1. First of all, let me answer a question: Can STM32 achieve low power consumption?
The answer is yes, and there is data to support this. When I tested the STM32101CB, FLASH: 128K, RAM: 16K and RTC working, the power consumption was 16uA, which is quite good.
2. What should I pay attention to when it comes to STM32 low power consumption?
When I first tested that the STM32 power consumption was 16uA, I was very happy and thought it could really be used for my application. My application is to make the MCU wake up regularly to work, work for a while and then go to sleep. The working time may be only a few dozen milliseconds. But later I found some problems (working in STOP mode):
1) Clock problem: After the STM32 is awakened, the clock automatically switches to the internal HIS RC oscillator. As we all know, the accuracy of the RC oscillator is not high. Moreover, the clock settings before going to bed are all restored to the reset state, only the clock is reset, and the others are not. This will also bring a problem. Maybe you use the internal clock before going to bed, but after going to bed, the clock changes, and the problem is UART and timer. Maybe you don't want to use PLL, that is, 8M, so that the clock HIS after waking up is also 8M. In this way, although there is no difference in the clock, the clock is unstable. The UART baud rate must not be too high, otherwise there will be communication problems.
2) Wake-up time: This is also a very big problem. The wake-up time given in the datasheet is 7us, which may be true, but after waking up, you can't do your work immediately. Why? Initialize IO. You may ask, can't I not initialize it? The answer should be no. Because if you want to use low power consumption, the IO ports should be set to analog input before going to bed, so that the 14uA on the datasheet can be achieved, but this also brings a problem, that is, initialize IO. You must initialize IO when you wake up. If you also want to switch the clock to an external clock, it will take even longer, close to 200ms, because the STM32 will wait for the external clock to stabilize before it can work, and then reinitialize all IO, which is very time-consuming. Maybe I only need to wake up for 10ms, but it takes 100ms to complete these tasks.
3) RTC wake-up: RTC is also a problem, why? Everyone needs to pay attention that RTC can only use alarm to wake up the MCU, and the second interrupt cannot wake up. And the alarm interrupt must be set continuously, and it will only take effect once set. After the interrupt is over, you need to set the time of the next interrupt. And there is another problem. The alarm interrupt must wait until the second interrupt arrives before it can be set, that is, it is set when the second register is updated once. This brings a problem, waiting for the second interrupt. If you want to be awakened by the alarm before going to bed, you must reset the alarm interrupt, and when setting the alarm interrupt, you need to wait until the second interrupt to set the new value. The waiting time is uncertain. It may be hundreds of milliseconds. So it takes hundreds of milliseconds to wait for the second interrupt flag to set the alarm interrupt. Maybe my MCU only needs to execute 10ms before it needs to go to sleep. It still takes hundreds of milliseconds to waste.
Summary: I have explained above the problems I found during use. I think the low power consumption of STM32 is too fake. Although the performance is good when asleep, the settings for waking up and entering sleep are too troublesome and time-consuming. This is a disadvantage. I think MSP430 is probably the best. Even AVR is better than it and is not so troublesome.
After getting the STM32L sample, I have been struggling with the low power consumption test. Because the configuration is different from the STM32F series, it led to a tragedy. After constant consultation with ST, I finally got the final result.
After testing, the power consumption is 500nA in STOP mode, and the performance is still good. The code is as follows:
/**
* @brief Main program.
* @param None
* @retval None
*/
int main(void)
{
/*!< At this stage the microcontroller clock setting is already configured,
this is done through SystemInit() function which is called from startup
file (startup_stm32l1xx_md.s) before to branch to application main.
To reconfigure the default setting of SystemInit() function, refer to
system_stm32l1xx.c file
*/
/* Configure all unused GPIO port pins in Analog Input mode (floating input
trigger OFF), this will reduce the power consumption and increase the device
immunity against EMI/EMC *****************************************************/
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOA | RCC_AHBPeriph_GPIOB | RCC_AHBPeriph_GPIOC
| RCC_AHBPeriph_GPIOD | RCC_AHBPeriph_GPIOE | RCC_AHBPeriph_GPIOH, ENABLE);
/* config all IO to Analog Input to reduce parasite power consumption */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_All;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_400KHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOC, &GPIO_InitStructure);
GPIO_Init(GPIOD, &GPIO_InitStructure);
GPIO_Init(GPIOE, &GPIO_InitStructure); GPIO_Init(GPIOH
, &GPIO_InitStructure);
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_Init(GPIOB, &GPIO_InitStructure);
/* Enable PB7 as external PVD input so as to set it as AIN_IN */
Set_PVD_To_Config_PB7();
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_GPIOA
| RCC_AHBPeriph_GPIOB
| RCC_AHBPeriph_GPIOC
| RCC_AHBPeriph_GPIOD
| RCC_AHBPeriph_GPIOE
| RCC_AHBPeriph_GPIOH, DISABLE);
PWR_UltraLowPowerCmd(ENABLE);
PWR_EnterSTOPMode(PWR_Regulator_LowPower, PWR_STOPEntry_WFI);
while(1);
}
/**
* @brief Enable PB7 as external PVD input so as to set it as AIN_IN
* @param None
* @retval None
*/
void Set_PVD_To_Config_PB7(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR, ENABLE);
/* Configure the PVD Level to 3 (2.5V) */
PWR_PVDLevelConfig(PWR_PVDLevel_7);
/* Enable the PVD Output */
PWR_PVDCmd(ENABLE);
}
Previous article:STM32L151C8 periodically wakes up from standby mode (RTC Wakeup Timer)
Next article:stm8L15x EEPORM study notes
Recommended ReadingLatest update time:2024-11-22 20:20
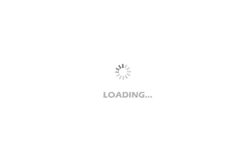
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Another technical solution for power-type plug-in hybrid: A brief discussion on Volvo T8 plug-in hybrid technology
- Invite you to make an appointment for TI live broadcast: Analysis of the classic and innovative TI ultrasonic gas flow meter solution + the latest SimpleLink platform wireless products
- 2017 MG6 smart key disassembly (NXP solution, with keyless entry, one-button start)
- Panasonic launches IC air gap interconnect technology to improve chip design performance
- [Sipeed LicheeRV 86 Panel Review] Review Project Summary Report - Desktop Calendar Weather Assistant
- 【AT-START-F425 Review】USB to CAN: Five Serial Port Commands Explained
- Evaluation board quick test - based on TI Sitara Cortex-A9(2)
- "Support Yatli, tell us your story with Yatli" Summary of netizens' sharing
- Qorvo PAC series highly integrated motor control chips and applications
- Electronics Competition Award-winning Work: Firefighting Flying Vehicle
- What are harmonics? The generation of harmonics and their hazards