A brief introduction to Arm920T registers:
R1-R15: General registers
R13: Stack pointer register
R14: Program connection register. When the BL subroutine call instruction is executed, R14 obtains a backup of R15 (program counter register pc). When an interrupt or exception occurs, the corresponding R14_svc, R14_irq, etc. save the return value of R15.
R15: Program counter pc
CPSR: Current Program Status Register
1. T bit (1 bit) Thumb/Arm.
2. I and F bits (bits 5 and 6) IRQ interrupt and FIQ interrupt switches.
SPSR: Program status save register, SPSR saves the CPSR value of the previous working mode.
When an exception occurs, it will switch to the corresponding exception mode, and the ARM920T cpu core will automatically complete the following tasks. Note that it is completed automatically.
1. The address of the next instruction to be executed in the previous working mode is saved in the connection register R14 of the exception mode (R15->R14_xxx). For the ARM state, this value is the current pc value plus 4 or 8.
2. Copy the value of CPSR to the SPSR of the exception mode (CPSR->SPSR). (All modes share a CPSR, and each exception mode has its own SPSR to save the CPSR value of the previous mode).
3. Set the working mode bit of CPSR to the working mode corresponding to this exception mode.
4. Set the pc value to the address of this exception mode in the exception vector table, that is, jump to execute the corresponding instruction in the exception vector table. Conversely, to return to the previous working mode from the exception mode, the following things need to be done by software:
1. Subtract an appropriate value from R14 in exception mode and assign it to the pc register.
2. Copy the value of SPSR back to CPSR.
For the calculation method of the value of pc when exiting abnormally, see Table 9.1 p146 of Wei Dongshan's "Complete Manual for Embedded Linux Application Development".
Interrupt handling process:
1. The interrupt controller collects the interrupt signals sent by various peripherals and then tells the CPU.
2. The CPU saves the running environment of the current program and calls the interrupt service routine (ISR, Interrupt Service Rountine).
3. In the ISR, read the interrupt controller and the relevant registers of the peripheral to identify which interrupt it is and perform corresponding processing.
4. Clear the interrupt by reading and writing the relevant registers of the interrupt controller and peripherals.
5. Restore the operating environment and continue execution.
Purpose of each register:
SUBSRCPND: Indicates whether interrupts such as INT_RXD0 and INT_TXD0 have occurred.
INTSUBMSK: Mask the interrupt identified by the SUBSRCPND register.
SRCPND: Each bit is used to indicate whether an interrupt (or a type of interrupt) has occurred. There are two types of interrupts: interrupt request source controlled by SUBSRCPND/INTSUBMSK (with sub register) and interrupt request source controlled by SRCPND/INTMSK (without sub register).
INTMSK: Mask the interrupt identified by the SRCPND register.
INTMOD: When a bit is set to 1, the corresponding interrupt is set to FIQ. Only one bit can be set at the same time, and only one FIQ can be set.
PRIORITY: Priority arbiter. When multiple normal interrupts occur at the same time, the highest priority processing is found based on this register.
INTPND: The interrupt flag that the CPU is about to process. Only one of them can be set at a time. The ISR can use this bit to determine which interrupt has occurred.
INTOFFSET: Indicates which bit of INTPND is set to 1.
There are two types of interrupts, request_source (without sub register) and request_source (with USB register). These two types correspond to different mask registers (INTMSK and INTSUBMSK) and interrupt flag registers (SRCPND and SUBSRCPND).
If the triggered interrupt has a fast interrupt (IFQ) (the one with 1 in the INTMOD register corresponds to FIQ), the CPU will enter fast interrupt processing. If several IRQs are triggered at the same time, the one with the highest priority will be selected, and its corresponding bit in the INTPND register will be set to 1, and then the CPU will enter interrupt mode processing.
The interrupt service routine can determine the interrupt source by reading the INTPND register or the INTOFFSET register.
The priority is set in the PRIORITY register.
Interrupt usage:
(1) Set up the stack in interrupt and fast interrupt mode
(2) Prepare the interrupt handling function
1. Exception vector
Set the jump function when entering interrupt mode or fast interrupt mode in the exception vector table. Their exception vector addresses are 0x00000018 and 0x0000001c respectively.
2. Interrupt Service Routine (ISR)
The jump function finally calls the specific interrupt service function. For IRQ, read the value of the INTPND register or the INTOFFSET register to determine the interrupt source, and then handle it separately. For FIQ, no judgment is required.
3. Clear interrupts
It can be before calling the ISR or after the ISR, depending on whether it is nested.
Clearing order: 1 peripheral 2 SUBSRCPND (if used) corresponding bit in SRCPND 3 light INTPND corresponding bit.
(3) When entering or exiting interrupt mode or fast interrupt mode, it is necessary to save or restore the operating environment of the interrupted program.
1. For IRQ, the entry and exit codes are as follows:
sub lr,lr,#4 @Calculate the return address
stmdb sp!,{r0-r12,lr} @Save the used registers
......@Handle interrupt
ldmia sp!,{r0-r12,pc}^@interrupt return
@ ^ means assigning the value of spsr to cpsr
2. For FIQ, the entry and exit codes are as follows:
sub lr,lr #4 @Calculate the return address
stmdb sp!,{r0-r7,lr} @Save the used registers
…… @Handling fast interrupts
ldima sp!,{r0-r7,pc}^ @ ^ means assigning the value of spsr to cpsr
(4) Set up related peripherals according to specific interrupts. For example, for GPIO interrupts, you need to set the function of the corresponding pin to "external interrupt", set the interrupt trigger condition (low level trigger, high level trigger, falling edge trigger or rising edge trigger), etc. Some interrupts have their own mask registers, which also need to be enabled.
(5) For interrupts in "request sources (without sub-register)", set the corresponding bits in the INTSUBMSK register to 0.
(6) Determine how to use this interrupt: FIQ or IRQ.
If it is FIQ, set the corresponding bit in the INTMOD register to 1.
If it is an IRQ, the priority is set in the RIORITY register.
(7) If it is an IRQ, set the corresponding bit in the INTMSK register to 0 (IFQ is not controlled by the INTMSK register).
(8) Set the I-bit (for IRQ) or F-bit (for FIQ) in the CPSR register to 0 to enable IRQ or IFQ.
Interrupt example: On the JZ2440 development board, set the CPU pins connected to the four buttons K1-K4 to external interrupt functions. The main function of this program is an infinite loop that does nothing. The program's functions are completely driven by interrupts: when a button is pressed, the CPU calls its interrupt service program to light up the corresponding LED. Mainly look at head.S and interrupt.c
@******************************************************************************
@ File:head.S
@ Function: Initialize, set interrupt mode, management mode stack, and set interrupt processing function
@******************************************************************************
.extern main
.text
.global _start
_start:
@******************************************************************************
@ Exception vector. In this program, except for Reset and HandleIRQ, other exceptions are not used.
@******************************************************************************
b Reset
@ 0x04: vector address of undefined instruction abort mode
HandleUndef:
b HandleUndef
@ 0x08: The vector address of the management mode, enter this mode through the SWI instruction
HandleSWI:
b HandleSWI
@ 0x0c: vector address of the exception caused by instruction prefetch termination
HandlePrefetchAbort:
b HandlePrefetchAbort
@ 0x10: vector address of the exception caused by data access termination
HandleDataAbort:
b HandleDataAbort
@ 0x14: Reserved
HandleNotUsed:
b HandleNotUsed
@ 0x18: interrupt mode vector address
b HandleIRQ
@ 0x1c: vector address of fast interrupt mode
HandleFIQ:
b HandleFIQ
Reset:
ldr sp, =4096 @ Set the stack pointer. The following are all C functions. You need to set the stack before calling them.
bl disable_watch_dog @ Turn off WATCHDOG, otherwise the CPU will restart continuously
msr cpsr_c, #0xd2 @ Enter interrupt mode
ldr sp, =3072 @ Set interrupt mode stack pointer
msr cpsr_c, #0xd3 @ Enter management mode
ldr sp, =4096 @ Set the management mode stack pointer,
@ In fact, after reset, the CPU is in management mode.
@ The previous "ldr sp, =4096" performs the same function, this sentence can be omitted
bl init_led @ Initialize the GPIO pin of LED
bl init_irq @ Call interrupt initialization function in init.c
msr cpsr_c, #0x53 @ Set I-bit = 0, enable IRQ interrupt
ldr lr, =halt_loop @ Set the return address
ldr pc, =main @ Call main function
halt_loop:
b halt_loop
HandleIRQ:
sub lr, lr, #4 @ Calculate the return address
stmdb sp!, { r0-r12,lr } @ Save the used registers
@ Note that the sp at this time is the sp in interrupt mode
@ The initial value is 3072 set above
ldr lr, =int_return @ Set the return address after calling ISR, namely EINT_Handle function
ldr pc, =EINT_Handle @ Call interrupt service function in interrupt.c
int_return:
ldmia sp!, { r0-r12,pc }^ @ interrupt return, ^ means copy the value of spsr to cpsr
interrupt.c:
#include "s3c24xx.h"
void EINT_Handle()
{
unsigned long oft = INTOFFSET;
unsigned long val;
Previous article:Linux kernel interrupt subsystem (VI): ARM interrupt processing process
Next article:STM32CubeMX study notes [1] - First attempt to build a project
Recommended ReadingLatest update time:2024-11-15 14:07
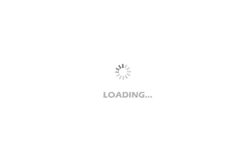
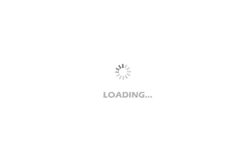
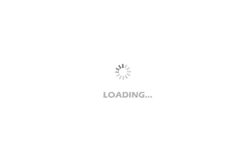
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- Do you know all the various motors commonly used in automotive electronics?
- What are the functions of the Internet of Vehicles? What are the uses and benefits of the Internet of Vehicles?
- Power Inverter - A critical safety system for electric vehicles
- Analysis of the information security mechanism of AUTOSAR, the automotive embedded software framework
- Brief Analysis of Automotive Ethernet Test Content and Test Methods
- How haptic technology can enhance driving safety
- Let’s talk about the “Three Musketeers” of radar in autonomous driving
- Why software-defined vehicles transform cars from tools into living spaces
- How Lucid is overtaking Tesla with smaller motors
- Wi-Fi 8 specification is on the way: 2.4/5/6GHz triple-band operation
- Problems with creating sheet symbols for multi-page schematics
- Zigbee Z-Stack 3.0.1 Modify channels using broadcasting
- The STM32 FFT library calculates the amplitude normally, but the phase is different each time. Has anyone encountered this problem?
- EEWORLD University----UCD3138 Analog Front End (AFE) Module
- "Show goods" to come to a wave of commonly used development boards
- Measurement of the phase difference between a sine wave and a square wave
- [Sipeed LicheeRV 86 Panel Review] - 6 waft-ui component tests (3)
- Hardware System Engineer's Handbook
- Integrated operational amplifier practical circuit diagram
- For electronic hardware, there are many circuit structures, which can be said to be the units that make up a component. All circuits...