The 8051 microcontroller can directly read and write a certain IO bit, and the Cortex-M3's bit-banding operation is an enhanced version of the 8051 bit addressing area. After using the bit-banding operation, you can use ordinary load/store instructions to read and write a single bit.
1. Related concepts
Bit-band region: An address region that supports bit-band operations.
Bit-band alias area: The access to the alias address ultimately affects the access to the bit-band area. The access to the bit-band alias area has an address mapping process.
2. Principle of bit-band operation
The ultimate goal of bit-banding operation is to perform independent read and write operations on the bits in the bit-band area, but it is achieved by operating on the bit-band alias area.
The specific process is as follows:
The bit-band alias area is accessed for reading and writing, the bit-band alias area is mapped to the corresponding bit-band area through an address mapping relationship, and the original bit reading and writing operations are performed on the bit-band area.
3. Address Mapping
The above briefly introduces the bit-band operation. So which addresses support the bit-band operation? What are their corresponding bit-band alias area addresses? What is the address mapping relationship between the two?
1. Addresses that support bit-band operations
The Cortex-M3 has two regions that support bit-banding operations. In addition to being used like normal RAM, these two regions can also be operated through bit-banding alias regions. The two regions (bit-banding regions) are:
The lowest 1MB in the SRAM area: 0x2000 0000 - 0x200F FFFF
The lowest 1MB of on-chip peripherals: 0x4000 0000 - 0x400F FFFF
The bit-band alias regions corresponding to these two bit-band regions are:
The bit-band alias area corresponding to the lowest 1MB bit-band area in the SRAM area is:
The lowest 1MB bit-band area in the on-chip peripherals corresponds to the bit-band alias area:
2. Address mapping relationship between bit-band area and bit-band alias area
The bit-band alias area expands each bit in the bit-band area into a 32-bit word, that is, each bit in the bit-band area corresponds to a 4-byte address in the bit-band alias area.
The following figure shows the expansion correspondence between the lowest 1MB bit-band area and the bit-band alias area in the SRAM area:
Calculation formula:
For a certain bit in the SRAM bit band area, let its byte address be A and the bit number be n (0<=n<=7), then the address of the bit band alias area corresponding to the bit is:
For a certain bit in the on-chip peripheral bit-band area, let its byte address be A and the bit number be n (0<=n<=7), then the address of the bit-band alias area corresponding to the bit is:
Note: "*4" means a word is 4 bytes, and "*8" means a byte has 8 bits.
4. Mechanism of read and write operations
In the bit-band area, although each bit is mapped to a word in the alias area, only the LSB (least significant bit) of the word in the alias area is valid, so the read and write operations are performed on the LSB of the word in the alias area, and the value of the LSB is 0 or 1.
1. Reading process:
for example:
Read the value of the second bit of SRAM address 0x2000 0000:
2. Writing process: read, modify, write
for example:
Set the second bit of SRAM address 0x2000 0000 to 1:
5. Bit-band operation programming implementation
There is no direct support for bit-banding in the C compiler. For example, the C compiler does not know that the same memory block can be accessed with different addresses, nor does it know that access to a bit-band aliased area is only valid for the LSB. To use bit-banding in C, the simplest way is to #define the address of a bit-band aliased area.
1. Macro definition of bit-band operation
To simplify bit-band operations, we can propose a macro that converts "bit-band address + bit number" to an alias address, and then create a macro that converts the alias address to a pointer type:
#define BITBAND(addr, bitnum) ((addr & 0xF0000000)+0x2000000+((addr &0xFFFFF)<<5)+(bitnum<<2)) /* Macro to convert "bit band address + bit number" to alias address */
#define MEM_ADDR(addr) *((volatile unsigned long *)(addr)) /* Macro to convert alias address to pointer type */
#define BIT_ADDR(addr, bitnum) MEM_ADDR(BITBAND(addr, bitnum)) /* Macro for bit-band operation of a certain bit at a certain address */
2. Give an example
The following uses the GPIOA->ODR register (address 0x40020014) of the STM32F407 as an example to read and write through bit-band operations, and compares it with the traditional reading and writing methods, and sends the information to the console for display through the serial port. The code is as follows:
Header file definition:
#define BITBAND(addr, bitnum) ((addr & 0xF0000000)+0x2000000+((addr &0xFFFFF)<<5)+(bitnum<<2))
#define MEM_ADDR(addr) *((volatile unsigned long *)(addr))
#define BIT_ADDR(addr, bitnum) MEM_ADDR(BITBAND(addr, bitnum))
//IO port address mapping
#define GPIOA_ODR_Addr (GPIOA_BASE+20) //0x40020014
#define PAout(n) BIT_ADDR(GPIOA_ODR_Addr,n)
main function:
int main(void)
{
u8 Temp;
u16 Data;
Data = 0;
Stm32_Clock_Init(336,8,2,7); //Initialize the clock to 168Mhz
delay_init(168); //Initialize delay function
uart_init(84,115200); //Serial port initialized to 115200
printf("init finished\r\n");
GPIOA->ODR = 0xffff; /* Traditional writing method: write 1 to each bit of GPIOA->ODR */
printf("Tratidional operate: GPIOA->ODR[0x%x]:0x%x\r\n", &GPIOA->ODR, GPIOA->ODR);
GPIOA->ODR = 0x0; /* Clear GPIOA->ODR*/
printf("Clear GPIOA->ODR: GPIOA->ODR[0x%x]:0x%x\r\n", &GPIOA->ODR, GPIOA->ODR);
for(Temp = 0; Temp < 16; Temp++)
{
PAout(Temp) = 1; /* Bit-band write operation: write GPIOA->ODR to 1 via the bit-band alias area */
}
printf("Bit-band write: GPIOA->ODR[0x%x]:0x%x\r\n", &GPIOA->ODR, GPIOA->ODR); /* You can see the value of each bit of GPIOA->ODR after the bit-band operation*/
for(Temp = 0; Temp < 16; Temp++)
{
Data |= (PAout(Temp) << Temp); /* Bit-band read operation: read the value of each bit of GPIOA->ODR through the bit-band alias area and store it in Data*/
}
printf("Bit-band read: GPIOA->ODR[0x%x]:0x%x\r\n", &GPIOA->ODR, Data); /* You can see the value of the alias area corresponding to each bit of GPIOA->ODR after the bit-band operation is read*/
while(1)
{
}
return 1;
}
Information printed by the serial port:
This article is mainly written with reference to the following materials:
Chapter 5 of "CM3 Definitive Guide" (pages 87-92)
"STM32F4xx Chinese Reference Manual"
Previous article:STM32 timer output PWM mode
Next article:STM32 bitband operation summary
Recommended ReadingLatest update time:2024-11-22 12:16
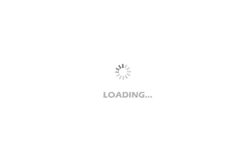
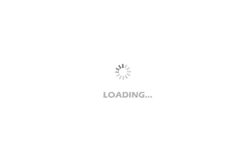
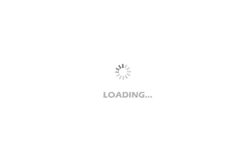
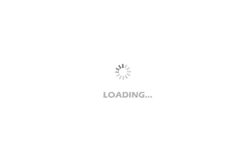
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- Vicor high-performance power modules enable the development of low-altitude avionics and EVTOL
- Chuangshi Technology's first appearance at electronica 2024: accelerating the overseas expansion of domestic distributors
- Chuangshi Technology's first appearance at electronica 2024: accelerating the overseas expansion of domestic distributors
- "Cross-chip" quantum entanglement helps build more powerful quantum computing capabilities
- Ultrasound patch can continuously and noninvasively monitor blood pressure
- Ultrasound patch can continuously and noninvasively monitor blood pressure
- Europe's three largest chip giants re-examine their supply chains
- Europe's three largest chip giants re-examine their supply chains
- Breaking through the intelligent competition, Changan Automobile opens the "God's perspective"
- The world's first fully digital chassis, looking forward to the debut of the U7 PHEV and EV versions
- I was badly hurt by "RZ7888"!!!
- I feel good today
- Review of STM32's "useless" RAM debugging
- The meaning of two and a half dimensions in RF simulation
- How to select rectifier modules for communication power systems
- Rohde & Schwarz launches combined spectrum analyzer and phase noise tester
- [Starting at 13:30 pm] TI wireless connectivity product series application design guidance, online hands-on demo
- Inductance and Q value of transformer
- MSP430 ADC analog-to-digital routines
- Shenying series SY-Y1000 intelligent partition long-range laser perimeter warning radar