ST 3-in-1 development board stm8 learning led
This example uses three peripherals: adc tim and gpio. The program function is to change
the flashing frequency and brightness of the led light according to the analog quantity input by adc.
Configuration process:
Adc related program:
void ADC_Init(void)
{
ADC_CR2 = 0x00; Configuration register 2 Configure whether the external trigger is enabled Select the external trigger mode Data alignment bit Scan mode is enabled bit Here it is configured to disable external trigger Conversion data left aligned Disable scan mode?
ADC_CR1 = 0x00; Configuration register 1 Configure pre-scaling bit Conversion mode is word or continuous ad conversion switch. Here it is configured as fadc=fmaster/2, single conversion, disable adc
ADC_CSR = 0x03; Control status register: configure various interrupt enable conversion completion flags and conversion channels. Here is the configuration to disable various interrupts Select channel 3 pb3
ADC_TDRL = 0x20; The ADC_TDRH and ADC_TDRL registers can be used to disable the Schmitt trigger in the AIN analog input pin. Disabling the Schmitt trigger can reduce the power consumption of the I/O pin.
There are many more registers. For specific uses, please refer to the relevant instructions.
}
GPIO related
void GPIO_Init(void)
{
/* LED IO Configuration */
/* LD3: PD3 */ Please refer to the GPIO explanation part
/* LD2: PD1 */
/* LD1: PD0 */
PD_DDR |= 0x0D; /* before
*/ PD_CR1 |= 0x0D; /* already */
PD_CR2 = 0x00; /* */ mentioned
/* External interrupt pd7 */
EXTI_CR1 = 0x00; Configuration Trigger mode Here it is configured as falling edge and low level trigger
EXTI_CR2 = 0x00; Interrupt trigger mode Falling edge and low level trigger
PD_DDR &=~0x80; PD7 input mode
PD_CR2 |= 0x80; Enable PD7 external interrupt
}
Tim related:
void TIM_Init(void)
{
/* TIM2 CC2 controls brightness */
TIM2_CCMR2 |= 0x70; Configured as pwm2 mode, PWM mode 2 - When counting up, once TIMx_CNT
TIM2_CCER1 |= 0x30; /*Select channel 2 Output low level Valid */
TIM2_ARRH = 0x00;
TIM2_ARRL = 0xff; /*Configure reload value ARRH default value is ff */
TIM2_CCR2 = 0x00; /*Output compare 2 value Used to compare with cnt Start
here is zero Output to the pin level is zero
(valid level) PWM2 mode */
TIM2_PSCR = 0x00; /*Prescaler value Value + 1 */
TIM2_CR1 |= 0x01; Enable count cr1 highest bit Enable preload function
Disable here
TIM2 channel 2 is configured as PWM2 mode above (When counting up, once TIMx_CNT
And configure the reload value and prescale value, and start counting. About the counting mode, there should be only the upward counting mode.
The following is the configuration of tim3 channel 1
*/
/* TIM3 CC1 controls flashing */
TIM3_CCMR1 |= 0x78; Configured as pwm2 mode Turn on the pre-load function
TIM3_CCER1 |= 0x03; /* Select channel 1 */
TIM3_ARRH = 0x03;
TIM3_ARRL = 0xff;
TIM3_CCR1H = 0x02;
TIM3_CCR1L = 0x00;
TIM3_PSCR |= 0x0d;
TIM3_CR1 |= 0x81;
}
The configuration of tim3 is the same as tim2. No more explanation.
The most commonly used timer is timing and generating different waveforms. For basic timing, we can directly set it to counting mode. Output comparison and pwm are as described above.
Turn on the output capture function and turn on the corresponding channel, and set the low level to the effective level.
void ADC_Init(void)
{
ADC_CR2 = 0x00;
ADC_CR1 = 0x00;
ADC_CSR = 0x03; Select analog channel 3 input
ADC_TDRL = 0x20; Turn off the corresponding Schmitt trigger
}
Set adc bit Single conversion Conversion data is left-aligned and stored. Select analog channel 3 input, turn off the corresponding Schmitt trigger (rectified wave),
Ok The above configures the gpio port of the led and the port of the external trigger interrupt, the two comparison outputs of the timer. And adc single conversion input. Take a look at the main program:
void LED_Control(unsigned char duration)
{
int i = 0;
unsigned char uc = 0;
unsigned long Temp;
ADC_CR1 |= 0x01;
i = 6;
while(i--);
ADC_CR1 |= 0x01;
while(!(ADC_CSR & 0x80));/* Waiting for AD convert finished (EOP=1).
To turn on the adc, you need to assign values to ADON twice. The first time is to turn on the power supply of the adc, and then assign values again when the power supply is stable to start the conversion./*
Store ADC value to AD_Value */
AD_Value = ((((unsigned int)ADC_DRH)<<2)+ADC_DRL);
/* The new duty cycle value is written in CCR. */
TIM2_CCR2H=0x00;
TIM2_CCR2L=(unsigned char)(AD_Value>>2);
Change the value of the compare register according to the value of the adc, because tim2 Used to change the brightness, so the low level time should be short
if (AD_Value>0x10)
{
Temp=(unsigned char)(AD_Value>>8);
if ((TIM3_ARRH>Temp+0x05)||(TIM3_ARRH
TIM3_ARRH = Temp;
TIM3_ARRL = (unsigned char)(AD_Value&0xff);
TIM3_CCR1H = (unsigned char)(AD_Value>>9);
TIM3_CCR1L = (unsigned char)((AD_Value>>1)&0xff);
}
}
When the conversion value is greater than 0x10, the light flashes and keeps changing.
/* Delay time = duration * Y */
while ( uc < duration ) /* The following loop is run "duration" times. */
{
while ( i < 1200 ) /* This loop "Y" waits approximately 4.3ms. */
{
i++;
}
i = 0;
uc++;
}
The above is the delay function
}
Ok So far for the LED example
Previous article:3-in-1 kit stm8 study notes css
Next article:Description of assert_param() of stm8s
Recommended ReadingLatest update time:2024-11-24 11:38
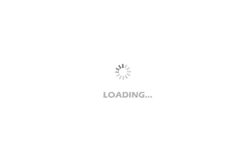
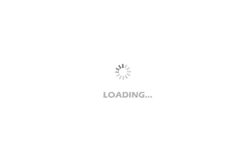
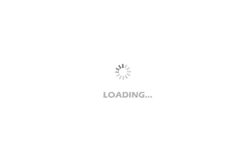
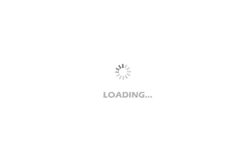
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- I would like to ask what the symbols on the LCD screen of the State Grid electricity meter mean. I have never understood what they mean. Please give me some advice. Thank you.
- The serial UART of DSP 5502 uses DMA to transfer data, 16 bytes at a time
- bq76930 error in reading temperature value
- Share the problem and solution of using TB to capture pulse width of MSP430
- When using CPLD to decode AES/EBU audio, the decoded data has spike pulses. What is the reason?
- Position2Go Review 4. Structure
- 51 single chip digital tube display
- [Sipeed LicheeRV 86 Panel Review] Developing RISCV Bare Metal Programming Using the Emerging Programming Language Rust
- Use WINHEX to check the SD card problem
- Keil and chip package