The STM32F407ZET6 is used. The four pins of the controlled LED lights are LED0 -> PF9, LED1 -> PF10, LED2-> PE13, LED3 -> PE14. The four pins of the buttons are KEY0--> PA0, KEY1--> PE2, KEY2--> PE3, KEY3--> PE4.
In the loop, determine whether the button is pressed (the corresponding pin input will become 0). If it is pressed, the corresponding LED pin will output a low level and light up.
1. Initialize the registers of LED lights and buttons respectively.
Initialize the LED light (set various registers): set the GPIO clock, configure the mode register (general output type), configure the output type (set to output push), set the register that controls the output rate, and finally configure the output data register (let the LED light be off by default)
Initialize the buttons (set various registers): set the GPIO clock, configure the key register (set to input mode), and set the register that controls the output rate.
2. In the main function, keep checking whether the button is pressed (whether the corresponding pin level is 0)
The function is implemented as follows:
#define rRCCAHB1CLKEN *((volatile unsigned long *)0x40023830)
#define rGPIOF_MODER *((volatile unsigned long *)0x40021400)
#define rGPIOF_OTYPER *((volatile unsigned long *)0x40021404)
#define rGPIOF_OSPEEDR *((volatile unsigned long *)0x40021408)
#define rGPIOF_IDR *((volatile unsigned long *)0x40021410)
#define rGPIOF_ODR *((volatile unsigned long *)0x40021414)
#define rGPIOE_MODER *((volatile unsigned long *)0x40021000)
#define rGPIOE_OTYPER *((volatile unsigned long *)0x40021004)
#define rGPIOE_OSPEEDR *((volatile unsigned long *)0x40021008)
#define rGPIOE_IDR *((volatile unsigned long *)0x40021010)
#define rGPIOE_ODR *((volatile unsigned long *)0x40021014)
#define rGPIOA_MODER *((volatile unsigned long *)0x40020000)
#define rGPIOA_OTYPER *((volatile unsigned long *)0x40020004)
#define rGPIOA_OSPEEDR *((volatile unsigned long *)0x40020008)
#define rGPIOA_IDR *((volatile unsigned long *)0x40020010)
#define rGPIOA_ODR *((volatile unsigned long *)0x40020014)
void key_init()
{
rRCCAHB1CLKEN |= 1 | (1 << 1);
rGPIOA_MODER&=~(1|(1<<1));
rGPIOF_OSPEEDR &= ~(1 | (1 << 1) );
rGPIOE_MODER&= ~(0x3f<<4);
rGPIOE_MODER &= ~(0x3f<<4);
}
void led_init()
{
rRCCAHB1CLKEN |= (1 << 5) | (1 << 4);
rGPIOF_MODER &= ~((0x3 << 18) | (0x3 << 20));
rGPIOF_MODER |= (1 << 18) | (1 << 20);
rGPIOF_OTYPER &= ~( (1 << 9) | (1 << 10));
rGPIOF_OSPEEDR &= ~((0x3 << 18) | (0x3 << 20) );
rGPIOF_ODR |= (1 << 9 | 1 << 10) ;
rGPIOE_MODER &= ~((0X3 << 26) | (0X3 << 28) );
rGPIOE_MODER |= (1 << 26) | (1 << 28);
rGPIOE_OTYPER &= ~( (1 << 13) | (1 << 14));
rGPIOE_OSPEEDR &= ~((0x3 << 26) | (0x3 << 28) );
rGPIOE_ODR |= (1 << 13 | 1 << 14) ;
}
void delay(int i)
{
int v = i;
while(v--);
}
void led_on(int i)
{
if (i == 0)
{
rGPIOF_ODR &= ~(1 << 9);
rGPIOF_ODR |= 1 << 10;
rGPIOE_ODR |= (1 << 13) | (1 << 14);
}
else if (i == 1)
{
rGPIOF_ODR |= (1 << 9);
rGPIOF_ODR &= ~(1 << 10);
rGPIOE_ODR |= (1 << 13) | (1 << 14);
}
else if (i == 2)
{
rGPIOF_ODR |= (1 << 9) | (1 << 10);
rGPIOE_ODR &= ~(1 << 13);
rGPIOE_ODR |= 1 << 14;
}
else if (i == 3)
{
rGPIOF_ODR |= (1 << 9) | (1 << 10);
rGPIOE_ODR &= ~(1 << 14);
rGPIOE_ODR |= 1 << 13;
}
}
int main()
{
int i = 0;
led_heat();
key_init();
while(1)
{
if(!(rGPIOA_IDR&1))
{
delay(50); //debounce
if(!(rGPIOA_IDR&1))
{
led_on(0);
}
}
else
{
rGPIOF_ODR |= 1 << 9;//µÆÃð
}
if(!(rGPIOE_IDR&(1<<2)))
{
delay(50);
if(!(rGPIOE_IDR&(1<<2)))
{
led_on(1);
}
}
else
{
rGPIOF_ODR |= 1 << 10;
}
if(!(rGPIOE_IDR&(1<<3)))
{
delay(50);
if(!(rGPIOE_IDR&(1<<3)))
{
led_on(2);
}
}
else
{
rGPIOE_ODR |= 1 << 13;
}
if(!(rGPIOE_IDR&(1<<4)))
{
delay(50);
if(!(rGPIOE_IDR&(1<<4)))
{
led_on(3);
}
}
else
{
rGPIOE_ODR |= 1 << 14;
}
}
}
Previous article:STM32-1-LED on and off
Next article:STM32 serial communication--data packaging and sending
Recommended ReadingLatest update time:2024-11-23 02:34
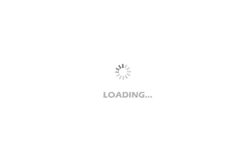
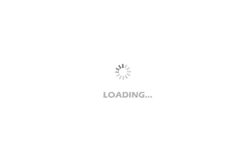
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- CCS project loading and debugging
- How did you electronic design engineers grow up?
- Whenever there is a heavy rainstorm, I face frequent network outages.
- An FPGA frequency multiplier problem
- [Review of Arteli Development Board AT32F421] 2. Read the MCU model
- TE official WeChat manual customer service function is now online. You can communicate directly with TE technical experts via WeChat!
- Building Boa web server under Ubuntu
- CH549EVT development board test - driving LCD5110 display
- EEWORLD University ---- Live playback: The most important component of the analog world - Signal chain and power supply: Interface special
- EEWORLD University Hall----Live Replay: ADI Digital Active Noise Cancelling Headphone Solution