SCI1CR1 = 0x00; //Mode configuration data bit: 8 check bit: none stop bit: 1 SCI1CR2 = 0x0c; //Send and receive configuration, receive enable, receiver full interrupt enable #endif } /*********************************************************** * Function name: void USART_SendByte(uint16_t Data) * Created by: ZXL * Function: Serial port sending function * Version: 1.0.0 * Date: January 2, 2019 ***********************************************************/ static void USART_SendByte(uint16_t Data) { SCI1DRL = Data; while(!SCI1SR1_TC) { ; } } /*********************************************************** * Function name: void DoPrint( const char *fmt, va_list ap ) //va_list char * Created by: ZXL * Function: Execute print function * Version: 1.0.0 * Date: January 2, 2019 ***********************************************************/ static void DoPrint( const char *fmt, va_list ap ) { char ch; char *ptr; int value; uint8_t fl_zero; uint32_t i, fl_len, cnt, mask = 1; while(1) { switch(ch = *fmt++) { case 0: return; case '%': if( *fmt != '%' ) { break; } else { } fmt++; default: USART_SendByte(ch); continue; } fl_zero = 0; cnt = 0; ch = *fmt++; if(ch == '0') { fl_zero = 1; ch = *fmt++; cnt = ch - '0'; ch = *fmt++; } else if( (ch >= '0') && (ch <= '9')) { cnt = ch - '0'; ch = *fmt++; } else { } fl_len = 4; switch(ch) { case 'l': case 'L': ch = *fmt++; fl_len = 4; break; case 'b': case 'B': ch = *fmt++; fl_len = 1; break; default: break; } switch(ch) { case 'd': case 'u': switch(fl_len) { case 1: if(ch == 'd') { value = (char)va_arg(ap, int); } else { value = (uint8_t)va_arg(ap, int); } break; case 4: if(ch == 'd') { value = (uint32_t)va_arg(ap, uint32_t); } else { value = (uint32_t)va_arg(ap, uint32_t); } break; default: break; } if(value < 0) { USART_SendByte('-'); value = value*(-1); } else { } if(cnt == 0) { if(value == 0) { USART_SendByte('0'); continue; } else { } for(cnt=0, mask=1; cnt<10; cnt++) { if((value / mask)==0) { break; } else { } mask = mask * 10; } } else { } for(i = 0, mask = 1; i < cnt-1; i++) { mask = mask*10; } while(1) { ch = (value / mask) + '0'; if((ch=='0') && (fl_zero==0) && (mask != 1)) { ch=' '; } else { fl_zero = 1; } USART_SendByte(ch); value = value % (mask); mask = mask / 10; if(mask == 0) { break; } else { } } continue; case 'x': case 'X': switch(fl_len) { case 1: value = (uint8_t)va_arg(ap, int); break; case 4: value = (uint32_t)va_arg(ap, int); break; default: break; } if(value <= 0x0F) { cnt = 1; } else if(value <= 0xFF) { cnt = 2; } else if(value <= 0xFFF) { cnt = 3; } else if(value <= 0xFFFF) { cnt = 4; } else if(value <= 0xFFFFF) { cnt = 5; } else if(value <= 0xFFFFFF) { cnt = 6; } else if(value <= 0xFFFFFFF) { cnt = 7; } else { cnt = 8; } for(i=0; i USART_SendByte(Hex[(value >> (cnt - i - 1) * 4) & 0x000F]); } continue; case 's': ptr = (char *)va_arg(ap, char*); while(*ptr!='') { USART_SendByte(*ptr++); } continue; case 'c': value = va_arg(ap, int); USART_SendByte((uint8_t)value); continue; default: value = (uint16_t)va_arg(ap, int); continue; } } } /*********************************************************** * Function name: void MFD_Printf(const char *fmt, ...) * Created by: ZXL * Function: Serial port printing function * Version: 1.0.0 * Date: January 2, 2018 ***********************************************************/ void MFD_Printf(const char *fmt, ...) { va_list ap; va_start(ap,fmt); DoPrint(fmt, ap); va_end(ap); } #else void MFD_Printf(const char *fmt, ...) { } #endif unsigned int JIESHOU() { unsigned int Result; unsigned int Result_high;
Previous article:freescale MC9S12G128 SCI, printf usage
Next article:MC9S12G128 PLL Settings
Recommended ReadingLatest update time:2024-11-23 11:38
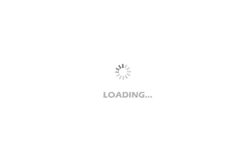
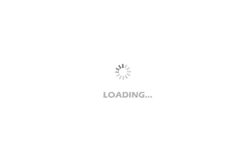
- Popular Resources
- Popular amplifiers
-
Practical Development of Automotive FlexRay Bus System (Written by Wu Baoxin, Guo Yonghong, Cao Yi, Zhao Dongyang, etc.)
-
Automotive Electronics KEA Series Microcontrollers——Based on ARM Cortex-M0+ Core
-
Automotive integrated circuits and their applications
-
ADS2008 RF Circuit Design and Simulation Examples (Edited by Xu Xingfu)
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Mini Adafruit QT Py Development Board
- [Motor Kit P-NUCLEO-IHM] Check-in for Chapter 6, Task 1
- openmv3 serial port hangs up
- Design and Implementation of VGA Image Controller Based on CPLDFPGA
- HC32F460 series virtual serial port problem
- It’s really stuffy to wear a mask when going out in summer. Is there any good solution?
- Smoke sensor as wireless power supply
- Address alignment trap problem occurs when ARM controls FPGA IP core
- Resonant soft switching technology and its application in inverter power supply
- What are the methods for upgrading the msp430 microcontroller program?