Pin Diagram
Pin 1: GND, ground terminal; Pin 2: VCC, 1.9V~3.6V
Pin 3: CE, NRF24L01 mode control terminal
Pin 4: CSN, chip select signal
Pin 5: SCK, SPI clock input
Pin 6: MOSI, SPI data input
Pin 7: MISO, SPI data output
Pin 8: IRQ, interrupt output terminal, low level enable, that is, interrupt output low level
Do not exceed 3.6V for the power supply, otherwise the chip may be damaged.
For port pins, they can withstand 5V, that is, they can be connected to TTL ports
Sending Side
#include #define uchar unsigned char #define TX_ADR_WIDTH 5 // 5-byte width of the transmit/receive address #define TX_PLOAD_WIDTH 4 // Data channel effective data width uchar code TX_ADDRESS[TX_ADR_WIDTH] = {0x34,0x43,0x10,0x10,0x01}; // Define a static send address uchar RX_BUF[TX_PLOAD_WIDTH]; uchar TX_BUF[TX_PLOAD_WIDTH]; uchar flag; float DATA = 0x01; float bdata sta; sbit RX_DR = sta^6; sbit TX_DS = sta^5; sbit MAX_RT = sta^4; sbit CE = P1^5; sbit CSN= P1^4; sbit SCK= P1^3; sbit MOSI= P1^2; sbit MISO= P1^1; sbit IRQ = P1^0; // SPI(nRF24L01) commands #define READ_REG 0x00 // Define read command to register #define WRITE_REG 0x20 // Define write command to register #define RD_RX_PLOAD 0x61 // Define RX payload register address #define WR_TX_PLOAD 0xA0 // Define TX payload register address #define FLUSH_TX 0xE1 // Define flush TX register command #define FLUSH_RX 0xE2 // Define flush RX register command #define REUSE_TX_PL 0xE3 // Define reuse TX payload register command #define NOP 0xFF // Define No Operation, might be used to read status register // SPI(nRF24L01) registers(addresses) #define CONFIG 0x00 // 'Config' register address #define EN_AA 0x01 // 'Enable Auto Acknowledgment' register address #define EN_RXADDR 0x02 // 'Enabled RX addresses' register address #define SETUP_AW 0x03 // 'Setup address width' register address #define SETUP_RETR 0x04 // 'Setup Auto. Retrans' register address #define RF_CH 0x05 // 'RF channel' register address #define RF_SETUP 0x06 // 'RF setup' register address #define STATUS 0x07 // 'Status' register address #define OBSERVE_TX 0x08 // 'Observe TX' register address #define CD 0x09 // 'Carrier Detect' register address #define RX_ADDR_P0 0x0A // 'RX address pipe0' register address #define RX_ADDR_P1 0x0B // 'RX address pipe1' register address #define RX_ADDR_P2 0x0C // 'RX address pipe2' register address #define RX_ADDR_P3 0x0D // 'RX address pipe3' register address #define RX_ADDR_P4 0x0E // 'RX address pipe4' register address #define RX_ADDR_P5 0x0F // 'RX address pipe5' register address #define TX_ADDR 0x10 // 'TX address' register address #define RX_PW_P0 0x11 // 'RX payload width, pipe0' register address #define RX_PW_P1 0x12 // 'RX payload width, pipe1' register address #define RX_PW_P2 0x13 // 'RX payload width, pipe2' register address #define RX_PW_P3 0x14 // 'RX payload width, pipe3' register address #define RX_PW_P4 0x15 // 'RX payload width, pipe4' register address #define RX_PW_P5 0x16 // 'RX payload width, pipe5' register address #define FIFO_STATUS 0x17 // 'FIFO Status Register' register address /************************************************** Function: init_io() describe: Initialize IO /**************************************************/ void init_io(void) { CE = 0; // Standby CSN = 1; // SPI disabled SCK = 0; // SPI clock low IRQ = 1; // Interrupt reset LED = 1; // Turn off the indicator light } /**************************************************/ /************************************************** Function: delay_ms() describe: Delay x milliseconds /**************************************************/ void delay_ms(uchar x) { flying i, j; i = 0; for(i=0; i j = 250; while(--j); j = 250; while(--j); } } /**************************************************/ /************************************************** Function: SPI_RW() describe: According to the SPI protocol, write one byte of data to nRF24L01 and Read one byte /**************************************************/ volatile SPI_RW(volatile byte) { flying i; for(i=0; i<8; i++) // loop 8 times { MOSI = (byte & 0x80); // byte highest bit is output to MOSI byte <<= 1; // shift the lower bit to the highest bit SCK = 1; // Pull SCK high, nRF24L01 reads 1 bit of data from MOSI and outputs 1 bit of data from MISO byte |= MISO; // Read MISO to the lowest bit of byte SCK = 0; // SCK is set low } return(byte); // Returns the read byte } /**************************************************/ /************************************************** Function: SPI_RW_Reg() describe: Write data value to register reg /**************************************************/ uchar SPI_RW_Reg(uchar reg, uchar value) { flying status; CSN = 0; // Set CSN to low and start transmitting data status = SPI_RW(reg); // Select register and return status word SPI_RW(value); // Then write data to this register CSN = 1; // Pull CSN high to end data transmission return(status); // Return status register } /**************************************************/ /************************************************** Function: SPI_Read() describe: Read a byte from register reg /**************************************************/ fly SPI_Read(fly reg) { fly reg_val; CSN = 0; // Set CSN to low and start transmitting data SPI_RW(reg); //Select register reg_val = SPI_RW(0); // Then read data from this register CSN = 1; // Pull CSN high to end data transmission return(reg_val); // Return register data } /**************************************************/ /************************************************** Function: SPI_Read_Buf() describe: Read bytes from the reg register, usually used to read the receiving channel Data or receiving/sending address /**************************************************/ uchar SPI_Read_Buf(uchar reg, uchar * pBuf, uchar bytes) { flying status, i; CSN = 0; // Set CSN to low and start transmitting data status = SPI_RW(reg); // Select register and return status word for(i=0; i CSN = 1; // Pull CSN high to end data transmission return(status); // Return status register } /**************************************************/ /************************************************** Function: SPI_Write_Buf() describe: Write the data in the pBuf buffer to nRF24L01, usually used to write the send Receive/send channel data or address /**************************************************/ uchar SPI_Write_Buf(uchar reg, uchar * pBuf, uchar bytes) { flying status, i; CSN = 0; // Set CSN to low and start transmitting data status = SPI_RW(reg); // Select register and return status word for(i=0; i CSN = 1; // Pull CSN high to end data transmission return(status); // Return status register } /**************************************************/ /************************************************** Function: RX_Mode() describe: This function sets the nRF24L01 to receive mode and waits for the data packet to be received from the sending device. /**************************************************/ void RX_Mode(void) { CE = 0; SPI_Write_Buf(WRITE_REG + RX_ADDR_P0, TX_ADDRESS, TX_ADR_WIDTH); // The receiving device receiving channel 0 uses the same transmit address as the transmitting device SPI_RW_Reg(WRITE_REG + EN_AA, 0x01); // Enable automatic response of receiving channel 0 SPI_RW_Reg(WRITE_REG + EN_RXADDR, 0x01); // Enable receive channel 0 SPI_RW_Reg(WRITE_REG + RF_CH, 40); // Select RF channel 0x40 SPI_RW_Reg(WRITE_REG + RX_PW_P0, TX_PLOAD_WIDTH); // Receive channel 0 selects the same valid data width as the transmit channel SPI_RW_Reg(WRITE_REG + RF_SETUP, 0x07); // Data rate 1Mbps, transmit power 0dBm, low noise amplifier gain SPI_RW_Reg(WRITE_REG + CONFIG, 0x0f); // CRC enable, 16-bit CRC check, power on, receive mode delay_ms(150); CE = 1; // Pull up CE to start the receiving device } /**************************************************/ /************************************************** Function: TX_Mode() describe: This function sets nRF24L01 to transmit mode (CE=1 for at least 10us). After 130us, the transmission starts. After the data transmission is completed, the sending module automatically switches to receiving. Mode waits for response signal. /**************************************************/ void TX_Mode(uchar * BUF) { CE = 0; SPI_Write_Buf(WRITE_REG + TX_ADDR, TX_ADDRESS, TX_ADR_WIDTH); // Write transmit address SPI_Write_Buf(WRITE_REG + RX_ADDR_P0, TX_ADDRESS, TX_ADR_WIDTH); // To respond to the receiving device, the receiving channel 0 address is the same as the sending address SPI_Write_Buf(WR_TX_PLOAD, BUF, TX_PLOAD_WIDTH); // Write data packet to TX FIFO SPI_RW_Reg(WRITE_REG + EN_AA, 0x01); // Enable automatic response of receiving channel 0 SPI_RW_Reg(WRITE_REG + EN_RXADDR, 0x01); // Enable receive channel 0 SPI_RW_Reg(WRITE_REG + SETUP_RETR, 0x0a); // Automatic resend delay wait 250us+86us, automatic resend 10 times SPI_RW_Reg(WRITE_REG + RF_CH, 40); // Select RF channel 0x40 SPI_RW_Reg(WRITE_REG + RF_SETUP, 0x07); // Data rate 1Mbps, transmit power 0dBm, low noise amplifier gain SPI_RW_Reg(WRITE_REG + CONFIG, 0x0e); // CRC enable, 16-bit CRC check, power on delay_ms(150); CE = 1; } /**************************************************/ /************************************************** Function: Check_ACK() describe: Check whether the receiving device has received the data packet, and set it to Is the number resent? /**************************************************/ uchar Check_ACK(bit clear)
Previous article:51 single chip matrix keyboard
Next article:51 single chip microcomputer LCD1602 display
Recommended ReadingLatest update time:2024-11-15 08:00
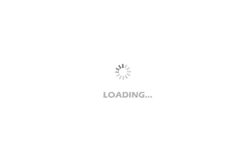
- Popular Resources
- Popular amplifiers
- Learn ARM development(16)
- Learn ARM development(17)
- Learn ARM development(18)
- Embedded system debugging simulation tool
- A small question that has been bothering me recently has finally been solved~~
- Learn ARM development (1)
- Learn ARM development (2)
- Learn ARM development (4)
- Learn ARM development (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- LED chemical incompatibility test to see which chemicals LEDs can be used with
- Application of ARM9 hardware coprocessor on WinCE embedded motherboard
- What are the key points for selecting rotor flowmeter?
- LM317 high power charger circuit
- A brief analysis of Embest's application and development of embedded medical devices
- Single-phase RC protection circuit
- stm32 PVD programmable voltage monitor
- Introduction and measurement of edge trigger and level trigger of 51 single chip microcomputer
- Improved design of Linux system software shell protection technology
- What to do if the ABB robot protection device stops
- CGD and Qorvo to jointly revolutionize motor control solutions
- CGD and Qorvo to jointly revolutionize motor control solutions
- Keysight Technologies FieldFox handheld analyzer with VDI spread spectrum module to achieve millimeter wave analysis function
- Infineon's PASCO2V15 XENSIV PAS CO2 5V Sensor Now Available at Mouser for Accurate CO2 Level Measurement
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- Advanced gameplay, Harting takes your PCB board connection to a new level!
- A new chapter in Great Wall Motors R&D: solid-state battery technology leads the future
- Naxin Micro provides full-scenario GaN driver IC solutions
- Interpreting Huawei’s new solid-state battery patent, will it challenge CATL in 2030?
- Are pure electric/plug-in hybrid vehicles going crazy? A Chinese company has launched the world's first -40℃ dischargeable hybrid battery that is not afraid of cold
- Application of millimeter wave radar technology in barriers
- (Bonus 3) GD32L233 Review - Unboxing and showing off the big box
- CPLD
- Help, after the load power supply has been working for a period of time, the microcontroller will shut down automatically
- The silk screen is AA6B SOT23-6 package with 2.2UH inductor 3.7~4.2V boost to 5V
- Add uart6 driver
- How fast can electric vehicles be “fast charged”?
- 5G millimeter wave RF algorithm technology
- Share NFC universal card reader circuit diagram
- SAM-L10-L11 series Questions and Answers