The schematic diagram of the wireless transmission circuit based on the nrfl2401 chip is as follows:
During the experiment, both the sender and the receiver used the same circuit.
The sender program is as follows:
org 0000H
AJMP START
; Delay 1/4s subroutine
YANSHI1S: MOV R7,#250
YANSHI1S1: MOV R6,#250
YANSHI1S2: NOP
NOP
DJNZ R6,YANSHI1S2
DJNZ R7,YANSHI1S1
RET
; Write single or multiple bytes starting with 58 (the number of bytes is in R3) into the chip
XIENB: MOV R0,#58H
CLR P1.7 ; SCN becomes low
XIE00: ACALL XIE1B
INC R0
DJNZ R3,XIE00
SETB P1.7
RET
;;Write the unit 1 pointed to by R0 into the module according to the SPI timing. Before calling this subroutine, the CSN line should be turned low
; after single or multiple calls, the CSN line will be turned high
XIE1B: MOV R2,#8
MOV A,@R0
XIE1B1: RLC A
MOV P1.5,C ;Send data to MOSI line
SETB P1.4 ;Move data into module
CLR P1.4
DJNZ R2,XIE1B1
RET
;Read chip status word Read chip status word to 5FH
DUZT: MOV R2,#8
SETB P1.5
CLR P1.7 ;CSN turns low,
DUZT1: SETB P1.4 ;Clock rises
MOV C,P1.3 ;Read data on MISO line
MOV A,5FH
RLC A ;Move data into 5FH register
MOV 5FH,A
CLR P1.4
DJNZ R2,DUZT1
SETB P1.7 ;CSN becomes high, completing a command
RET
START: MOV P1,#0AFH ;Module standby
MOV 58H,#20H ;Prepare to write register 0
MOV 59H,#0EH ;Power on, transmission mode
MOV R3,#02H
ACALL XIENB
MOV 58H,#21H ;01 register
MOV 59H,#03H ;0,1 channel allows automatic response
MOV R3,#02H
ACALL XIENB
MOV 58H,#22H ;02
MOV 59H,#03H
MOV R3,#02H
ACALL XIENB
MOV 58H,#23H ;03 register
MOV 59H,#03H ;5-byte address broadband
MOV R3,#02H
ACALL XIENB
MOV 58H,#24H ;04
MOV 59H,#14H ;Retransmit wait 500uS, retransmit 4 times, 1A retransmit 10 times, at 206c
MOV R3,#02H
ACALL XIENB
MOV 58H,#25H ;05 register
MOV 59H,#07H ;RF frequency (at 2076 after assembly)
MOV R3,#02H
ACALL XIENB
MOV 58H,#26H ;06
MOV 59H,#27H ;07 is 1M transmission rate, 0dB gain, 27 is 250k transmission rate
MOV R3,#02H
ACALL XIENB
MOV 58H,#27H ;07
MOV 59H,#70H ; Clear interrupts in the module
MOV R3,#02H
ACALL XIENB
MOV 58H,#2AH ; 0A register (channel 0)
MOV 59H,#02H ; Configuration address
MOV 5AH,#3AH
MOV 5BH,#39H
MOV 5CH,#38H
MOV 5DH,#37H
MOV R3,#06H
ACALL XIENB
MOV 58H,#30H ; 10 register
MOV R3,#06H ; Send address
ACALL XIENB
MOV 58H,#2BH ; 0B register (channel 1)
MOV 59H,#01H ; Local address
MOV R3,#06H
ACALL XIENB
MOV 58H,#31H ; 11 register
MOV 59H,#10H ; 0 channel effective data bandwidth 16 bytes
MOV R3,#02H
ACALL XIENB
MOV 58H,#32H ;12 registers
MOV 59H,#10H ;1 channel effective data width 16 bytes
MOV R3,#02H
ACALL XIENB
XIEFS: MOV 58H,#0A0H ;Write and send data to chip
MOV R0,#58H
MOV R3,#10H
CLR P1.7
ACALL XIE1B
MOV R0,#70H
XIEXUN: ACALL XIE1B
INC R0
DJNZ R3,XIEXUN
SETB P1.7
SETB P1.6 ;Start transmission
MOV R7,#5
DJNZ R7,$
CLR P1.6
JB P3.2,$
cpl p1.0
ACALL DUZT ;Read status register
MOV 58H,#27H ;07
MOV 59H,#70H ;Clear interrupt
MOV R3,#02H
ACALL XIENB
MOV 58H,#0E1H ;Clear module send buffer
MOV R3,#01H
ACALL XIENB
ACALL YANSHI1S
SJMP XIEFS
end
Receiving end program:
org 0000H
AJMP START
org 0003H
AJMP EXINT0
;Delay 1/4s subroutine
YANSHI1S: MOV R7,#250
YANSHI1S1: MOV R6,#250
YANSHI1S2: NOP
NOP
DJNZ R6,YANSHI1S2
DJNZ R7,YANSHI1S1
RET
;Write the single or multiple bytes starting from 58 (the number of bytes is in R3) into the chip
XIENB: MOV R0,#58H
CLR P1.7 ;SCN becomes low
XIE00: ACALL XIE1B
INC R0
DJNZ R3,XIE00
SETB P1.7
RET
;;Write the unit 1 pointed to by R0 into the module according to the SPI timing. Before calling this subroutine, the CSN line should be low
; after single or multiple calls, the CSN line will be high
XIE1B: MOV R2,#8
MOV A,@R0
XIE1B1: RLC A
MOV P1.5,C ;Send data to MOSI line
SETB P1.4 ;Move data into module
CLR P1.4
DJNZ R2,XIE1B1
RET
;Read one byte of the module into the unit pointed to by R0 of the microcontroller according to the SPI timing. Before calling this subroutine, the CSN line should be changed to low
; after single or multiple calls, the CSN line will be changed to high
DU1B: MOV R2,#8
DU1B1: SETB P1.4
MOV C,P1.3
RLC A
CLR P1.4
DJNZ R2,DU1B1
MOV @R0,A
RET
;Read the module receive buffer data into the unit pointed to by R0 of the microcontroller according to the SPI timing, the number of bytes is in R3
DUNB: PUSH 00H
MOV 58H,#61H ;Write one byte command
MOV R0,#58H
CLR P1.7
ACALL XIE1B
POP 00H ;R0 points to the first address of the receiving buffer
DUXUN: ACALL DU1B
INC R0
DJNZ R3,DUXUN
SETB P1.7
RET
;Read chip status word Read chip status word to 5FH
DUZT: MOV R2,#8
SETB P1.5 ;Input chip 1
CLR P1.7 ;CSN becomes low,
DUZT1: SETB P1.4 ;Clock rises
MOV C,P1.3 ;Read data on MISO line
MOV A,5FH
RLC A ;Move data into 5FH register
MOV 5FH,A
CLR P1.4
DJNZ R2,DUZT1
SETB P1.7 ;CSN becomes high, complete a command
RET
...
Previous article:51 single chip microcomputer automatic water vending machine
Next article:Distance detection based on single chip microcomputer and ultrasonic ranging module
Recommended ReadingLatest update time:2024-11-15 13:41
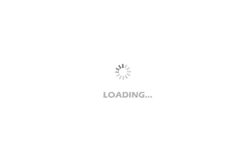
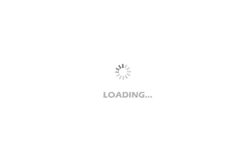
- Popular Resources
- Popular amplifiers
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Fundamentals and Applications of Single Chip Microcomputers (Edited by Zhang Liguang and Chen Zhongxiao)
-
Single chip microcomputer control technology (Li Shuping, Wang Yan, Zhu Yu, Zhang Xiaoyun)
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- How Lucid is overtaking Tesla with smaller motors
- Wi-Fi 8 specification is on the way: 2.4/5/6GHz triple-band operation
- Wi-Fi 8 specification is on the way: 2.4/5/6GHz triple-band operation
- Vietnam's chip packaging and testing business is growing, and supply-side fragmentation is splitting the market
- Vietnam's chip packaging and testing business is growing, and supply-side fragmentation is splitting the market
- Three steps to govern hybrid multicloud environments
- Three steps to govern hybrid multicloud environments
- Microchip Accelerates Real-Time Edge AI Deployment with NVIDIA Holoscan Platform
- Microchip Accelerates Real-Time Edge AI Deployment with NVIDIA Holoscan Platform
- Melexis launches ultra-low power automotive contactless micro-power switch chip
- Please, administrator, please restore the ability to invite 8 people. I will no longer suggest pressing the Enter key to automatically query.
- Develop the Internet of Things in 10 minutes: Remote formaldehyde monitoring (4G module)
- 【TouchGFX Design】Use TouchGFX and IAR interactive development based on C language
- Xunwei 4412 development board Qt_for_Android
- SensorTile.box Bluetooth connection
- What toys have electrician dads made for their cute kids? Put down your phone and play with the toys your dad has customized for you.
- Infineon Position2Go Development Kit Review @1. Unboxing
- 【Perf-V Review】LED Dot Matrix
- 【EVK-NINA-B400 Evaluation Kit】+Unboxing
- EEWORLD University Hall----Live Replay: Fluke's new 8.5-digit digital multimeter technology development and application