I am currently studying how to use AVR to drive MAXIN's DS18B20. After a few days of thinking, I finally wrote its driver.
Using IAR C++ Compiler
#ifndef DS18B20_H
#define DS18B20_H
/****************************************Just define the following macros in the source program
#define seDDS() (seDB0())
#define clDDS() (clDB0())
#define seDS() (sePB0())
#define clDS() (clPB0())
#define GetDS() (GetPB0())
*********************************************/
/*********************************** Function Description *********************
void DS18B20::DS18B20(void) Constructor
void DS18B20::init() Initialize DS18B20
char DS18B20::GetID() Read the device ID. Return 1 if successful, and the 64-bit ID number of the device is saved in DS18B20.id[4]. Return 0 if failed.
char DS18B20::convter() reads the temperature and returns 1 if successful, and the temperature value is saved in DS18B20.temp. If not ready, it returns 0. This program needs to be called several times to convert correctly.
The calling cycle must not be less than 2ms.
char DS18B20::GetPower() Reads the bus power mode. Normally it returns 1, and parasitic returns 0.
char DS18B20::SearchAlarm() Reads the alarm. It returns 1 if there is an alarm, and 0 if there is no alarm.
****************************************************/
#define DS_ReadROM 0x33
#define DS_CopyROM 0x48
#define DS_MatchROM 0x55
#define DS_SkipROM 0xCC
#define DS_SearchROM 0xF0
#define DS_AlarmSearch 0xEC
#define DS_Convert 0x44
#define DS_ReadRAM 0xBE
#define DS_WriteRAM 0x4E #define DS_ReadPower
0xB4
#define DS_9BIT 0
#define DS_10BIT 1
#define DS_11BIT 2
#define DS_12BIT 3
#include "c:incstatus.h"
#include "c:inccrc8.c"
#include "c:incdelay.c"
#include "c:incformat.h"
#include
class DS18B20
{
public:
float temp;// temperature
int rel;// temperature offset
char id[8];// device ID
char mode;// conversion mode 9-12bit
unsigned char TH;// warning temperature upper limit
unsigned char TL;// warning temperature lower limit
char power;// 0: parasitic power supply 1: power supply
char status;// single-wire bus status 0: not connected 1: connected
char sbuf[10];
private:
char setup;
/********************************************Reset bus***********************/
char Reset_DS()
{
unsigned int x;
seDS();
seDDS();
DELAY_80us();
if(GetDS())
{
clDS();
DELAY_600us();
seDS();
clDDS();
for(x=0;x<30;)
{
if(GetDS())x++;
else
{
for(x=0;x<30;)
{
if(GetDS())
{
seDDS();
DELAY_600us();//
return 1;
}
else
x++;
DELAY_10us();
}
clDDS();
return 0;
}
DELAY_10us();//
}
}
clDDS();
return 0;
}
/******************************************************Write bus*******************/
void Write_DS(unsigned dat,unsigned char wide)// dat: data wide: data width (number of bits)
{
unsigned char i,sreg;
seDDS();
for(i=0;i
sreg=SREG;
SREG&=0x7F;// turn off interrupt
if(dat&0x01)
{
clDS();
DELAY_10us();
seDS();
DELAY_80us();
}
else
{
clDS();
DELAY_80us();
seDS();
DELAY_10us();
}
SREG=sreg;// Resume interrupt The interrupt that occurred will be executed in sequence
dat>>=1;
}
}
/*******************************************************Read bus*******************/
unsigned char Read_DS(unsigned char wide)
{
unsigned char dat,i,sreg;
for(i=dat=0;i
if(GetDS())
{
sreg=SREG;
SREG&=0x7F;
clDS();
seDDS();
DELAY_10us();
seDS();
clDDS();
dat>>=1;
DELAY_10us();
if(GetDS())dat|=0x80;
seDDS();
SREG=sreg;
DELAY_80us();
}
else
{
setup=0;
}
}
return dat;
}
char getid()
{
char i;
for(i=0;i
return 1;
return 0;
}
public:
char convert()// The calling cycle of this program is at least 2ms or above
{
static unsigned char sbuf[9];
static unsigned int i;
switch(setup)
{
case 0:
if(Reset_DS())// Start
{
status=1;
setup++;
}
else
status=0;
break;
case 1:
if(getid())
{
Write_DS(DS_MatchROM,8);// Match ROM
for(i=0;i<8;i++)
Write_DS(id,8);
}
else
{
Write_DS(DS_SkipROM,8);// Skip match
}
Write_DS(DS_Convert,8);// Start conversion
i=power?0:500;// Select power mode to achieve the fastest speed
setup++;
break;
case 2:
if(i)// Delay
{
i--;
}
else if(Read_DS(8)==0xFF)// Wait for conversion to end
{
Reset_DS();
if(getid())
{
Write_DS(DS_MatchROM,8);// Match ROM
for(i=0;i<8;i++)
Write_DS(id,8);
}
else
Write_DS(DS_SkipROM,8);
Write_DS(DS_ReadRAM,8);
i=0;
setup++;
}
break;
case 3:
sbuf=Read_DS(8);
if(i>=9)
{
Reset_DS();
if(GetCRC8(sbuf,8)==sbuf[8])// Check CRC
{
int i;
i=sbuf[1]*0x100+sbuf[0];
i+=rel;
temp=i/16+(i%16)*0.125;
setup=0;
status=1;
return 1;
}
setup=0;
}
break;
}
return 0;
}
char GetPower()
{
unsigned char x;
if(Reset_DS())
{
Write_DS(DS_SkipROM,8);
Write_DS(DS_ReadPower,8);
x=Read_DS(8);
Reset_DS();
if(x)
return 1;
}
return 0;
}
/*********************************************Alarm search****************************/
char SearchAlarm()
{
if(Reset_DS())
{
Write_DS(DS_AlarmSearch,8);
if(Read_DS(2)==0x80)// Read two bits
{
Reset_DS();
return 1;
}
}
Reset_DS();
return 0;
}
//TH: high temperature limit TL: low temperature limit
//mode: conversion mode 0:9bit(93.75ms) 1:10bit(187.5ms) 2:11bit(375ms) 3:12bit(750ms)
//power: bus power mode
//ID: device ID number
char init()
{
unsigned char i,sbuf[9];
if(Reset_DS())
{
if(getid())
{
Write_DS(DS_MatchROM,8);
for(i=0;i<8;i++)
Write_DS(id,8);
}
else
Write_DS(DS_SkipROM,8);
Write_DS(DS_WriteRAM,8);
Write_DS(TH,8);
Write_DS(TL,8);
Write_DS(((mode&0x03)<<5)|0x1F,8);
Reset_DS();
if(getid())
{
Write_DS(DS_MatchROM,8);
for(i=0;i<8;i++)
Write_DS(id,8);
}
else
Write_DS(DS_SkipROM,8);
Write_DS(DS_ReadRAM,8);
for(i=0;i<9;i++)
sbuf=Read_DS(8);
if(GetCRC8(sbuf,8)==sbuf[8])// 校验CRC
{
if(sbuf[2]==TH&&sbuf[3]==TL)
{
Reset_DS();
if(getid())
{
Write_DS(DS_MatchROM,8);
for(i=0;i<8;i++)
Write_DS(id,8);
}
else
Write_DS(DS_SkipROM,8);
Write_DS(DS_CopyROM,8);
DELAY_200ms();
Reset_DS();
power=GetPower();
Reset_DS();
return 1;// 完成
}
}
}
return 0;
}
/*********************************************读取DS18B20的64位ROM******************/
char GetID()
{
unsigned char i;
unsigned char sbuf[8];
if(Reset_DS())
{
Write_DS(DS_ReadROM,8);
for(i=0;i<8;i++)
{
sbuf=Read_DS(8);
}
Reset_DS();
if(GetCRC8(sbuf,7)==sbuf[7])// 校验CRC
{
for(i=0;i<8;i++)
id=sbuf;// 拷贝数据到ROM
return 1;
}
}
return 0;
}
char* disp(char width=0)
{
int i=(int)temp;
int j=(int)(temp*10);
j%=10;
if(temp<0)
j=0-j;
switch(width)
{
case 2:
sprintf(sbuf,"%2d.%d",i,j);
break;
case 3:
sprintf(sbuf,"%3d.%d",i,j);
break;
default:
sprintf(sbuf,"%d.%d",i,j);
break;
}
return sbuf;
}
DS18B20()
{
format(id,sizeof(id),0);
mode=DS_12BIT;
TH=100;
TL=0;
power=0;
rel=0;
status=0;
setup=0;
seDDS();
seDS();
}
};
#endif
Previous article:Detailed explanation of ds1302 clock program for avr microcontroller
Next article:Phase Corrected PWM Mode Setting for AVR Timer 1
Recommended ReadingLatest update time:2024-11-16 09:18
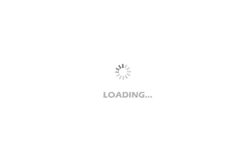
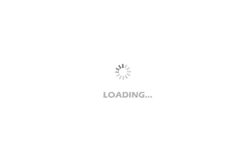
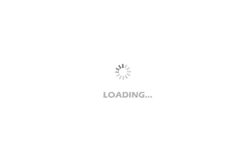
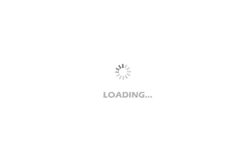
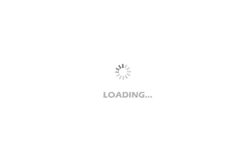
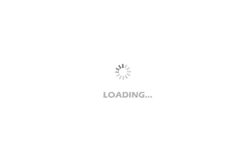
- Popular Resources
- Popular amplifiers
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
Modern Product Design Guide
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Getting Started with Smart Home Audio Design
- Power Density Basics Technical Brief
- Competition Sharing 2: RSL10 Bluetooth SoC BHI160 Sensor Data Collection
- The following is the basic principle block diagram of LDO. I don’t quite understand how to achieve constant voltage?
- Can this drive circuit work at 5KHZ?
- SHT31 Review + First Prototyping Expansion Board
- FPGA Introduction Series 4--Assignment Statement
- An almost brand new Tencent OS Tiny IoT development board is released
- Chip Manufacturing 4-Semiconductor Cleaning
- STM32 uart configuration